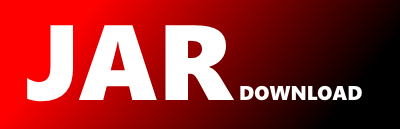
com.citrix.sharefile.api.models.SFToolSurvey Maven / Gradle / Ivy
// ------------------------------------------------------------------------------
//
// This code was generated by a tool.
//
// Changes to this file may cause incorrect behavior and will be lost if
// the code is regenerated.
//
// Copyright (c) 2017 Citrix ShareFile. All rights reserved.
//
// ------------------------------------------------------------------------------
package com.citrix.sharefile.api.models;
import java.io.InputStream;
import java.util.ArrayList;
import java.net.URI;
import java.util.Date;
import java.util.Map;
import java.util.HashMap;
import com.google.gson.annotations.SerializedName;
import com.citrix.sharefile.api.*;
import com.citrix.sharefile.api.enumerations.*;
import com.citrix.sharefile.api.models.*;
public class SFToolSurvey extends SFODataObject {
@SerializedName("SurveyURL")
private String SurveyURL;
@SerializedName("AppCode")
private SFSafeEnum AppCode;
@SerializedName("SurveyStartDate")
private Date SurveyStartDate;
@SerializedName("SurveyEndDate")
private Date SurveyEndDate;
@SerializedName("PercentUsersToDisplayTo")
private Double PercentUsersToDisplayTo;
@SerializedName("MaximumNumberOfResponses")
private Integer MaximumNumberOfResponses;
@SerializedName("CurrentNumberOfResponses")
private Integer CurrentNumberOfResponses;
@SerializedName("MinimumNumberOfDaysToSurveyUserAgain")
private Integer MinimumNumberOfDaysToSurveyUserAgain;
/**
* The location of the survey to display.
*/
public String getSurveyURL() {
return this.SurveyURL;
}
/**
* The location of the survey to display.
*/
public void setSurveyURL(String surveyurl) {
this.SurveyURL = surveyurl;
}
/**
* The tool whose users are being surveyed.
*/
public SFSafeEnum getAppCode() {
return this.AppCode;
}
/**
* The tool whose users are being surveyed.
*/
public void setAppCode(SFSafeEnum appcode) {
this.AppCode = appcode;
}
/**
* The beginning of the survey period.
*/
public Date getSurveyStartDate() {
return this.SurveyStartDate;
}
/**
* The beginning of the survey period.
*/
public void setSurveyStartDate(Date surveystartdate) {
this.SurveyStartDate = surveystartdate;
}
/**
* The end of the survey period. If not specified, the survey closes when CurrentNumberOfResponses == MaximumNumberOfResponses.
*/
public Date getSurveyEndDate() {
return this.SurveyEndDate;
}
/**
* The end of the survey period. If not specified, the survey closes when CurrentNumberOfResponses == MaximumNumberOfResponses.
*/
public void setSurveyEndDate(Date surveyenddate) {
this.SurveyEndDate = surveyenddate;
}
/**
* The percentage of tool instances that should display this survey on a single day.
* Value is a percentage, ex. "14.5%" is 14.5, not .145.
*/
public Double getPercentUsersToDisplayTo() {
return this.PercentUsersToDisplayTo;
}
/**
* The percentage of tool instances that should display this survey on a single day.
* Value is a percentage, ex. "14.5%" is 14.5, not .145.
*/
public void setPercentUsersToDisplayTo(Double percentuserstodisplayto) {
this.PercentUsersToDisplayTo = percentuserstodisplayto;
}
/**
* The number of survey responses after which the survey period is completed.
*/
public Integer getMaximumNumberOfResponses() {
return this.MaximumNumberOfResponses;
}
/**
* The number of survey responses after which the survey period is completed.
*/
public void setMaximumNumberOfResponses(Integer maximumnumberofresponses) {
this.MaximumNumberOfResponses = maximumnumberofresponses;
}
/**
* The number of survey responses received to date.
*/
public Integer getCurrentNumberOfResponses() {
return this.CurrentNumberOfResponses;
}
/**
* The number of survey responses received to date.
*/
public void setCurrentNumberOfResponses(Integer currentnumberofresponses) {
this.CurrentNumberOfResponses = currentnumberofresponses;
}
/**
* If a user has completed a survey within this number of days, they should not be prompted with this survey.
*/
public Integer getMinimumNumberOfDaysToSurveyUserAgain() {
return this.MinimumNumberOfDaysToSurveyUserAgain;
}
/**
* If a user has completed a survey within this number of days, they should not be prompted with this survey.
*/
public void setMinimumNumberOfDaysToSurveyUserAgain(Integer minimumnumberofdaystosurveyuseragain) {
this.MinimumNumberOfDaysToSurveyUserAgain = minimumnumberofdaystosurveyuseragain;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy