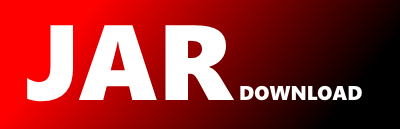
com.clickzetta.client.RowStream Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickzetta-java Show documentation
Show all versions of clickzetta-java Show documentation
The java SDK for clickzetta's Lakehouse
package com.clickzetta.client;
import com.clickzetta.platform.client.Table;
import com.clickzetta.platform.client.api.Row;
import com.clickzetta.platform.client.api.Stream;
import java.io.IOException;
import java.sql.SQLException;
public interface RowStream {
public enum RealTimeOperate {
CDC,
APPEND_ONLY
}
public enum BulkLoadOperate {
APPEND,
OVERWRITE,
UPSERT
}
/**
* Get table meta data.
*/
Table getTable();
/**
* Create a row for RealtimeStream/BulkloadStream.
* @return
* @throws SQLException
*/
Row createRow() throws SQLException;
/**
* Create a row for RealtimeStream.
* @param operator
* @return
* @throws SQLException
*/
Row createRow(Stream.Operator operator) throws SQLException;
/**
* Create a row for BulkloadStream.
* @param partitionId
* @return
* @throws SQLException
*/
Row createRow(int partitionId) throws SQLException;
/**
* Apply row to RealtimeStream.
* @param row
* @throws IOException
* @throws SQLException
*/
void apply(Row row) throws IOException, SQLException;
/**
* Apply row to BulkloadStream.
* @param row
* @param partitionId
* @throws SQLException
*/
void apply(Row row, int partitionId) throws SQLException, IOException;
/**
* Close Stream
* This method should be called after all rows are applied.
* @throws IOException
* @throws SQLException
*/
void close() throws IOException, SQLException;
/**
* Get StreamId
* @return
*/
String getStreamId();
/**
* Get StreamState
* @return
*/
StreamState getState();
/**
* Get ErrorMessage
* @return
*/
String getErrorMessage();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy