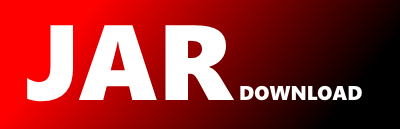
com.citrix.sharefile.api.models.SFSFObjectItemStatus Maven / Gradle / Ivy
// ------------------------------------------------------------------------------
//
// This code was generated by a tool.
//
// Changes to this file may cause incorrect behavior and will be lost if
// the code is regenerated.
//
// Copyright (c) 2017 Citrix ShareFile. All rights reserved.
//
// ------------------------------------------------------------------------------
package com.citrix.sharefile.api.models;
import java.io.InputStream;
import java.util.ArrayList;
import java.net.URI;
import java.util.Date;
import java.util.Map;
import java.util.HashMap;
import com.google.gson.annotations.SerializedName;
import com.citrix.sharefile.api.*;
import com.citrix.sharefile.api.enumerations.*;
import com.citrix.sharefile.api.models.*;
public class SFSFObjectItemStatus extends SFODataObject {
@SerializedName("SFObjectId")
private String SFObjectId;
@SerializedName("ObjectType")
private SFSafeEnum ObjectType;
@SerializedName("Status")
private SFItemStatus Status;
@SerializedName("ExternalReferenceId")
private String ExternalReferenceId;
@SerializedName("ObjectInfo")
private String ObjectInfo;
@SerializedName("CreatedBy")
private SFUser CreatedBy;
@SerializedName("CreatedDate")
private Date CreatedDate;
@SerializedName("UpdatedBy")
private SFUser UpdatedBy;
@SerializedName("UpdatedDate")
private Date UpdatedDate;
/**
* The Id of the ShareFile object
*/
public String getSFObjectId() {
return this.SFObjectId;
}
/**
* The Id of the ShareFile object
*/
public void setSFObjectId(String sfobjectid) {
this.SFObjectId = sfobjectid;
}
/**
* The type of the ShareFile object
*/
public SFSafeEnum getObjectType() {
return this.ObjectType;
}
/**
* The type of the ShareFile object
*/
public void setObjectType(SFSafeEnum objecttype) {
this.ObjectType = objecttype;
}
/**
* The external party's status for the ShareFile object
*/
public SFItemStatus getStatus() {
return this.Status;
}
/**
* The external party's status for the ShareFile object
*/
public void setStatus(SFItemStatus status) {
this.Status = status;
}
/**
* An Id generated by the external party for mapping to their own data store
*/
public String getExternalReferenceId() {
return this.ExternalReferenceId;
}
/**
* An Id generated by the external party for mapping to their own data store
*/
public void setExternalReferenceId(String externalreferenceid) {
this.ExternalReferenceId = externalreferenceid;
}
/**
* Additional information for this status binding
*/
public String getObjectInfo() {
return this.ObjectInfo;
}
/**
* Additional information for this status binding
*/
public void setObjectInfo(String objectinfo) {
this.ObjectInfo = objectinfo;
}
public SFUser getCreatedBy() {
return this.CreatedBy;
}
public void setCreatedBy(SFUser createdby) {
this.CreatedBy = createdby;
}
public Date getCreatedDate() {
return this.CreatedDate;
}
public void setCreatedDate(Date createddate) {
this.CreatedDate = createddate;
}
public SFUser getUpdatedBy() {
return this.UpdatedBy;
}
public void setUpdatedBy(SFUser updatedby) {
this.UpdatedBy = updatedby;
}
public Date getUpdatedDate() {
return this.UpdatedDate;
}
public void setUpdatedDate(Date updateddate) {
this.UpdatedDate = updateddate;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy