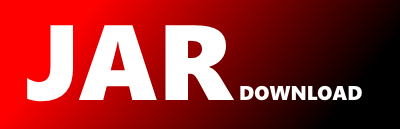
com.clickhouse.client.grpc.impl.QueryInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickhouse-grpc-client Show documentation
Show all versions of clickhouse-grpc-client Show documentation
gRPC client for ClickHouse planed to be deprecated from version 0.6.0 and removed at 0.7.0
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: clickhouse_grpc.proto
package com.clickhouse.client.grpc.impl;
/**
*
* Information about a query which a client sends to a ClickHouse server.
* The first QueryInfo can set any of the following fields. Extra QueryInfos only add extra data.
* In extra QueryInfos only `input_data`, `external_tables`, `next_query_info` and `cancel` fields can be set.
*
*
* Protobuf type {@code clickhouse.grpc.QueryInfo}
*/
public final class QueryInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:clickhouse.grpc.QueryInfo)
QueryInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use QueryInfo.newBuilder() to construct.
private QueryInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private QueryInfo() {
query_ = "";
queryId_ = "";
database_ = "";
inputData_ = com.google.protobuf.ByteString.EMPTY;
inputDataDelimiter_ = com.google.protobuf.ByteString.EMPTY;
outputFormat_ = "";
externalTables_ = java.util.Collections.emptyList();
userName_ = "";
password_ = "";
quota_ = "";
sessionId_ = "";
inputCompressionType_ = "";
outputCompressionType_ = "";
transportCompressionType_ = "";
obsoleteCompressionType_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new QueryInfo();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.clickhouse.client.grpc.impl.ClickHouseGrpcImpl.internal_static_clickhouse_grpc_QueryInfo_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 3:
return internalGetSettings();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.clickhouse.client.grpc.impl.ClickHouseGrpcImpl.internal_static_clickhouse_grpc_QueryInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.clickhouse.client.grpc.impl.QueryInfo.class, com.clickhouse.client.grpc.impl.QueryInfo.Builder.class);
}
public static final int QUERY_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object query_ = "";
/**
* string query = 1;
* @return The query.
*/
@java.lang.Override
public java.lang.String getQuery() {
java.lang.Object ref = query_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
query_ = s;
return s;
}
}
/**
* string query = 1;
* @return The bytes for query.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getQueryBytes() {
java.lang.Object ref = query_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
query_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int QUERY_ID_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object queryId_ = "";
/**
* string query_id = 2;
* @return The queryId.
*/
@java.lang.Override
public java.lang.String getQueryId() {
java.lang.Object ref = queryId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
queryId_ = s;
return s;
}
}
/**
* string query_id = 2;
* @return The bytes for queryId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getQueryIdBytes() {
java.lang.Object ref = queryId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
queryId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SETTINGS_FIELD_NUMBER = 3;
private static final class SettingsDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, java.lang.String> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
com.clickhouse.client.grpc.impl.ClickHouseGrpcImpl.internal_static_clickhouse_grpc_QueryInfo_SettingsEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.STRING,
"");
}
@SuppressWarnings("serial")
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> settings_;
private com.google.protobuf.MapField
internalGetSettings() {
if (settings_ == null) {
return com.google.protobuf.MapField.emptyMapField(
SettingsDefaultEntryHolder.defaultEntry);
}
return settings_;
}
public int getSettingsCount() {
return internalGetSettings().getMap().size();
}
/**
* map<string, string> settings = 3;
*/
@java.lang.Override
public boolean containsSettings(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetSettings().getMap().containsKey(key);
}
/**
* Use {@link #getSettingsMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getSettings() {
return getSettingsMap();
}
/**
* map<string, string> settings = 3;
*/
@java.lang.Override
public java.util.Map getSettingsMap() {
return internalGetSettings().getMap();
}
/**
* map<string, string> settings = 3;
*/
@java.lang.Override
public /* nullable */
java.lang.String getSettingsOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetSettings().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<string, string> settings = 3;
*/
@java.lang.Override
public java.lang.String getSettingsOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetSettings().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int DATABASE_FIELD_NUMBER = 4;
@SuppressWarnings("serial")
private volatile java.lang.Object database_ = "";
/**
*
* Default database.
*
*
* string database = 4;
* @return The database.
*/
@java.lang.Override
public java.lang.String getDatabase() {
java.lang.Object ref = database_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
database_ = s;
return s;
}
}
/**
*
* Default database.
*
*
* string database = 4;
* @return The bytes for database.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getDatabaseBytes() {
java.lang.Object ref = database_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
database_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int INPUT_DATA_FIELD_NUMBER = 5;
private com.google.protobuf.ByteString inputData_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Input data, used both as data for INSERT query and as data for the input() function.
*
*
* bytes input_data = 5;
* @return The inputData.
*/
@java.lang.Override
public com.google.protobuf.ByteString getInputData() {
return inputData_;
}
public static final int INPUT_DATA_DELIMITER_FIELD_NUMBER = 6;
private com.google.protobuf.ByteString inputDataDelimiter_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Delimiter for input_data, inserted between input_data from adjacent QueryInfos.
*
*
* bytes input_data_delimiter = 6;
* @return The inputDataDelimiter.
*/
@java.lang.Override
public com.google.protobuf.ByteString getInputDataDelimiter() {
return inputDataDelimiter_;
}
public static final int OUTPUT_FORMAT_FIELD_NUMBER = 7;
@SuppressWarnings("serial")
private volatile java.lang.Object outputFormat_ = "";
/**
*
* Default output format. If not specified, 'TabSeparated' is used.
*
*
* string output_format = 7;
* @return The outputFormat.
*/
@java.lang.Override
public java.lang.String getOutputFormat() {
java.lang.Object ref = outputFormat_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
outputFormat_ = s;
return s;
}
}
/**
*
* Default output format. If not specified, 'TabSeparated' is used.
*
*
* string output_format = 7;
* @return The bytes for outputFormat.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getOutputFormatBytes() {
java.lang.Object ref = outputFormat_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
outputFormat_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SEND_OUTPUT_COLUMNS_FIELD_NUMBER = 24;
private boolean sendOutputColumns_ = false;
/**
*
* Set it if you want the names and the types of output columns to be sent to the client.
*
*
* bool send_output_columns = 24;
* @return The sendOutputColumns.
*/
@java.lang.Override
public boolean getSendOutputColumns() {
return sendOutputColumns_;
}
public static final int EXTERNAL_TABLES_FIELD_NUMBER = 8;
@SuppressWarnings("serial")
private java.util.List externalTables_;
/**
* repeated .clickhouse.grpc.ExternalTable external_tables = 8;
*/
@java.lang.Override
public java.util.List getExternalTablesList() {
return externalTables_;
}
/**
* repeated .clickhouse.grpc.ExternalTable external_tables = 8;
*/
@java.lang.Override
public java.util.List extends com.clickhouse.client.grpc.impl.ExternalTableOrBuilder>
getExternalTablesOrBuilderList() {
return externalTables_;
}
/**
* repeated .clickhouse.grpc.ExternalTable external_tables = 8;
*/
@java.lang.Override
public int getExternalTablesCount() {
return externalTables_.size();
}
/**
* repeated .clickhouse.grpc.ExternalTable external_tables = 8;
*/
@java.lang.Override
public com.clickhouse.client.grpc.impl.ExternalTable getExternalTables(int index) {
return externalTables_.get(index);
}
/**
* repeated .clickhouse.grpc.ExternalTable external_tables = 8;
*/
@java.lang.Override
public com.clickhouse.client.grpc.impl.ExternalTableOrBuilder getExternalTablesOrBuilder(
int index) {
return externalTables_.get(index);
}
public static final int USER_NAME_FIELD_NUMBER = 9;
@SuppressWarnings("serial")
private volatile java.lang.Object userName_ = "";
/**
* string user_name = 9;
* @return The userName.
*/
@java.lang.Override
public java.lang.String getUserName() {
java.lang.Object ref = userName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
userName_ = s;
return s;
}
}
/**
* string user_name = 9;
* @return The bytes for userName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getUserNameBytes() {
java.lang.Object ref = userName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
userName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PASSWORD_FIELD_NUMBER = 10;
@SuppressWarnings("serial")
private volatile java.lang.Object password_ = "";
/**
* string password = 10;
* @return The password.
*/
@java.lang.Override
public java.lang.String getPassword() {
java.lang.Object ref = password_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
password_ = s;
return s;
}
}
/**
* string password = 10;
* @return The bytes for password.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getPasswordBytes() {
java.lang.Object ref = password_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
password_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int QUOTA_FIELD_NUMBER = 11;
@SuppressWarnings("serial")
private volatile java.lang.Object quota_ = "";
/**
* string quota = 11;
* @return The quota.
*/
@java.lang.Override
public java.lang.String getQuota() {
java.lang.Object ref = quota_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
quota_ = s;
return s;
}
}
/**
* string quota = 11;
* @return The bytes for quota.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getQuotaBytes() {
java.lang.Object ref = quota_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
quota_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SESSION_ID_FIELD_NUMBER = 12;
@SuppressWarnings("serial")
private volatile java.lang.Object sessionId_ = "";
/**
*
* Works exactly like sessions in the HTTP protocol.
*
*
* string session_id = 12;
* @return The sessionId.
*/
@java.lang.Override
public java.lang.String getSessionId() {
java.lang.Object ref = sessionId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sessionId_ = s;
return s;
}
}
/**
*
* Works exactly like sessions in the HTTP protocol.
*
*
* string session_id = 12;
* @return The bytes for sessionId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSessionIdBytes() {
java.lang.Object ref = sessionId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sessionId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SESSION_CHECK_FIELD_NUMBER = 13;
private boolean sessionCheck_ = false;
/**
* bool session_check = 13;
* @return The sessionCheck.
*/
@java.lang.Override
public boolean getSessionCheck() {
return sessionCheck_;
}
public static final int SESSION_TIMEOUT_FIELD_NUMBER = 14;
private int sessionTimeout_ = 0;
/**
* uint32 session_timeout = 14;
* @return The sessionTimeout.
*/
@java.lang.Override
public int getSessionTimeout() {
return sessionTimeout_;
}
public static final int CANCEL_FIELD_NUMBER = 15;
private boolean cancel_ = false;
/**
*
* Set `cancel` to true to stop executing the query.
*
*
* bool cancel = 15;
* @return The cancel.
*/
@java.lang.Override
public boolean getCancel() {
return cancel_;
}
public static final int NEXT_QUERY_INFO_FIELD_NUMBER = 16;
private boolean nextQueryInfo_ = false;
/**
*
* If true there will be at least one more QueryInfo in the input stream.
* `next_query_info` is allowed to be set only if a method with streaming input (i.e. ExecuteQueryWithStreamInput() or ExecuteQueryWithStreamIO()) is used.
*
*
* bool next_query_info = 16;
* @return The nextQueryInfo.
*/
@java.lang.Override
public boolean getNextQueryInfo() {
return nextQueryInfo_;
}
public static final int INPUT_COMPRESSION_TYPE_FIELD_NUMBER = 20;
@SuppressWarnings("serial")
private volatile java.lang.Object inputCompressionType_ = "";
/**
*
* Compression type for `input_data`.
* Supported compression types: none, gzip(gz), deflate, brotli(br), lzma(xz), zstd(zst), lz4, bz2.
* The client is responsible to compress data before putting it into `input_data`.
*
*
* string input_compression_type = 20;
* @return The inputCompressionType.
*/
@java.lang.Override
public java.lang.String getInputCompressionType() {
java.lang.Object ref = inputCompressionType_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
inputCompressionType_ = s;
return s;
}
}
/**
*
* Compression type for `input_data`.
* Supported compression types: none, gzip(gz), deflate, brotli(br), lzma(xz), zstd(zst), lz4, bz2.
* The client is responsible to compress data before putting it into `input_data`.
*
*
* string input_compression_type = 20;
* @return The bytes for inputCompressionType.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getInputCompressionTypeBytes() {
java.lang.Object ref = inputCompressionType_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
inputCompressionType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int OUTPUT_COMPRESSION_TYPE_FIELD_NUMBER = 21;
@SuppressWarnings("serial")
private volatile java.lang.Object outputCompressionType_ = "";
/**
*
* Compression type for `output_data`, `totals` and `extremes`.
* Supported compression types: none, gzip(gz), deflate, brotli(br), lzma(xz), zstd(zst), lz4, bz2.
* The client receives compressed data and should decompress it by itself.
* Consider also setting `output_compression_level`.
*
*
* string output_compression_type = 21;
* @return The outputCompressionType.
*/
@java.lang.Override
public java.lang.String getOutputCompressionType() {
java.lang.Object ref = outputCompressionType_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
outputCompressionType_ = s;
return s;
}
}
/**
*
* Compression type for `output_data`, `totals` and `extremes`.
* Supported compression types: none, gzip(gz), deflate, brotli(br), lzma(xz), zstd(zst), lz4, bz2.
* The client receives compressed data and should decompress it by itself.
* Consider also setting `output_compression_level`.
*
*
* string output_compression_type = 21;
* @return The bytes for outputCompressionType.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getOutputCompressionTypeBytes() {
java.lang.Object ref = outputCompressionType_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
outputCompressionType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int OUTPUT_COMPRESSION_LEVEL_FIELD_NUMBER = 19;
private int outputCompressionLevel_ = 0;
/**
*
* Compression level.
* WARNING: If it's not specified the compression level is set to zero by default which might be not the best choice for some compression types (see below).
* The compression level should be in the following range (the higher the number, the better the compression):
* none: compression level isn't used
* gzip: 0..9; 0 means no compression, 6 is recommended by default (compression level -1 also means 6)
* brotli: 0..11
* lzma: 0..9; 6 is recommended by default
* zstd: 1..22; 3 is recommended by default (compression level 0 also means 3)
* lz4: 0..16; values < 0 mean fast acceleration
* bz2: 1..9
*
*
* int32 output_compression_level = 19;
* @return The outputCompressionLevel.
*/
@java.lang.Override
public int getOutputCompressionLevel() {
return outputCompressionLevel_;
}
public static final int TRANSPORT_COMPRESSION_TYPE_FIELD_NUMBER = 22;
@SuppressWarnings("serial")
private volatile java.lang.Object transportCompressionType_ = "";
/**
*
* Transport compression is an alternative way to make the server to compress its response.
* This kind of compression implies that instead of compressing just `output` the server will compress whole packed messages of the `Result` type,
* and then gRPC implementation on client side will decompress those messages so client code won't be bothered with decompression.
* Here is a big difference between the transport compression and the compression enabled by setting `output_compression_type` because
* in case of the transport compression the client code receives already decompressed data in `output`.
* If the transport compression is not set here it can still be enabled by the server configuration.
* Supported compression types: none, deflate, gzip, stream_gzip
* Supported compression levels: 0..3
* WARNING: Don't set `transport_compression` and `output_compression` at the same time because it will make the server to compress its output twice!
*
*
* string transport_compression_type = 22;
* @return The transportCompressionType.
*/
@java.lang.Override
public java.lang.String getTransportCompressionType() {
java.lang.Object ref = transportCompressionType_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
transportCompressionType_ = s;
return s;
}
}
/**
*
* Transport compression is an alternative way to make the server to compress its response.
* This kind of compression implies that instead of compressing just `output` the server will compress whole packed messages of the `Result` type,
* and then gRPC implementation on client side will decompress those messages so client code won't be bothered with decompression.
* Here is a big difference between the transport compression and the compression enabled by setting `output_compression_type` because
* in case of the transport compression the client code receives already decompressed data in `output`.
* If the transport compression is not set here it can still be enabled by the server configuration.
* Supported compression types: none, deflate, gzip, stream_gzip
* Supported compression levels: 0..3
* WARNING: Don't set `transport_compression` and `output_compression` at the same time because it will make the server to compress its output twice!
*
*
* string transport_compression_type = 22;
* @return The bytes for transportCompressionType.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTransportCompressionTypeBytes() {
java.lang.Object ref = transportCompressionType_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
transportCompressionType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TRANSPORT_COMPRESSION_LEVEL_FIELD_NUMBER = 23;
private int transportCompressionLevel_ = 0;
/**
* int32 transport_compression_level = 23;
* @return The transportCompressionLevel.
*/
@java.lang.Override
public int getTransportCompressionLevel() {
return transportCompressionLevel_;
}
public static final int OBSOLETE_RESULT_COMPRESSION_FIELD_NUMBER = 17;
private com.clickhouse.client.grpc.impl.ObsoleteTransportCompression obsoleteResultCompression_;
/**
*
*/ Obsolete fields, should not be used in new code.
*
*
* .clickhouse.grpc.ObsoleteTransportCompression obsolete_result_compression = 17;
* @return Whether the obsoleteResultCompression field is set.
*/
@java.lang.Override
public boolean hasObsoleteResultCompression() {
return obsoleteResultCompression_ != null;
}
/**
*
*/ Obsolete fields, should not be used in new code.
*
*
* .clickhouse.grpc.ObsoleteTransportCompression obsolete_result_compression = 17;
* @return The obsoleteResultCompression.
*/
@java.lang.Override
public com.clickhouse.client.grpc.impl.ObsoleteTransportCompression getObsoleteResultCompression() {
return obsoleteResultCompression_ == null ? com.clickhouse.client.grpc.impl.ObsoleteTransportCompression.getDefaultInstance() : obsoleteResultCompression_;
}
/**
*
*/ Obsolete fields, should not be used in new code.
*
*
* .clickhouse.grpc.ObsoleteTransportCompression obsolete_result_compression = 17;
*/
@java.lang.Override
public com.clickhouse.client.grpc.impl.ObsoleteTransportCompressionOrBuilder getObsoleteResultCompressionOrBuilder() {
return obsoleteResultCompression_ == null ? com.clickhouse.client.grpc.impl.ObsoleteTransportCompression.getDefaultInstance() : obsoleteResultCompression_;
}
public static final int OBSOLETE_COMPRESSION_TYPE_FIELD_NUMBER = 18;
@SuppressWarnings("serial")
private volatile java.lang.Object obsoleteCompressionType_ = "";
/**
* string obsolete_compression_type = 18;
* @return The obsoleteCompressionType.
*/
@java.lang.Override
public java.lang.String getObsoleteCompressionType() {
java.lang.Object ref = obsoleteCompressionType_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
obsoleteCompressionType_ = s;
return s;
}
}
/**
* string obsolete_compression_type = 18;
* @return The bytes for obsoleteCompressionType.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getObsoleteCompressionTypeBytes() {
java.lang.Object ref = obsoleteCompressionType_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
obsoleteCompressionType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(query_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, query_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(queryId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, queryId_);
}
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetSettings(),
SettingsDefaultEntryHolder.defaultEntry,
3);
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(database_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, database_);
}
if (!inputData_.isEmpty()) {
output.writeBytes(5, inputData_);
}
if (!inputDataDelimiter_.isEmpty()) {
output.writeBytes(6, inputDataDelimiter_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(outputFormat_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 7, outputFormat_);
}
for (int i = 0; i < externalTables_.size(); i++) {
output.writeMessage(8, externalTables_.get(i));
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(userName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 9, userName_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(password_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 10, password_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(quota_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 11, quota_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(sessionId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 12, sessionId_);
}
if (sessionCheck_ != false) {
output.writeBool(13, sessionCheck_);
}
if (sessionTimeout_ != 0) {
output.writeUInt32(14, sessionTimeout_);
}
if (cancel_ != false) {
output.writeBool(15, cancel_);
}
if (nextQueryInfo_ != false) {
output.writeBool(16, nextQueryInfo_);
}
if (obsoleteResultCompression_ != null) {
output.writeMessage(17, getObsoleteResultCompression());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(obsoleteCompressionType_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 18, obsoleteCompressionType_);
}
if (outputCompressionLevel_ != 0) {
output.writeInt32(19, outputCompressionLevel_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(inputCompressionType_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 20, inputCompressionType_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(outputCompressionType_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 21, outputCompressionType_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(transportCompressionType_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 22, transportCompressionType_);
}
if (transportCompressionLevel_ != 0) {
output.writeInt32(23, transportCompressionLevel_);
}
if (sendOutputColumns_ != false) {
output.writeBool(24, sendOutputColumns_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(query_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, query_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(queryId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, queryId_);
}
for (java.util.Map.Entry entry
: internalGetSettings().getMap().entrySet()) {
com.google.protobuf.MapEntry
settings__ = SettingsDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, settings__);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(database_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, database_);
}
if (!inputData_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(5, inputData_);
}
if (!inputDataDelimiter_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(6, inputDataDelimiter_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(outputFormat_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(7, outputFormat_);
}
for (int i = 0; i < externalTables_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, externalTables_.get(i));
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(userName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(9, userName_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(password_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(10, password_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(quota_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(11, quota_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(sessionId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(12, sessionId_);
}
if (sessionCheck_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(13, sessionCheck_);
}
if (sessionTimeout_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(14, sessionTimeout_);
}
if (cancel_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(15, cancel_);
}
if (nextQueryInfo_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(16, nextQueryInfo_);
}
if (obsoleteResultCompression_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(17, getObsoleteResultCompression());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(obsoleteCompressionType_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(18, obsoleteCompressionType_);
}
if (outputCompressionLevel_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(19, outputCompressionLevel_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(inputCompressionType_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(20, inputCompressionType_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(outputCompressionType_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(21, outputCompressionType_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(transportCompressionType_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(22, transportCompressionType_);
}
if (transportCompressionLevel_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(23, transportCompressionLevel_);
}
if (sendOutputColumns_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(24, sendOutputColumns_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.clickhouse.client.grpc.impl.QueryInfo)) {
return super.equals(obj);
}
com.clickhouse.client.grpc.impl.QueryInfo other = (com.clickhouse.client.grpc.impl.QueryInfo) obj;
if (!getQuery()
.equals(other.getQuery())) return false;
if (!getQueryId()
.equals(other.getQueryId())) return false;
if (!internalGetSettings().equals(
other.internalGetSettings())) return false;
if (!getDatabase()
.equals(other.getDatabase())) return false;
if (!getInputData()
.equals(other.getInputData())) return false;
if (!getInputDataDelimiter()
.equals(other.getInputDataDelimiter())) return false;
if (!getOutputFormat()
.equals(other.getOutputFormat())) return false;
if (getSendOutputColumns()
!= other.getSendOutputColumns()) return false;
if (!getExternalTablesList()
.equals(other.getExternalTablesList())) return false;
if (!getUserName()
.equals(other.getUserName())) return false;
if (!getPassword()
.equals(other.getPassword())) return false;
if (!getQuota()
.equals(other.getQuota())) return false;
if (!getSessionId()
.equals(other.getSessionId())) return false;
if (getSessionCheck()
!= other.getSessionCheck()) return false;
if (getSessionTimeout()
!= other.getSessionTimeout()) return false;
if (getCancel()
!= other.getCancel()) return false;
if (getNextQueryInfo()
!= other.getNextQueryInfo()) return false;
if (!getInputCompressionType()
.equals(other.getInputCompressionType())) return false;
if (!getOutputCompressionType()
.equals(other.getOutputCompressionType())) return false;
if (getOutputCompressionLevel()
!= other.getOutputCompressionLevel()) return false;
if (!getTransportCompressionType()
.equals(other.getTransportCompressionType())) return false;
if (getTransportCompressionLevel()
!= other.getTransportCompressionLevel()) return false;
if (hasObsoleteResultCompression() != other.hasObsoleteResultCompression()) return false;
if (hasObsoleteResultCompression()) {
if (!getObsoleteResultCompression()
.equals(other.getObsoleteResultCompression())) return false;
}
if (!getObsoleteCompressionType()
.equals(other.getObsoleteCompressionType())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + QUERY_FIELD_NUMBER;
hash = (53 * hash) + getQuery().hashCode();
hash = (37 * hash) + QUERY_ID_FIELD_NUMBER;
hash = (53 * hash) + getQueryId().hashCode();
if (!internalGetSettings().getMap().isEmpty()) {
hash = (37 * hash) + SETTINGS_FIELD_NUMBER;
hash = (53 * hash) + internalGetSettings().hashCode();
}
hash = (37 * hash) + DATABASE_FIELD_NUMBER;
hash = (53 * hash) + getDatabase().hashCode();
hash = (37 * hash) + INPUT_DATA_FIELD_NUMBER;
hash = (53 * hash) + getInputData().hashCode();
hash = (37 * hash) + INPUT_DATA_DELIMITER_FIELD_NUMBER;
hash = (53 * hash) + getInputDataDelimiter().hashCode();
hash = (37 * hash) + OUTPUT_FORMAT_FIELD_NUMBER;
hash = (53 * hash) + getOutputFormat().hashCode();
hash = (37 * hash) + SEND_OUTPUT_COLUMNS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getSendOutputColumns());
if (getExternalTablesCount() > 0) {
hash = (37 * hash) + EXTERNAL_TABLES_FIELD_NUMBER;
hash = (53 * hash) + getExternalTablesList().hashCode();
}
hash = (37 * hash) + USER_NAME_FIELD_NUMBER;
hash = (53 * hash) + getUserName().hashCode();
hash = (37 * hash) + PASSWORD_FIELD_NUMBER;
hash = (53 * hash) + getPassword().hashCode();
hash = (37 * hash) + QUOTA_FIELD_NUMBER;
hash = (53 * hash) + getQuota().hashCode();
hash = (37 * hash) + SESSION_ID_FIELD_NUMBER;
hash = (53 * hash) + getSessionId().hashCode();
hash = (37 * hash) + SESSION_CHECK_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getSessionCheck());
hash = (37 * hash) + SESSION_TIMEOUT_FIELD_NUMBER;
hash = (53 * hash) + getSessionTimeout();
hash = (37 * hash) + CANCEL_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getCancel());
hash = (37 * hash) + NEXT_QUERY_INFO_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getNextQueryInfo());
hash = (37 * hash) + INPUT_COMPRESSION_TYPE_FIELD_NUMBER;
hash = (53 * hash) + getInputCompressionType().hashCode();
hash = (37 * hash) + OUTPUT_COMPRESSION_TYPE_FIELD_NUMBER;
hash = (53 * hash) + getOutputCompressionType().hashCode();
hash = (37 * hash) + OUTPUT_COMPRESSION_LEVEL_FIELD_NUMBER;
hash = (53 * hash) + getOutputCompressionLevel();
hash = (37 * hash) + TRANSPORT_COMPRESSION_TYPE_FIELD_NUMBER;
hash = (53 * hash) + getTransportCompressionType().hashCode();
hash = (37 * hash) + TRANSPORT_COMPRESSION_LEVEL_FIELD_NUMBER;
hash = (53 * hash) + getTransportCompressionLevel();
if (hasObsoleteResultCompression()) {
hash = (37 * hash) + OBSOLETE_RESULT_COMPRESSION_FIELD_NUMBER;
hash = (53 * hash) + getObsoleteResultCompression().hashCode();
}
hash = (37 * hash) + OBSOLETE_COMPRESSION_TYPE_FIELD_NUMBER;
hash = (53 * hash) + getObsoleteCompressionType().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.clickhouse.client.grpc.impl.QueryInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.clickhouse.client.grpc.impl.QueryInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.clickhouse.client.grpc.impl.QueryInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.clickhouse.client.grpc.impl.QueryInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.clickhouse.client.grpc.impl.QueryInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.clickhouse.client.grpc.impl.QueryInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.clickhouse.client.grpc.impl.QueryInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.clickhouse.client.grpc.impl.QueryInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.clickhouse.client.grpc.impl.QueryInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.clickhouse.client.grpc.impl.QueryInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.clickhouse.client.grpc.impl.QueryInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.clickhouse.client.grpc.impl.QueryInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.clickhouse.client.grpc.impl.QueryInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Information about a query which a client sends to a ClickHouse server.
* The first QueryInfo can set any of the following fields. Extra QueryInfos only add extra data.
* In extra QueryInfos only `input_data`, `external_tables`, `next_query_info` and `cancel` fields can be set.
*
*
* Protobuf type {@code clickhouse.grpc.QueryInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:clickhouse.grpc.QueryInfo)
com.clickhouse.client.grpc.impl.QueryInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.clickhouse.client.grpc.impl.ClickHouseGrpcImpl.internal_static_clickhouse_grpc_QueryInfo_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 3:
return internalGetSettings();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMutableMapField(
int number) {
switch (number) {
case 3:
return internalGetMutableSettings();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.clickhouse.client.grpc.impl.ClickHouseGrpcImpl.internal_static_clickhouse_grpc_QueryInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.clickhouse.client.grpc.impl.QueryInfo.class, com.clickhouse.client.grpc.impl.QueryInfo.Builder.class);
}
// Construct using com.clickhouse.client.grpc.impl.QueryInfo.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
query_ = "";
queryId_ = "";
internalGetMutableSettings().clear();
database_ = "";
inputData_ = com.google.protobuf.ByteString.EMPTY;
inputDataDelimiter_ = com.google.protobuf.ByteString.EMPTY;
outputFormat_ = "";
sendOutputColumns_ = false;
if (externalTablesBuilder_ == null) {
externalTables_ = java.util.Collections.emptyList();
} else {
externalTables_ = null;
externalTablesBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000100);
userName_ = "";
password_ = "";
quota_ = "";
sessionId_ = "";
sessionCheck_ = false;
sessionTimeout_ = 0;
cancel_ = false;
nextQueryInfo_ = false;
inputCompressionType_ = "";
outputCompressionType_ = "";
outputCompressionLevel_ = 0;
transportCompressionType_ = "";
transportCompressionLevel_ = 0;
obsoleteResultCompression_ = null;
if (obsoleteResultCompressionBuilder_ != null) {
obsoleteResultCompressionBuilder_.dispose();
obsoleteResultCompressionBuilder_ = null;
}
obsoleteCompressionType_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.clickhouse.client.grpc.impl.ClickHouseGrpcImpl.internal_static_clickhouse_grpc_QueryInfo_descriptor;
}
@java.lang.Override
public com.clickhouse.client.grpc.impl.QueryInfo getDefaultInstanceForType() {
return com.clickhouse.client.grpc.impl.QueryInfo.getDefaultInstance();
}
@java.lang.Override
public com.clickhouse.client.grpc.impl.QueryInfo build() {
com.clickhouse.client.grpc.impl.QueryInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.clickhouse.client.grpc.impl.QueryInfo buildPartial() {
com.clickhouse.client.grpc.impl.QueryInfo result = new com.clickhouse.client.grpc.impl.QueryInfo(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.clickhouse.client.grpc.impl.QueryInfo result) {
if (externalTablesBuilder_ == null) {
if (((bitField0_ & 0x00000100) != 0)) {
externalTables_ = java.util.Collections.unmodifiableList(externalTables_);
bitField0_ = (bitField0_ & ~0x00000100);
}
result.externalTables_ = externalTables_;
} else {
result.externalTables_ = externalTablesBuilder_.build();
}
}
private void buildPartial0(com.clickhouse.client.grpc.impl.QueryInfo result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.query_ = query_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.queryId_ = queryId_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.settings_ = internalGetSettings();
result.settings_.makeImmutable();
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.database_ = database_;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.inputData_ = inputData_;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.inputDataDelimiter_ = inputDataDelimiter_;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.outputFormat_ = outputFormat_;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.sendOutputColumns_ = sendOutputColumns_;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
result.userName_ = userName_;
}
if (((from_bitField0_ & 0x00000400) != 0)) {
result.password_ = password_;
}
if (((from_bitField0_ & 0x00000800) != 0)) {
result.quota_ = quota_;
}
if (((from_bitField0_ & 0x00001000) != 0)) {
result.sessionId_ = sessionId_;
}
if (((from_bitField0_ & 0x00002000) != 0)) {
result.sessionCheck_ = sessionCheck_;
}
if (((from_bitField0_ & 0x00004000) != 0)) {
result.sessionTimeout_ = sessionTimeout_;
}
if (((from_bitField0_ & 0x00008000) != 0)) {
result.cancel_ = cancel_;
}
if (((from_bitField0_ & 0x00010000) != 0)) {
result.nextQueryInfo_ = nextQueryInfo_;
}
if (((from_bitField0_ & 0x00020000) != 0)) {
result.inputCompressionType_ = inputCompressionType_;
}
if (((from_bitField0_ & 0x00040000) != 0)) {
result.outputCompressionType_ = outputCompressionType_;
}
if (((from_bitField0_ & 0x00080000) != 0)) {
result.outputCompressionLevel_ = outputCompressionLevel_;
}
if (((from_bitField0_ & 0x00100000) != 0)) {
result.transportCompressionType_ = transportCompressionType_;
}
if (((from_bitField0_ & 0x00200000) != 0)) {
result.transportCompressionLevel_ = transportCompressionLevel_;
}
if (((from_bitField0_ & 0x00400000) != 0)) {
result.obsoleteResultCompression_ = obsoleteResultCompressionBuilder_ == null
? obsoleteResultCompression_
: obsoleteResultCompressionBuilder_.build();
}
if (((from_bitField0_ & 0x00800000) != 0)) {
result.obsoleteCompressionType_ = obsoleteCompressionType_;
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.clickhouse.client.grpc.impl.QueryInfo) {
return mergeFrom((com.clickhouse.client.grpc.impl.QueryInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.clickhouse.client.grpc.impl.QueryInfo other) {
if (other == com.clickhouse.client.grpc.impl.QueryInfo.getDefaultInstance()) return this;
if (!other.getQuery().isEmpty()) {
query_ = other.query_;
bitField0_ |= 0x00000001;
onChanged();
}
if (!other.getQueryId().isEmpty()) {
queryId_ = other.queryId_;
bitField0_ |= 0x00000002;
onChanged();
}
internalGetMutableSettings().mergeFrom(
other.internalGetSettings());
bitField0_ |= 0x00000004;
if (!other.getDatabase().isEmpty()) {
database_ = other.database_;
bitField0_ |= 0x00000008;
onChanged();
}
if (other.getInputData() != com.google.protobuf.ByteString.EMPTY) {
setInputData(other.getInputData());
}
if (other.getInputDataDelimiter() != com.google.protobuf.ByteString.EMPTY) {
setInputDataDelimiter(other.getInputDataDelimiter());
}
if (!other.getOutputFormat().isEmpty()) {
outputFormat_ = other.outputFormat_;
bitField0_ |= 0x00000040;
onChanged();
}
if (other.getSendOutputColumns() != false) {
setSendOutputColumns(other.getSendOutputColumns());
}
if (externalTablesBuilder_ == null) {
if (!other.externalTables_.isEmpty()) {
if (externalTables_.isEmpty()) {
externalTables_ = other.externalTables_;
bitField0_ = (bitField0_ & ~0x00000100);
} else {
ensureExternalTablesIsMutable();
externalTables_.addAll(other.externalTables_);
}
onChanged();
}
} else {
if (!other.externalTables_.isEmpty()) {
if (externalTablesBuilder_.isEmpty()) {
externalTablesBuilder_.dispose();
externalTablesBuilder_ = null;
externalTables_ = other.externalTables_;
bitField0_ = (bitField0_ & ~0x00000100);
externalTablesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getExternalTablesFieldBuilder() : null;
} else {
externalTablesBuilder_.addAllMessages(other.externalTables_);
}
}
}
if (!other.getUserName().isEmpty()) {
userName_ = other.userName_;
bitField0_ |= 0x00000200;
onChanged();
}
if (!other.getPassword().isEmpty()) {
password_ = other.password_;
bitField0_ |= 0x00000400;
onChanged();
}
if (!other.getQuota().isEmpty()) {
quota_ = other.quota_;
bitField0_ |= 0x00000800;
onChanged();
}
if (!other.getSessionId().isEmpty()) {
sessionId_ = other.sessionId_;
bitField0_ |= 0x00001000;
onChanged();
}
if (other.getSessionCheck() != false) {
setSessionCheck(other.getSessionCheck());
}
if (other.getSessionTimeout() != 0) {
setSessionTimeout(other.getSessionTimeout());
}
if (other.getCancel() != false) {
setCancel(other.getCancel());
}
if (other.getNextQueryInfo() != false) {
setNextQueryInfo(other.getNextQueryInfo());
}
if (!other.getInputCompressionType().isEmpty()) {
inputCompressionType_ = other.inputCompressionType_;
bitField0_ |= 0x00020000;
onChanged();
}
if (!other.getOutputCompressionType().isEmpty()) {
outputCompressionType_ = other.outputCompressionType_;
bitField0_ |= 0x00040000;
onChanged();
}
if (other.getOutputCompressionLevel() != 0) {
setOutputCompressionLevel(other.getOutputCompressionLevel());
}
if (!other.getTransportCompressionType().isEmpty()) {
transportCompressionType_ = other.transportCompressionType_;
bitField0_ |= 0x00100000;
onChanged();
}
if (other.getTransportCompressionLevel() != 0) {
setTransportCompressionLevel(other.getTransportCompressionLevel());
}
if (other.hasObsoleteResultCompression()) {
mergeObsoleteResultCompression(other.getObsoleteResultCompression());
}
if (!other.getObsoleteCompressionType().isEmpty()) {
obsoleteCompressionType_ = other.obsoleteCompressionType_;
bitField0_ |= 0x00800000;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
query_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
queryId_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 18
case 26: {
com.google.protobuf.MapEntry
settings__ = input.readMessage(
SettingsDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
internalGetMutableSettings().getMutableMap().put(
settings__.getKey(), settings__.getValue());
bitField0_ |= 0x00000004;
break;
} // case 26
case 34: {
database_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000008;
break;
} // case 34
case 42: {
inputData_ = input.readBytes();
bitField0_ |= 0x00000010;
break;
} // case 42
case 50: {
inputDataDelimiter_ = input.readBytes();
bitField0_ |= 0x00000020;
break;
} // case 50
case 58: {
outputFormat_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000040;
break;
} // case 58
case 66: {
com.clickhouse.client.grpc.impl.ExternalTable m =
input.readMessage(
com.clickhouse.client.grpc.impl.ExternalTable.parser(),
extensionRegistry);
if (externalTablesBuilder_ == null) {
ensureExternalTablesIsMutable();
externalTables_.add(m);
} else {
externalTablesBuilder_.addMessage(m);
}
break;
} // case 66
case 74: {
userName_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000200;
break;
} // case 74
case 82: {
password_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000400;
break;
} // case 82
case 90: {
quota_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000800;
break;
} // case 90
case 98: {
sessionId_ = input.readStringRequireUtf8();
bitField0_ |= 0x00001000;
break;
} // case 98
case 104: {
sessionCheck_ = input.readBool();
bitField0_ |= 0x00002000;
break;
} // case 104
case 112: {
sessionTimeout_ = input.readUInt32();
bitField0_ |= 0x00004000;
break;
} // case 112
case 120: {
cancel_ = input.readBool();
bitField0_ |= 0x00008000;
break;
} // case 120
case 128: {
nextQueryInfo_ = input.readBool();
bitField0_ |= 0x00010000;
break;
} // case 128
case 138: {
input.readMessage(
getObsoleteResultCompressionFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00400000;
break;
} // case 138
case 146: {
obsoleteCompressionType_ = input.readStringRequireUtf8();
bitField0_ |= 0x00800000;
break;
} // case 146
case 152: {
outputCompressionLevel_ = input.readInt32();
bitField0_ |= 0x00080000;
break;
} // case 152
case 162: {
inputCompressionType_ = input.readStringRequireUtf8();
bitField0_ |= 0x00020000;
break;
} // case 162
case 170: {
outputCompressionType_ = input.readStringRequireUtf8();
bitField0_ |= 0x00040000;
break;
} // case 170
case 178: {
transportCompressionType_ = input.readStringRequireUtf8();
bitField0_ |= 0x00100000;
break;
} // case 178
case 184: {
transportCompressionLevel_ = input.readInt32();
bitField0_ |= 0x00200000;
break;
} // case 184
case 192: {
sendOutputColumns_ = input.readBool();
bitField0_ |= 0x00000080;
break;
} // case 192
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object query_ = "";
/**
* string query = 1;
* @return The query.
*/
public java.lang.String getQuery() {
java.lang.Object ref = query_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
query_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string query = 1;
* @return The bytes for query.
*/
public com.google.protobuf.ByteString
getQueryBytes() {
java.lang.Object ref = query_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
query_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string query = 1;
* @param value The query to set.
* @return This builder for chaining.
*/
public Builder setQuery(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
query_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* string query = 1;
* @return This builder for chaining.
*/
public Builder clearQuery() {
query_ = getDefaultInstance().getQuery();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* string query = 1;
* @param value The bytes for query to set.
* @return This builder for chaining.
*/
public Builder setQueryBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
query_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object queryId_ = "";
/**
* string query_id = 2;
* @return The queryId.
*/
public java.lang.String getQueryId() {
java.lang.Object ref = queryId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
queryId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string query_id = 2;
* @return The bytes for queryId.
*/
public com.google.protobuf.ByteString
getQueryIdBytes() {
java.lang.Object ref = queryId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
queryId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string query_id = 2;
* @param value The queryId to set.
* @return This builder for chaining.
*/
public Builder setQueryId(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
queryId_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* string query_id = 2;
* @return This builder for chaining.
*/
public Builder clearQueryId() {
queryId_ = getDefaultInstance().getQueryId();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* string query_id = 2;
* @param value The bytes for queryId to set.
* @return This builder for chaining.
*/
public Builder setQueryIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
queryId_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> settings_;
private com.google.protobuf.MapField
internalGetSettings() {
if (settings_ == null) {
return com.google.protobuf.MapField.emptyMapField(
SettingsDefaultEntryHolder.defaultEntry);
}
return settings_;
}
private com.google.protobuf.MapField
internalGetMutableSettings() {
if (settings_ == null) {
settings_ = com.google.protobuf.MapField.newMapField(
SettingsDefaultEntryHolder.defaultEntry);
}
if (!settings_.isMutable()) {
settings_ = settings_.copy();
}
bitField0_ |= 0x00000004;
onChanged();
return settings_;
}
public int getSettingsCount() {
return internalGetSettings().getMap().size();
}
/**
* map<string, string> settings = 3;
*/
@java.lang.Override
public boolean containsSettings(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetSettings().getMap().containsKey(key);
}
/**
* Use {@link #getSettingsMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getSettings() {
return getSettingsMap();
}
/**
* map<string, string> settings = 3;
*/
@java.lang.Override
public java.util.Map getSettingsMap() {
return internalGetSettings().getMap();
}
/**
* map<string, string> settings = 3;
*/
@java.lang.Override
public /* nullable */
java.lang.String getSettingsOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetSettings().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<string, string> settings = 3;
*/
@java.lang.Override
public java.lang.String getSettingsOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetSettings().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearSettings() {
bitField0_ = (bitField0_ & ~0x00000004);
internalGetMutableSettings().getMutableMap()
.clear();
return this;
}
/**
* map<string, string> settings = 3;
*/
public Builder removeSettings(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
internalGetMutableSettings().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableSettings() {
bitField0_ |= 0x00000004;
return internalGetMutableSettings().getMutableMap();
}
/**
* map<string, string> settings = 3;
*/
public Builder putSettings(
java.lang.String key,
java.lang.String value) {
if (key == null) { throw new NullPointerException("map key"); }
if (value == null) { throw new NullPointerException("map value"); }
internalGetMutableSettings().getMutableMap()
.put(key, value);
bitField0_ |= 0x00000004;
return this;
}
/**
* map<string, string> settings = 3;
*/
public Builder putAllSettings(
java.util.Map values) {
internalGetMutableSettings().getMutableMap()
.putAll(values);
bitField0_ |= 0x00000004;
return this;
}
private java.lang.Object database_ = "";
/**
*
* Default database.
*
*
* string database = 4;
* @return The database.
*/
public java.lang.String getDatabase() {
java.lang.Object ref = database_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
database_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Default database.
*
*
* string database = 4;
* @return The bytes for database.
*/
public com.google.protobuf.ByteString
getDatabaseBytes() {
java.lang.Object ref = database_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
database_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Default database.
*
*
* string database = 4;
* @param value The database to set.
* @return This builder for chaining.
*/
public Builder setDatabase(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
database_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* Default database.
*
*
* string database = 4;
* @return This builder for chaining.
*/
public Builder clearDatabase() {
database_ = getDefaultInstance().getDatabase();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
return this;
}
/**
*
* Default database.
*
*
* string database = 4;
* @param value The bytes for database to set.
* @return This builder for chaining.
*/
public Builder setDatabaseBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
database_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
private com.google.protobuf.ByteString inputData_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Input data, used both as data for INSERT query and as data for the input() function.
*
*
* bytes input_data = 5;
* @return The inputData.
*/
@java.lang.Override
public com.google.protobuf.ByteString getInputData() {
return inputData_;
}
/**
*
* Input data, used both as data for INSERT query and as data for the input() function.
*
*
* bytes input_data = 5;
* @param value The inputData to set.
* @return This builder for chaining.
*/
public Builder setInputData(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
inputData_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* Input data, used both as data for INSERT query and as data for the input() function.
*
*
* bytes input_data = 5;
* @return This builder for chaining.
*/
public Builder clearInputData() {
bitField0_ = (bitField0_ & ~0x00000010);
inputData_ = getDefaultInstance().getInputData();
onChanged();
return this;
}
private com.google.protobuf.ByteString inputDataDelimiter_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Delimiter for input_data, inserted between input_data from adjacent QueryInfos.
*
*
* bytes input_data_delimiter = 6;
* @return The inputDataDelimiter.
*/
@java.lang.Override
public com.google.protobuf.ByteString getInputDataDelimiter() {
return inputDataDelimiter_;
}
/**
*
* Delimiter for input_data, inserted between input_data from adjacent QueryInfos.
*
*
* bytes input_data_delimiter = 6;
* @param value The inputDataDelimiter to set.
* @return This builder for chaining.
*/
public Builder setInputDataDelimiter(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
inputDataDelimiter_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
* Delimiter for input_data, inserted between input_data from adjacent QueryInfos.
*
*
* bytes input_data_delimiter = 6;
* @return This builder for chaining.
*/
public Builder clearInputDataDelimiter() {
bitField0_ = (bitField0_ & ~0x00000020);
inputDataDelimiter_ = getDefaultInstance().getInputDataDelimiter();
onChanged();
return this;
}
private java.lang.Object outputFormat_ = "";
/**
*
* Default output format. If not specified, 'TabSeparated' is used.
*
*
* string output_format = 7;
* @return The outputFormat.
*/
public java.lang.String getOutputFormat() {
java.lang.Object ref = outputFormat_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
outputFormat_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Default output format. If not specified, 'TabSeparated' is used.
*
*
* string output_format = 7;
* @return The bytes for outputFormat.
*/
public com.google.protobuf.ByteString
getOutputFormatBytes() {
java.lang.Object ref = outputFormat_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
outputFormat_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Default output format. If not specified, 'TabSeparated' is used.
*
*
* string output_format = 7;
* @param value The outputFormat to set.
* @return This builder for chaining.
*/
public Builder setOutputFormat(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
outputFormat_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
* Default output format. If not specified, 'TabSeparated' is used.
*
*
* string output_format = 7;
* @return This builder for chaining.
*/
public Builder clearOutputFormat() {
outputFormat_ = getDefaultInstance().getOutputFormat();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
return this;
}
/**
*
* Default output format. If not specified, 'TabSeparated' is used.
*
*
* string output_format = 7;
* @param value The bytes for outputFormat to set.
* @return This builder for chaining.
*/
public Builder setOutputFormatBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
outputFormat_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
private boolean sendOutputColumns_ ;
/**
*
* Set it if you want the names and the types of output columns to be sent to the client.
*
*
* bool send_output_columns = 24;
* @return The sendOutputColumns.
*/
@java.lang.Override
public boolean getSendOutputColumns() {
return sendOutputColumns_;
}
/**
*
* Set it if you want the names and the types of output columns to be sent to the client.
*
*
* bool send_output_columns = 24;
* @param value The sendOutputColumns to set.
* @return This builder for chaining.
*/
public Builder setSendOutputColumns(boolean value) {
sendOutputColumns_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
* Set it if you want the names and the types of output columns to be sent to the client.
*
*
* bool send_output_columns = 24;
* @return This builder for chaining.
*/
public Builder clearSendOutputColumns() {
bitField0_ = (bitField0_ & ~0x00000080);
sendOutputColumns_ = false;
onChanged();
return this;
}
private java.util.List externalTables_ =
java.util.Collections.emptyList();
private void ensureExternalTablesIsMutable() {
if (!((bitField0_ & 0x00000100) != 0)) {
externalTables_ = new java.util.ArrayList(externalTables_);
bitField0_ |= 0x00000100;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.clickhouse.client.grpc.impl.ExternalTable, com.clickhouse.client.grpc.impl.ExternalTable.Builder, com.clickhouse.client.grpc.impl.ExternalTableOrBuilder> externalTablesBuilder_;
/**
* repeated .clickhouse.grpc.ExternalTable external_tables = 8;
*/
public java.util.List getExternalTablesList() {
if (externalTablesBuilder_ == null) {
return java.util.Collections.unmodifiableList(externalTables_);
} else {
return externalTablesBuilder_.getMessageList();
}
}
/**
* repeated .clickhouse.grpc.ExternalTable external_tables = 8;
*/
public int getExternalTablesCount() {
if (externalTablesBuilder_ == null) {
return externalTables_.size();
} else {
return externalTablesBuilder_.getCount();
}
}
/**
* repeated .clickhouse.grpc.ExternalTable external_tables = 8;
*/
public com.clickhouse.client.grpc.impl.ExternalTable getExternalTables(int index) {
if (externalTablesBuilder_ == null) {
return externalTables_.get(index);
} else {
return externalTablesBuilder_.getMessage(index);
}
}
/**
* repeated .clickhouse.grpc.ExternalTable external_tables = 8;
*/
public Builder setExternalTables(
int index, com.clickhouse.client.grpc.impl.ExternalTable value) {
if (externalTablesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureExternalTablesIsMutable();
externalTables_.set(index, value);
onChanged();
} else {
externalTablesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .clickhouse.grpc.ExternalTable external_tables = 8;
*/
public Builder setExternalTables(
int index, com.clickhouse.client.grpc.impl.ExternalTable.Builder builderForValue) {
if (externalTablesBuilder_ == null) {
ensureExternalTablesIsMutable();
externalTables_.set(index, builderForValue.build());
onChanged();
} else {
externalTablesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .clickhouse.grpc.ExternalTable external_tables = 8;
*/
public Builder addExternalTables(com.clickhouse.client.grpc.impl.ExternalTable value) {
if (externalTablesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureExternalTablesIsMutable();
externalTables_.add(value);
onChanged();
} else {
externalTablesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .clickhouse.grpc.ExternalTable external_tables = 8;
*/
public Builder addExternalTables(
int index, com.clickhouse.client.grpc.impl.ExternalTable value) {
if (externalTablesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureExternalTablesIsMutable();
externalTables_.add(index, value);
onChanged();
} else {
externalTablesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .clickhouse.grpc.ExternalTable external_tables = 8;
*/
public Builder addExternalTables(
com.clickhouse.client.grpc.impl.ExternalTable.Builder builderForValue) {
if (externalTablesBuilder_ == null) {
ensureExternalTablesIsMutable();
externalTables_.add(builderForValue.build());
onChanged();
} else {
externalTablesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .clickhouse.grpc.ExternalTable external_tables = 8;
*/
public Builder addExternalTables(
int index, com.clickhouse.client.grpc.impl.ExternalTable.Builder builderForValue) {
if (externalTablesBuilder_ == null) {
ensureExternalTablesIsMutable();
externalTables_.add(index, builderForValue.build());
onChanged();
} else {
externalTablesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .clickhouse.grpc.ExternalTable external_tables = 8;
*/
public Builder addAllExternalTables(
java.lang.Iterable extends com.clickhouse.client.grpc.impl.ExternalTable> values) {
if (externalTablesBuilder_ == null) {
ensureExternalTablesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, externalTables_);
onChanged();
} else {
externalTablesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .clickhouse.grpc.ExternalTable external_tables = 8;
*/
public Builder clearExternalTables() {
if (externalTablesBuilder_ == null) {
externalTables_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000100);
onChanged();
} else {
externalTablesBuilder_.clear();
}
return this;
}
/**
* repeated .clickhouse.grpc.ExternalTable external_tables = 8;
*/
public Builder removeExternalTables(int index) {
if (externalTablesBuilder_ == null) {
ensureExternalTablesIsMutable();
externalTables_.remove(index);
onChanged();
} else {
externalTablesBuilder_.remove(index);
}
return this;
}
/**
* repeated .clickhouse.grpc.ExternalTable external_tables = 8;
*/
public com.clickhouse.client.grpc.impl.ExternalTable.Builder getExternalTablesBuilder(
int index) {
return getExternalTablesFieldBuilder().getBuilder(index);
}
/**
* repeated .clickhouse.grpc.ExternalTable external_tables = 8;
*/
public com.clickhouse.client.grpc.impl.ExternalTableOrBuilder getExternalTablesOrBuilder(
int index) {
if (externalTablesBuilder_ == null) {
return externalTables_.get(index); } else {
return externalTablesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .clickhouse.grpc.ExternalTable external_tables = 8;
*/
public java.util.List extends com.clickhouse.client.grpc.impl.ExternalTableOrBuilder>
getExternalTablesOrBuilderList() {
if (externalTablesBuilder_ != null) {
return externalTablesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(externalTables_);
}
}
/**
* repeated .clickhouse.grpc.ExternalTable external_tables = 8;
*/
public com.clickhouse.client.grpc.impl.ExternalTable.Builder addExternalTablesBuilder() {
return getExternalTablesFieldBuilder().addBuilder(
com.clickhouse.client.grpc.impl.ExternalTable.getDefaultInstance());
}
/**
* repeated .clickhouse.grpc.ExternalTable external_tables = 8;
*/
public com.clickhouse.client.grpc.impl.ExternalTable.Builder addExternalTablesBuilder(
int index) {
return getExternalTablesFieldBuilder().addBuilder(
index, com.clickhouse.client.grpc.impl.ExternalTable.getDefaultInstance());
}
/**
* repeated .clickhouse.grpc.ExternalTable external_tables = 8;
*/
public java.util.List
getExternalTablesBuilderList() {
return getExternalTablesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.clickhouse.client.grpc.impl.ExternalTable, com.clickhouse.client.grpc.impl.ExternalTable.Builder, com.clickhouse.client.grpc.impl.ExternalTableOrBuilder>
getExternalTablesFieldBuilder() {
if (externalTablesBuilder_ == null) {
externalTablesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.clickhouse.client.grpc.impl.ExternalTable, com.clickhouse.client.grpc.impl.ExternalTable.Builder, com.clickhouse.client.grpc.impl.ExternalTableOrBuilder>(
externalTables_,
((bitField0_ & 0x00000100) != 0),
getParentForChildren(),
isClean());
externalTables_ = null;
}
return externalTablesBuilder_;
}
private java.lang.Object userName_ = "";
/**
* string user_name = 9;
* @return The userName.
*/
public java.lang.String getUserName() {
java.lang.Object ref = userName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
userName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string user_name = 9;
* @return The bytes for userName.
*/
public com.google.protobuf.ByteString
getUserNameBytes() {
java.lang.Object ref = userName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
userName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string user_name = 9;
* @param value The userName to set.
* @return This builder for chaining.
*/
public Builder setUserName(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
userName_ = value;
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
* string user_name = 9;
* @return This builder for chaining.
*/
public Builder clearUserName() {
userName_ = getDefaultInstance().getUserName();
bitField0_ = (bitField0_ & ~0x00000200);
onChanged();
return this;
}
/**
* string user_name = 9;
* @param value The bytes for userName to set.
* @return This builder for chaining.
*/
public Builder setUserNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
userName_ = value;
bitField0_ |= 0x00000200;
onChanged();
return this;
}
private java.lang.Object password_ = "";
/**
* string password = 10;
* @return The password.
*/
public java.lang.String getPassword() {
java.lang.Object ref = password_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
password_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string password = 10;
* @return The bytes for password.
*/
public com.google.protobuf.ByteString
getPasswordBytes() {
java.lang.Object ref = password_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
password_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string password = 10;
* @param value The password to set.
* @return This builder for chaining.
*/
public Builder setPassword(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
password_ = value;
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
* string password = 10;
* @return This builder for chaining.
*/
public Builder clearPassword() {
password_ = getDefaultInstance().getPassword();
bitField0_ = (bitField0_ & ~0x00000400);
onChanged();
return this;
}
/**
* string password = 10;
* @param value The bytes for password to set.
* @return This builder for chaining.
*/
public Builder setPasswordBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
password_ = value;
bitField0_ |= 0x00000400;
onChanged();
return this;
}
private java.lang.Object quota_ = "";
/**
* string quota = 11;
* @return The quota.
*/
public java.lang.String getQuota() {
java.lang.Object ref = quota_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
quota_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string quota = 11;
* @return The bytes for quota.
*/
public com.google.protobuf.ByteString
getQuotaBytes() {
java.lang.Object ref = quota_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
quota_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string quota = 11;
* @param value The quota to set.
* @return This builder for chaining.
*/
public Builder setQuota(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
quota_ = value;
bitField0_ |= 0x00000800;
onChanged();
return this;
}
/**
* string quota = 11;
* @return This builder for chaining.
*/
public Builder clearQuota() {
quota_ = getDefaultInstance().getQuota();
bitField0_ = (bitField0_ & ~0x00000800);
onChanged();
return this;
}
/**
* string quota = 11;
* @param value The bytes for quota to set.
* @return This builder for chaining.
*/
public Builder setQuotaBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
quota_ = value;
bitField0_ |= 0x00000800;
onChanged();
return this;
}
private java.lang.Object sessionId_ = "";
/**
*
* Works exactly like sessions in the HTTP protocol.
*
*
* string session_id = 12;
* @return The sessionId.
*/
public java.lang.String getSessionId() {
java.lang.Object ref = sessionId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sessionId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Works exactly like sessions in the HTTP protocol.
*
*
* string session_id = 12;
* @return The bytes for sessionId.
*/
public com.google.protobuf.ByteString
getSessionIdBytes() {
java.lang.Object ref = sessionId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sessionId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Works exactly like sessions in the HTTP protocol.
*
*
* string session_id = 12;
* @param value The sessionId to set.
* @return This builder for chaining.
*/
public Builder setSessionId(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
sessionId_ = value;
bitField0_ |= 0x00001000;
onChanged();
return this;
}
/**
*
* Works exactly like sessions in the HTTP protocol.
*
*
* string session_id = 12;
* @return This builder for chaining.
*/
public Builder clearSessionId() {
sessionId_ = getDefaultInstance().getSessionId();
bitField0_ = (bitField0_ & ~0x00001000);
onChanged();
return this;
}
/**
*
* Works exactly like sessions in the HTTP protocol.
*
*
* string session_id = 12;
* @param value The bytes for sessionId to set.
* @return This builder for chaining.
*/
public Builder setSessionIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
sessionId_ = value;
bitField0_ |= 0x00001000;
onChanged();
return this;
}
private boolean sessionCheck_ ;
/**
* bool session_check = 13;
* @return The sessionCheck.
*/
@java.lang.Override
public boolean getSessionCheck() {
return sessionCheck_;
}
/**
* bool session_check = 13;
* @param value The sessionCheck to set.
* @return This builder for chaining.
*/
public Builder setSessionCheck(boolean value) {
sessionCheck_ = value;
bitField0_ |= 0x00002000;
onChanged();
return this;
}
/**
* bool session_check = 13;
* @return This builder for chaining.
*/
public Builder clearSessionCheck() {
bitField0_ = (bitField0_ & ~0x00002000);
sessionCheck_ = false;
onChanged();
return this;
}
private int sessionTimeout_ ;
/**
* uint32 session_timeout = 14;
* @return The sessionTimeout.
*/
@java.lang.Override
public int getSessionTimeout() {
return sessionTimeout_;
}
/**
* uint32 session_timeout = 14;
* @param value The sessionTimeout to set.
* @return This builder for chaining.
*/
public Builder setSessionTimeout(int value) {
sessionTimeout_ = value;
bitField0_ |= 0x00004000;
onChanged();
return this;
}
/**
* uint32 session_timeout = 14;
* @return This builder for chaining.
*/
public Builder clearSessionTimeout() {
bitField0_ = (bitField0_ & ~0x00004000);
sessionTimeout_ = 0;
onChanged();
return this;
}
private boolean cancel_ ;
/**
*
* Set `cancel` to true to stop executing the query.
*
*
* bool cancel = 15;
* @return The cancel.
*/
@java.lang.Override
public boolean getCancel() {
return cancel_;
}
/**
*
* Set `cancel` to true to stop executing the query.
*
*
* bool cancel = 15;
* @param value The cancel to set.
* @return This builder for chaining.
*/
public Builder setCancel(boolean value) {
cancel_ = value;
bitField0_ |= 0x00008000;
onChanged();
return this;
}
/**
*
* Set `cancel` to true to stop executing the query.
*
*
* bool cancel = 15;
* @return This builder for chaining.
*/
public Builder clearCancel() {
bitField0_ = (bitField0_ & ~0x00008000);
cancel_ = false;
onChanged();
return this;
}
private boolean nextQueryInfo_ ;
/**
*
* If true there will be at least one more QueryInfo in the input stream.
* `next_query_info` is allowed to be set only if a method with streaming input (i.e. ExecuteQueryWithStreamInput() or ExecuteQueryWithStreamIO()) is used.
*
*
* bool next_query_info = 16;
* @return The nextQueryInfo.
*/
@java.lang.Override
public boolean getNextQueryInfo() {
return nextQueryInfo_;
}
/**
*
* If true there will be at least one more QueryInfo in the input stream.
* `next_query_info` is allowed to be set only if a method with streaming input (i.e. ExecuteQueryWithStreamInput() or ExecuteQueryWithStreamIO()) is used.
*
*
* bool next_query_info = 16;
* @param value The nextQueryInfo to set.
* @return This builder for chaining.
*/
public Builder setNextQueryInfo(boolean value) {
nextQueryInfo_ = value;
bitField0_ |= 0x00010000;
onChanged();
return this;
}
/**
*
* If true there will be at least one more QueryInfo in the input stream.
* `next_query_info` is allowed to be set only if a method with streaming input (i.e. ExecuteQueryWithStreamInput() or ExecuteQueryWithStreamIO()) is used.
*
*
* bool next_query_info = 16;
* @return This builder for chaining.
*/
public Builder clearNextQueryInfo() {
bitField0_ = (bitField0_ & ~0x00010000);
nextQueryInfo_ = false;
onChanged();
return this;
}
private java.lang.Object inputCompressionType_ = "";
/**
*
* Compression type for `input_data`.
* Supported compression types: none, gzip(gz), deflate, brotli(br), lzma(xz), zstd(zst), lz4, bz2.
* The client is responsible to compress data before putting it into `input_data`.
*
*
* string input_compression_type = 20;
* @return The inputCompressionType.
*/
public java.lang.String getInputCompressionType() {
java.lang.Object ref = inputCompressionType_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
inputCompressionType_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Compression type for `input_data`.
* Supported compression types: none, gzip(gz), deflate, brotli(br), lzma(xz), zstd(zst), lz4, bz2.
* The client is responsible to compress data before putting it into `input_data`.
*
*
* string input_compression_type = 20;
* @return The bytes for inputCompressionType.
*/
public com.google.protobuf.ByteString
getInputCompressionTypeBytes() {
java.lang.Object ref = inputCompressionType_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
inputCompressionType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Compression type for `input_data`.
* Supported compression types: none, gzip(gz), deflate, brotli(br), lzma(xz), zstd(zst), lz4, bz2.
* The client is responsible to compress data before putting it into `input_data`.
*
*
* string input_compression_type = 20;
* @param value The inputCompressionType to set.
* @return This builder for chaining.
*/
public Builder setInputCompressionType(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
inputCompressionType_ = value;
bitField0_ |= 0x00020000;
onChanged();
return this;
}
/**
*
* Compression type for `input_data`.
* Supported compression types: none, gzip(gz), deflate, brotli(br), lzma(xz), zstd(zst), lz4, bz2.
* The client is responsible to compress data before putting it into `input_data`.
*
*
* string input_compression_type = 20;
* @return This builder for chaining.
*/
public Builder clearInputCompressionType() {
inputCompressionType_ = getDefaultInstance().getInputCompressionType();
bitField0_ = (bitField0_ & ~0x00020000);
onChanged();
return this;
}
/**
*
* Compression type for `input_data`.
* Supported compression types: none, gzip(gz), deflate, brotli(br), lzma(xz), zstd(zst), lz4, bz2.
* The client is responsible to compress data before putting it into `input_data`.
*
*
* string input_compression_type = 20;
* @param value The bytes for inputCompressionType to set.
* @return This builder for chaining.
*/
public Builder setInputCompressionTypeBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
inputCompressionType_ = value;
bitField0_ |= 0x00020000;
onChanged();
return this;
}
private java.lang.Object outputCompressionType_ = "";
/**
*
* Compression type for `output_data`, `totals` and `extremes`.
* Supported compression types: none, gzip(gz), deflate, brotli(br), lzma(xz), zstd(zst), lz4, bz2.
* The client receives compressed data and should decompress it by itself.
* Consider also setting `output_compression_level`.
*
*
* string output_compression_type = 21;
* @return The outputCompressionType.
*/
public java.lang.String getOutputCompressionType() {
java.lang.Object ref = outputCompressionType_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
outputCompressionType_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Compression type for `output_data`, `totals` and `extremes`.
* Supported compression types: none, gzip(gz), deflate, brotli(br), lzma(xz), zstd(zst), lz4, bz2.
* The client receives compressed data and should decompress it by itself.
* Consider also setting `output_compression_level`.
*
*
* string output_compression_type = 21;
* @return The bytes for outputCompressionType.
*/
public com.google.protobuf.ByteString
getOutputCompressionTypeBytes() {
java.lang.Object ref = outputCompressionType_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
outputCompressionType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Compression type for `output_data`, `totals` and `extremes`.
* Supported compression types: none, gzip(gz), deflate, brotli(br), lzma(xz), zstd(zst), lz4, bz2.
* The client receives compressed data and should decompress it by itself.
* Consider also setting `output_compression_level`.
*
*
* string output_compression_type = 21;
* @param value The outputCompressionType to set.
* @return This builder for chaining.
*/
public Builder setOutputCompressionType(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
outputCompressionType_ = value;
bitField0_ |= 0x00040000;
onChanged();
return this;
}
/**
*
* Compression type for `output_data`, `totals` and `extremes`.
* Supported compression types: none, gzip(gz), deflate, brotli(br), lzma(xz), zstd(zst), lz4, bz2.
* The client receives compressed data and should decompress it by itself.
* Consider also setting `output_compression_level`.
*
*
* string output_compression_type = 21;
* @return This builder for chaining.
*/
public Builder clearOutputCompressionType() {
outputCompressionType_ = getDefaultInstance().getOutputCompressionType();
bitField0_ = (bitField0_ & ~0x00040000);
onChanged();
return this;
}
/**
*
* Compression type for `output_data`, `totals` and `extremes`.
* Supported compression types: none, gzip(gz), deflate, brotli(br), lzma(xz), zstd(zst), lz4, bz2.
* The client receives compressed data and should decompress it by itself.
* Consider also setting `output_compression_level`.
*
*
* string output_compression_type = 21;
* @param value The bytes for outputCompressionType to set.
* @return This builder for chaining.
*/
public Builder setOutputCompressionTypeBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
outputCompressionType_ = value;
bitField0_ |= 0x00040000;
onChanged();
return this;
}
private int outputCompressionLevel_ ;
/**
*
* Compression level.
* WARNING: If it's not specified the compression level is set to zero by default which might be not the best choice for some compression types (see below).
* The compression level should be in the following range (the higher the number, the better the compression):
* none: compression level isn't used
* gzip: 0..9; 0 means no compression, 6 is recommended by default (compression level -1 also means 6)
* brotli: 0..11
* lzma: 0..9; 6 is recommended by default
* zstd: 1..22; 3 is recommended by default (compression level 0 also means 3)
* lz4: 0..16; values < 0 mean fast acceleration
* bz2: 1..9
*
*
* int32 output_compression_level = 19;
* @return The outputCompressionLevel.
*/
@java.lang.Override
public int getOutputCompressionLevel() {
return outputCompressionLevel_;
}
/**
*
* Compression level.
* WARNING: If it's not specified the compression level is set to zero by default which might be not the best choice for some compression types (see below).
* The compression level should be in the following range (the higher the number, the better the compression):
* none: compression level isn't used
* gzip: 0..9; 0 means no compression, 6 is recommended by default (compression level -1 also means 6)
* brotli: 0..11
* lzma: 0..9; 6 is recommended by default
* zstd: 1..22; 3 is recommended by default (compression level 0 also means 3)
* lz4: 0..16; values < 0 mean fast acceleration
* bz2: 1..9
*
*
* int32 output_compression_level = 19;
* @param value The outputCompressionLevel to set.
* @return This builder for chaining.
*/
public Builder setOutputCompressionLevel(int value) {
outputCompressionLevel_ = value;
bitField0_ |= 0x00080000;
onChanged();
return this;
}
/**
*
* Compression level.
* WARNING: If it's not specified the compression level is set to zero by default which might be not the best choice for some compression types (see below).
* The compression level should be in the following range (the higher the number, the better the compression):
* none: compression level isn't used
* gzip: 0..9; 0 means no compression, 6 is recommended by default (compression level -1 also means 6)
* brotli: 0..11
* lzma: 0..9; 6 is recommended by default
* zstd: 1..22; 3 is recommended by default (compression level 0 also means 3)
* lz4: 0..16; values < 0 mean fast acceleration
* bz2: 1..9
*
*
* int32 output_compression_level = 19;
* @return This builder for chaining.
*/
public Builder clearOutputCompressionLevel() {
bitField0_ = (bitField0_ & ~0x00080000);
outputCompressionLevel_ = 0;
onChanged();
return this;
}
private java.lang.Object transportCompressionType_ = "";
/**
*
* Transport compression is an alternative way to make the server to compress its response.
* This kind of compression implies that instead of compressing just `output` the server will compress whole packed messages of the `Result` type,
* and then gRPC implementation on client side will decompress those messages so client code won't be bothered with decompression.
* Here is a big difference between the transport compression and the compression enabled by setting `output_compression_type` because
* in case of the transport compression the client code receives already decompressed data in `output`.
* If the transport compression is not set here it can still be enabled by the server configuration.
* Supported compression types: none, deflate, gzip, stream_gzip
* Supported compression levels: 0..3
* WARNING: Don't set `transport_compression` and `output_compression` at the same time because it will make the server to compress its output twice!
*
*
* string transport_compression_type = 22;
* @return The transportCompressionType.
*/
public java.lang.String getTransportCompressionType() {
java.lang.Object ref = transportCompressionType_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
transportCompressionType_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Transport compression is an alternative way to make the server to compress its response.
* This kind of compression implies that instead of compressing just `output` the server will compress whole packed messages of the `Result` type,
* and then gRPC implementation on client side will decompress those messages so client code won't be bothered with decompression.
* Here is a big difference between the transport compression and the compression enabled by setting `output_compression_type` because
* in case of the transport compression the client code receives already decompressed data in `output`.
* If the transport compression is not set here it can still be enabled by the server configuration.
* Supported compression types: none, deflate, gzip, stream_gzip
* Supported compression levels: 0..3
* WARNING: Don't set `transport_compression` and `output_compression` at the same time because it will make the server to compress its output twice!
*
*
* string transport_compression_type = 22;
* @return The bytes for transportCompressionType.
*/
public com.google.protobuf.ByteString
getTransportCompressionTypeBytes() {
java.lang.Object ref = transportCompressionType_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
transportCompressionType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Transport compression is an alternative way to make the server to compress its response.
* This kind of compression implies that instead of compressing just `output` the server will compress whole packed messages of the `Result` type,
* and then gRPC implementation on client side will decompress those messages so client code won't be bothered with decompression.
* Here is a big difference between the transport compression and the compression enabled by setting `output_compression_type` because
* in case of the transport compression the client code receives already decompressed data in `output`.
* If the transport compression is not set here it can still be enabled by the server configuration.
* Supported compression types: none, deflate, gzip, stream_gzip
* Supported compression levels: 0..3
* WARNING: Don't set `transport_compression` and `output_compression` at the same time because it will make the server to compress its output twice!
*
*
* string transport_compression_type = 22;
* @param value The transportCompressionType to set.
* @return This builder for chaining.
*/
public Builder setTransportCompressionType(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
transportCompressionType_ = value;
bitField0_ |= 0x00100000;
onChanged();
return this;
}
/**
*
* Transport compression is an alternative way to make the server to compress its response.
* This kind of compression implies that instead of compressing just `output` the server will compress whole packed messages of the `Result` type,
* and then gRPC implementation on client side will decompress those messages so client code won't be bothered with decompression.
* Here is a big difference between the transport compression and the compression enabled by setting `output_compression_type` because
* in case of the transport compression the client code receives already decompressed data in `output`.
* If the transport compression is not set here it can still be enabled by the server configuration.
* Supported compression types: none, deflate, gzip, stream_gzip
* Supported compression levels: 0..3
* WARNING: Don't set `transport_compression` and `output_compression` at the same time because it will make the server to compress its output twice!
*
*
* string transport_compression_type = 22;
* @return This builder for chaining.
*/
public Builder clearTransportCompressionType() {
transportCompressionType_ = getDefaultInstance().getTransportCompressionType();
bitField0_ = (bitField0_ & ~0x00100000);
onChanged();
return this;
}
/**
*
* Transport compression is an alternative way to make the server to compress its response.
* This kind of compression implies that instead of compressing just `output` the server will compress whole packed messages of the `Result` type,
* and then gRPC implementation on client side will decompress those messages so client code won't be bothered with decompression.
* Here is a big difference between the transport compression and the compression enabled by setting `output_compression_type` because
* in case of the transport compression the client code receives already decompressed data in `output`.
* If the transport compression is not set here it can still be enabled by the server configuration.
* Supported compression types: none, deflate, gzip, stream_gzip
* Supported compression levels: 0..3
* WARNING: Don't set `transport_compression` and `output_compression` at the same time because it will make the server to compress its output twice!
*
*
* string transport_compression_type = 22;
* @param value The bytes for transportCompressionType to set.
* @return This builder for chaining.
*/
public Builder setTransportCompressionTypeBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
transportCompressionType_ = value;
bitField0_ |= 0x00100000;
onChanged();
return this;
}
private int transportCompressionLevel_ ;
/**
* int32 transport_compression_level = 23;
* @return The transportCompressionLevel.
*/
@java.lang.Override
public int getTransportCompressionLevel() {
return transportCompressionLevel_;
}
/**
* int32 transport_compression_level = 23;
* @param value The transportCompressionLevel to set.
* @return This builder for chaining.
*/
public Builder setTransportCompressionLevel(int value) {
transportCompressionLevel_ = value;
bitField0_ |= 0x00200000;
onChanged();
return this;
}
/**
* int32 transport_compression_level = 23;
* @return This builder for chaining.
*/
public Builder clearTransportCompressionLevel() {
bitField0_ = (bitField0_ & ~0x00200000);
transportCompressionLevel_ = 0;
onChanged();
return this;
}
private com.clickhouse.client.grpc.impl.ObsoleteTransportCompression obsoleteResultCompression_;
private com.google.protobuf.SingleFieldBuilderV3<
com.clickhouse.client.grpc.impl.ObsoleteTransportCompression, com.clickhouse.client.grpc.impl.ObsoleteTransportCompression.Builder, com.clickhouse.client.grpc.impl.ObsoleteTransportCompressionOrBuilder> obsoleteResultCompressionBuilder_;
/**
*
*/ Obsolete fields, should not be used in new code.
*
*
* .clickhouse.grpc.ObsoleteTransportCompression obsolete_result_compression = 17;
* @return Whether the obsoleteResultCompression field is set.
*/
public boolean hasObsoleteResultCompression() {
return ((bitField0_ & 0x00400000) != 0);
}
/**
*
*/ Obsolete fields, should not be used in new code.
*
*
* .clickhouse.grpc.ObsoleteTransportCompression obsolete_result_compression = 17;
* @return The obsoleteResultCompression.
*/
public com.clickhouse.client.grpc.impl.ObsoleteTransportCompression getObsoleteResultCompression() {
if (obsoleteResultCompressionBuilder_ == null) {
return obsoleteResultCompression_ == null ? com.clickhouse.client.grpc.impl.ObsoleteTransportCompression.getDefaultInstance() : obsoleteResultCompression_;
} else {
return obsoleteResultCompressionBuilder_.getMessage();
}
}
/**
*
*/ Obsolete fields, should not be used in new code.
*
*
* .clickhouse.grpc.ObsoleteTransportCompression obsolete_result_compression = 17;
*/
public Builder setObsoleteResultCompression(com.clickhouse.client.grpc.impl.ObsoleteTransportCompression value) {
if (obsoleteResultCompressionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
obsoleteResultCompression_ = value;
} else {
obsoleteResultCompressionBuilder_.setMessage(value);
}
bitField0_ |= 0x00400000;
onChanged();
return this;
}
/**
*
*/ Obsolete fields, should not be used in new code.
*
*
* .clickhouse.grpc.ObsoleteTransportCompression obsolete_result_compression = 17;
*/
public Builder setObsoleteResultCompression(
com.clickhouse.client.grpc.impl.ObsoleteTransportCompression.Builder builderForValue) {
if (obsoleteResultCompressionBuilder_ == null) {
obsoleteResultCompression_ = builderForValue.build();
} else {
obsoleteResultCompressionBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00400000;
onChanged();
return this;
}
/**
*
*/ Obsolete fields, should not be used in new code.
*
*
* .clickhouse.grpc.ObsoleteTransportCompression obsolete_result_compression = 17;
*/
public Builder mergeObsoleteResultCompression(com.clickhouse.client.grpc.impl.ObsoleteTransportCompression value) {
if (obsoleteResultCompressionBuilder_ == null) {
if (((bitField0_ & 0x00400000) != 0) &&
obsoleteResultCompression_ != null &&
obsoleteResultCompression_ != com.clickhouse.client.grpc.impl.ObsoleteTransportCompression.getDefaultInstance()) {
getObsoleteResultCompressionBuilder().mergeFrom(value);
} else {
obsoleteResultCompression_ = value;
}
} else {
obsoleteResultCompressionBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00400000;
onChanged();
return this;
}
/**
*
*/ Obsolete fields, should not be used in new code.
*
*
* .clickhouse.grpc.ObsoleteTransportCompression obsolete_result_compression = 17;
*/
public Builder clearObsoleteResultCompression() {
bitField0_ = (bitField0_ & ~0x00400000);
obsoleteResultCompression_ = null;
if (obsoleteResultCompressionBuilder_ != null) {
obsoleteResultCompressionBuilder_.dispose();
obsoleteResultCompressionBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*/ Obsolete fields, should not be used in new code.
*
*
* .clickhouse.grpc.ObsoleteTransportCompression obsolete_result_compression = 17;
*/
public com.clickhouse.client.grpc.impl.ObsoleteTransportCompression.Builder getObsoleteResultCompressionBuilder() {
bitField0_ |= 0x00400000;
onChanged();
return getObsoleteResultCompressionFieldBuilder().getBuilder();
}
/**
*
*/ Obsolete fields, should not be used in new code.
*
*
* .clickhouse.grpc.ObsoleteTransportCompression obsolete_result_compression = 17;
*/
public com.clickhouse.client.grpc.impl.ObsoleteTransportCompressionOrBuilder getObsoleteResultCompressionOrBuilder() {
if (obsoleteResultCompressionBuilder_ != null) {
return obsoleteResultCompressionBuilder_.getMessageOrBuilder();
} else {
return obsoleteResultCompression_ == null ?
com.clickhouse.client.grpc.impl.ObsoleteTransportCompression.getDefaultInstance() : obsoleteResultCompression_;
}
}
/**
*
*/ Obsolete fields, should not be used in new code.
*
*
* .clickhouse.grpc.ObsoleteTransportCompression obsolete_result_compression = 17;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.clickhouse.client.grpc.impl.ObsoleteTransportCompression, com.clickhouse.client.grpc.impl.ObsoleteTransportCompression.Builder, com.clickhouse.client.grpc.impl.ObsoleteTransportCompressionOrBuilder>
getObsoleteResultCompressionFieldBuilder() {
if (obsoleteResultCompressionBuilder_ == null) {
obsoleteResultCompressionBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.clickhouse.client.grpc.impl.ObsoleteTransportCompression, com.clickhouse.client.grpc.impl.ObsoleteTransportCompression.Builder, com.clickhouse.client.grpc.impl.ObsoleteTransportCompressionOrBuilder>(
getObsoleteResultCompression(),
getParentForChildren(),
isClean());
obsoleteResultCompression_ = null;
}
return obsoleteResultCompressionBuilder_;
}
private java.lang.Object obsoleteCompressionType_ = "";
/**
* string obsolete_compression_type = 18;
* @return The obsoleteCompressionType.
*/
public java.lang.String getObsoleteCompressionType() {
java.lang.Object ref = obsoleteCompressionType_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
obsoleteCompressionType_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string obsolete_compression_type = 18;
* @return The bytes for obsoleteCompressionType.
*/
public com.google.protobuf.ByteString
getObsoleteCompressionTypeBytes() {
java.lang.Object ref = obsoleteCompressionType_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
obsoleteCompressionType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string obsolete_compression_type = 18;
* @param value The obsoleteCompressionType to set.
* @return This builder for chaining.
*/
public Builder setObsoleteCompressionType(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
obsoleteCompressionType_ = value;
bitField0_ |= 0x00800000;
onChanged();
return this;
}
/**
* string obsolete_compression_type = 18;
* @return This builder for chaining.
*/
public Builder clearObsoleteCompressionType() {
obsoleteCompressionType_ = getDefaultInstance().getObsoleteCompressionType();
bitField0_ = (bitField0_ & ~0x00800000);
onChanged();
return this;
}
/**
* string obsolete_compression_type = 18;
* @param value The bytes for obsoleteCompressionType to set.
* @return This builder for chaining.
*/
public Builder setObsoleteCompressionTypeBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
obsoleteCompressionType_ = value;
bitField0_ |= 0x00800000;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:clickhouse.grpc.QueryInfo)
}
// @@protoc_insertion_point(class_scope:clickhouse.grpc.QueryInfo)
private static final com.clickhouse.client.grpc.impl.QueryInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.clickhouse.client.grpc.impl.QueryInfo();
}
public static com.clickhouse.client.grpc.impl.QueryInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public QueryInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.clickhouse.client.grpc.impl.QueryInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy