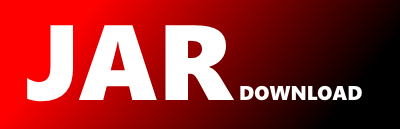
com.clickzetta.client.jdbc.core.CZConnection Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickzetta-java Show documentation
Show all versions of clickzetta-java Show documentation
The java SDK for clickzetta's Lakehouse
package com.clickzetta.client.jdbc.core;
import java.sql.*;
import java.util.*;
import java.util.concurrent.Executor;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class CZConnection implements java.sql.Connection {
static private final Logger logger = LoggerFactory.getLogger(CZConnection.class);
private boolean readOnly = false;
private boolean autoCommit = false;
private int networkTimeout = 0;
private boolean isClosed;
private final String connectionGuid = UUID.randomUUID().toString();
private CZConnectContext connectContext;
private Timer timer = null;
private int transactionIsolation = TRANSACTION_REPEATABLE_READ;
public CZConnection(CZConnectContext context) {
initConnection(context);
logger.info("Create Connection: " + connectionGuid);
}
/**
* export interface
*/
String getURL() {
return connectContext.getURL();
}
String getUserName() {
return connectContext.getUserName();
}
public String getId() {
return connectionGuid;
}
public CZConnectContext.RunningMode getRunningMode() {
return connectContext.getRunningMode();
}
public void setConfig(String key, String value) {
connectContext.setConfig(key, value);
}
public void resetConfig(String key) {
connectContext.resetConfig(key);
}
/**
* connection interface
*/
@Override
public Statement createStatement() throws SQLException {
CZStatement statement = new CZStatement(connectContext);
// openStatements.add(statement);
return statement;
}
@Override
public PreparedStatement prepareStatement(String s) throws SQLException {
logger.info("call CZConnection::prepareStatement");
CZPreparedStatment ps = new CZPreparedStatment(s, connectContext);
// openStatements.add(ps);
return ps;
}
@Override
public CallableStatement prepareCall(String s) throws SQLException {
logger.info("call CZConnection::prepareCall");
throw new CZExceptionNotSupported("CZConnection::prepareCall");
}
@Override
public String nativeSQL(String s) throws SQLException {
return s;
}
@Override
public void setAutoCommit(boolean b) throws SQLException {
// Fine Report won't work if throw exception here
logger.info("call CZConnection::setAutoCommit");
autoCommit = b;
return;
// throw new CZExceptionNotSupported("CZConnection::setAutoCommit");
}
@Override
public boolean getAutoCommit() throws SQLException {
// Fine Report won't work if throw exception here
logger.info("call CZConnection::getAutoCommit");
return autoCommit;
// throw new CZExceptionNotSupported("CZConnection::getAutoCommit");
}
@Override
public void commit() throws SQLException {
logger.info("call CZConnection::commit");
// throw new CZExceptionNotSupported("CZConnection::commit");
}
@Override
public void rollback() throws SQLException {
logger.info("call CZConnection::rollback");
// throw new CZExceptionNotSupported("CZConnection::rollback");
}
@Override
public void close() throws SQLException {
if (isClosed) {
return;
}
if (timer != null) {
timer.cancel();
}
isClosed = true;
}
@Override
public boolean isClosed() throws SQLException {
// logger.debug(" public boolean isClosed()");
return isClosed;
}
@Override
public DatabaseMetaData getMetaData() throws SQLException {
CZDatabaseMetaData metaData = new CZDatabaseMetaData(this);
return metaData;
}
@Override
public void setReadOnly(boolean b) throws SQLException {
logger.info("call CZConnection::setReadOnly");
readOnly = b;
}
@Override
public boolean isReadOnly() throws SQLException {
logger.info("call CZConnection::isReadOnly");
return readOnly;
}
@Override
public void setCatalog(String s) throws SQLException {
connectContext.setWorkspace(s);
}
@Override
public String getCatalog() throws SQLException {
return connectContext.getWorkspace();
}
@Override
public void setTransactionIsolation(int i) throws SQLException {
transactionIsolation = i;
}
@Override
public int getTransactionIsolation() throws SQLException {
logger.info("call CZConnection::getTransactionIsolation");
return TRANSACTION_REPEATABLE_READ;
}
@Override
public SQLWarning getWarnings() throws SQLException {
return null;
}
@Override
public void clearWarnings() throws SQLException {
}
@Override
public PreparedStatement prepareStatement(String s, int i, int i1) throws SQLException {
logger.info("call CZConnection::prepareStatement, with type {} and concurrency {}", i, i1);
// only support ResultSet.TYPE_FORWARD_ONLY and ResultSet.CONCUR_READ_ONLY
return new CZPreparedStatment(s, connectContext);
}
@Override
public CallableStatement prepareCall(String s, int i, int i1) throws SQLException {
logger.info("call CZConnection::prepareCall");
throw new CZExceptionNotSupported("CZConnection::prepareCall");
}
@Override
public Map> getTypeMap() throws SQLException {
logger.info("call CZConnection::getTypeMap");
throw new CZExceptionNotSupported("CZConnection::getTypeMap");
}
@Override
public void setTypeMap(Map> map) throws SQLException {
logger.info("call CZConnection::setTypeMap");
throw new CZExceptionNotSupported("CZConnection::setTypeMap");
}
@Override
public void setHoldability(int i) throws SQLException {
logger.info("call CZConnection::setHoldability");
throw new CZExceptionNotSupported("CZConnection::setHoldability");
}
@Override
public int getHoldability() throws SQLException {
logger.info("call CZConnection::getHoldability");
throw new CZExceptionNotSupported("CZConnection::getHoldability");
}
@Override
public Savepoint setSavepoint() throws SQLException {
logger.info("call CZConnection::setSavepoint");
throw new CZExceptionNotSupported("CZConnection::setSavepoint");
}
@Override
public Savepoint setSavepoint(String s) throws SQLException {
logger.info("call CZConnection::setSavepointString");
throw new CZExceptionNotSupported("CZConnection::setSavepointString");
}
@Override
public void rollback(Savepoint savepoint) throws SQLException {
logger.info("call CZConnection::rollback");
throw new CZExceptionNotSupported("CZConnection::rollback");
}
@Override
public void releaseSavepoint(Savepoint savepoint) throws SQLException {
logger.info("call CZConnection::releaseSavepoint");
throw new CZExceptionNotSupported("CZConnection::releaseSavepoint");
}
@Override
public Statement createStatement(int i, int i1) throws SQLException {
logger.info("call CZConnection::createStatementIntInt");
CZStatement statement = new CZStatement(connectContext);
// openStatements.add(statement);
return statement;
}
@Override
public Statement createStatement(int i, int i1, int i2) throws SQLException {
logger.info("call CZConnection::createStatementIntIntInt");
CZStatement statement = new CZStatement(connectContext);
// openStatements.add(statement);
return statement;
}
@Override
public PreparedStatement prepareStatement(String s, int i, int i1, int i2) throws SQLException {
logger.info("call CZConnection::prepareStatement, with resultSetType {}, " +
"resultSetConcurrency {} and resultSetHoldability {}", i, i1, i2);
// only support ResultSet.TYPE_FORWARD_ONLY ResultSet.CONCUR_READ_ONLY and ResultSet.HOLD_CURSORS_OVER_COMMIT
return new CZPreparedStatment(s, connectContext);
}
@Override
public CallableStatement prepareCall(String s, int i, int i1, int i2) throws SQLException {
logger.info("call CZConnection::prepareCallStringIntIntInt");
throw new CZExceptionNotSupported("CZConnection::prepareCallStringIntIntInt");
}
@Override
public PreparedStatement prepareStatement(String s, int i) throws SQLException {
logger.info("call CZConnection::prepareStatementStringInt");
throw new CZExceptionNotSupported("CZConnection::prepareStatementStringInt");
}
@Override
public PreparedStatement prepareStatement(String s, int[] ints) throws SQLException {
logger.info("call CZConnection::prepareStatementStringInts");
throw new CZExceptionNotSupported("CZConnection::prepareStatementStringInts");
}
@Override
public PreparedStatement prepareStatement(String s, String[] strings) throws SQLException {
logger.info("call CZConnection::prepareStatementStringStrings");
throw new CZExceptionNotSupported("CZConnection::prepareStatementStringStrings");
}
@Override
public Clob createClob() throws SQLException {
logger.info("call CZConnection::createClob");
throw new CZExceptionNotSupported("CZConnection::createClob");
}
@Override
public Blob createBlob() throws SQLException {
logger.info("call CZConnection::createBlob");
throw new CZExceptionNotSupported("CZConnection::createBlob");
}
@Override
public NClob createNClob() throws SQLException {
logger.info("call CZConnection::createNClob");
throw new CZExceptionNotSupported("CZConnection::createNClob");
}
@Override
public SQLXML createSQLXML() throws SQLException {
logger.info("call CZConnection::createSQLXML");
throw new CZExceptionNotSupported("CZConnection::createSQLXML");
}
@Override
public boolean isValid(int i) throws SQLException {
logger.info("call CZConnection::isValid");
if (i < 0) {
throw new SQLException("timeout value is negative");
}
int originalTimeout = connectContext.getQueryTimeout();
connectContext.setQueryTimeout(i);
String schema = connectContext.getSchema();
if (schema == null || schema.isEmpty()) {
connectContext.setSchema("public");
}
try (Statement statement = createStatement()) {
statement.execute("select 1");
} catch (Throwable t) {
return false;
} finally {
connectContext.setQueryTimeout(originalTimeout);
connectContext.setSchema(schema);
}
return true;
}
@Override
public void setClientInfo(String s, String s1) throws SQLClientInfoException {
logger.info("call CZConnection::setClientInfoString");
throw new SQLClientInfoException();
}
@Override
public void setClientInfo(Properties properties) throws SQLClientInfoException {
logger.info("call CZConnection::setClientInfo");
throw new SQLClientInfoException();
}
@Override
public String getClientInfo(String s) throws SQLException {
logger.info("call CZConnection::getClientInfoString");
throw new CZExceptionNotSupported("CZConnection::getClientInfoString");
}
@Override
public Properties getClientInfo() throws SQLException {
logger.info("call CZConnection::getClientInfo");
throw new CZExceptionNotSupported("CZConnection::getClientInfo");
}
@Override
public Array createArrayOf(String s, Object[] objects) throws SQLException {
logger.info("call CZConnection::createArrayOf");
throw new CZExceptionNotSupported("CZConnection::createArrayOf");
}
@Override
public Struct createStruct(String s, Object[] objects) throws SQLException {
logger.info("call CZConnection::createStruct");
throw new CZExceptionNotSupported("CZConnection::createStruct");
}
@Override
public void setSchema(String s) throws SQLException {
logger.info("call CZConnection::setSchema");
connectContext.setSchema(s);
}
@Override
public String getSchema() throws SQLException {
logger.info("call CZConnection::getSchema");
return connectContext.getSchema();
}
@Override
public void abort(Executor executor) throws SQLException {
logger.info("call CZConnection::abort");
throw new CZExceptionNotSupported("CZConnection::abort");
}
@Override
public void setNetworkTimeout(Executor executor, int i) throws SQLException {
logger.info("call CZConnection::setNetworkTimeout, not support yet.");
networkTimeout = i;
// throw new CZExceptionNotSupported("CZConnection::setNetworkTimeout");
}
@Override
public int getNetworkTimeout() throws SQLException {
logger.info("call CZConnection::getNetworkTimeout, not support yet.");
return networkTimeout;
// throw new CZExceptionNotSupported("CZConnection::getNetworkTimeout");
}
@Override
public T unwrap(Class aClass) throws SQLException {
if (!aClass.isInstance(this)) {
throw new SQLException(
this.getClass().getName() + " not unwrappable from " + aClass.getName());
}
return (T) this;
}
@Override
public boolean isWrapperFor(Class> aClass) throws SQLException {
return aClass.isInstance(this);
}
private void initConnection(CZConnectContext czc) {
if (czc.getRunningMode() == CZConnectContext.RunningMode.GATEWAY) {
czc.setLakehouseClient(new LakehouseHttpClient());
long delay = czc.getTokenExpireTimeMs();
if (czc.getToken() == null || czc.getToken().isEmpty()) {
getToken(czc);
} else {
delay = 0;
}
timer = new Timer(true);
TimerTask refreshTokenTask = new TimerTask() {
@Override
public void run() {
getToken(czc);
}
};
timer.schedule(refreshTokenTask, delay, czc.getTokenExpireTimeMs());
}
connectContext = czc;
}
private void getToken(CZConnectContext czc) {
logger.info("TrytoGetToken...");
try {
String token = czc.getHttpHelper().getToken(czc);
czc.setToken(token);
logger.info("Get token success.");
} catch (Exception e) {
logger.error("Refresh token fail, {}", e.getMessage());
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy