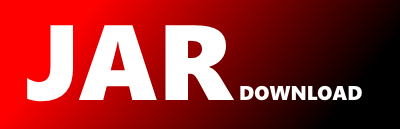
com.clickzetta.platform.client.CZClientBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickzetta-java Show documentation
Show all versions of clickzetta-java Show documentation
The java SDK for clickzetta's Lakehouse
package com.clickzetta.platform.client;
import com.clickzetta.platform.client.api.Client;
import com.clickzetta.platform.client.api.ClientBuilder;
import com.clickzetta.platform.common.CZException;
import com.clickzetta.platform.util.Pair;
import java.util.*;
public class CZClientBuilder implements ClientBuilder {
protected List> crlAddrs = new ArrayList<>();
protected List> workerAddrs = new ArrayList<>();
protected long instanceId = 1L;
protected String workspace = "default";
protected String streamUrl;
protected Properties properties = new Properties();
protected CZClientBuilder() {}
public static ClientBuilder getBuilder() {
return new CZClientBuilder();
}
@Override
public ClientBuilder crlAddr(String host, Integer port) {
addOrIgnoreAddr(crlAddrs, host, port);
return this;
}
@Override
public ClientBuilder workerAddr(String host, Integer port) {
addOrIgnoreAddr(workerAddrs, host, port);
return this;
}
@Override
public ClientBuilder instanceId(long instanceId) {
this.properties.put("instanceId", instanceId);
this.instanceId = instanceId;
return this;
}
@Override
public ClientBuilder workspace(String workspace) {
this.workspace = workspace;
return this;
}
@Override
public ClientBuilder authenticate(boolean authentication) {
this.properties.put("authentication", authentication);
return this;
}
@Override
public ClientBuilder streamUrl(String streamUrl) {
this.streamUrl = streamUrl;
return this;
}
@Override
public ClientBuilder networkMode(boolean isInternal, boolean direct) {
this.properties.put("isInternal", isInternal);
this.properties.put("isDirect", direct);
return this;
}
@Override
public ClientBuilder showDebugLog(boolean showDebugLog) {
this.properties.put("showDebugLog", showDebugLog);
return this;
}
@Override
public ClientBuilder properties(Properties properties) {
this.properties.putAll(properties);
return this;
}
@Override
public ClientBuilder addProperty(Object key, Object value) {
this.properties.put(key, value);
return this;
}
@Override
public Client build() {
// build igs context.
String clientId = UUID.randomUUID().toString();
CZIgsContext igsContext = null;
if (!crlAddrs.isEmpty()) {
igsContext = CZIgsContext.parser(clientId, crlAddrs, workerAddrs, instanceId, workspace, properties);
} else {
igsContext = CZIgsContext.parser(clientId, streamUrl, workerAddrs, properties);
}
if (igsContext == CZIgsContext.INVALID_CONNECT_STRING) {
throw new CZException("igs invalid connection with crlAddrs or streamUrl.");
}
// build real client.
CZClient client = new CZClient();
client.clientId = igsContext.clientId();
client.clientContext = igsContext;
return client;
}
private void addOrIgnoreAddr(List> list, String host, Integer port) {
Pair pair = new Pair<>(host, port);
if (list != null && !list.contains(pair)) {
list.add(pair);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy