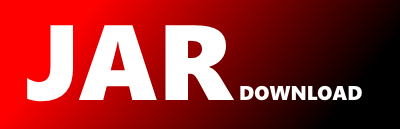
com.cjcrafter.openai.threads.message.TextAnnotation.kt Maven / Gradle / Ivy
package com.cjcrafter.openai.threads.message
import com.cjcrafter.openai.assistants.Assistant
import com.cjcrafter.openai.chat.tool.Tool.RetrievalTool
import com.cjcrafter.openai.chat.tool.Tool.CodeInterpreterTool
import com.fasterxml.jackson.annotation.JsonProperty
import com.fasterxml.jackson.annotation.JsonSubTypes
import com.fasterxml.jackson.annotation.JsonTypeInfo
/**
* A [TextContent] created by an [Assistant] may contain annotations.
*
* Annotations will be present in the [TextContent.Text.value], and may look
* like this:
* - `【13†source】`
* - `sandbox:/mnt/data/file.csv`
*
* You should replace the text in the message content with however you would like
* to annotate your files. For example, you could replace the text with a link
* to the file, or you could replace the text with the file contents.
*/
@JsonTypeInfo(use = JsonTypeInfo.Id.NAME, include = JsonTypeInfo.As.PROPERTY, property = "type")
@JsonSubTypes(
JsonSubTypes.Type(TextAnnotation.FileCitation::class, name = "file_citation"),
JsonSubTypes.Type(TextAnnotation.FilePath::class, name = "file_path"),
)
sealed class TextAnnotation {
/**
* File citations are created by the [RetrievalTool] and define references
* to a specific quote in a specific file that used by the [Assistant] to
* generate the response.
*
* @property text The text in the message content that needs to be replaced
* @property fileCitation The specific quote that was used in retrieval
* @property startIndex The index of the first character that needs to be replaced
* @property endIndex The index of the first character that does not need to be replaced
*/
data class FileCitation(
@JsonProperty(required = true) val text: String,
@JsonProperty("file_citation", required = true) val fileCitation: FileQuote,
@JsonProperty("start_index", required = true) val startIndex: Int,
@JsonProperty("end_index", required = true) val endIndex: Int,
): TextAnnotation() {
/**
* Holds data about the file quote generated by the retrieval tool.
*
* @property fileId The id of the file the quote was taken from
* @property quote The quote that was used to generate the response
*/
data class FileQuote(
@JsonProperty("file_id", required = true) val fileId: String,
@JsonProperty(required = true) val quote: String,
)
}
/**
* File paths are created by the [CodeInterpreterTool] and contain references
* to the files generated by the tool.
*
* @property text The text in the message content that needs to be replaced
* @property filePath The file that was generated by the code interpreter
* @property startIndex The index of the first character that needs to be replaced
* @property endIndex The index of the first character that does not need to be replaced
*/
data class FilePath(
@JsonProperty(required = true) val text: String,
@JsonProperty("file_path", required = true) val filePath: FileWrapper,
@JsonProperty("start_index", required = true) val startIndex: Int,
@JsonProperty("end_index", required = true) val endIndex: Int,
): TextAnnotation() {
/**
* Holds data about the file generated by the code interpreter.
*
* @property fileId The id of the file, can be used to retrieve the file
*/
data class FileWrapper(
@JsonProperty("file_id", required = true) val fileId: String,
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy