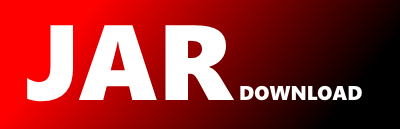
com.clarolab.bamboo.entities.BambooArtifact Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bamboo-rest-api-client Show documentation
Show all versions of bamboo-rest-api-client Show documentation
This library allows to extract information from projects, plans and builds on Bamboo
package com.clarolab.bamboo.entities;
import com.clarolab.bamboo.client.BambooApiClient;
import com.clarolab.http.utils.UrlUtils;
import com.google.common.base.Strings;
import com.google.common.collect.Maps;
import com.google.gson.annotations.SerializedName;
import lombok.Getter;
import lombok.Setter;
import lombok.ToString;
import org.apache.commons.io.FilenameUtils;
import org.apache.http.auth.AuthenticationException;
import org.json.XML;
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
import org.jsoup.nodes.Element;
import org.jsoup.select.Elements;
import java.io.IOException;
import java.net.URISyntaxException;
import java.util.Map;
@Getter
@Setter
@ToString
public class BambooArtifact {
private BambooApiClient bambooApiClient;
@ToString.Include
private String name;
@ToString.Include
@SerializedName("producerJobKey")
private String jobKey;
@ToString.Include
private BambooLink link;
public String getContent() throws URISyntaxException, IOException, AuthenticationException {
if(isXml())
return getContentAsJson();
else
return bambooApiClient.getHttpClient().get(UrlUtils.getEndpoint(link.getHref()).toString());
}
public Map getContentAtDirectory() throws AuthenticationException, IOException, URISyntaxException {
Map contents = Maps.newHashMap();
Document doc = Jsoup.parse(getContent());
Elements links = doc.select("tbody tr td a");
for (Element element : links) {
String content;
if(element.text().endsWith("xml"))
content = getContentAsJson(bambooApiClient.getHttpClient().get(element.attr("href")));
else
content = bambooApiClient.getHttpClient().get(element.attr("href"));
contents.put(element.text(), content);
}
return contents;
}
private String getContentAsJson() throws URISyntaxException, IOException, AuthenticationException {
return getContentAsJson(getContent());
}
private String getContentAsJson(String content) {
return XML.toJSONObject(content).toString(4);
}
public String getResourceLink(){
return link.getHref();
}
public String getResourceName(){
return getResourceLink().substring(getResourceLink().lastIndexOf("/") + 1);
}
public String getResourceExtension(){
return FilenameUtils.getExtension(getResourceName());
}
public boolean isLog(){
return getResourceExtension().endsWith("log");
}
public boolean isImage(){
return getResourceExtension().matches("jpg|png|gif|bmp|jpeg");
}
public boolean isXml(){
return getResourceExtension().endsWith("xml");
}
public boolean isJson(){
return getResourceExtension().endsWith("json");
}
public boolean isADirectory(){
// return getResourceLink().endsWith("/");
return Strings.isNullOrEmpty(getResourceExtension());
}
public boolean is(String fileExtension){
return getResourceExtension().endsWith(fileExtension);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy