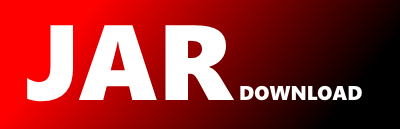
io.swagger.client.model.Student Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of client Show documentation
Show all versions of client Show documentation
Client Library for the Clever API
/*
* Clever API
* The Clever API
*
* OpenAPI spec version: 1.2.0
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package io.swagger.client.model;
import java.util.Objects;
import com.google.gson.annotations.SerializedName;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.client.model.Location;
import io.swagger.client.model.Name;
import java.util.ArrayList;
import java.util.List;
/**
* Student
*/
@javax.annotation.Generated(value = "io.swagger.codegen.languages.JavaClientCodegen", date = "2017-04-06T11:52:21.984-07:00")
public class Student {
@SerializedName("id")
private String id = null;
@SerializedName("created")
private String created = null;
@SerializedName("district")
private String district = null;
@SerializedName("dob")
private String dob = null;
@SerializedName("ell_status")
private String ellStatus = null;
@SerializedName("email")
private String email = null;
@SerializedName("frl_status")
private String frlStatus = null;
@SerializedName("gender")
private String gender = null;
@SerializedName("grade")
private String grade = null;
@SerializedName("hispanic_ethnicity")
private String hispanicEthnicity = null;
@SerializedName("iep_status")
private String iepStatus = null;
@SerializedName("last_modified")
private String lastModified = null;
@SerializedName("location")
private Location location = null;
@SerializedName("name")
private Name name = null;
@SerializedName("race")
private String race = null;
@SerializedName("school")
private String school = null;
@SerializedName("schools")
private List schools = new ArrayList();
@SerializedName("sis_id")
private String sisId = null;
@SerializedName("state_id")
private String stateId = null;
@SerializedName("student_number")
private String studentNumber = null;
public Student id(String id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
**/
@ApiModelProperty(value = "")
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public Student created(String created) {
this.created = created;
return this;
}
/**
* Get created
* @return created
**/
@ApiModelProperty(value = "")
public String getCreated() {
return created;
}
public void setCreated(String created) {
this.created = created;
}
public Student district(String district) {
this.district = district;
return this;
}
/**
* Get district
* @return district
**/
@ApiModelProperty(value = "")
public String getDistrict() {
return district;
}
public void setDistrict(String district) {
this.district = district;
}
public Student dob(String dob) {
this.dob = dob;
return this;
}
/**
* Get dob
* @return dob
**/
@ApiModelProperty(value = "")
public String getDob() {
return dob;
}
public void setDob(String dob) {
this.dob = dob;
}
public Student ellStatus(String ellStatus) {
this.ellStatus = ellStatus;
return this;
}
/**
* Get ellStatus
* @return ellStatus
**/
@ApiModelProperty(value = "")
public String getEllStatus() {
return ellStatus;
}
public void setEllStatus(String ellStatus) {
this.ellStatus = ellStatus;
}
public Student email(String email) {
this.email = email;
return this;
}
/**
* Get email
* @return email
**/
@ApiModelProperty(value = "")
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public Student frlStatus(String frlStatus) {
this.frlStatus = frlStatus;
return this;
}
/**
* Get frlStatus
* @return frlStatus
**/
@ApiModelProperty(value = "")
public String getFrlStatus() {
return frlStatus;
}
public void setFrlStatus(String frlStatus) {
this.frlStatus = frlStatus;
}
public Student gender(String gender) {
this.gender = gender;
return this;
}
/**
* Get gender
* @return gender
**/
@ApiModelProperty(value = "")
public String getGender() {
return gender;
}
public void setGender(String gender) {
this.gender = gender;
}
public Student grade(String grade) {
this.grade = grade;
return this;
}
/**
* Get grade
* @return grade
**/
@ApiModelProperty(value = "")
public String getGrade() {
return grade;
}
public void setGrade(String grade) {
this.grade = grade;
}
public Student hispanicEthnicity(String hispanicEthnicity) {
this.hispanicEthnicity = hispanicEthnicity;
return this;
}
/**
* Get hispanicEthnicity
* @return hispanicEthnicity
**/
@ApiModelProperty(value = "")
public String getHispanicEthnicity() {
return hispanicEthnicity;
}
public void setHispanicEthnicity(String hispanicEthnicity) {
this.hispanicEthnicity = hispanicEthnicity;
}
public Student iepStatus(String iepStatus) {
this.iepStatus = iepStatus;
return this;
}
/**
* Get iepStatus
* @return iepStatus
**/
@ApiModelProperty(value = "")
public String getIepStatus() {
return iepStatus;
}
public void setIepStatus(String iepStatus) {
this.iepStatus = iepStatus;
}
public Student lastModified(String lastModified) {
this.lastModified = lastModified;
return this;
}
/**
* Get lastModified
* @return lastModified
**/
@ApiModelProperty(value = "")
public String getLastModified() {
return lastModified;
}
public void setLastModified(String lastModified) {
this.lastModified = lastModified;
}
public Student location(Location location) {
this.location = location;
return this;
}
/**
* Get location
* @return location
**/
@ApiModelProperty(value = "")
public Location getLocation() {
return location;
}
public void setLocation(Location location) {
this.location = location;
}
public Student name(Name name) {
this.name = name;
return this;
}
/**
* Get name
* @return name
**/
@ApiModelProperty(value = "")
public Name getName() {
return name;
}
public void setName(Name name) {
this.name = name;
}
public Student race(String race) {
this.race = race;
return this;
}
/**
* Get race
* @return race
**/
@ApiModelProperty(value = "")
public String getRace() {
return race;
}
public void setRace(String race) {
this.race = race;
}
public Student school(String school) {
this.school = school;
return this;
}
/**
* Get school
* @return school
**/
@ApiModelProperty(value = "")
public String getSchool() {
return school;
}
public void setSchool(String school) {
this.school = school;
}
public Student schools(List schools) {
this.schools = schools;
return this;
}
public Student addSchoolsItem(String schoolsItem) {
this.schools.add(schoolsItem);
return this;
}
/**
* Get schools
* @return schools
**/
@ApiModelProperty(value = "")
public List getSchools() {
return schools;
}
public void setSchools(List schools) {
this.schools = schools;
}
public Student sisId(String sisId) {
this.sisId = sisId;
return this;
}
/**
* Get sisId
* @return sisId
**/
@ApiModelProperty(value = "")
public String getSisId() {
return sisId;
}
public void setSisId(String sisId) {
this.sisId = sisId;
}
public Student stateId(String stateId) {
this.stateId = stateId;
return this;
}
/**
* Get stateId
* @return stateId
**/
@ApiModelProperty(value = "")
public String getStateId() {
return stateId;
}
public void setStateId(String stateId) {
this.stateId = stateId;
}
public Student studentNumber(String studentNumber) {
this.studentNumber = studentNumber;
return this;
}
/**
* Get studentNumber
* @return studentNumber
**/
@ApiModelProperty(value = "")
public String getStudentNumber() {
return studentNumber;
}
public void setStudentNumber(String studentNumber) {
this.studentNumber = studentNumber;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Student student = (Student) o;
return Objects.equals(this.id, student.id) &&
Objects.equals(this.created, student.created) &&
Objects.equals(this.district, student.district) &&
Objects.equals(this.dob, student.dob) &&
Objects.equals(this.ellStatus, student.ellStatus) &&
Objects.equals(this.email, student.email) &&
Objects.equals(this.frlStatus, student.frlStatus) &&
Objects.equals(this.gender, student.gender) &&
Objects.equals(this.grade, student.grade) &&
Objects.equals(this.hispanicEthnicity, student.hispanicEthnicity) &&
Objects.equals(this.iepStatus, student.iepStatus) &&
Objects.equals(this.lastModified, student.lastModified) &&
Objects.equals(this.location, student.location) &&
Objects.equals(this.name, student.name) &&
Objects.equals(this.race, student.race) &&
Objects.equals(this.school, student.school) &&
Objects.equals(this.schools, student.schools) &&
Objects.equals(this.sisId, student.sisId) &&
Objects.equals(this.stateId, student.stateId) &&
Objects.equals(this.studentNumber, student.studentNumber);
}
@Override
public int hashCode() {
return Objects.hash(id, created, district, dob, ellStatus, email, frlStatus, gender, grade, hispanicEthnicity, iepStatus, lastModified, location, name, race, school, schools, sisId, stateId, studentNumber);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Student {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" created: ").append(toIndentedString(created)).append("\n");
sb.append(" district: ").append(toIndentedString(district)).append("\n");
sb.append(" dob: ").append(toIndentedString(dob)).append("\n");
sb.append(" ellStatus: ").append(toIndentedString(ellStatus)).append("\n");
sb.append(" email: ").append(toIndentedString(email)).append("\n");
sb.append(" frlStatus: ").append(toIndentedString(frlStatus)).append("\n");
sb.append(" gender: ").append(toIndentedString(gender)).append("\n");
sb.append(" grade: ").append(toIndentedString(grade)).append("\n");
sb.append(" hispanicEthnicity: ").append(toIndentedString(hispanicEthnicity)).append("\n");
sb.append(" iepStatus: ").append(toIndentedString(iepStatus)).append("\n");
sb.append(" lastModified: ").append(toIndentedString(lastModified)).append("\n");
sb.append(" location: ").append(toIndentedString(location)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" race: ").append(toIndentedString(race)).append("\n");
sb.append(" school: ").append(toIndentedString(school)).append("\n");
sb.append(" schools: ").append(toIndentedString(schools)).append("\n");
sb.append(" sisId: ").append(toIndentedString(sisId)).append("\n");
sb.append(" stateId: ").append(toIndentedString(stateId)).append("\n");
sb.append(" studentNumber: ").append(toIndentedString(studentNumber)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy