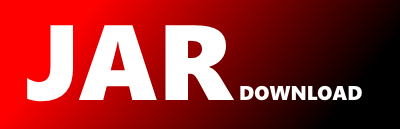
com.cleverpine.viravaspringhelper.aop.BaseViravaSecuredAspect Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cp-virava-spring-helper Show documentation
Show all versions of cp-virava-spring-helper Show documentation
Library for Spring Framework projects using Virava
package com.cleverpine.viravaspringhelper.aop;
import com.cleverpine.cpspringerrorutil.exception.AuthorisationException;
import com.cleverpine.viravaspringhelper.core.BaseResource;
import com.cleverpine.viravaspringhelper.core.ViravaPrincipalProvider;
import com.cleverpine.viravaspringhelper.dto.ScopeType;
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.reflect.MethodSignature;
public class BaseViravaSecuredAspect {
private final ViravaPrincipalProvider> viravaPrincipalProvider;
public BaseViravaSecuredAspect(ViravaPrincipalProvider> viravaPrincipalProvider) {
this.viravaPrincipalProvider = viravaPrincipalProvider;
}
protected void authorise(
JoinPoint joinPoint,
BaseResource resource,
String resourceIdParamName,
boolean requireAllResourceIds,
ScopeType[] scopeList) {
var authentication = viravaPrincipalProvider.getAuthentication()
.orElseThrow(() -> new AuthorisationException("Invalid SecurityContextHolder"));
var principal = authentication.getPrincipal();
if (principal == null) {
throw new AuthorisationException("Missing ViravaUserPrincipal on method requiring authorisation");
}
Long resourceId = getMethodSuppliedResourceId(joinPoint, resourceIdParamName);
if (!principal.authorized(resource, resourceId, requireAllResourceIds, scopeList)) {
throw new AuthorisationException("User doesn't have required permissions");
}
}
private Long getMethodSuppliedResourceId(JoinPoint joinPoint, String resourceIdParamName) {
if (resourceIdParamName == null || resourceIdParamName.isEmpty()) {
return null;
}
var methodSig = (MethodSignature) joinPoint.getSignature();
var parameters = methodSig.getParameterNames();
if (parameters == null) {
throw new AssertionError("ViravaSecuredAsspect::authorise called on a method without parameters");
}
var args = joinPoint.getArgs();
if (args == null) {
throw new AssertionError("ViravaSecuredAsspect::authorise called without arguments");
}
if (args.length != parameters.length) {
throw new AssertionError("ViravaSecuredAsspect::authorise parameter count does not match arg count");
}
Long resourceId = null;
for (int i = 0; i < parameters.length; i++) {
if (parameters[i].equals(resourceIdParamName)) {
var arg = args[i];
if (arg instanceof Long) {
resourceId = (Long) arg;
}
break;
}
}
return resourceId;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy