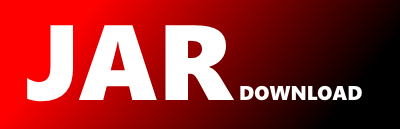
com.clickzetta.platform.bulkload.ProtoUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickzetta-java Show documentation
Show all versions of clickzetta-java Show documentation
The java SDK for clickzetta's Lakehouse
package com.clickzetta.platform.bulkload;
import com.clickzetta.platform.client.api.BulkLoadOperation;
import com.clickzetta.platform.client.api.BulkLoadState;
import com.google.common.base.Preconditions;
import cz.proto.FileFormatTypeOuterClass;
import cz.proto.ingestion.v2.IngestionV2;
public class ProtoUtils {
private ProtoUtils() {
}
public static FileFormatType fromProto(FileFormatTypeOuterClass.FileFormatType type) {
Preconditions.checkNotNull(type);
switch (type) {
case PARQUET:
return FileFormatType.PARQUET;
case ARROW:
return FileFormatType.ARROW;
default:
throw new UnsupportedOperationException("Unsupported file format " + type);
}
}
// proto v2
public static BulkLoadOperation fromProtoV2(IngestionV2.BulkLoadStreamOperation operation) {
Preconditions.checkNotNull(operation);
switch (operation) {
case BL_APPEND:
return BulkLoadOperation.APPEND;
case BL_UPSERT:
return BulkLoadOperation.UPSERT;
case BL_OVERWRITE:
return BulkLoadOperation.OVERWRITE;
default:
throw new IllegalArgumentException("Unknown BulkLoadOperation: " + operation);
}
}
public static IngestionV2.BulkLoadStreamOperation toProtoV2(BulkLoadOperation operation) {
Preconditions.checkNotNull(operation);
switch (operation) {
case APPEND:
return IngestionV2.BulkLoadStreamOperation.BL_APPEND;
case UPSERT:
return IngestionV2.BulkLoadStreamOperation.BL_UPSERT;
case OVERWRITE:
return IngestionV2.BulkLoadStreamOperation.BL_OVERWRITE;
default:
throw new IllegalArgumentException("Unknown BulkLoadOperation: " + operation);
}
}
public static BulkLoadState fromProtoV2(IngestionV2.BulkLoadStreamState state) {
Preconditions.checkNotNull(state);
switch (state) {
case BL_CREATED:
return BulkLoadState.CREATED;
case BL_SEALED:
return BulkLoadState.SEALED;
case BL_COMMIT_SUBMITTED:
return BulkLoadState.COMMIT_SUBMITTED;
case BL_COMMIT_SUCCESS:
return BulkLoadState.COMMIT_SUCCESS;
case BL_COMMIT_FAILED:
return BulkLoadState.COMMIT_FAILED;
case BL_CANCELLED:
return BulkLoadState.CANCELLED;
default:
throw new IllegalArgumentException("Unknown BulkLoadState: " + state);
}
}
public static IngestionV2.BulkLoadStreamState toProtoV2(BulkLoadState state) {
Preconditions.checkNotNull(state);
switch (state) {
case CREATED:
return IngestionV2.BulkLoadStreamState.BL_CREATED;
case SEALED:
return IngestionV2.BulkLoadStreamState.BL_SEALED;
case COMMIT_SUBMITTED:
return IngestionV2.BulkLoadStreamState.BL_COMMIT_SUBMITTED;
case COMMIT_SUCCESS:
return IngestionV2.BulkLoadStreamState.BL_COMMIT_SUCCESS;
case COMMIT_FAILED:
return IngestionV2.BulkLoadStreamState.BL_COMMIT_FAILED;
case CANCELLED:
return IngestionV2.BulkLoadStreamState.BL_CANCELLED;
default:
throw new IllegalArgumentException("Unknown BulkLoadState: " + state);
}
}
public static BulkLoadCommitMode fromProtoV2(IngestionV2.CommitBulkLoadStreamRequest.CommitMode mode) {
Preconditions.checkNotNull(mode);
switch (mode) {
case COMMIT_STREAM:
return BulkLoadCommitMode.COMMIT_STREAM;
case ABORT_STREAM:
return BulkLoadCommitMode.ABORT_STREAM;
default:
throw new IllegalArgumentException("Unknown BulkLoadCommitMode: " + mode);
}
}
public static IngestionV2.CommitBulkLoadStreamRequest.CommitMode toProtoV2(BulkLoadCommitMode mode) {
Preconditions.checkNotNull(mode);
switch (mode) {
case COMMIT_STREAM:
return IngestionV2.CommitBulkLoadStreamRequest.CommitMode.COMMIT_STREAM;
case ABORT_STREAM:
return IngestionV2.CommitBulkLoadStreamRequest.CommitMode.ABORT_STREAM;
default:
throw new IllegalArgumentException("Unknown BulkLoadCommitMode: " + mode);
}
}
public static StagingConfig fromProtoV2(IngestionV2.StagingPathInfo info) {
Preconditions.checkNotNull(info);
if (info.hasOssPath()) {
IngestionV2.OssStagingPathInfo ossPath = info.getOssPath();
return new StagingConfig(
ossPath.getPath(),
ossPath.getStsAkId(),
ossPath.getStsAkSecret(),
ossPath.getStsToken(),
ossPath.getOssEndpoint(),
ossPath.getOssInternalEndpoint(),
ossPath.getOssExpireTime());
} else if (info.hasCosPath()) {
IngestionV2.CosStagingPathInfo cosPath = info.getCosPath();
return new StagingConfig(
cosPath.getPath(),
cosPath.getStsAkId(),
cosPath.getStsAkSecret(),
cosPath.getStsToken(),
cosPath.getCosRegion(),
null,
cosPath.getCosExpireTime());
} else if (info.hasS3Path()) {
IngestionV2.S3StagingPathInfo s3Path = info.getS3Path();
return new StagingConfig(
s3Path.getPath(),
s3Path.getStsAkId(),
s3Path.getStsAkSecret(),
s3Path.getStsToken(),
s3Path.getS3Region(),
null,
s3Path.getS3ExpireTime());
} else if (info.hasGcsPath()) {
IngestionV2.GcsStagingPathInfo gcsPath = info.getGcsPath();
return new StagingConfig(
gcsPath.getPath(),
gcsPath.getStsAkId(),
gcsPath.getStsAkSecret(),
gcsPath.getStsToken(),
gcsPath.getGcsRegion(),
null,
gcsPath.getGcsExpireTime());
} else if (info.hasLocalPath()) {
IngestionV2.LocalStagingPathInfo localPath = info.getLocalPath();
return new StagingConfig(localPath.getPath(), null, null, null, null, null, Long.MAX_VALUE);
} else {
throw new UnsupportedOperationException("Unsupported StagingPathInfo: " + info);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy