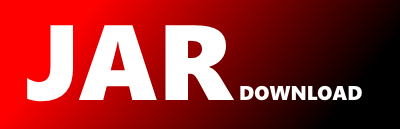
com.cleverpine.cpspringdatacrud.test.CRUDServiceImplTest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cp-spring-data-crud Show documentation
Show all versions of cp-spring-data-crud Show documentation
Library providing generic classes and implementation for basic CRUD operations
The newest version!
package com.cleverpine.cpspringdatacrud.test;
import com.cleverpine.cpspringdatacrud.mapper.BusinessLayerMapper;
import com.cleverpine.cpspringdatacrud.repository.CommonRepository;
import com.cleverpine.cpspringdatacrud.service.impl.CRUDServiceImpl;
import org.junit.jupiter.api.Test;
import org.junit.jupiter.api.function.Executable;
import javax.persistence.EntityNotFoundException;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import static com.cleverpine.cpspringdatacrud.service.impl.CRUDServiceImpl.ENTITY_NOT_FOUND_ERROR_MESSAGE;
import static org.junit.jupiter.api.Assertions.*;
import static org.mockito.ArgumentMatchers.any;
import static org.mockito.Mockito.*;
public abstract class CRUDServiceImplTest {
private CommonRepository mockRepository;
private BusinessLayerMapper mockMapper;
protected Class entityClass;
private CRUDServiceImpl crudService;
protected void setUp(CommonRepository mockRepository,
BusinessLayerMapper mockMapper,
Class entityClass,
CRUDServiceImpl crudService) {
this.mockRepository = mockRepository;
this.mockMapper = mockMapper;
this.entityClass = entityClass;
this.crudService = crudService;
}
protected abstract Entity getEntity();
protected abstract EntityId getEntityId();
protected abstract BusinessLayerDTO getBusinessLayerDTO();
protected abstract Class getBusinessLayerDTOClass();
@Test
void getTest_onEntityNotFound_shouldThrow() {
assertEntityNotFound(() -> crudService.get(getEntityId()));
}
@Test
void getTest_positiveCase() {
var businessLayerDTO = getBusinessLayerDTO();
mockFindByIdResult(Optional.of(getEntity()));
when(mockMapper.entityToBusinessLayerDTO(any(entityClass))).thenReturn(businessLayerDTO);
var result = crudService.get(getEntityId());
assertEquals(businessLayerDTO, result);
}
@Test
void getAllTest_onEmptyResponse_shouldReturnEmpty() {
when(mockRepository.findAll()).thenReturn(Collections.emptyList());
List result = crudService.getAll();
verify(mockMapper, times(0)).entityToBusinessLayerDTO(any(entityClass));
assertTrue(result.isEmpty());
}
@Test
void getAll_positiveCase() {
var businessLayerDTO = getBusinessLayerDTO();
when(mockRepository.findAll()).thenReturn(List.of(getEntity()));
when(mockMapper.entityToBusinessLayerDTO(any(entityClass))).thenReturn(businessLayerDTO);
List result = crudService.getAll();
assertFalse(result.isEmpty());
assertEquals(businessLayerDTO, result.get(0));
}
@Test
void updateTest_onEntityNotFound_shouldThrow() {
assertEntityNotFound(() -> crudService.update(getEntityId(), getBusinessLayerDTO()));
}
@Test
void updateTest_positiveCase() {
var entity = getEntity();
var businessLayerDTO = getBusinessLayerDTO();
mockFindByIdResult(Optional.of(entity));
when(mockMapper.businessLayerDTOToEntity(any(entityClass), any(getBusinessLayerDTOClass()))).thenReturn(entity);
when(mockMapper.entityToBusinessLayerDTO(any(entityClass))).thenReturn(businessLayerDTO);
var result = crudService.update(getEntityId(), businessLayerDTO);
verify(mockRepository).save(any(entityClass));
assertEquals(businessLayerDTO, result);
}
@Test
void createTest_positiveCase() {
var businessLayerDTO = getBusinessLayerDTO();
when(mockMapper.businessLayerDTOToEntity(any(getBusinessLayerDTOClass()))).thenReturn(getEntity());
when(mockMapper.entityToBusinessLayerDTO(any(entityClass))).thenReturn(businessLayerDTO);
var result = crudService.create(businessLayerDTO);
verify(mockRepository).save(any(entityClass));
assertEquals(businessLayerDTO, result);
}
@Test
void deleteTest_onEntityNotFound_shouldThrow() {
assertEntityNotFound(() -> crudService.delete(getEntityId()));
}
@Test
void deleteTest_positiveCase() {
var entityId = getEntityId();
mockFindByIdResult(Optional.of(getEntity()));
crudService.delete(entityId);
verify(mockRepository).deleteById(entityId);
}
private void mockFindByIdResult(Optional optionalEntity) {
when(mockRepository.findById(any())).thenReturn(optionalEntity);
}
private void assertEntityNotFound(Executable executable) {
mockFindByIdResult(Optional.empty());
var entityNotFoundException = assertThrows(EntityNotFoundException.class, executable);
assertEquals(String.format(ENTITY_NOT_FOUND_ERROR_MESSAGE, entityClass.getSimpleName(), getEntityId()),
entityNotFoundException.getMessage());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy