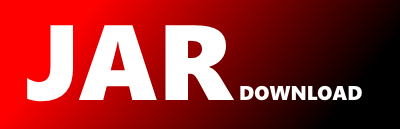
com.clickzetta.platform.arrow.writer.TimestampWriter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickzetta-java Show documentation
Show all versions of clickzetta-java Show documentation
The java SDK for clickzetta's Lakehouse
package com.clickzetta.platform.arrow.writer;
import com.clickzetta.platform.catalyst.data.DataGetters;
import com.clickzetta.platform.util.TimestampUtil;
import org.apache.arrow.vector.TimeStampVector;
import org.apache.arrow.vector.types.TimeUnit;
import org.apache.arrow.vector.types.Types;
import java.sql.Timestamp;
public class TimestampWriter extends ArrowFieldWriter {
private static final long EMPTY_LONG = 0L;
private TimeStampVector timeStampVector;
private TimeUnit timeUnit;
private String timeZoneId;
public TimestampWriter(TimeStampVector timeStampVector) {
super(timeStampVector);
this.timeStampVector = timeStampVector;
Types.MinorType minorType = timeStampVector.getMinorType();
switch (minorType) {
case TIMESTAMPSEC:
case TIMESTAMPSECTZ:
this.timeUnit = TimeUnit.SECOND;
break;
case TIMESTAMPMILLI:
case TIMESTAMPMILLITZ:
this.timeUnit = TimeUnit.MILLISECOND;
break;
case TIMESTAMPMICRO:
case TIMESTAMPMICROTZ:
this.timeUnit = TimeUnit.MICROSECOND;
break;
case TIMESTAMPNANO:
case TIMESTAMPNANOTZ:
this.timeUnit = TimeUnit.NANOSECOND;
break;
default:
throw new UnsupportedOperationException("not supported timestamp type with timeUnit: " + minorType);
}
}
@Override
public void setNull() {
timeStampVector.setNull(count);
}
@Override
void setDefaultValue() {
timeStampVector.setSafe(count, EMPTY_LONG);
}
@Override
void setValue(DataGetters row, int ordinal) {
Long time = null;
// Timestamp value.
Timestamp timestamp = row.getTimestamp(ordinal).toSQLTimestamp();
switch (this.timeUnit) {
case SECOND:
time = TimestampUtil.timestampToSec(timestamp);
break;
case MILLISECOND:
time = TimestampUtil.timestampToMillis(timestamp);
break;
case MICROSECOND:
time = TimestampUtil.timestampToMicros(timestamp);
break;
case NANOSECOND:
time = TimestampUtil.timestampToNano(timestamp);
break;
default:
throw new UnsupportedOperationException("not supported timeUnit with: " + timeUnit);
}
timeStampVector.setSafe(count, time);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy