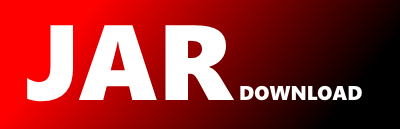
com.clickzetta.client.ClickZettaClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickzetta-java Show documentation
Show all versions of clickzetta-java Show documentation
The java SDK for clickzetta's Lakehouse
package com.clickzetta.client;
import com.clickzetta.client.jdbc.ClickZettaDriver;
import com.clickzetta.platform.client.api.Client;
import java.io.IOException;
import java.io.InputStream;
import java.sql.Connection;
import java.sql.DriverManager;
import java.util.Map;
import java.util.Properties;
public class ClickZettaClient {
public static String SDK_INFO;
static {
try (InputStream input = ClickZettaDriver.class.getClassLoader().getResourceAsStream("sdk.properties");
InputStream gitInput = ClickZettaDriver.class.getClassLoader().getResourceAsStream("git.properties")) {
Properties properties = new Properties();
Properties gitProperties = new Properties();
properties.load(input);
gitProperties.load(gitInput);
String name = properties.getProperty("sdk.name");
String version = properties.getProperty("sdk.version");
String gitCommitId = gitProperties.getProperty("git.commit.id.abbrev");
SDK_INFO = String.format("%s:%s:%s", name, version, gitCommitId);
} catch (IOException e) {
e.printStackTrace();
}
}
public static final String JDBC_PREFIX = "jdbc:";
public static final String IGS_PREFIX = "igs:";
private Client igsClient;
private Connection conn;
private String service;
private String instance;
private String workspace;
private String schema;
private String vcluster;
private String url;
public static ClickZettaClientBuilder newBuilder() {
return new ClickZettaClientBuilder();
}
public ClickZettaClient(String service,
String instance,
String workspace,
String vcluster,
Map optionalParams) {
this.service = service;
this.instance = instance;
this.workspace = workspace;
this.schema = optionalParams.get("schema");
this.vcluster = vcluster;
validate(optionalParams);
this.url = ClickZettaClientBuilder.getUrl(service, instance, workspace, vcluster, optionalParams);
}
private void validate(Map optionalParams) {
if (this.service == null || this.service.isEmpty()) {
throw new IllegalArgumentException("service must be specified");
}
if (this.instance == null || this.instance.isEmpty()) {
throw new IllegalArgumentException("instance must be specified");
}
if (this.workspace == null || this.workspace.isEmpty()) {
throw new IllegalArgumentException("workspace must be specified");
}
if (vcluster == null || vcluster.isEmpty()) {
throw new IllegalArgumentException("vcluster must be specified");
}
if (!(optionalParams.containsKey("username") && optionalParams.containsKey("password")) &&
!optionalParams.containsKey("magic_token")) {
throw new IllegalArgumentException("username and password or token must be specified");
}
if (!optionalParams.containsKey("schema")) {
optionalParams.put("schema", "public");
}
}
public synchronized Connection getJdbcConnection() {
if (conn == null) {
try {
Class.forName("com.clickzetta.client.jdbc.ClickZettaDriver");
conn = DriverManager.getConnection(JDBC_PREFIX + url, new Properties());
} catch (Exception e) {
throw new RuntimeException(e);
}
}
return conn;
}
public synchronized Client getIgsClient() {
if (igsClient == null) {
igsClient = Client.getBuilder()
.authenticate(true)
.streamUrl(IGS_PREFIX + url)
.build();
}
return igsClient;
}
public String getService() {
return service;
}
public String getInstance() {
return instance;
}
public String getWorkspace() {
return workspace;
}
public String getSchema() {
return schema;
}
public String getVCluster() {
return vcluster;
}
public RealtimeStreamBuilder newRealtimeStreamBuilder() {
return new RealtimeStreamBuilder(this);
}
public BulkloadStreamBuilder newBulkloadStreamBuilder() {
return new BulkloadStreamBuilder(this);
}
public MultiStreamBuilder newMultiStreamBuilder() {
return new MultiStreamBuilder(this);
}
public void close() {
try {
if (igsClient != null) {
igsClient.close();
}
if (conn != null) {
conn.close();
}
} catch (Exception e) {
throw new RuntimeException(e);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy