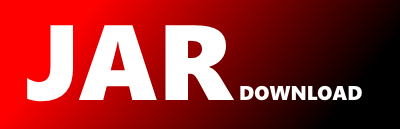
com.clickzetta.platform.catalyst.data.GenericMap Maven / Gradle / Ivy
Show all versions of clickzetta-java Show documentation
package com.clickzetta.platform.catalyst.data;
import java.io.Serializable;
import java.util.Map;
import java.util.Objects;
public final class GenericMap implements InternalMap, Serializable {
private static final long serialVersionUID = 1L;
private final Map, ?> map;
/**
* Creates an instance of {@link GenericMap} using the given Java map.
*
* Note: All keys and values of the map must be internal data structures.
*/
public GenericMap(Map, ?> map) {
this.map = map;
}
/**
* Returns the value to which the specified key is mapped, or {@code null} if this map contains
* no mapping for the key. The returned value is in internal data structure.
*/
public Object get(Object key) {
return map.get(key);
}
@Override
public int size() {
return map.size();
}
@Override
public InternalArray keyArray() {
Object[] keys = map.keySet().toArray();
return new GenericArray(keys);
}
@Override
public InternalArray valueArray() {
Object[] values = map.values().toArray();
return new GenericArray(values);
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (!(o instanceof GenericMap)) {
return false;
}
// deepEquals for values of byte[]
return deepEquals(map, ((GenericMap) o).map);
}
private static boolean deepEquals(Map m1, Map, ?> m2) {
// copied from HashMap.equals but with deepEquals comparison
if (m1.size() != m2.size()) {
return false;
}
try {
for (Map.Entry e : m1.entrySet()) {
K key = e.getKey();
V value = e.getValue();
if (value == null) {
if (!(m2.get(key) == null && m2.containsKey(key))) {
return false;
}
} else {
if (!Objects.deepEquals(value, m2.get(key))) {
return false;
}
}
}
} catch (ClassCastException | NullPointerException unused) {
return false;
}
return true;
}
@Override
public int hashCode() {
int result = 0;
for (Object key : map.keySet()) {
// only include key because values can contain byte[]
result += 31 * Objects.hashCode(key);
}
return result;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("GenericMap{");
int i = 0;
for (Map.Entry, ?> entry : map.entrySet()) {
if (i != 0) {
sb.append(",");
}
sb.append(entry.getKey());
sb.append(":");
sb.append(entry.getValue());
i++;
}
sb.append("}");
return sb.toString();
}
}