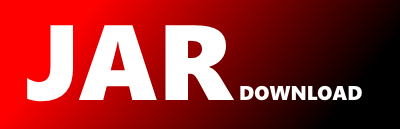
com.citrix.sharefile.api.models.SFUploadRequestParams Maven / Gradle / Ivy
// ------------------------------------------------------------------------------
//
// This code was generated by a tool.
//
// Changes to this file may cause incorrect behavior and will be lost if
// the code is regenerated.
//
// Copyright (c) 2017 Citrix ShareFile. All rights reserved.
//
// ------------------------------------------------------------------------------
package com.citrix.sharefile.api.models;
import java.io.InputStream;
import java.util.ArrayList;
import java.net.URI;
import java.util.Date;
import java.util.Map;
import java.util.HashMap;
import com.google.gson.annotations.SerializedName;
import com.citrix.sharefile.api.*;
import com.citrix.sharefile.api.enumerations.*;
import com.citrix.sharefile.api.models.*;
public class SFUploadRequestParams extends SFODataObject {
@SerializedName("Method")
private SFSafeEnum Method;
@SerializedName("Raw")
private Boolean Raw;
@SerializedName("FileName")
private String FileName;
@SerializedName("FileSize")
private Long FileSize;
@SerializedName("BatchId")
private String BatchId;
@SerializedName("BatchLast")
private Boolean BatchLast;
@SerializedName("CanResume")
private Boolean CanResume;
@SerializedName("StartOver")
private Boolean StartOver;
@SerializedName("Unzip")
private Boolean Unzip;
@SerializedName("Overwrite")
private Boolean Overwrite;
@SerializedName("Opid")
private String Opid;
@SerializedName("Title")
private String Title;
@SerializedName("Tool")
private String Tool;
@SerializedName("Details")
private String Details;
@SerializedName("IsSend")
private Boolean IsSend;
@SerializedName("SendGuid")
private String SendGuid;
@SerializedName("Notify")
private Boolean Notify;
@SerializedName("ThreadCount")
private Integer ThreadCount;
@SerializedName("ClientCreatedDate")
private Date ClientCreatedDate;
@SerializedName("ClientModifiedDate")
private Date ClientModifiedDate;
@SerializedName("BaseFileId")
private String BaseFileId;
public SFSafeEnum getMethod() {
return this.Method;
}
public void setMethod(SFSafeEnum method) {
this.Method = method;
}
public Boolean getRaw() {
return this.Raw;
}
public void setRaw(Boolean raw) {
this.Raw = raw;
}
public String getFileName() {
return this.FileName;
}
public void setFileName(String filename) {
this.FileName = filename;
}
public Long getFileSize() {
return this.FileSize;
}
public void setFileSize(Long filesize) {
this.FileSize = filesize;
}
public String getBatchId() {
return this.BatchId;
}
public void setBatchId(String batchid) {
this.BatchId = batchid;
}
public Boolean getBatchLast() {
return this.BatchLast;
}
public void setBatchLast(Boolean batchlast) {
this.BatchLast = batchlast;
}
public Boolean getCanResume() {
return this.CanResume;
}
public void setCanResume(Boolean canresume) {
this.CanResume = canresume;
}
public Boolean getStartOver() {
return this.StartOver;
}
public void setStartOver(Boolean startover) {
this.StartOver = startover;
}
public Boolean getUnzip() {
return this.Unzip;
}
public void setUnzip(Boolean unzip) {
this.Unzip = unzip;
}
public Boolean getOverwrite() {
return this.Overwrite;
}
public void setOverwrite(Boolean overwrite) {
this.Overwrite = overwrite;
}
public String getOpid() {
return this.Opid;
}
public void setOpid(String opid) {
this.Opid = opid;
}
public String getTitle() {
return this.Title;
}
public void setTitle(String title) {
this.Title = title;
}
public String getTool() {
return this.Tool;
}
public void setTool(String tool) {
this.Tool = tool;
}
public String getDetails() {
return this.Details;
}
public void setDetails(String details) {
this.Details = details;
}
public Boolean getIsSend() {
return this.IsSend;
}
public void setIsSend(Boolean issend) {
this.IsSend = issend;
}
public String getSendGuid() {
return this.SendGuid;
}
public void setSendGuid(String sendguid) {
this.SendGuid = sendguid;
}
public Boolean getNotify() {
return this.Notify;
}
public void setNotify(Boolean notify) {
this.Notify = notify;
}
public Integer getThreadCount() {
return this.ThreadCount;
}
public void setThreadCount(Integer threadcount) {
this.ThreadCount = threadcount;
}
public Date getClientCreatedDate() {
return this.ClientCreatedDate;
}
public void setClientCreatedDate(Date clientcreateddate) {
this.ClientCreatedDate = clientcreateddate;
}
public Date getClientModifiedDate() {
return this.ClientModifiedDate;
}
public void setClientModifiedDate(Date clientmodifieddate) {
this.ClientModifiedDate = clientmodifieddate;
}
/**
* BaseFileId is a used to check conflict in file during File Upload.
* BaseFileId is passed by client and contains value of their local copy itemId.
* API will check if the version passed is still current or someone else has updated file since clients last read.
*/
public String getBaseFileId() {
return this.BaseFileId;
}
/**
* BaseFileId is a used to check conflict in file during File Upload.
* BaseFileId is passed by client and contains value of their local copy itemId.
* API will check if the version passed is still current or someone else has updated file since clients last read.
*/
public void setBaseFileId(String basefileid) {
this.BaseFileId = basefileid;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy