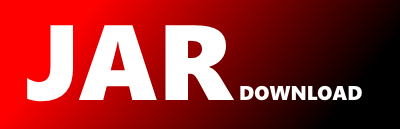
com.citrix.sharefile.api.models.SFReseller Maven / Gradle / Ivy
// ------------------------------------------------------------------------------
//
// This code was generated by a tool.
//
// Changes to this file may cause incorrect behavior and will be lost if
// the code is regenerated.
//
// Copyright (c) 2017 Citrix ShareFile. All rights reserved.
//
// ------------------------------------------------------------------------------
package com.citrix.sharefile.api.models;
import java.io.InputStream;
import java.util.ArrayList;
import java.net.URI;
import java.util.Date;
import java.util.Map;
import java.util.HashMap;
import com.google.gson.annotations.SerializedName;
import com.citrix.sharefile.api.*;
import com.citrix.sharefile.api.enumerations.*;
import com.citrix.sharefile.api.models.*;
public class SFReseller extends SFODataObject {
@SerializedName("CompanyName")
private String CompanyName;
@SerializedName("CorporateOrganization")
private String CorporateOrganization;
@SerializedName("CheckTo")
private String CheckTo;
@SerializedName("FirstName")
private String FirstName;
@SerializedName("LastName")
private String LastName;
@SerializedName("Email")
private String Email;
@SerializedName("Phone")
private String Phone;
@SerializedName("CompanyUrl")
private String CompanyUrl;
@SerializedName("Address1")
private String Address1;
@SerializedName("Address2")
private String Address2;
@SerializedName("City")
private String City;
@SerializedName("State")
private String State;
@SerializedName("Zip")
private String Zip;
@SerializedName("Country")
private String Country;
@SerializedName("ResellerType")
private String ResellerType;
@SerializedName("CreationDate")
private Date CreationDate;
@SerializedName("DemoAccountId")
private String DemoAccountId;
@SerializedName("AccountBalance")
private Double AccountBalance;
@SerializedName("DefaultShowBillingInfo")
private Boolean DefaultShowBillingInfo;
@SerializedName("Note")
private String Note;
@SerializedName("ResellerAccountType")
private String ResellerAccountType;
public String getCompanyName() {
return this.CompanyName;
}
public void setCompanyName(String companyname) {
this.CompanyName = companyname;
}
public String getCorporateOrganization() {
return this.CorporateOrganization;
}
public void setCorporateOrganization(String corporateorganization) {
this.CorporateOrganization = corporateorganization;
}
public String getCheckTo() {
return this.CheckTo;
}
public void setCheckTo(String checkto) {
this.CheckTo = checkto;
}
public String getFirstName() {
return this.FirstName;
}
public void setFirstName(String firstname) {
this.FirstName = firstname;
}
public String getLastName() {
return this.LastName;
}
public void setLastName(String lastname) {
this.LastName = lastname;
}
public String getEmail() {
return this.Email;
}
public void setEmail(String email) {
this.Email = email;
}
public String getPhone() {
return this.Phone;
}
public void setPhone(String phone) {
this.Phone = phone;
}
public String getCompanyUrl() {
return this.CompanyUrl;
}
public void setCompanyUrl(String companyurl) {
this.CompanyUrl = companyurl;
}
public String getAddress1() {
return this.Address1;
}
public void setAddress1(String address1) {
this.Address1 = address1;
}
public String getAddress2() {
return this.Address2;
}
public void setAddress2(String address2) {
this.Address2 = address2;
}
public String getCity() {
return this.City;
}
public void setCity(String city) {
this.City = city;
}
public String getState() {
return this.State;
}
public void setState(String state) {
this.State = state;
}
public String getZip() {
return this.Zip;
}
public void setZip(String zip) {
this.Zip = zip;
}
public String getCountry() {
return this.Country;
}
public void setCountry(String country) {
this.Country = country;
}
public String getResellerType() {
return this.ResellerType;
}
public void setResellerType(String resellertype) {
this.ResellerType = resellertype;
}
public Date getCreationDate() {
return this.CreationDate;
}
public void setCreationDate(Date creationdate) {
this.CreationDate = creationdate;
}
public String getDemoAccountId() {
return this.DemoAccountId;
}
public void setDemoAccountId(String demoaccountid) {
this.DemoAccountId = demoaccountid;
}
public Double getAccountBalance() {
return this.AccountBalance;
}
public void setAccountBalance(Double accountbalance) {
this.AccountBalance = accountbalance;
}
public Boolean getDefaultShowBillingInfo() {
return this.DefaultShowBillingInfo;
}
public void setDefaultShowBillingInfo(Boolean defaultshowbillinginfo) {
this.DefaultShowBillingInfo = defaultshowbillinginfo;
}
public String getNote() {
return this.Note;
}
public void setNote(String note) {
this.Note = note;
}
public String getResellerAccountType() {
return this.ResellerAccountType;
}
public void setResellerAccountType(String reselleraccounttype) {
this.ResellerAccountType = reselleraccounttype;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy