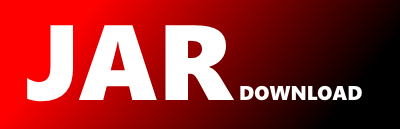
com.cleverpine.cpspringdatacrud.service.impl.CRUDServiceImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cp-spring-data-crud Show documentation
Show all versions of cp-spring-data-crud Show documentation
Library providing generic classes and implementation for basic CRUD operations
The newest version!
package com.cleverpine.cpspringdatacrud.service.impl;
import com.cleverpine.cpspringdatacrud.mapper.BusinessLayerMapper;
import com.cleverpine.cpspringdatacrud.repository.CommonRepository;
import com.cleverpine.cpspringdatacrud.service.contract.CRUDService;
import lombok.RequiredArgsConstructor;
import org.springframework.stereotype.Service;
import javax.persistence.EntityNotFoundException;
import java.util.List;
import java.util.stream.Collectors;
@Service
@RequiredArgsConstructor
public abstract class CRUDServiceImpl implements CRUDService {
public static final String ENTITY_NOT_FOUND_ERROR_MESSAGE = "Could not find %s with id: [%s]";
private final CommonRepository repository;
private final BusinessLayerMapper mapper;
private final Class entityClass;
@Override
public BusinessLayerDTO get(EntityId id) {
return mapper.entityToBusinessLayerDTO(this.findById(id));
}
@Override
public List getAll() {
return repository.findAll()
.stream()
.map(mapper::entityToBusinessLayerDTO)
.collect(Collectors.toList());
}
@Override
public BusinessLayerDTO update(EntityId id, BusinessLayerDTO businessLayerDto) {
var updatedEntity = mapper.businessLayerDTOToEntity(this.findById(id), businessLayerDto);
repository.save(updatedEntity);
return mapper.entityToBusinessLayerDTO(updatedEntity);
}
@Override
public BusinessLayerDTO create(BusinessLayerDTO businessLayerDto) {
var entity = mapper.businessLayerDTOToEntity(businessLayerDto);
repository.save(entity);
return mapper.entityToBusinessLayerDTO(entity);
}
@Override
public void delete(EntityId id) {
this.findById(id);
repository.deleteById(id);
}
protected Entity findById(EntityId id) {
return repository.findById(id)
.orElseThrow(() -> new EntityNotFoundException(
String.format(ENTITY_NOT_FOUND_ERROR_MESSAGE, entityClass.getSimpleName(), id)));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy