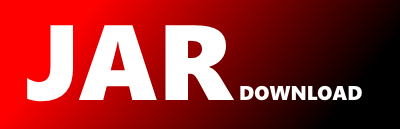
com.citrix.sharefile.api.models.SFSearchResult Maven / Gradle / Ivy
// ------------------------------------------------------------------------------
//
// This code was generated by a tool.
//
// Changes to this file may cause incorrect behavior and will be lost if
// the code is regenerated.
//
// Copyright (c) 2017 Citrix ShareFile. All rights reserved.
//
// ------------------------------------------------------------------------------
package com.citrix.sharefile.api.models;
import java.io.InputStream;
import java.util.ArrayList;
import java.net.URI;
import java.util.Date;
import java.util.Map;
import java.util.HashMap;
import com.google.gson.annotations.SerializedName;
import com.citrix.sharefile.api.*;
import com.citrix.sharefile.api.enumerations.*;
import com.citrix.sharefile.api.models.*;
public class SFSearchResult extends SFODataObject {
@SerializedName("Rank")
private Integer Rank;
@SerializedName("Score")
private Double Score;
@SerializedName("ItemID")
private String ItemID;
@SerializedName("ParentID")
private String ParentID;
@SerializedName("ParentName")
private String ParentName;
@SerializedName("ItemType")
private String ItemType;
@SerializedName("FileName")
private String FileName;
@SerializedName("DisplayName")
private String DisplayName;
@SerializedName("Size")
private Long Size;
@SerializedName("CreatorID")
private String CreatorID;
@SerializedName("CreatorName")
private String CreatorName;
@SerializedName("CreatorFirstName")
private String CreatorFirstName;
@SerializedName("CreatorLastName")
private String CreatorLastName;
@SerializedName("CreationDate")
private Date CreationDate;
@SerializedName("Details")
private String Details;
@SerializedName("MD5")
private String MD5;
@SerializedName("PreviewStatus")
private Integer PreviewStatus;
@SerializedName("VirusStatus")
private Integer VirusStatus;
@SerializedName("Url")
private String Url;
@SerializedName("CanDownload")
private Boolean CanDownload;
@SerializedName("CanView")
private Boolean CanView;
@SerializedName("CanDelete")
private Boolean CanDelete;
@SerializedName("ParentSemanticPath")
private String ParentSemanticPath;
@SerializedName("Path")
private String Path;
@SerializedName("StreamID")
private String StreamID;
@SerializedName("AccountID")
private String AccountID;
public Integer getRank() {
return this.Rank;
}
public void setRank(Integer rank) {
this.Rank = rank;
}
public Double getScore() {
return this.Score;
}
public void setScore(Double score) {
this.Score = score;
}
public String getItemID() {
return this.ItemID;
}
public void setItemID(String itemid) {
this.ItemID = itemid;
}
public String getParentID() {
return this.ParentID;
}
public void setParentID(String parentid) {
this.ParentID = parentid;
}
public String getParentName() {
return this.ParentName;
}
public void setParentName(String parentname) {
this.ParentName = parentname;
}
public String getItemType() {
return this.ItemType;
}
public void setItemType(String itemtype) {
this.ItemType = itemtype;
}
public String getFileName() {
return this.FileName;
}
public void setFileName(String filename) {
this.FileName = filename;
}
public String getDisplayName() {
return this.DisplayName;
}
public void setDisplayName(String displayname) {
this.DisplayName = displayname;
}
public Long getSize() {
return this.Size;
}
public void setSize(Long size) {
this.Size = size;
}
public String getCreatorID() {
return this.CreatorID;
}
public void setCreatorID(String creatorid) {
this.CreatorID = creatorid;
}
public String getCreatorName() {
return this.CreatorName;
}
public void setCreatorName(String creatorname) {
this.CreatorName = creatorname;
}
public String getCreatorFirstName() {
return this.CreatorFirstName;
}
public void setCreatorFirstName(String creatorfirstname) {
this.CreatorFirstName = creatorfirstname;
}
public String getCreatorLastName() {
return this.CreatorLastName;
}
public void setCreatorLastName(String creatorlastname) {
this.CreatorLastName = creatorlastname;
}
public Date getCreationDate() {
return this.CreationDate;
}
public void setCreationDate(Date creationdate) {
this.CreationDate = creationdate;
}
public String getDetails() {
return this.Details;
}
public void setDetails(String details) {
this.Details = details;
}
public String getMD5() {
return this.MD5;
}
public void setMD5(String md5) {
this.MD5 = md5;
}
public Integer getPreviewStatus() {
return this.PreviewStatus;
}
public void setPreviewStatus(Integer previewstatus) {
this.PreviewStatus = previewstatus;
}
public Integer getVirusStatus() {
return this.VirusStatus;
}
public void setVirusStatus(Integer virusstatus) {
this.VirusStatus = virusstatus;
}
public String getUrl() {
return this.Url;
}
public void setUrl(String url) {
this.Url = url;
}
public Boolean getCanDownload() {
return this.CanDownload;
}
public void setCanDownload(Boolean candownload) {
this.CanDownload = candownload;
}
public Boolean getCanView() {
return this.CanView;
}
public void setCanView(Boolean canview) {
this.CanView = canview;
}
public Boolean getCanDelete() {
return this.CanDelete;
}
public void setCanDelete(Boolean candelete) {
this.CanDelete = candelete;
}
public String getParentSemanticPath() {
return this.ParentSemanticPath;
}
public void setParentSemanticPath(String parentsemanticpath) {
this.ParentSemanticPath = parentsemanticpath;
}
public String getPath() {
return this.Path;
}
public void setPath(String path) {
this.Path = path;
}
public String getStreamID() {
return this.StreamID;
}
public void setStreamID(String streamid) {
this.StreamID = streamid;
}
public String getAccountID() {
return this.AccountID;
}
public void setAccountID(String accountid) {
this.AccountID = accountid;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy