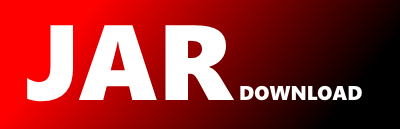
com.clickzetta.client.jdbc.core.CZDatabaseMetaData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickzetta-java Show documentation
Show all versions of clickzetta-java Show documentation
The java SDK for clickzetta's Lakehouse
package com.clickzetta.client.jdbc.core;
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.JSONObject;
import cz.proto.coordinator.CoordinatorServiceOuterClass;
import java.sql.*;
import java.util.*;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class CZDatabaseMetaData implements DatabaseMetaData {
private final CZConnection connection;
static Logger logger = LoggerFactory.getLogger(CZDatabaseMetaData.class);
public static final String NumericFunctionsSupported =
"ABS,ACOS,ASIN,ATAN,ATAN2,CBRT,CEILING,COS,COT,DEGREES,EXP,FACTORIAL,"
+ "FLOOR,HAVERSINE,LN,LOG,MOD,PI,POWER,RADIANS,RAND,"
+ "ROUND,SIGN,SIN,SQRT,SQUARE,TAN,TRUNCATE";
public static final String StringFunctionsSupported =
"ASCII,BIT_LENGTH,CHAR,CONCAT,INSERT,LCASE,LEFT,LENGTH,LPAD,"
+ "LOCATE,LTRIM,OCTET_LENGTH,PARSE_IP,PARSE_URL,REPEAT,REVERSE,"
+ "REPLACE,RPAD,RTRIMMED_LENGTH,SPACE,SPLIT,SPLIT_PART,"
+ "SPLIT_TO_TABLE,STRTOK,STRTOK_TO_ARRAY,STRTOK_SPLIT_TO_TABLE,"
+ "TRANSLATE,TRIM,UNICODE,UUID_STRING,INITCAP,LOWER,UPPER,REGEXP,"
+ "REGEXP_COUNT,REGEXP_INSTR,REGEXP_LIKE,REGEXP_REPLACE,"
+ "REGEXP_SUBSTR,RLIKE,CHARINDEX,CONTAINS,EDITDISTANCE,ENDSWITH,"
+ "ILIKE,ILIKE ANY,LIKE,LIKE ALL,LIKE ANY,POSITION,REPLACE,RIGHT,"
+ "STARTSWITH,SUBSTRING,COMPRESS,DECOMPRESS_BINARY,DECOMPRESS_STRING,"
+ "BASE64_DECODE_BINARY,BASE64_DECODE_STRING,BASE64_ENCODE,"
+ "HEX_DECODE_BINARY,HEX_DECODE_STRING,HEX_ENCODE,"
+ "TRY_BASE64_DECODE_BINARY,TRY_BASE64_DECODE_STRING,"
+ "TRY_HEX_DECODE_BINARY,TRY_HEX_DECODE_STRING,MD_5,MD5_HEX,"
+ "MD5_BINARY,SHA1,SHA1_HEX,SHA2,SHA1_BINARY,SHA2_HEX,SHA2_BINARY,"
+ " HASH,HASH_AGG,COLLATE,COLLATION";
private static final String DateAndTimeFunctionsSupported =
"CURDATE,"
+ "CURTIME,DAYNAME,DAYOFMONTH,DAYOFWEEK,DAYOFYEAR,HOUR,MINUTE,MONTH,"
+ "MONTHNAME,NOW,QUARTER,SECOND,TIMESTAMPADD,TIMESTAMPDIFF,WEEK,YEAR";
public CZDatabaseMetaData(CZConnection connection) throws SQLException {
logger.info("call DatabaseMetaData::CZDatabaseMetaData");
this.connection = connection;
}
@Override
public boolean allProceduresAreCallable() throws SQLException {
return false;
}
@Override
public boolean allTablesAreSelectable() throws SQLException {
return true;
}
@Override
public String getURL() throws SQLException {
logger.info("getURL called: {}", connection.getURL());
return connection.getURL();
}
@Override
public String getUserName() throws SQLException {
logger.info("getUserName called: {}", connection.getUserName());
return connection.getUserName();
}
@Override
public boolean isReadOnly() throws SQLException {
return false;
}
@Override
public boolean nullsAreSortedHigh() throws SQLException {
return false;
}
@Override
public boolean nullsAreSortedLow() throws SQLException {
return true;
}
@Override
public boolean nullsAreSortedAtStart() throws SQLException {
return false;
}
@Override
public boolean nullsAreSortedAtEnd() throws SQLException {
return false;
}
@Override
public String getDatabaseProductName() throws SQLException {
return "Lakehouse";
// throw new CZExceptionNotSupported("DatabaseMetaData::getDatabaseProductName");
}
@Override
public String getDatabaseProductVersion() throws SQLException {
// throw new CZExceptionNotSupported("DatabaseMetaData::getDatabaseProductVersion");
return "V1";
}
@Override
public String getDriverName() throws SQLException {
return "Lakehouse";
// throw new CZExceptionNotSupported("DatabaseMetaData::getDriverName");
}
@Override
public String getDriverVersion() throws SQLException {
return "v1.1";
// throw new CZExceptionNotSupported("DatabaseMetaData::getDriverVersion");
}
@Override
public int getDriverMajorVersion() {
// TODO: yingjie
return 1;
}
@Override
public int getDriverMinorVersion() {
// TODO: yingjie
return 1;
}
@Override
public boolean usesLocalFiles() throws SQLException {
return false;
}
@Override
public boolean usesLocalFilePerTable() throws SQLException {
return false;
}
@Override
public boolean supportsMixedCaseIdentifiers() throws SQLException {
return false;
}
@Override
public boolean storesUpperCaseIdentifiers() throws SQLException {
return false;
}
@Override
public boolean storesLowerCaseIdentifiers() throws SQLException {
return true;
}
@Override
public boolean storesMixedCaseIdentifiers() throws SQLException {
return false;
}
@Override
public boolean supportsMixedCaseQuotedIdentifiers() throws SQLException {
return false;
}
@Override
public boolean storesUpperCaseQuotedIdentifiers() throws SQLException {
return false;
}
@Override
public boolean storesLowerCaseQuotedIdentifiers() throws SQLException {
return false;
}
@Override
public boolean storesMixedCaseQuotedIdentifiers() throws SQLException {
return false;
}
@Override
public String getIdentifierQuoteString() throws SQLException {
return "`";
}
@Override
public String getSQLKeywords() throws SQLException {
throw new CZExceptionNotSupported("DatabaseMetaData::getSQLKeywords");
}
@Override
public String getNumericFunctions() throws SQLException {
logger.debug("public String getNumericFunctions()");
return NumericFunctionsSupported;
}
@Override
public String getStringFunctions() throws SQLException {
logger.debug("public String getStringFunctions()");
return StringFunctionsSupported;
}
@Override
public String getSystemFunctions() throws SQLException {
throw new CZExceptionNotSupported("DatabaseMetaData::getSystemFunctions");
}
@Override
public String getTimeDateFunctions() throws SQLException {
logger.info("public String getTimeDateFunctions()");
return DateAndTimeFunctionsSupported;
}
@Override
public String getSearchStringEscape() throws SQLException {
return "\\";
}
@Override
public String getExtraNameCharacters() throws SQLException {
return "";
}
@Override
public boolean supportsAlterTableWithAddColumn() throws SQLException {
return true;
}
@Override
public boolean supportsAlterTableWithDropColumn() throws SQLException {
return true;
}
@Override
public boolean supportsColumnAliasing() throws SQLException {
return true;
}
@Override
public boolean nullPlusNonNullIsNull() throws SQLException {
return true;
}
@Override
public boolean supportsConvert() throws SQLException {
return false;
}
@Override
public boolean supportsConvert(int fromType, int toType) throws SQLException {
return false;
}
@Override
public boolean supportsTableCorrelationNames() throws SQLException {
throw new CZExceptionNotSupported("DatabaseMetaData::supportsTableCorrelationNames");
}
@Override
public boolean supportsDifferentTableCorrelationNames() throws SQLException {
throw new CZExceptionNotSupported("DatabaseMetaData::supportsDifferentTableCorrelationNames");
}
@Override
public boolean supportsExpressionsInOrderBy() throws SQLException {
return true;
}
@Override
public boolean supportsOrderByUnrelated() throws SQLException {
return true;
}
@Override
public boolean supportsGroupBy() throws SQLException {
return true;
}
@Override
public boolean supportsGroupByUnrelated() throws SQLException {
return true;
}
@Override
public boolean supportsGroupByBeyondSelect() throws SQLException {
throw new CZExceptionNotSupported("DatabaseMetaData::supportsGroupByBeyondSelect");
}
@Override
public boolean supportsLikeEscapeClause() throws SQLException {
return false;
}
@Override
public boolean supportsMultipleResultSets() throws SQLException {
return false;
}
@Override
public boolean supportsMultipleTransactions() throws SQLException {
return false;
}
@Override
public boolean supportsNonNullableColumns() throws SQLException {
return true;
}
@Override
public boolean supportsMinimumSQLGrammar() throws SQLException {
return false;
}
@Override
public boolean supportsCoreSQLGrammar() throws SQLException {
return false;
}
@Override
public boolean supportsExtendedSQLGrammar() throws SQLException {
return false;
}
@Override
public boolean supportsANSI92EntryLevelSQL() throws SQLException {
return false;
}
@Override
public boolean supportsANSI92IntermediateSQL() throws SQLException {
return false;
}
@Override
public boolean supportsANSI92FullSQL() throws SQLException {
return false;
}
@Override
public boolean supportsIntegrityEnhancementFacility() throws SQLException {
throw new CZExceptionNotSupported("DatabaseMetaData::supportsIntegrityEnhancementFacility");
}
@Override
public boolean supportsOuterJoins() throws SQLException {
return true;
}
@Override
public boolean supportsFullOuterJoins() throws SQLException {
return true;
}
@Override
public boolean supportsLimitedOuterJoins() throws SQLException {
return true;
}
@Override
public String getSchemaTerm() throws SQLException {
throw new CZExceptionNotSupported("DatabaseMetaData::getSchemaTerm");
}
@Override
public String getProcedureTerm() throws SQLException {
throw new CZExceptionNotSupported("DatabaseMetaData::getProcedureTerm");
}
@Override
public String getCatalogTerm() throws SQLException {
return connection.getCatalog();
// throw new CZExceptionNotSupported("DatabaseMetaData::getCatalogTerm");
}
@Override
public boolean isCatalogAtStart() throws SQLException {
return true;
}
@Override
public String getCatalogSeparator() throws SQLException {
return ".";
}
@Override
public boolean supportsSchemasInDataManipulation() throws SQLException {
throw new CZExceptionNotSupported("DatabaseMetaData::supportsSchemasInDataManipulation");
}
@Override
public boolean supportsSchemasInProcedureCalls() throws SQLException {
throw new CZExceptionNotSupported("DatabaseMetaData::supportsSchemasInProcedureCalls");
}
@Override
public boolean supportsSchemasInTableDefinitions() throws SQLException {
throw new CZExceptionNotSupported("DatabaseMetaData::supportsSchemasInTableDefinitions");
}
@Override
public boolean supportsSchemasInIndexDefinitions() throws SQLException {
throw new CZExceptionNotSupported("DatabaseMetaData::supportsSchemasInIndexDefinitions");
}
@Override
public boolean supportsSchemasInPrivilegeDefinitions() throws SQLException {
throw new CZExceptionNotSupported("DatabaseMetaData::supportsSchemasInPrivilegeDefinitions");
}
@Override
public boolean supportsCatalogsInDataManipulation() throws SQLException {
throw new CZExceptionNotSupported("DatabaseMetaData::supportsCatalogsInDataManipulation");
}
@Override
public boolean supportsCatalogsInProcedureCalls() throws SQLException {
throw new CZExceptionNotSupported("DatabaseMetaData::supportsCatalogsInProcedureCalls");
}
@Override
public boolean supportsCatalogsInTableDefinitions() throws SQLException {
throw new CZExceptionNotSupported("DatabaseMetaData::supportsCatalogsInTableDefinitions");
}
@Override
public boolean supportsCatalogsInIndexDefinitions() throws SQLException {
throw new CZExceptionNotSupported("DatabaseMetaData::supportsCatalogsInIndexDefinitions");
}
@Override
public boolean supportsCatalogsInPrivilegeDefinitions() throws SQLException {
throw new CZExceptionNotSupported("DatabaseMetaData::supportsCatalogsInPrivilegeDefinitions");
}
@Override
public boolean supportsPositionedDelete() throws SQLException {
throw new CZExceptionNotSupported("DatabaseMetaData::supportsPositionedDelete");
}
@Override
public boolean supportsPositionedUpdate() throws SQLException {
throw new CZExceptionNotSupported("DatabaseMetaData::supportsPositionedUpdate");
}
@Override
public boolean supportsSelectForUpdate() throws SQLException {
throw new CZExceptionNotSupported("DatabaseMetaData::supportsSelectForUpdate");
}
@Override
public boolean supportsStoredProcedures() throws SQLException {
return false;
}
@Override
public boolean supportsSubqueriesInComparisons() throws SQLException {
throw new CZExceptionNotSupported("DatabaseMetaData::supportsSubqueriesInComparisons");
}
@Override
public boolean supportsSubqueriesInExists() throws SQLException {
throw new CZExceptionNotSupported("DatabaseMetaData::supportsSubqueriesInExists");
}
@Override
public boolean supportsSubqueriesInIns() throws SQLException {
throw new CZExceptionNotSupported("DatabaseMetaData::supportsSubqueriesInIns");
}
@Override
public boolean supportsSubqueriesInQuantifieds() throws SQLException {
throw new CZExceptionNotSupported("DatabaseMetaData::supportsSubqueriesInQuantifieds");
}
@Override
public boolean supportsCorrelatedSubqueries() throws SQLException {
throw new CZExceptionNotSupported("DatabaseMetaData::supportsCorrelatedSubqueries");
}
@Override
public boolean supportsUnion() throws SQLException {
return true;
}
@Override
public boolean supportsUnionAll() throws SQLException {
return true;
}
@Override
public boolean supportsOpenCursorsAcrossCommit() throws SQLException {
return false;
}
@Override
public boolean supportsOpenCursorsAcrossRollback() throws SQLException {
return false;
}
@Override
public boolean supportsOpenStatementsAcrossCommit() throws SQLException {
return false;
}
@Override
public boolean supportsOpenStatementsAcrossRollback() throws SQLException {
return false;
}
@Override
public int getMaxBinaryLiteralLength() throws SQLException {
logger.info("call DatabaseMetaData::getMaxBinaryLiteralLength");
return 8388608;
}
@Override
public int getMaxCharLiteralLength() throws SQLException {
logger.info("call DatabaseMetaData::getMaxCharLiteralLength");
return 16777216;
}
@Override
public int getMaxColumnNameLength() throws SQLException {
logger.info("call DatabaseMetaData::getMaxColumnNameLength");
return 255;
}
@Override
public int getMaxColumnsInGroupBy() throws SQLException {
logger.info("call DatabaseMetaData::getMaxColumnsInGroupBy");
return 0;
}
@Override
public int getMaxColumnsInIndex() throws SQLException {
logger.info("call DatabaseMetaData::getMaxColumnsInIndex");
throw new CZExceptionNotSupported("DatabaseMetaData::getMaxColumnsInIndex");
}
@Override
public int getMaxColumnsInOrderBy() throws SQLException {
logger.info("call DatabaseMetaData::getMaxColumnsInOrderBy");
throw new CZExceptionNotSupported("DatabaseMetaData::getMaxColumnsInOrderBy");
}
@Override
public int getMaxColumnsInSelect() throws SQLException {
logger.info("call DatabaseMetaData::getMaxColumnsInSelect");
throw new CZExceptionNotSupported("DatabaseMetaData::getMaxColumnsInSelect");
}
@Override
public int getMaxColumnsInTable() throws SQLException {
logger.info("call DatabaseMetaData::getMaxColumnsInTable");
throw new CZExceptionNotSupported("DatabaseMetaData::getMaxColumnsInTable");
}
@Override
public int getMaxConnections() throws SQLException {
logger.info("call DatabaseMetaData::getMaxConnections");
throw new CZExceptionNotSupported("DatabaseMetaData::getMaxConnections");
}
@Override
public int getMaxCursorNameLength() throws SQLException {
logger.info("call DatabaseMetaData::getMaxCursorNameLength");
throw new CZExceptionNotSupported("DatabaseMetaData::getMaxCursorNameLength");
}
@Override
public int getMaxIndexLength() throws SQLException {
logger.info("call DatabaseMetaData::getMaxIndexLength");
throw new CZExceptionNotSupported("DatabaseMetaData::getMaxIndexLength");
}
@Override
public int getMaxSchemaNameLength() throws SQLException {
logger.info("call DatabaseMetaData::getMaxSchemaNameLength");
return 255;
}
@Override
public int getMaxProcedureNameLength() throws SQLException {
logger.info("call DatabaseMetaData::getMaxProcedureNameLength");
throw new CZExceptionNotSupported("DatabaseMetaData::getMaxProcedureNameLength");
}
@Override
public int getMaxCatalogNameLength() throws SQLException {
logger.info("call DatabaseMetaData::getMaxCatalogNameLength");
return 255;
}
@Override
public int getMaxRowSize() throws SQLException {
logger.info("call DatabaseMetaData::getMaxRowSize");
throw new CZExceptionNotSupported("DatabaseMetaData::getMaxRowSize");
}
@Override
public boolean doesMaxRowSizeIncludeBlobs() throws SQLException {
logger.info("call DatabaseMetaData::doesMaxRowSizeIncludeBlobs");
throw new CZExceptionNotSupported("DatabaseMetaData::doesMaxRowSizeIncludeBlobs");
}
@Override
public int getMaxStatementLength() throws SQLException {
logger.info("call DatabaseMetaData::getMaxStatementLength");
throw new CZExceptionNotSupported("DatabaseMetaData::getMaxStatementLength");
}
@Override
public int getMaxStatements() throws SQLException {
logger.info("call DatabaseMetaData::getMaxStatements");
throw new CZExceptionNotSupported("DatabaseMetaData::getMaxStatements");
}
@Override
public int getMaxTableNameLength() throws SQLException {
logger.info("call DatabaseMetaData::getMaxTableNameLength");
return 255;
}
@Override
public int getMaxTablesInSelect() throws SQLException {
logger.info("call DatabaseMetaData::getMaxTablesInSelect");
throw new CZExceptionNotSupported("DatabaseMetaData::getMaxTablesInSelect");
}
@Override
public int getMaxUserNameLength() throws SQLException {
logger.info("call DatabaseMetaData::getMaxUserNameLength");
return 255;
}
@Override
public int getDefaultTransactionIsolation() throws SQLException {
logger.info("call DatabaseMetaData::getDefaultTransactionIsolation");
return Connection.TRANSACTION_REPEATABLE_READ;
// throw new CZExceptionNotSupported("DatabaseMetaData::getDefaultTransactionIsolation");
}
@Override
public boolean supportsTransactions() throws SQLException {
logger.info("call DatabaseMetaData::supportsTransactions");
return false;
// throw new CZExceptionNotSupported("DatabaseMetaData::supportsTransactions");
}
@Override
public boolean supportsTransactionIsolationLevel(int level) throws SQLException {
logger.info("call DatabaseMetaData::supportsTransactions");
return (level == Connection.TRANSACTION_NONE) || (level == Connection.TRANSACTION_REPEATABLE_READ);
}
@Override
public boolean supportsDataDefinitionAndDataManipulationTransactions() throws SQLException {
logger.info("call DatabaseMetaData::supportsDataDefinitionAndDataManipulationTransactions");
throw new CZExceptionNotSupported("DatabaseMetaData::supportsDataDefinitionAndDataManipulationTransactions");
}
@Override
public boolean supportsDataManipulationTransactionsOnly() throws SQLException {
logger.info("call DatabaseMetaData::supportsDataManipulationTransactionsOnly");
throw new CZExceptionNotSupported("DatabaseMetaData::supportsDataManipulationTransactionsOnly");
}
@Override
public boolean dataDefinitionCausesTransactionCommit() throws SQLException {
logger.info("call DatabaseMetaData::dataDefinitionCausesTransactionCommit");
throw new CZExceptionNotSupported("DatabaseMetaData::dataDefinitionCausesTransactionCommit");
}
@Override
public boolean dataDefinitionIgnoredInTransactions() throws SQLException {
logger.info("call DatabaseMetaData::dataDefinitionIgnoredInTransactions");
throw new CZExceptionNotSupported("DatabaseMetaData::dataDefinitionIgnoredInTransactions");
}
@Override
public ResultSet getProcedures(
final String catalog, final String schemaPattern, final String procedureNamePattern)
throws SQLException {
throw new CZExceptionNotSupported("DatabaseMetaData::getProcedures");
}
@Override
public ResultSet getProcedureColumns(
final String catalog,
final String schemaPattern,
final String procedureNamePattern,
final String columnNamePattern)
throws SQLException {
throw new CZExceptionNotSupported("DatabaseMetaData::getProcedureColumns");
}
@Override
public ResultSet getBestRowIdentifier(
String catalog, String schema, String table, int scope, boolean nullable)
throws SQLException {
throw new CZExceptionNotSupported("DatabaseMetaData::getBestRowIdentifier");
}
@Override
public ResultSet getVersionColumns(String catalog, String schema, String table)
throws SQLException {
throw new CZExceptionNotSupported("DatabaseMetaData::getVersionColumns");
}
@Override
public ResultSet getPrimaryKeys(String originalCatalog, String originalSchema, final String table)
throws SQLException {
/*
logger.info("call DatabaseMetaData::getPrimaryKeys");
List columnMetaDatas = new ArrayList<>();
CZColumnMetaData columnMetaData = new CZColumnMetaData("TABLE_CAT", "VARCHAR", java.sql.Types.VARCHAR);
columnMetaDatas.add(columnMetaData);
CZColumnMetaData columnMetaData2 = new CZColumnMetaData("TABLE_SCHEM", "VARCHAR", java.sql.Types.VARCHAR);
columnMetaDatas.add(columnMetaData2);
CZColumnMetaData columnMetaData3 = new CZColumnMetaData("TABLE_NAME", "VARCHAR", java.sql.Types.VARCHAR);
columnMetaDatas.add(columnMetaData3);
CZColumnMetaData columnMetaData4 = new CZColumnMetaData("COLUMN_NAME", "VARCHAR", java.sql.Types.VARCHAR);
columnMetaDatas.add(columnMetaData4);
CZColumnMetaData columnMetaData5 = new CZColumnMetaData("KEY_SEQ", "VARCHAR", java.sql.Types.VARCHAR);
columnMetaDatas.add(columnMetaData5);
CZColumnMetaData columnMetaData6 = new CZColumnMetaData("PK_NAME", "VARCHAR", java.sql.Types.VARCHAR);
columnMetaDatas.add(columnMetaData6);
List> dataList = new ArrayList<>();
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy