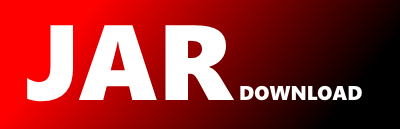
com.clickzetta.client.RealtimeStream Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickzetta-java Show documentation
Show all versions of clickzetta-java Show documentation
The java SDK for clickzetta's Lakehouse
package com.clickzetta.client;
import com.clickzetta.platform.client.Table;
import com.clickzetta.platform.client.api.Options;
import com.clickzetta.platform.client.api.Row;
import com.clickzetta.platform.client.api.Stream;
import java.io.IOException;
import java.sql.SQLException;
public class RealtimeStream implements RowStream {
private RealTimeOperate operate;
private Stream stream;
RealtimeStream(ClickZettaClient client, RealTimeOperate operate, String schema, String table, Options options)
throws IOException {
this.operate = operate;
this.stream = client.getIgsClient().createStream(schema, table, options);
}
@Override
public Table getTable() {
return stream.getTable();
}
@Override
public Row createRow() throws SQLException {
return createRow(Stream.Operator.UPSERT);
}
/**
* Create a Row with operator for RealtimeStream.
* @param operator operator is used to identify which operation the row belongs to.
* @return
* @throws SQLException
*/
@Override
public Row createRow(Stream.Operator operator) throws SQLException {
if (operate == RealTimeOperate.APPEND_ONLY && operator != Stream.Operator.INSERT) {
throw new SQLException("Append Only stream only support insert operation");
}
return stream.createRow(operator);
}
/**
* Not Suggested in RealtimeStream.
* @param partitionId
* @return
* @throws SQLException
*/
@Override
public Row createRow(int partitionId) throws SQLException {
return createRow(Stream.Operator.UPSERT);
}
/**
* Apply row to RealtimeStream.
* @param row
* @throws IOException
* @throws SQLException
*/
@Override
public void apply(Row row) throws IOException, SQLException {
if (stream != null) {
stream.apply(row);
} else {
throw new SQLException("realtime stream not init yet");
}
}
/**
* Not Avaliable in RealtimeStream.
* @param row
* @param partitionId
* @throws SQLException
*/
@Override
public void apply(Row row, int partitionId) throws SQLException, IOException {
this.apply(row);
}
/**
* Close the stream to Release Resources for RealtimeStream.
* @throws IOException
* @throws SQLException
*/
@Override
public void close() throws IOException, SQLException {
if (stream != null) {
stream.flush();
stream.close();
} else {
throw new SQLException("realtime stream not init yet");
}
}
@Override
public String getStreamId() {
return null;
}
@Override
public StreamState getState() {
return StreamState.SUCCESS;
}
@Override
public String getErrorMessage() {
return null;
}
public void flush() throws IOException {
stream.flush();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy