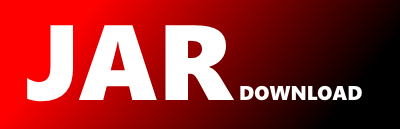
com.citrix.sharefile.api.models.SFUser Maven / Gradle / Ivy
// ------------------------------------------------------------------------------
//
// This code was generated by a tool.
//
// Changes to this file may cause incorrect behavior and will be lost if
// the code is regenerated.
//
// Copyright (c) 2017 Citrix ShareFile. All rights reserved.
//
// ------------------------------------------------------------------------------
package com.citrix.sharefile.api.models;
import java.io.InputStream;
import java.util.ArrayList;
import java.net.URI;
import java.util.Date;
import java.util.Map;
import java.util.HashMap;
import com.google.gson.annotations.SerializedName;
import com.citrix.sharefile.api.*;
import com.citrix.sharefile.api.enumerations.*;
import com.citrix.sharefile.api.models.*;
public class SFUser extends SFPrincipal {
@SerializedName("Account")
private SFAccount Account;
@SerializedName("Company")
private String Company;
@SerializedName("TotalSharedFiles")
private Integer TotalSharedFiles;
@SerializedName("Contacted")
private Integer Contacted;
@SerializedName("FullName")
private String FullName;
@SerializedName("ReferredBy")
private String ReferredBy;
@SerializedName("DefaultZone")
private SFZone DefaultZone;
@SerializedName("FirstName")
private String FirstName;
@SerializedName("LastName")
private String LastName;
@SerializedName("DateCreated")
private Date DateCreated;
@SerializedName("FullNameShort")
private String FullNameShort;
@SerializedName("Emails")
private ArrayList Emails;
@SerializedName("EmailAddresses")
private ArrayList EmailAddresses;
@SerializedName("IsConfirmed")
private Boolean IsConfirmed;
@SerializedName("Password")
private String Password;
@SerializedName("Preferences")
private SFUserPreferences Preferences;
@SerializedName("Security")
private SFUserSecurity Security;
@SerializedName("FavoriteFolders")
private ArrayList FavoriteFolders;
@SerializedName("HomeFolder")
private SFFolder HomeFolder;
@SerializedName("Devices")
private ArrayList Devices;
@SerializedName("Integrations")
private ArrayList> Integrations;
@SerializedName("VirtualRoot")
private SFFolder VirtualRoot;
@SerializedName("Roles")
private ArrayList> Roles;
@SerializedName("Info")
private SFUserInfo Info;
@SerializedName("AffiliatedPartnerUserId")
private String AffiliatedPartnerUserId;
@SerializedName("Favorites")
private ArrayList Favorites;
@SerializedName("Groups")
private ArrayList Groups;
@SerializedName("CanAccessConnectors")
private Boolean CanAccessConnectors;
@SerializedName("IsBillingContact")
private Boolean IsBillingContact;
public SFAccount getAccount() {
return this.Account;
}
public void setAccount(SFAccount account) {
this.Account = account;
}
public String getCompany() {
return this.Company;
}
public void setCompany(String company) {
this.Company = company;
}
public Integer getTotalSharedFiles() {
return this.TotalSharedFiles;
}
public void setTotalSharedFiles(Integer totalsharedfiles) {
this.TotalSharedFiles = totalsharedfiles;
}
public Integer getContacted() {
return this.Contacted;
}
public void setContacted(Integer contacted) {
this.Contacted = contacted;
}
/**
* The first and last name of the user
*/
public String getFullName() {
return this.FullName;
}
/**
* The first and last name of the user
*/
public void setFullName(String fullname) {
this.FullName = fullname;
}
public String getReferredBy() {
return this.ReferredBy;
}
public void setReferredBy(String referredby) {
this.ReferredBy = referredby;
}
public SFZone getDefaultZone() {
return this.DefaultZone;
}
public void setDefaultZone(SFZone defaultzone) {
this.DefaultZone = defaultzone;
}
public String getFirstName() {
return this.FirstName;
}
public void setFirstName(String firstname) {
this.FirstName = firstname;
}
public String getLastName() {
return this.LastName;
}
public void setLastName(String lastname) {
this.LastName = lastname;
}
public Date getDateCreated() {
return this.DateCreated;
}
public void setDateCreated(Date datecreated) {
this.DateCreated = datecreated;
}
public String getFullNameShort() {
return this.FullNameShort;
}
public void setFullNameShort(String fullnameshort) {
this.FullNameShort = fullnameshort;
}
public ArrayList getEmails() {
return this.Emails;
}
public void setEmails(ArrayList emails) {
this.Emails = emails;
}
public ArrayList getEmailAddresses() {
return this.EmailAddresses;
}
public void setEmailAddresses(ArrayList emailaddresses) {
this.EmailAddresses = emailaddresses;
}
public Boolean getIsConfirmed() {
return this.IsConfirmed;
}
public void setIsConfirmed(Boolean isconfirmed) {
this.IsConfirmed = isconfirmed;
}
public String getPassword() {
return this.Password;
}
public void setPassword(String password) {
this.Password = password;
}
public SFUserPreferences getPreferences() {
return this.Preferences;
}
public void setPreferences(SFUserPreferences preferences) {
this.Preferences = preferences;
}
public SFUserSecurity getSecurity() {
return this.Security;
}
public void setSecurity(SFUserSecurity security) {
this.Security = security;
}
/**
* This property would be deprecated in favor of the new property 'Favorites'
*/
public ArrayList getFavoriteFolders() {
return this.FavoriteFolders;
}
/**
* This property would be deprecated in favor of the new property 'Favorites'
*/
public void setFavoriteFolders(ArrayList favoritefolders) {
this.FavoriteFolders = favoritefolders;
}
public SFFolder getHomeFolder() {
return this.HomeFolder;
}
public void setHomeFolder(SFFolder homefolder) {
this.HomeFolder = homefolder;
}
public ArrayList getDevices() {
return this.Devices;
}
public void setDevices(ArrayList devices) {
this.Devices = devices;
}
public ArrayList> getIntegrations() {
return this.Integrations;
}
public void setIntegrations(ArrayList> integrations) {
this.Integrations = integrations;
}
public SFFolder getVirtualRoot() {
return this.VirtualRoot;
}
public void setVirtualRoot(SFFolder virtualroot) {
this.VirtualRoot = virtualroot;
}
public ArrayList> getRoles() {
return this.Roles;
}
public void setRoles(ArrayList> roles) {
this.Roles = roles;
}
public SFUserInfo getInfo() {
return this.Info;
}
public void setInfo(SFUserInfo info) {
this.Info = info;
}
public String getAffiliatedPartnerUserId() {
return this.AffiliatedPartnerUserId;
}
public void setAffiliatedPartnerUserId(String affiliatedpartneruserid) {
this.AffiliatedPartnerUserId = affiliatedpartneruserid;
}
/**
* List of Favorite items associated with the user
*/
public ArrayList getFavorites() {
return this.Favorites;
}
/**
* List of Favorite items associated with the user
*/
public void setFavorites(ArrayList favorites) {
this.Favorites = favorites;
}
/**
* List of Groups the user belongs. Only available when authenticated user and user match.
*/
public ArrayList getGroups() {
return this.Groups;
}
/**
* List of Groups the user belongs. Only available when authenticated user and user match.
*/
public void setGroups(ArrayList groups) {
this.Groups = groups;
}
/**
* This property is true if the user can create or has access to SymbolicLinks.
*/
public Boolean getCanAccessConnectors() {
return this.CanAccessConnectors;
}
/**
* This property is true if the user can create or has access to SymbolicLinks.
*/
public void setCanAccessConnectors(Boolean canaccessconnectors) {
this.CanAccessConnectors = canaccessconnectors;
}
/**
* Whether or not this user is the account's billing contact
*/
public Boolean getIsBillingContact() {
return this.IsBillingContact;
}
/**
* Whether or not this user is the account's billing contact
*/
public void setIsBillingContact(Boolean isbillingcontact) {
this.IsBillingContact = isbillingcontact;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy