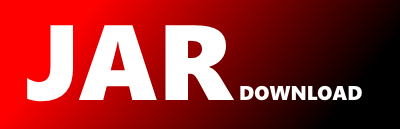
com.clickzetta.platform.client.PartitionSchema Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickzetta-java Show documentation
Show all versions of clickzetta-java Show documentation
The java SDK for clickzetta's Lakehouse
package com.clickzetta.platform.client;
import com.clickzetta.platform.common.Schema;
import com.clickzetta.platform.operator.KeyEncoder;
import com.clickzetta.platform.operator.PartialRow;
import java.util.List;
public class PartitionSchema {
private final RangeSchema rangeSchema;
private final List hashBucketSchemas;
private final boolean isSimple;
public PartitionSchema(RangeSchema rangeSchema,
List hashBucketSchemas,
Schema schema) {
this.rangeSchema = rangeSchema;
this.hashBucketSchemas = hashBucketSchemas;
boolean isSimple = hashBucketSchemas.isEmpty() &&
rangeSchema.columns.size() == schema.getPrimaryKeyColumnCount();
if (isSimple) {
int i = 0;
for (Integer id : rangeSchema.columns) {
if (schema.getColumnIndex(id) != i++) {
isSimple = false;
break;
}
}
}
this.isSimple = isSimple;
}
public byte[] encodePartitionKey(PartialRow row) {
return KeyEncoder.encodePartitionKey(row, this);
}
public RangeSchema getRangeSchema() {
return rangeSchema;
}
public List getHashBucketSchemas() {
return hashBucketSchemas;
}
boolean isSimpleRangePartitioning() {
return isSimple;
}
public static class RangeSchema {
private final List columns;
public RangeSchema(List columns) {
this.columns = columns;
}
public List getColumns() {
return columns;
}
public List getColumnIds() {
return columns;
}
}
public static class HashBucketSchema {
private final List columnIds;
private int numBuckets;
private int seed;
public HashBucketSchema(List columnIds, int numBuckets, int seed) {
this.columnIds = columnIds;
this.numBuckets = numBuckets;
this.seed = seed;
}
public List getColumnIds() {
return columnIds;
}
public int getNumBuckets() {
return numBuckets;
}
public int getSeed() {
return seed;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy