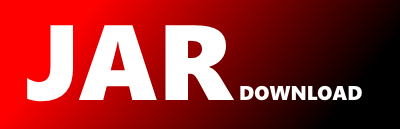
com.clickzetta.platform.client.api.BulkLoadStream Maven / Gradle / Ivy
Show all versions of clickzetta-java Show documentation
package com.clickzetta.platform.client.api;
import com.clickzetta.platform.client.Table;
import com.clickzetta.platform.common.Schema;
import java.io.Closeable;
import java.io.IOException;
import java.util.List;
import java.util.Optional;
/**
* A two-step stream to bulk load data into the lakehouse.
*
* 1) Load data to a staging area via a BulkLoadWriter. Data in the staging area
* is not yet visible to the end user.
* 2) Commits the BulkLoadStream by issuing a COPY job to transfer data from
* the staging area to the target table. Data is visible to the end user once
* the COPY job is finished.
*/
public interface BulkLoadStream extends Closeable {
// Getters
/**
* Return the stream id of the current BulkLoadStream.
*/
String getStreamId();
/**
* Operation of the current BulkLoadStream.
*
* Supported values: APPEND, UPSERT, OVERWRITE.
*/
BulkLoadOperation getOperation();
/**
* Return the precise state and may involve RPC call.
*/
BulkLoadState getStreamState() throws IOException;
/**
* Return the sql error message.
*/
String getSqlErrorMsg();
/**
* Get table schema. Virtual or hidden columns are excluded.
*/
Schema getSchema();
/**
* Get table meta data.
*/
Table getTable();
/**
* Get partition specs when the BulkLoadStream is created.
*/
Optional getPartitionSpecs();
/**
* Get record keys when the BulkLoadStream is created.
*/
List getRecordKeys();
// Write
/**
* Create a BulkLoadWriter with an unique partition id.
*/
BulkLoadWriter openWriter(int partitionId) throws IOException;
// Finish
/**
* Commit the stream with a set of options.
*/
void commit(BulkLoadCommitOptions options) throws IOException;
/**
* Cancel the BulkLoadStream.
*
* The behavior of cancel depends on the BulkLoadState:
* - CREATED: follow-up write or commit is no longer allowed.
* - SEALED: follow-up write or commit is no longer allowed.
* - COMMIT_SUBMITTED: try to cancel the submitted SQL job but not guaranteed.
* - COMMIT_SUCCESS: no-op
* - COMMIT_FAILED: no-op
* - CANCELLED: no-op
*/
void cancel() throws IOException;
}