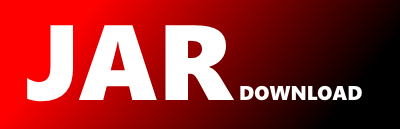
com.clickzetta.client.BulkloadStreamBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickzetta-java Show documentation
Show all versions of clickzetta-java Show documentation
The java SDK for clickzetta's Lakehouse
package com.clickzetta.client;
import com.clickzetta.platform.client.api.BulkLoadOperation;
import com.clickzetta.platform.client.api.BulkLoadOptions;
import java.io.IOException;
import java.util.List;
public class BulkloadStreamBuilder {
private ClickZettaClient client;
private String streamId;
private String schema;
private String table;
private RowStream.BulkLoadOperate operate = RowStream.BulkLoadOperate.APPEND;
private List recordKeys;
BulkloadStreamBuilder(ClickZettaClient client) {
this.client = client;
}
public BulkloadStreamBuilder schema(String schema) {
this.schema = schema;
return this;
}
public BulkloadStreamBuilder table(String table) {
this.table = table;
return this;
}
public BulkloadStreamBuilder operate(RowStream.BulkLoadOperate operate) {
this.operate = operate;
return this;
}
public BulkloadStreamBuilder recordKeys(List recordKeys) {
this.recordKeys = recordKeys;
return this;
}
public BulkloadStreamBuilder streamId(String streamId) {
this.streamId = streamId;
return this;
}
private void validate() {
if (client == null) {
throw new IllegalArgumentException("client is null");
}
if (schema == null || schema.isEmpty()) {
if (client.getSchema() == null || client.getSchema().isEmpty()) {
throw new IllegalArgumentException("schema is null or empty");
} else {
schema = client.getSchema();
}
}
if (table == null || table.isEmpty()) {
throw new IllegalArgumentException("table is null or empty");
}
}
public BulkloadStream build() throws IOException {
if (streamId != null && !streamId.isEmpty()) {
return new BulkloadStream(client, streamId);
}
validate();
BulkLoadOperation operation = BulkLoadOperation.APPEND;
if (operate == RowStream.BulkLoadOperate.OVERWRITE) {
operation = BulkLoadOperation.OVERWRITE;
} else if (operate == RowStream.BulkLoadOperate.UPSERT) {
operation = BulkLoadOperation.UPSERT;
}
BulkLoadOptions options = BulkLoadOptions.newBuilder()
.withOperation(operation)
.withRecordKeys(recordKeys)
.build();
return new BulkloadStream(client, schema, table, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy