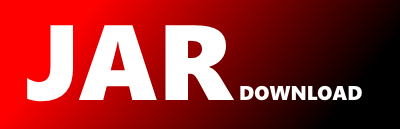
com.clickntap.square.ux.UX Maven / Gradle / Ivy
package com.clickntap.square.ux;
import java.io.File;
import java.nio.charset.StandardCharsets;
import java.util.HashMap;
import java.util.Map;
import org.apache.commons.io.FileUtils;
import org.apache.commons.io.monitor.FileAlterationListener;
import org.apache.commons.io.monitor.FileAlterationMonitor;
import org.apache.commons.io.monitor.FileAlterationObserver;
import org.json.JSONObject;
import com.clickntap.square.App;
import com.clickntap.square.Runner;
import com.clickntap.square.utils.CryptoUtils;
import com.clickntap.square.utils.StringUtils;
public class UX implements FileAlterationListener {
private File dir;
private FileAlterationMonitor monitor;
private UXJavascriptWorker javascriptWorker;
private UXLessWorker lessWorker;
private UXHTMLWorker htmlWorker;
private boolean skip;
public UX() {
javascriptWorker = new UXJavascriptWorker();
lessWorker = new UXLessWorker();
htmlWorker = new UXHTMLWorker();
skip = false;
}
public File getDir() {
return dir;
}
public void setDir(File dir) {
this.dir = dir;
}
public String src(String path) throws Exception {
StringBuffer sb = new StringBuffer("/ux/");
sb.append(path);
sb.append('?');
sb.append(CryptoUtils.md5(getFile(path)));
return sb.toString();
}
public String lib(String name) throws Exception {
return StringUtils.getStringFromResource(new StringBuffer("com/clickntap/square/javascript/").append(name).toString());
}
public File getFile(String path) {
StringBuffer sb = new StringBuffer(dir.getAbsolutePath());
sb.append('/');
sb.append(path);
return new File(sb.toString());
}
public String include(String path) throws Exception {
String value = FileUtils.readFileToString(getFile(path), StandardCharsets.UTF_8);
return value;
}
public String include(String path, String model) throws Exception {
String value = FileUtils.readFileToString(getFile(path), StandardCharsets.UTF_8);
return App.getInstance().parse(value, new JSONObject(model));
}
public void onFileChange(File file) {
String absolutePath = file.getAbsolutePath();
if (absolutePath.contains("/ux/js/"))
return;
if (absolutePath.contains("/ux/css/"))
return;
if (skip) {
skip = false;
return;
}
long lo = System.currentTimeMillis();
if (file.getName().endsWith(".html")) {
htmlWorker.build(this);
} else {
try {
if (file.getName().endsWith(".js") || file.getName().endsWith(".less") || file.getName().endsWith(".css")) {
String code = FileUtils.readFileToString(file, StandardCharsets.UTF_8);
code = StringUtils.format(code);
FileUtils.writeStringToFile(file, code, StandardCharsets.UTF_8);
skip = true;
}
} catch (Exception e) {
}
}
for (File sourceFile : dir.listFiles()) {
if (sourceFile.getName().endsWith(".js")) {
compile(sourceFile, javascriptWorker);
}
if (sourceFile.getName().endsWith(".less")) {
compile(sourceFile, lessWorker);
}
}
System.out.println(new StringBuffer(file.getName()).append(' ').append(System.currentTimeMillis() - lo).append(" millis"));
}
public String jsEval(String code) throws Exception {
return javascriptWorker.eval(code);
}
public String lessEval(String code) throws Exception {
return lessWorker.eval(code);
}
private void compile(File sourceFile, UXWorker compiler) {
String code = null;
try {
JSONObject conf = App.getInstance().getConf();
code = FileUtils.readFileToString(sourceFile, StandardCharsets.UTF_8);
Map beans = new HashMap();
beans.put("ux", this);
JSONObject model = new JSONObject();
Runner.settings(conf, model);
code = App.getInstance().parse(code, model, beans);
code = compiler.eval(code);
String name = sourceFile.getName();
name = name.replace(compiler.getInputExtension(), compiler.getOutputExtension());
String destinationPath = new StringBuffer(compiler.getOutputExtension()).append("/").append(name).toString();
File destinationFile = getFile(destinationPath);
destinationFile.getParentFile().mkdirs();
FileUtils.writeStringToFile(destinationFile, code, StandardCharsets.UTF_8);
} catch (Exception e) {
e.printStackTrace();
}
}
public void init() throws Exception {
FileAlterationObserver observer = new FileAlterationObserver(getDir());
observer.addListener(this);
monitor = new FileAlterationMonitor(500);
monitor.addObserver(observer);
monitor.start();
}
public void onDirectoryChange(File directory) {
onFileChange(directory);
}
public void onDirectoryCreate(File directory) {
onFileChange(directory);
}
public void onDirectoryDelete(File directory) {
onFileChange(directory);
}
public void onFileCreate(File file) {
onFileChange(file);
}
public void onFileDelete(File file) {
onFileChange(file);
}
public void onStart(FileAlterationObserver observer) {
}
public void onStop(FileAlterationObserver observer) {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy