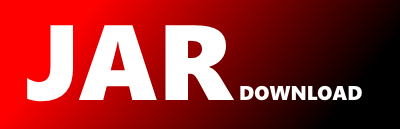
com.clickzetta.platform.client.CZSessionOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickzetta-java Show documentation
Show all versions of clickzetta-java Show documentation
The java SDK for clickzetta's Lakehouse
package com.clickzetta.platform.client;
import com.clickzetta.platform.client.api.*;
import com.clickzetta.platform.common.Constant;
import com.clickzetta.platform.metrics.MetricConf;
import com.google.common.base.Preconditions;
import io.grpc.CallOptions;
import java.io.Serializable;
import java.util.*;
import java.util.stream.Collectors;
public final class CZSessionOptions implements Options, Serializable {
// default conf.
private static final FlushMode FLUSH_MODE = FlushMode.AUTO_FLUSH_BACKGROUND;
private static final long MUTATION_FLUSH_INTERVAL = Constant.MUTATION_FLUSH_INTERVAL;
private static final int MUTATION_BUFFER_SIZE = Constant.MUTATION_BUFFER_SIZE;
private static final int MUTATION_BUFFER_MAX_NUM = Constant.MUTATION_BUFFER_MAX_NUM;
private static final int MUTATION_LINES_NUM = Constant.MUTATION_LINES_NUM;
private static final ErrorTypeHandler DEFAULT_HANDLER = ErrorTypeHandler.TERMINATE_INSTANCE;
private static final boolean REQUEST_FAILED_RETRY_ENABLE = true;
private static final RetryMode REQUEST_FAILED_RETRY_MODE = RetryMode.BATCH_REQUEST_MODE;
private static final int REQUEST_FAILED_RETRY_TIMES = 5;
private static final long REQUEST_FAILED_RETRY_INTERNAL_MS = 5000;
private static final boolean REQUEST_FAILED_RETRY_LOG_DEBUG_ENABLE = true;
private static final RegisterStatus REQUEST_FAILED_RETRY_REGISTER_STATUS = RegisterStatus.DEFAULT;
private static final MetricConf METRIC_DEBUG_CONF = MetricConf.DEFAULT;
private static final CallOptions DEFAULT_CALL_OPTIONS = CallOptions.DEFAULT;
private static final ProtocolType DEFAULT_PROTOCOL_TYPE = ProtocolType.V2;
private static final Properties DEFAULT_PROPERTIES = new Properties();
private FlushMode flushMode;
private int mutationBufferSpace;
private Long flushInterval;
private Integer mutationBufferMaxNum;
private Integer mutationBufferLinesNum;
private ErrorTypeHandler errorTypeHandler;
private boolean requestFailedRetryEnable;
private RetryMode requestFailedRetryMode;
private int requestFailedRetryTimes;
private long requestFailedRetryInternalMs;
private boolean requestFailedRetryLogDebugEnable;
private RegisterStatus registerStatus;
private MetricConf metricConf;
private CallOptions rpcCallOptions;
private ProtocolType protocolType;
private Properties properties;
// default options for cz session.
public static final CZSessionOptions DEFAULT_INSTANCE = new CZSessionOptions();
private CZSessionOptions() {
this(FLUSH_MODE,
MUTATION_FLUSH_INTERVAL,
MUTATION_BUFFER_SIZE,
MUTATION_BUFFER_MAX_NUM,
MUTATION_LINES_NUM,
DEFAULT_HANDLER,
REQUEST_FAILED_RETRY_ENABLE,
REQUEST_FAILED_RETRY_MODE,
REQUEST_FAILED_RETRY_TIMES,
REQUEST_FAILED_RETRY_INTERNAL_MS,
REQUEST_FAILED_RETRY_LOG_DEBUG_ENABLE,
REQUEST_FAILED_RETRY_REGISTER_STATUS,
METRIC_DEBUG_CONF,
DEFAULT_CALL_OPTIONS,
DEFAULT_PROTOCOL_TYPE,
DEFAULT_PROPERTIES);
}
private CZSessionOptions(
FlushMode flushMode,
Long flushInterval,
Integer mutationBufferSpace,
Integer mutationBufferMaxNum,
Integer mutationBufferLinesNum,
ErrorTypeHandler errorTypeHandler,
Boolean requestFailedRetryEnable,
RetryMode requestFailedRetryMode,
Integer requestFailedRetryTimes,
Long requestFailedRetryInternalMs,
Boolean requestFailedRetryLogDebugEnable,
RegisterStatus registerStatus,
MetricConf metricConf,
CallOptions rpcCallOptions,
ProtocolType protocolType,
Properties properties) {
this.flushMode = flushMode != null ? flushMode : FLUSH_MODE;
this.flushInterval = flushInterval != null ? flushInterval : MUTATION_FLUSH_INTERVAL;
this.mutationBufferSpace = mutationBufferSpace != null ? mutationBufferSpace : MUTATION_BUFFER_SIZE;
this.mutationBufferMaxNum = mutationBufferMaxNum != null ? mutationBufferMaxNum : MUTATION_BUFFER_MAX_NUM;
this.mutationBufferLinesNum = mutationBufferLinesNum != null ? mutationBufferLinesNum : MUTATION_LINES_NUM;
this.errorTypeHandler = errorTypeHandler != null ? errorTypeHandler : DEFAULT_HANDLER;
this.requestFailedRetryEnable = requestFailedRetryEnable != null ? requestFailedRetryEnable : REQUEST_FAILED_RETRY_ENABLE;
this.requestFailedRetryMode = requestFailedRetryMode != null ? requestFailedRetryMode : REQUEST_FAILED_RETRY_MODE;
this.requestFailedRetryTimes = requestFailedRetryTimes != null ? requestFailedRetryTimes : REQUEST_FAILED_RETRY_TIMES;
this.requestFailedRetryInternalMs = requestFailedRetryInternalMs != null ? requestFailedRetryInternalMs : REQUEST_FAILED_RETRY_INTERNAL_MS;
this.requestFailedRetryLogDebugEnable = requestFailedRetryLogDebugEnable != null ? requestFailedRetryLogDebugEnable : REQUEST_FAILED_RETRY_LOG_DEBUG_ENABLE;
this.registerStatus = registerStatus != null ? registerStatus : REQUEST_FAILED_RETRY_REGISTER_STATUS;
this.metricConf = metricConf != null ? metricConf : METRIC_DEBUG_CONF;
this.rpcCallOptions = rpcCallOptions != null ? rpcCallOptions : DEFAULT_CALL_OPTIONS;
this.protocolType = protocolType != null ? protocolType : DEFAULT_PROTOCOL_TYPE;
this.properties = properties != null ? properties : DEFAULT_PROPERTIES;
}
@Override
public FlushMode getFlushMode() {
return flushMode;
}
@Override
public Long getFlushInterval() {
return flushInterval;
}
@Override
public int getMutationBufferSpace() {
return mutationBufferSpace;
}
@Override
public Integer getMutationBufferMaxNum() {
return mutationBufferMaxNum;
}
@Override
public Integer getMutationBufferLinesNum() {
return mutationBufferLinesNum;
}
@Override
public ErrorTypeHandler getErrorTypeHandler() {
return errorTypeHandler;
}
@Override
public boolean getRequestFailedRetryEnable() {
return requestFailedRetryEnable;
}
@Override
public RetryMode getRequestFailedRetryMode() {
return requestFailedRetryMode;
}
@Override
public int getRequestFailedRetryTimes() {
return requestFailedRetryTimes;
}
@Override
public long getRequestFailedRetryInternalMs() {
return requestFailedRetryInternalMs;
}
@Override
public boolean getRequestFailedRetryLogDebugEnable() {
return requestFailedRetryLogDebugEnable;
}
@Override
public RegisterStatus getRequestFailedRetryStatus() {
return registerStatus;
}
@Override
public MetricConf getMetricDebugConf() {
return metricConf;
}
@Override
public CallOptions getRpcCallOptions() {
return rpcCallOptions;
}
@Override
public ProtocolType getProtocolType() {
return protocolType;
}
@Override
public Properties getProperties() {
return properties;
}
public static Builder builder() {
return new CZSessionOptionsBuilder();
}
@Override
public Builder toBuilder() {
Builder builder = Options.builder()
.withFlushMode(flushMode)
.withFlushInterval(flushInterval)
.withMutationBufferSpace(mutationBufferSpace)
.withMutationBufferMaxNum(mutationBufferMaxNum)
.withMutationBufferLinesNum(mutationBufferLinesNum)
.withErrorTypeHandler(errorTypeHandler)
.withRequestFailedRetryEnable(requestFailedRetryEnable)
.withRequestFailedRetryMode(requestFailedRetryMode)
.withRequestFailedRetryTimes(requestFailedRetryTimes)
.withRequestFailedRetryInternalMs(requestFailedRetryInternalMs)
.withRequestFailedRetryLogDebugEnable(requestFailedRetryLogDebugEnable)
.withMetricDebugConf(metricConf)
.withRpcCallOptions(rpcCallOptions)
.withProtocolType(protocolType)
.withProperties(properties);
if (registerStatus != null) {
Set set = new HashSet<>();
set.addAll(registerStatus.getCodeList().stream().map(Enum::name).collect(Collectors.toSet()));
set.addAll(registerStatus.getV2CodeList().stream().map(Enum::name).collect(Collectors.toSet()));
List list = set.stream().map(RegisterStatus.RetryStatus::valueOf).collect(Collectors.toList());
for (RegisterStatus.RetryStatus status : list) {
builder.withRequestFailedRetryStatus(status);
}
}
return builder;
}
@Override
public String toString() {
return "CZSessionOptions{" +
"flushMode=" + flushMode +
", mutationBufferSpace=" + mutationBufferSpace +
", flushInterval=" + flushInterval +
", mutationBufferMaxNum=" + mutationBufferMaxNum +
", mutationBufferLinesNum=" + mutationBufferLinesNum +
", errorTypeHandler=" + errorTypeHandler +
", requestFailedRetryEnable=" + requestFailedRetryEnable +
", requestFailedRetryMode=" + requestFailedRetryMode +
", requestFailedRetryTimes=" + requestFailedRetryTimes +
", requestFailedRetryInternalMs=" + requestFailedRetryInternalMs +
", requestFailedRetryLogDebugEnable=" + requestFailedRetryLogDebugEnable +
", registerStatus=" + registerStatus +
", metricConf=" + metricConf +
", rpcCallOptions=" + rpcCallOptions +
", protocolType=" + protocolType +
", properties=" + properties +
'}';
}
static class CZSessionOptionsBuilder implements Builder {
private FlushMode flushMode;
private Long flushInterval;
private Integer mutationBufferSpace;
private Integer mutationBufferMaxNum;
private Integer mutationBufferLinesNum;
private ErrorTypeHandler errorTypeHandler;
// failed request retry enabled.
private Boolean requestFailedRetryEnable;
private RetryMode requestFailedRetryMode;
private Integer requestFailedRetryTimes;
private Long requestFailedRetryInternalMs;
private boolean requestFailedRetryLogDebugEnable = true;
private volatile RegisterStatus registerStatus;
// metrics debug conf.
private MetricConf metricConf;
// grpc connection call options
private CallOptions rpcCallOptions;
// data transform protocol
private ProtocolType protocolType;
private Properties properties;
private CZSessionOptionsBuilder() {
}
@Override
public Builder withFlushMode(FlushMode flushMode) {
this.flushMode = flushMode;
return this;
}
@Override
public Builder withFlushInterval(long flushInterval) {
this.flushInterval = flushInterval;
return this;
}
@Override
public Builder withMutationBufferSpace(int mutationBufferSpace) {
this.mutationBufferSpace = mutationBufferSpace;
return this;
}
@Override
public Builder withMutationBufferMaxNum(int mutationBufferMaxNum) {
this.mutationBufferMaxNum = mutationBufferMaxNum;
return this;
}
@Override
public Builder withMutationBufferLinesNum(int mutationBufferLinesNum) {
this.mutationBufferLinesNum = mutationBufferLinesNum;
return this;
}
@Override
public Builder withErrorTypeHandler(ErrorTypeHandler handler) {
this.errorTypeHandler = handler;
return this;
}
@Override
public Builder withRequestFailedRetryEnable(boolean enabled) {
this.requestFailedRetryEnable = enabled;
return this;
}
@Override
public Builder withRequestFailedRetryMode(RetryMode retryMode) {
this.requestFailedRetryMode = retryMode;
return this;
}
@Override
public Builder withRequestFailedRetryTimes(int retryMaxTimes) {
this.requestFailedRetryTimes = retryMaxTimes;
return this;
}
@Override
public Builder withRequestFailedRetryInternalMs(long retryInternalMs) {
this.requestFailedRetryInternalMs = retryInternalMs;
return this;
}
@Override
public Builder withRequestFailedRetryLogDebugEnable(boolean logDebugEnable) {
this.requestFailedRetryLogDebugEnable = logDebugEnable;
return this;
}
@Override
public Builder withRequestFailedRetryStatus(RegisterStatus.RetryStatus... retryStatus) {
if (registerStatus == null) {
registerStatus = new RegisterStatus();
}
if (retryStatus != null) {
Arrays.stream(retryStatus).forEach(status -> registerStatus.registerCode(status));
}
return this;
}
@Override
public Builder withMetricDebugConf(MetricConf metricConf) {
this.metricConf = metricConf;
return this;
}
@Override
public Builder withRpcCallOptions(CallOptions callOptions) {
this.rpcCallOptions = callOptions;
return this;
}
@Override
public Builder withProtocolType(ProtocolType protocolType) {
this.protocolType = protocolType;
return this;
}
@Override
public Builder withProperties(Properties properties) {
if (this.properties == null) {
this.properties = new Properties();
}
if (properties != null) {
this.properties.putAll(properties);
}
return this;
}
@Override
public Options build() {
if (requestFailedRetryEnable != null && requestFailedRetryEnable) {
Preconditions.checkArgument(
requestFailedRetryTimes > 0,
"request failed retry times more than 0 when request failed retry enable.");
if (requestFailedRetryInternalMs != null) {
Preconditions.checkArgument(
requestFailedRetryInternalMs > 0,
"request retry internal ms more than 0 when request failed retry enable.");
}
}
Preconditions.checkArgument(flushInterval == null || flushInterval >= 0,
"flushInterval be default value null or must >= 0");
return new CZSessionOptions(
flushMode,
flushInterval,
mutationBufferSpace,
mutationBufferMaxNum,
mutationBufferLinesNum,
errorTypeHandler,
requestFailedRetryEnable,
requestFailedRetryMode,
requestFailedRetryTimes,
requestFailedRetryInternalMs,
requestFailedRetryLogDebugEnable,
registerStatus,
metricConf,
rpcCallOptions,
protocolType,
properties
);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy