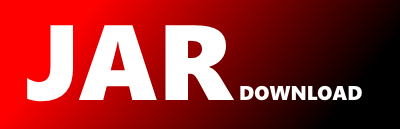
com.clickzetta.client.jdbc.core.CZResultSetMetaData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickzetta-java Show documentation
Show all versions of clickzetta-java Show documentation
The java SDK for clickzetta's Lakehouse
package com.clickzetta.client.jdbc.core;;
import java.sql.*;
import java.util.List;
public class CZResultSetMetaData implements ResultSetMetaData {
CZResultSetMetaData(List columnMetadatas) {
columnCount = columnMetadatas.size();
this.columnMetaDatas = columnMetadatas;
}
private final int columnCount;
private final List columnMetaDatas;
cz.proto.FieldSchema getFieldSchema(int column) {
return columnMetaDatas.get(column - 1).fieldSchema;
}
@Override
public int getColumnCount() throws SQLException {
return columnCount;
}
@Override
public boolean isAutoIncrement(int column) throws SQLException {
return false;
}
@Override
public boolean isCaseSensitive(int column) throws SQLException {
return false;
}
@Override
public boolean isSearchable(int column) throws SQLException {
return true;
}
@Override
public boolean isCurrency(int column) throws SQLException {
return false;
}
@Override
public int isNullable(int column) throws SQLException {
return columnMetaDatas.get(column - 1).isNullable();
}
@Override
public boolean isSigned(int column) throws SQLException {
return columnMetaDatas.get(column - 1).isSigned();
}
@Override
public int getColumnDisplaySize(int column) throws SQLException {
if (columnCount >= column && columnMetaDatas != null) {
return columnMetaDatas.get(column - 1).getColumnDisplayLength();
} else {
return 0;
}
}
@Override
public String getColumnLabel(int column) throws SQLException {
if (columnCount >= column && columnMetaDatas != null) {
return columnMetaDatas.get(column - 1).getColumnName();
} else {
return "C" + (column - 1);
}
}
@Override
public String getColumnName(int column) throws SQLException {
if (columnCount >= column && columnMetaDatas != null) {
return columnMetaDatas.get(column - 1).getColumnName();
} else {
return "C" + (column - 1);
}
}
@Override
public String getSchemaName(int column) throws SQLException {
throw new SQLException("NotSupported");
}
@Override
public int getPrecision(int column) throws SQLException {
return columnMetaDatas.get(column - 1).getPrecision();
}
@Override
public int getScale(int column) throws SQLException {
return columnMetaDatas.get(column - 1).getScale();
}
@Override
public String getTableName(int column) throws SQLException {
throw new SQLException("NotSupported");
}
@Override
public String getCatalogName(int column) throws SQLException {
throw new SQLException("NotSupported");
}
@Override
public int getColumnType(int column) throws SQLException {
if (columnCount >= column && columnMetaDatas != null) {
return columnMetaDatas.get(column - 1).getColumnType();
} else {
return 0;
}
}
@Override
public String getColumnTypeName(int column) throws SQLException {
if (columnCount >= column && columnMetaDatas != null) {
return columnMetaDatas.get(column - 1).getColumnTypeName();
} else {
return "";
}
}
@Override
public boolean isReadOnly(int column) throws SQLException {
return true;
}
@Override
public boolean isWritable(int column) throws SQLException {
return false;
}
@Override
public boolean isDefinitelyWritable(int column) throws SQLException {
return false;
}
@Override
public String getColumnClassName(int column) throws SQLException {
if (columnCount >= column && columnMetaDatas != null) {
return CZColumnMetaData.getColumnClassName(columnMetaDatas.get(column - 1).getColumnType());
}
throw new SQLException("getColumnClassName " + column + " error");
}
@Override
public T unwrap(Class iface) throws SQLException {
return null;
}
@Override
public boolean isWrapperFor(Class> iface) throws SQLException {
return false;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy