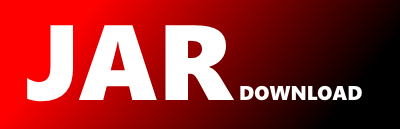
com.clearbit.client.model.Company Maven / Gradle / Ivy
The newest version!
// Generated by delombok at Wed Mar 21 19:16:59 PDT 2018
package com.clearbit.client.model;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonProperty;
public class Company {
@JsonProperty
String id;
@JsonProperty
String name;
@JsonProperty
String legalName;
@JsonProperty
String domain;
@JsonProperty
List domainAliases;
@JsonProperty
String logo;
@JsonProperty
Site site;
@JsonProperty
List tags;
@JsonProperty
Category category;
@JsonProperty
String description;
@JsonProperty
Integer foundedYear;
@JsonProperty
String location;
@JsonProperty
String timeZone;
@JsonProperty
Long utcOffset;
@JsonProperty
Geo geo;
@JsonProperty
Metrics metrics;
@JsonProperty
Facebook facebook;
@JsonProperty
LinkedIn linkedin;
@JsonProperty
Twitter twitter;
@JsonProperty
Crunchbase crunchbase;
@JsonProperty
Boolean emailProvider;
@JsonProperty
String type;
@JsonProperty
String ticker;
@JsonProperty
String phone;
@JsonProperty
String indexedAt;
@JsonProperty
List tech;
@JsonProperty
Parent parent;
public static class Site {
@JsonProperty
String title;
@JsonProperty
String h1;
@JsonProperty
String metaDescription;
@JsonProperty
List phoneNumbers;
@JsonProperty
List emailAddresses;
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Site() {
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getTitle() {
return this.title;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getH1() {
return this.h1;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getMetaDescription() {
return this.metaDescription;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public List getPhoneNumbers() {
return this.phoneNumbers;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public List getEmailAddresses() {
return this.emailAddresses;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setTitle(final String title) {
this.title = title;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setH1(final String h1) {
this.h1 = h1;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setMetaDescription(final String metaDescription) {
this.metaDescription = metaDescription;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setPhoneNumbers(final List phoneNumbers) {
this.phoneNumbers = phoneNumbers;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setEmailAddresses(final List emailAddresses) {
this.emailAddresses = emailAddresses;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Company.Site)) return false;
final Site other = (Site)o;
if (!other.canEqual((java.lang.Object)this)) return false;
final java.lang.Object this$title = this.getTitle();
final java.lang.Object other$title = other.getTitle();
if (this$title == null ? other$title != null : !this$title.equals(other$title)) return false;
final java.lang.Object this$h1 = this.getH1();
final java.lang.Object other$h1 = other.getH1();
if (this$h1 == null ? other$h1 != null : !this$h1.equals(other$h1)) return false;
final java.lang.Object this$metaDescription = this.getMetaDescription();
final java.lang.Object other$metaDescription = other.getMetaDescription();
if (this$metaDescription == null ? other$metaDescription != null : !this$metaDescription.equals(other$metaDescription)) return false;
final java.lang.Object this$phoneNumbers = this.getPhoneNumbers();
final java.lang.Object other$phoneNumbers = other.getPhoneNumbers();
if (this$phoneNumbers == null ? other$phoneNumbers != null : !this$phoneNumbers.equals(other$phoneNumbers)) return false;
final java.lang.Object this$emailAddresses = this.getEmailAddresses();
final java.lang.Object other$emailAddresses = other.getEmailAddresses();
if (this$emailAddresses == null ? other$emailAddresses != null : !this$emailAddresses.equals(other$emailAddresses)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Company.Site;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $title = this.getTitle();
result = result * PRIME + ($title == null ? 43 : $title.hashCode());
final java.lang.Object $h1 = this.getH1();
result = result * PRIME + ($h1 == null ? 43 : $h1.hashCode());
final java.lang.Object $metaDescription = this.getMetaDescription();
result = result * PRIME + ($metaDescription == null ? 43 : $metaDescription.hashCode());
final java.lang.Object $phoneNumbers = this.getPhoneNumbers();
result = result * PRIME + ($phoneNumbers == null ? 43 : $phoneNumbers.hashCode());
final java.lang.Object $emailAddresses = this.getEmailAddresses();
result = result * PRIME + ($emailAddresses == null ? 43 : $emailAddresses.hashCode());
return result;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public java.lang.String toString() {
return "Company.Site(title=" + this.getTitle() + ", h1=" + this.getH1() + ", metaDescription=" + this.getMetaDescription() + ", phoneNumbers=" + this.getPhoneNumbers() + ", emailAddresses=" + this.getEmailAddresses() + ")";
}
}
public static class Category {
@JsonProperty
String sector;
@JsonProperty
String industryGroup;
@JsonProperty
String industry;
@JsonProperty
String subIndustry;
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Category() {
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getSector() {
return this.sector;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getIndustryGroup() {
return this.industryGroup;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getIndustry() {
return this.industry;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getSubIndustry() {
return this.subIndustry;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setSector(final String sector) {
this.sector = sector;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setIndustryGroup(final String industryGroup) {
this.industryGroup = industryGroup;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setIndustry(final String industry) {
this.industry = industry;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setSubIndustry(final String subIndustry) {
this.subIndustry = subIndustry;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Company.Category)) return false;
final Category other = (Category)o;
if (!other.canEqual((java.lang.Object)this)) return false;
final java.lang.Object this$sector = this.getSector();
final java.lang.Object other$sector = other.getSector();
if (this$sector == null ? other$sector != null : !this$sector.equals(other$sector)) return false;
final java.lang.Object this$industryGroup = this.getIndustryGroup();
final java.lang.Object other$industryGroup = other.getIndustryGroup();
if (this$industryGroup == null ? other$industryGroup != null : !this$industryGroup.equals(other$industryGroup)) return false;
final java.lang.Object this$industry = this.getIndustry();
final java.lang.Object other$industry = other.getIndustry();
if (this$industry == null ? other$industry != null : !this$industry.equals(other$industry)) return false;
final java.lang.Object this$subIndustry = this.getSubIndustry();
final java.lang.Object other$subIndustry = other.getSubIndustry();
if (this$subIndustry == null ? other$subIndustry != null : !this$subIndustry.equals(other$subIndustry)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Company.Category;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $sector = this.getSector();
result = result * PRIME + ($sector == null ? 43 : $sector.hashCode());
final java.lang.Object $industryGroup = this.getIndustryGroup();
result = result * PRIME + ($industryGroup == null ? 43 : $industryGroup.hashCode());
final java.lang.Object $industry = this.getIndustry();
result = result * PRIME + ($industry == null ? 43 : $industry.hashCode());
final java.lang.Object $subIndustry = this.getSubIndustry();
result = result * PRIME + ($subIndustry == null ? 43 : $subIndustry.hashCode());
return result;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public java.lang.String toString() {
return "Company.Category(sector=" + this.getSector() + ", industryGroup=" + this.getIndustryGroup() + ", industry=" + this.getIndustry() + ", subIndustry=" + this.getSubIndustry() + ")";
}
}
public static class Geo {
@JsonProperty
String streetNumber;
@JsonProperty
String streetName;
@JsonProperty
String subPremise;
@JsonProperty
String city;
@JsonProperty
String state;
@JsonProperty
String stateCode;
@JsonProperty
String postalCode;
@JsonProperty
String country;
@JsonProperty
String countryCode;
@JsonProperty
Double lat;
@JsonProperty
Double lng;
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Geo() {
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getStreetNumber() {
return this.streetNumber;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getStreetName() {
return this.streetName;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getSubPremise() {
return this.subPremise;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getCity() {
return this.city;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getState() {
return this.state;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getStateCode() {
return this.stateCode;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getPostalCode() {
return this.postalCode;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getCountry() {
return this.country;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getCountryCode() {
return this.countryCode;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Double getLat() {
return this.lat;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Double getLng() {
return this.lng;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setStreetNumber(final String streetNumber) {
this.streetNumber = streetNumber;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setStreetName(final String streetName) {
this.streetName = streetName;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setSubPremise(final String subPremise) {
this.subPremise = subPremise;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setCity(final String city) {
this.city = city;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setState(final String state) {
this.state = state;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setStateCode(final String stateCode) {
this.stateCode = stateCode;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setPostalCode(final String postalCode) {
this.postalCode = postalCode;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setCountry(final String country) {
this.country = country;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setCountryCode(final String countryCode) {
this.countryCode = countryCode;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setLat(final Double lat) {
this.lat = lat;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setLng(final Double lng) {
this.lng = lng;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Company.Geo)) return false;
final Geo other = (Geo)o;
if (!other.canEqual((java.lang.Object)this)) return false;
final java.lang.Object this$streetNumber = this.getStreetNumber();
final java.lang.Object other$streetNumber = other.getStreetNumber();
if (this$streetNumber == null ? other$streetNumber != null : !this$streetNumber.equals(other$streetNumber)) return false;
final java.lang.Object this$streetName = this.getStreetName();
final java.lang.Object other$streetName = other.getStreetName();
if (this$streetName == null ? other$streetName != null : !this$streetName.equals(other$streetName)) return false;
final java.lang.Object this$subPremise = this.getSubPremise();
final java.lang.Object other$subPremise = other.getSubPremise();
if (this$subPremise == null ? other$subPremise != null : !this$subPremise.equals(other$subPremise)) return false;
final java.lang.Object this$city = this.getCity();
final java.lang.Object other$city = other.getCity();
if (this$city == null ? other$city != null : !this$city.equals(other$city)) return false;
final java.lang.Object this$state = this.getState();
final java.lang.Object other$state = other.getState();
if (this$state == null ? other$state != null : !this$state.equals(other$state)) return false;
final java.lang.Object this$stateCode = this.getStateCode();
final java.lang.Object other$stateCode = other.getStateCode();
if (this$stateCode == null ? other$stateCode != null : !this$stateCode.equals(other$stateCode)) return false;
final java.lang.Object this$postalCode = this.getPostalCode();
final java.lang.Object other$postalCode = other.getPostalCode();
if (this$postalCode == null ? other$postalCode != null : !this$postalCode.equals(other$postalCode)) return false;
final java.lang.Object this$country = this.getCountry();
final java.lang.Object other$country = other.getCountry();
if (this$country == null ? other$country != null : !this$country.equals(other$country)) return false;
final java.lang.Object this$countryCode = this.getCountryCode();
final java.lang.Object other$countryCode = other.getCountryCode();
if (this$countryCode == null ? other$countryCode != null : !this$countryCode.equals(other$countryCode)) return false;
final java.lang.Object this$lat = this.getLat();
final java.lang.Object other$lat = other.getLat();
if (this$lat == null ? other$lat != null : !this$lat.equals(other$lat)) return false;
final java.lang.Object this$lng = this.getLng();
final java.lang.Object other$lng = other.getLng();
if (this$lng == null ? other$lng != null : !this$lng.equals(other$lng)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Company.Geo;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $streetNumber = this.getStreetNumber();
result = result * PRIME + ($streetNumber == null ? 43 : $streetNumber.hashCode());
final java.lang.Object $streetName = this.getStreetName();
result = result * PRIME + ($streetName == null ? 43 : $streetName.hashCode());
final java.lang.Object $subPremise = this.getSubPremise();
result = result * PRIME + ($subPremise == null ? 43 : $subPremise.hashCode());
final java.lang.Object $city = this.getCity();
result = result * PRIME + ($city == null ? 43 : $city.hashCode());
final java.lang.Object $state = this.getState();
result = result * PRIME + ($state == null ? 43 : $state.hashCode());
final java.lang.Object $stateCode = this.getStateCode();
result = result * PRIME + ($stateCode == null ? 43 : $stateCode.hashCode());
final java.lang.Object $postalCode = this.getPostalCode();
result = result * PRIME + ($postalCode == null ? 43 : $postalCode.hashCode());
final java.lang.Object $country = this.getCountry();
result = result * PRIME + ($country == null ? 43 : $country.hashCode());
final java.lang.Object $countryCode = this.getCountryCode();
result = result * PRIME + ($countryCode == null ? 43 : $countryCode.hashCode());
final java.lang.Object $lat = this.getLat();
result = result * PRIME + ($lat == null ? 43 : $lat.hashCode());
final java.lang.Object $lng = this.getLng();
result = result * PRIME + ($lng == null ? 43 : $lng.hashCode());
return result;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public java.lang.String toString() {
return "Company.Geo(streetNumber=" + this.getStreetNumber() + ", streetName=" + this.getStreetName() + ", subPremise=" + this.getSubPremise() + ", city=" + this.getCity() + ", state=" + this.getState() + ", stateCode=" + this.getStateCode() + ", postalCode=" + this.getPostalCode() + ", country=" + this.getCountry() + ", countryCode=" + this.getCountryCode() + ", lat=" + this.getLat() + ", lng=" + this.getLng() + ")";
}
}
public static class Metrics {
@JsonProperty
Long alexaUsRank;
@JsonProperty
Long alexaGlobalRank;
@JsonProperty
Long employees;
@JsonProperty
String employeesRange;
@JsonProperty
Long marketCap;
@JsonProperty
Long raised;
@JsonProperty
Long annualRevenue;
@JsonProperty
Long fiscalYearEnd;
@JsonProperty
String estimatedAnnualRevenue;
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Metrics() {
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Long getAlexaUsRank() {
return this.alexaUsRank;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Long getAlexaGlobalRank() {
return this.alexaGlobalRank;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Long getEmployees() {
return this.employees;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getEmployeesRange() {
return this.employeesRange;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Long getMarketCap() {
return this.marketCap;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Long getRaised() {
return this.raised;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Long getAnnualRevenue() {
return this.annualRevenue;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Long getFiscalYearEnd() {
return this.fiscalYearEnd;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getEstimatedAnnualRevenue() {
return this.estimatedAnnualRevenue;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setAlexaUsRank(final Long alexaUsRank) {
this.alexaUsRank = alexaUsRank;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setAlexaGlobalRank(final Long alexaGlobalRank) {
this.alexaGlobalRank = alexaGlobalRank;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setEmployees(final Long employees) {
this.employees = employees;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setEmployeesRange(final String employeesRange) {
this.employeesRange = employeesRange;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setMarketCap(final Long marketCap) {
this.marketCap = marketCap;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setRaised(final Long raised) {
this.raised = raised;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setAnnualRevenue(final Long annualRevenue) {
this.annualRevenue = annualRevenue;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setFiscalYearEnd(final Long fiscalYearEnd) {
this.fiscalYearEnd = fiscalYearEnd;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setEstimatedAnnualRevenue(final String estimatedAnnualRevenue) {
this.estimatedAnnualRevenue = estimatedAnnualRevenue;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Company.Metrics)) return false;
final Metrics other = (Metrics)o;
if (!other.canEqual((java.lang.Object)this)) return false;
final java.lang.Object this$alexaUsRank = this.getAlexaUsRank();
final java.lang.Object other$alexaUsRank = other.getAlexaUsRank();
if (this$alexaUsRank == null ? other$alexaUsRank != null : !this$alexaUsRank.equals(other$alexaUsRank)) return false;
final java.lang.Object this$alexaGlobalRank = this.getAlexaGlobalRank();
final java.lang.Object other$alexaGlobalRank = other.getAlexaGlobalRank();
if (this$alexaGlobalRank == null ? other$alexaGlobalRank != null : !this$alexaGlobalRank.equals(other$alexaGlobalRank)) return false;
final java.lang.Object this$employees = this.getEmployees();
final java.lang.Object other$employees = other.getEmployees();
if (this$employees == null ? other$employees != null : !this$employees.equals(other$employees)) return false;
final java.lang.Object this$employeesRange = this.getEmployeesRange();
final java.lang.Object other$employeesRange = other.getEmployeesRange();
if (this$employeesRange == null ? other$employeesRange != null : !this$employeesRange.equals(other$employeesRange)) return false;
final java.lang.Object this$marketCap = this.getMarketCap();
final java.lang.Object other$marketCap = other.getMarketCap();
if (this$marketCap == null ? other$marketCap != null : !this$marketCap.equals(other$marketCap)) return false;
final java.lang.Object this$raised = this.getRaised();
final java.lang.Object other$raised = other.getRaised();
if (this$raised == null ? other$raised != null : !this$raised.equals(other$raised)) return false;
final java.lang.Object this$annualRevenue = this.getAnnualRevenue();
final java.lang.Object other$annualRevenue = other.getAnnualRevenue();
if (this$annualRevenue == null ? other$annualRevenue != null : !this$annualRevenue.equals(other$annualRevenue)) return false;
final java.lang.Object this$fiscalYearEnd = this.getFiscalYearEnd();
final java.lang.Object other$fiscalYearEnd = other.getFiscalYearEnd();
if (this$fiscalYearEnd == null ? other$fiscalYearEnd != null : !this$fiscalYearEnd.equals(other$fiscalYearEnd)) return false;
final java.lang.Object this$estimatedAnnualRevenue = this.getEstimatedAnnualRevenue();
final java.lang.Object other$estimatedAnnualRevenue = other.getEstimatedAnnualRevenue();
if (this$estimatedAnnualRevenue == null ? other$estimatedAnnualRevenue != null : !this$estimatedAnnualRevenue.equals(other$estimatedAnnualRevenue)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Company.Metrics;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $alexaUsRank = this.getAlexaUsRank();
result = result * PRIME + ($alexaUsRank == null ? 43 : $alexaUsRank.hashCode());
final java.lang.Object $alexaGlobalRank = this.getAlexaGlobalRank();
result = result * PRIME + ($alexaGlobalRank == null ? 43 : $alexaGlobalRank.hashCode());
final java.lang.Object $employees = this.getEmployees();
result = result * PRIME + ($employees == null ? 43 : $employees.hashCode());
final java.lang.Object $employeesRange = this.getEmployeesRange();
result = result * PRIME + ($employeesRange == null ? 43 : $employeesRange.hashCode());
final java.lang.Object $marketCap = this.getMarketCap();
result = result * PRIME + ($marketCap == null ? 43 : $marketCap.hashCode());
final java.lang.Object $raised = this.getRaised();
result = result * PRIME + ($raised == null ? 43 : $raised.hashCode());
final java.lang.Object $annualRevenue = this.getAnnualRevenue();
result = result * PRIME + ($annualRevenue == null ? 43 : $annualRevenue.hashCode());
final java.lang.Object $fiscalYearEnd = this.getFiscalYearEnd();
result = result * PRIME + ($fiscalYearEnd == null ? 43 : $fiscalYearEnd.hashCode());
final java.lang.Object $estimatedAnnualRevenue = this.getEstimatedAnnualRevenue();
result = result * PRIME + ($estimatedAnnualRevenue == null ? 43 : $estimatedAnnualRevenue.hashCode());
return result;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public java.lang.String toString() {
return "Company.Metrics(alexaUsRank=" + this.getAlexaUsRank() + ", alexaGlobalRank=" + this.getAlexaGlobalRank() + ", employees=" + this.getEmployees() + ", employeesRange=" + this.getEmployeesRange() + ", marketCap=" + this.getMarketCap() + ", raised=" + this.getRaised() + ", annualRevenue=" + this.getAnnualRevenue() + ", fiscalYearEnd=" + this.getFiscalYearEnd() + ", estimatedAnnualRevenue=" + this.getEstimatedAnnualRevenue() + ")";
}
}
public static class Facebook {
@JsonProperty
String handle;
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Facebook() {
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getHandle() {
return this.handle;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setHandle(final String handle) {
this.handle = handle;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Company.Facebook)) return false;
final Facebook other = (Facebook)o;
if (!other.canEqual((java.lang.Object)this)) return false;
final java.lang.Object this$handle = this.getHandle();
final java.lang.Object other$handle = other.getHandle();
if (this$handle == null ? other$handle != null : !this$handle.equals(other$handle)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Company.Facebook;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $handle = this.getHandle();
result = result * PRIME + ($handle == null ? 43 : $handle.hashCode());
return result;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public java.lang.String toString() {
return "Company.Facebook(handle=" + this.getHandle() + ")";
}
}
public static class LinkedIn {
@JsonProperty
String handle;
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public LinkedIn() {
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getHandle() {
return this.handle;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setHandle(final String handle) {
this.handle = handle;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Company.LinkedIn)) return false;
final LinkedIn other = (LinkedIn)o;
if (!other.canEqual((java.lang.Object)this)) return false;
final java.lang.Object this$handle = this.getHandle();
final java.lang.Object other$handle = other.getHandle();
if (this$handle == null ? other$handle != null : !this$handle.equals(other$handle)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Company.LinkedIn;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $handle = this.getHandle();
result = result * PRIME + ($handle == null ? 43 : $handle.hashCode());
return result;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public java.lang.String toString() {
return "Company.LinkedIn(handle=" + this.getHandle() + ")";
}
}
public static class Twitter {
@JsonProperty
String handle;
@JsonProperty
String id;
@JsonProperty
String bio;
@JsonProperty
Long followers;
@JsonProperty
Long following;
@JsonProperty
String location;
@JsonProperty
String site;
@JsonProperty
String avatar;
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Twitter() {
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getHandle() {
return this.handle;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getId() {
return this.id;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getBio() {
return this.bio;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Long getFollowers() {
return this.followers;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Long getFollowing() {
return this.following;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getLocation() {
return this.location;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getSite() {
return this.site;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getAvatar() {
return this.avatar;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setHandle(final String handle) {
this.handle = handle;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setId(final String id) {
this.id = id;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setBio(final String bio) {
this.bio = bio;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setFollowers(final Long followers) {
this.followers = followers;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setFollowing(final Long following) {
this.following = following;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setLocation(final String location) {
this.location = location;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setSite(final String site) {
this.site = site;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setAvatar(final String avatar) {
this.avatar = avatar;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Company.Twitter)) return false;
final Twitter other = (Twitter)o;
if (!other.canEqual((java.lang.Object)this)) return false;
final java.lang.Object this$handle = this.getHandle();
final java.lang.Object other$handle = other.getHandle();
if (this$handle == null ? other$handle != null : !this$handle.equals(other$handle)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$bio = this.getBio();
final java.lang.Object other$bio = other.getBio();
if (this$bio == null ? other$bio != null : !this$bio.equals(other$bio)) return false;
final java.lang.Object this$followers = this.getFollowers();
final java.lang.Object other$followers = other.getFollowers();
if (this$followers == null ? other$followers != null : !this$followers.equals(other$followers)) return false;
final java.lang.Object this$following = this.getFollowing();
final java.lang.Object other$following = other.getFollowing();
if (this$following == null ? other$following != null : !this$following.equals(other$following)) return false;
final java.lang.Object this$location = this.getLocation();
final java.lang.Object other$location = other.getLocation();
if (this$location == null ? other$location != null : !this$location.equals(other$location)) return false;
final java.lang.Object this$site = this.getSite();
final java.lang.Object other$site = other.getSite();
if (this$site == null ? other$site != null : !this$site.equals(other$site)) return false;
final java.lang.Object this$avatar = this.getAvatar();
final java.lang.Object other$avatar = other.getAvatar();
if (this$avatar == null ? other$avatar != null : !this$avatar.equals(other$avatar)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Company.Twitter;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $handle = this.getHandle();
result = result * PRIME + ($handle == null ? 43 : $handle.hashCode());
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $bio = this.getBio();
result = result * PRIME + ($bio == null ? 43 : $bio.hashCode());
final java.lang.Object $followers = this.getFollowers();
result = result * PRIME + ($followers == null ? 43 : $followers.hashCode());
final java.lang.Object $following = this.getFollowing();
result = result * PRIME + ($following == null ? 43 : $following.hashCode());
final java.lang.Object $location = this.getLocation();
result = result * PRIME + ($location == null ? 43 : $location.hashCode());
final java.lang.Object $site = this.getSite();
result = result * PRIME + ($site == null ? 43 : $site.hashCode());
final java.lang.Object $avatar = this.getAvatar();
result = result * PRIME + ($avatar == null ? 43 : $avatar.hashCode());
return result;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public java.lang.String toString() {
return "Company.Twitter(handle=" + this.getHandle() + ", id=" + this.getId() + ", bio=" + this.getBio() + ", followers=" + this.getFollowers() + ", following=" + this.getFollowing() + ", location=" + this.getLocation() + ", site=" + this.getSite() + ", avatar=" + this.getAvatar() + ")";
}
}
public static class AngelList {
@JsonProperty
String handle;
@JsonProperty
String bio;
@JsonProperty
String blog;
@JsonProperty
String site;
@JsonProperty
Long followers;
@JsonProperty
String avatar;
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public AngelList() {
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getHandle() {
return this.handle;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getBio() {
return this.bio;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getBlog() {
return this.blog;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getSite() {
return this.site;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Long getFollowers() {
return this.followers;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getAvatar() {
return this.avatar;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setHandle(final String handle) {
this.handle = handle;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setBio(final String bio) {
this.bio = bio;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setBlog(final String blog) {
this.blog = blog;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setSite(final String site) {
this.site = site;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setFollowers(final Long followers) {
this.followers = followers;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setAvatar(final String avatar) {
this.avatar = avatar;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Company.AngelList)) return false;
final AngelList other = (AngelList)o;
if (!other.canEqual((java.lang.Object)this)) return false;
final java.lang.Object this$handle = this.getHandle();
final java.lang.Object other$handle = other.getHandle();
if (this$handle == null ? other$handle != null : !this$handle.equals(other$handle)) return false;
final java.lang.Object this$bio = this.getBio();
final java.lang.Object other$bio = other.getBio();
if (this$bio == null ? other$bio != null : !this$bio.equals(other$bio)) return false;
final java.lang.Object this$blog = this.getBlog();
final java.lang.Object other$blog = other.getBlog();
if (this$blog == null ? other$blog != null : !this$blog.equals(other$blog)) return false;
final java.lang.Object this$site = this.getSite();
final java.lang.Object other$site = other.getSite();
if (this$site == null ? other$site != null : !this$site.equals(other$site)) return false;
final java.lang.Object this$followers = this.getFollowers();
final java.lang.Object other$followers = other.getFollowers();
if (this$followers == null ? other$followers != null : !this$followers.equals(other$followers)) return false;
final java.lang.Object this$avatar = this.getAvatar();
final java.lang.Object other$avatar = other.getAvatar();
if (this$avatar == null ? other$avatar != null : !this$avatar.equals(other$avatar)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Company.AngelList;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $handle = this.getHandle();
result = result * PRIME + ($handle == null ? 43 : $handle.hashCode());
final java.lang.Object $bio = this.getBio();
result = result * PRIME + ($bio == null ? 43 : $bio.hashCode());
final java.lang.Object $blog = this.getBlog();
result = result * PRIME + ($blog == null ? 43 : $blog.hashCode());
final java.lang.Object $site = this.getSite();
result = result * PRIME + ($site == null ? 43 : $site.hashCode());
final java.lang.Object $followers = this.getFollowers();
result = result * PRIME + ($followers == null ? 43 : $followers.hashCode());
final java.lang.Object $avatar = this.getAvatar();
result = result * PRIME + ($avatar == null ? 43 : $avatar.hashCode());
return result;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public java.lang.String toString() {
return "Company.AngelList(handle=" + this.getHandle() + ", bio=" + this.getBio() + ", blog=" + this.getBlog() + ", site=" + this.getSite() + ", followers=" + this.getFollowers() + ", avatar=" + this.getAvatar() + ")";
}
}
public static class Crunchbase {
@JsonProperty
String handle;
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Crunchbase() {
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getHandle() {
return this.handle;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setHandle(final String handle) {
this.handle = handle;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Company.Crunchbase)) return false;
final Crunchbase other = (Crunchbase)o;
if (!other.canEqual((java.lang.Object)this)) return false;
final java.lang.Object this$handle = this.getHandle();
final java.lang.Object other$handle = other.getHandle();
if (this$handle == null ? other$handle != null : !this$handle.equals(other$handle)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Company.Crunchbase;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $handle = this.getHandle();
result = result * PRIME + ($handle == null ? 43 : $handle.hashCode());
return result;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public java.lang.String toString() {
return "Company.Crunchbase(handle=" + this.getHandle() + ")";
}
}
public static class Parent {
@JsonProperty
String domain;
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Parent() {
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getDomain() {
return this.domain;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setDomain(final String domain) {
this.domain = domain;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Company.Parent)) return false;
final Parent other = (Parent)o;
if (!other.canEqual((java.lang.Object)this)) return false;
final java.lang.Object this$domain = this.getDomain();
final java.lang.Object other$domain = other.getDomain();
if (this$domain == null ? other$domain != null : !this$domain.equals(other$domain)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Company.Parent;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $domain = this.getDomain();
result = result * PRIME + ($domain == null ? 43 : $domain.hashCode());
return result;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public java.lang.String toString() {
return "Company.Parent(domain=" + this.getDomain() + ")";
}
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Company() {
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getId() {
return this.id;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getName() {
return this.name;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getLegalName() {
return this.legalName;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getDomain() {
return this.domain;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public List getDomainAliases() {
return this.domainAliases;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getLogo() {
return this.logo;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Site getSite() {
return this.site;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public List getTags() {
return this.tags;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Category getCategory() {
return this.category;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getDescription() {
return this.description;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Integer getFoundedYear() {
return this.foundedYear;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getLocation() {
return this.location;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getTimeZone() {
return this.timeZone;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Long getUtcOffset() {
return this.utcOffset;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Geo getGeo() {
return this.geo;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Metrics getMetrics() {
return this.metrics;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Facebook getFacebook() {
return this.facebook;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public LinkedIn getLinkedin() {
return this.linkedin;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Twitter getTwitter() {
return this.twitter;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Crunchbase getCrunchbase() {
return this.crunchbase;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Boolean getEmailProvider() {
return this.emailProvider;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getType() {
return this.type;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getTicker() {
return this.ticker;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getPhone() {
return this.phone;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getIndexedAt() {
return this.indexedAt;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public List getTech() {
return this.tech;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Parent getParent() {
return this.parent;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setId(final String id) {
this.id = id;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setName(final String name) {
this.name = name;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setLegalName(final String legalName) {
this.legalName = legalName;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setDomain(final String domain) {
this.domain = domain;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setDomainAliases(final List domainAliases) {
this.domainAliases = domainAliases;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setLogo(final String logo) {
this.logo = logo;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setSite(final Site site) {
this.site = site;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setTags(final List tags) {
this.tags = tags;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setCategory(final Category category) {
this.category = category;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setDescription(final String description) {
this.description = description;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setFoundedYear(final Integer foundedYear) {
this.foundedYear = foundedYear;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setLocation(final String location) {
this.location = location;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setTimeZone(final String timeZone) {
this.timeZone = timeZone;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setUtcOffset(final Long utcOffset) {
this.utcOffset = utcOffset;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setGeo(final Geo geo) {
this.geo = geo;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setMetrics(final Metrics metrics) {
this.metrics = metrics;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setFacebook(final Facebook facebook) {
this.facebook = facebook;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setLinkedin(final LinkedIn linkedin) {
this.linkedin = linkedin;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setTwitter(final Twitter twitter) {
this.twitter = twitter;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setCrunchbase(final Crunchbase crunchbase) {
this.crunchbase = crunchbase;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setEmailProvider(final Boolean emailProvider) {
this.emailProvider = emailProvider;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setType(final String type) {
this.type = type;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setTicker(final String ticker) {
this.ticker = ticker;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setPhone(final String phone) {
this.phone = phone;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setIndexedAt(final String indexedAt) {
this.indexedAt = indexedAt;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setTech(final List tech) {
this.tech = tech;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public void setParent(final Parent parent) {
this.parent = parent;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Company)) return false;
final Company other = (Company)o;
if (!other.canEqual((java.lang.Object)this)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$name = this.getName();
final java.lang.Object other$name = other.getName();
if (this$name == null ? other$name != null : !this$name.equals(other$name)) return false;
final java.lang.Object this$legalName = this.getLegalName();
final java.lang.Object other$legalName = other.getLegalName();
if (this$legalName == null ? other$legalName != null : !this$legalName.equals(other$legalName)) return false;
final java.lang.Object this$domain = this.getDomain();
final java.lang.Object other$domain = other.getDomain();
if (this$domain == null ? other$domain != null : !this$domain.equals(other$domain)) return false;
final java.lang.Object this$domainAliases = this.getDomainAliases();
final java.lang.Object other$domainAliases = other.getDomainAliases();
if (this$domainAliases == null ? other$domainAliases != null : !this$domainAliases.equals(other$domainAliases)) return false;
final java.lang.Object this$logo = this.getLogo();
final java.lang.Object other$logo = other.getLogo();
if (this$logo == null ? other$logo != null : !this$logo.equals(other$logo)) return false;
final java.lang.Object this$site = this.getSite();
final java.lang.Object other$site = other.getSite();
if (this$site == null ? other$site != null : !this$site.equals(other$site)) return false;
final java.lang.Object this$tags = this.getTags();
final java.lang.Object other$tags = other.getTags();
if (this$tags == null ? other$tags != null : !this$tags.equals(other$tags)) return false;
final java.lang.Object this$category = this.getCategory();
final java.lang.Object other$category = other.getCategory();
if (this$category == null ? other$category != null : !this$category.equals(other$category)) return false;
final java.lang.Object this$description = this.getDescription();
final java.lang.Object other$description = other.getDescription();
if (this$description == null ? other$description != null : !this$description.equals(other$description)) return false;
final java.lang.Object this$foundedYear = this.getFoundedYear();
final java.lang.Object other$foundedYear = other.getFoundedYear();
if (this$foundedYear == null ? other$foundedYear != null : !this$foundedYear.equals(other$foundedYear)) return false;
final java.lang.Object this$location = this.getLocation();
final java.lang.Object other$location = other.getLocation();
if (this$location == null ? other$location != null : !this$location.equals(other$location)) return false;
final java.lang.Object this$timeZone = this.getTimeZone();
final java.lang.Object other$timeZone = other.getTimeZone();
if (this$timeZone == null ? other$timeZone != null : !this$timeZone.equals(other$timeZone)) return false;
final java.lang.Object this$utcOffset = this.getUtcOffset();
final java.lang.Object other$utcOffset = other.getUtcOffset();
if (this$utcOffset == null ? other$utcOffset != null : !this$utcOffset.equals(other$utcOffset)) return false;
final java.lang.Object this$geo = this.getGeo();
final java.lang.Object other$geo = other.getGeo();
if (this$geo == null ? other$geo != null : !this$geo.equals(other$geo)) return false;
final java.lang.Object this$metrics = this.getMetrics();
final java.lang.Object other$metrics = other.getMetrics();
if (this$metrics == null ? other$metrics != null : !this$metrics.equals(other$metrics)) return false;
final java.lang.Object this$facebook = this.getFacebook();
final java.lang.Object other$facebook = other.getFacebook();
if (this$facebook == null ? other$facebook != null : !this$facebook.equals(other$facebook)) return false;
final java.lang.Object this$linkedin = this.getLinkedin();
final java.lang.Object other$linkedin = other.getLinkedin();
if (this$linkedin == null ? other$linkedin != null : !this$linkedin.equals(other$linkedin)) return false;
final java.lang.Object this$twitter = this.getTwitter();
final java.lang.Object other$twitter = other.getTwitter();
if (this$twitter == null ? other$twitter != null : !this$twitter.equals(other$twitter)) return false;
final java.lang.Object this$crunchbase = this.getCrunchbase();
final java.lang.Object other$crunchbase = other.getCrunchbase();
if (this$crunchbase == null ? other$crunchbase != null : !this$crunchbase.equals(other$crunchbase)) return false;
final java.lang.Object this$emailProvider = this.getEmailProvider();
final java.lang.Object other$emailProvider = other.getEmailProvider();
if (this$emailProvider == null ? other$emailProvider != null : !this$emailProvider.equals(other$emailProvider)) return false;
final java.lang.Object this$type = this.getType();
final java.lang.Object other$type = other.getType();
if (this$type == null ? other$type != null : !this$type.equals(other$type)) return false;
final java.lang.Object this$ticker = this.getTicker();
final java.lang.Object other$ticker = other.getTicker();
if (this$ticker == null ? other$ticker != null : !this$ticker.equals(other$ticker)) return false;
final java.lang.Object this$phone = this.getPhone();
final java.lang.Object other$phone = other.getPhone();
if (this$phone == null ? other$phone != null : !this$phone.equals(other$phone)) return false;
final java.lang.Object this$indexedAt = this.getIndexedAt();
final java.lang.Object other$indexedAt = other.getIndexedAt();
if (this$indexedAt == null ? other$indexedAt != null : !this$indexedAt.equals(other$indexedAt)) return false;
final java.lang.Object this$tech = this.getTech();
final java.lang.Object other$tech = other.getTech();
if (this$tech == null ? other$tech != null : !this$tech.equals(other$tech)) return false;
final java.lang.Object this$parent = this.getParent();
final java.lang.Object other$parent = other.getParent();
if (this$parent == null ? other$parent != null : !this$parent.equals(other$parent)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Company;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $name = this.getName();
result = result * PRIME + ($name == null ? 43 : $name.hashCode());
final java.lang.Object $legalName = this.getLegalName();
result = result * PRIME + ($legalName == null ? 43 : $legalName.hashCode());
final java.lang.Object $domain = this.getDomain();
result = result * PRIME + ($domain == null ? 43 : $domain.hashCode());
final java.lang.Object $domainAliases = this.getDomainAliases();
result = result * PRIME + ($domainAliases == null ? 43 : $domainAliases.hashCode());
final java.lang.Object $logo = this.getLogo();
result = result * PRIME + ($logo == null ? 43 : $logo.hashCode());
final java.lang.Object $site = this.getSite();
result = result * PRIME + ($site == null ? 43 : $site.hashCode());
final java.lang.Object $tags = this.getTags();
result = result * PRIME + ($tags == null ? 43 : $tags.hashCode());
final java.lang.Object $category = this.getCategory();
result = result * PRIME + ($category == null ? 43 : $category.hashCode());
final java.lang.Object $description = this.getDescription();
result = result * PRIME + ($description == null ? 43 : $description.hashCode());
final java.lang.Object $foundedYear = this.getFoundedYear();
result = result * PRIME + ($foundedYear == null ? 43 : $foundedYear.hashCode());
final java.lang.Object $location = this.getLocation();
result = result * PRIME + ($location == null ? 43 : $location.hashCode());
final java.lang.Object $timeZone = this.getTimeZone();
result = result * PRIME + ($timeZone == null ? 43 : $timeZone.hashCode());
final java.lang.Object $utcOffset = this.getUtcOffset();
result = result * PRIME + ($utcOffset == null ? 43 : $utcOffset.hashCode());
final java.lang.Object $geo = this.getGeo();
result = result * PRIME + ($geo == null ? 43 : $geo.hashCode());
final java.lang.Object $metrics = this.getMetrics();
result = result * PRIME + ($metrics == null ? 43 : $metrics.hashCode());
final java.lang.Object $facebook = this.getFacebook();
result = result * PRIME + ($facebook == null ? 43 : $facebook.hashCode());
final java.lang.Object $linkedin = this.getLinkedin();
result = result * PRIME + ($linkedin == null ? 43 : $linkedin.hashCode());
final java.lang.Object $twitter = this.getTwitter();
result = result * PRIME + ($twitter == null ? 43 : $twitter.hashCode());
final java.lang.Object $crunchbase = this.getCrunchbase();
result = result * PRIME + ($crunchbase == null ? 43 : $crunchbase.hashCode());
final java.lang.Object $emailProvider = this.getEmailProvider();
result = result * PRIME + ($emailProvider == null ? 43 : $emailProvider.hashCode());
final java.lang.Object $type = this.getType();
result = result * PRIME + ($type == null ? 43 : $type.hashCode());
final java.lang.Object $ticker = this.getTicker();
result = result * PRIME + ($ticker == null ? 43 : $ticker.hashCode());
final java.lang.Object $phone = this.getPhone();
result = result * PRIME + ($phone == null ? 43 : $phone.hashCode());
final java.lang.Object $indexedAt = this.getIndexedAt();
result = result * PRIME + ($indexedAt == null ? 43 : $indexedAt.hashCode());
final java.lang.Object $tech = this.getTech();
result = result * PRIME + ($tech == null ? 43 : $tech.hashCode());
final java.lang.Object $parent = this.getParent();
result = result * PRIME + ($parent == null ? 43 : $parent.hashCode());
return result;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public java.lang.String toString() {
return "Company(id=" + this.getId() + ", name=" + this.getName() + ", legalName=" + this.getLegalName() + ", domain=" + this.getDomain() + ", domainAliases=" + this.getDomainAliases() + ", logo=" + this.getLogo() + ", site=" + this.getSite() + ", tags=" + this.getTags() + ", category=" + this.getCategory() + ", description=" + this.getDescription() + ", foundedYear=" + this.getFoundedYear() + ", location=" + this.getLocation() + ", timeZone=" + this.getTimeZone() + ", utcOffset=" + this.getUtcOffset() + ", geo=" + this.getGeo() + ", metrics=" + this.getMetrics() + ", facebook=" + this.getFacebook() + ", linkedin=" + this.getLinkedin() + ", twitter=" + this.getTwitter() + ", crunchbase=" + this.getCrunchbase() + ", emailProvider=" + this.getEmailProvider() + ", type=" + this.getType() + ", ticker=" + this.getTicker() + ", phone=" + this.getPhone() + ", indexedAt=" + this.getIndexedAt() + ", tech=" + this.getTech() + ", parent=" + this.getParent() + ")";
}
}