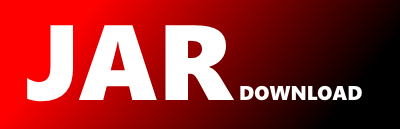
com.clickzetta.platform.client.api.CZStream Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickzetta-java Show documentation
Show all versions of clickzetta-java Show documentation
The java SDK for clickzetta's Lakehouse
package com.clickzetta.platform.client.api;
import com.clickzetta.platform.client.*;
import com.clickzetta.platform.client.pool.AbstractRowPoolSupport;
import com.clickzetta.platform.client.schemachange.EvolutionManager;
import com.clickzetta.platform.client.schemachange.NoopEvolutionManager;
import com.clickzetta.platform.metrics.MetricBase;
import com.clickzetta.platform.operator.WriteOperation;
import java.io.IOException;
public final class CZStream extends AbstractRowPoolSupport implements Stream {
Table table;
CZClient client;
CZSession czSession;
public CZStream(CZClient client, Table table, Options options) {
this.client = client;
this.table = table;
this.czSession = CZSession.build(client, this, options);
// row pool support.
initRowPool(options);
}
@Override
public Row createInsertRow() {
return getOrCreateRow(WriteOperation.ChangeType.INSERT);
}
@Override
public Row createInsertIgnoreRow() {
return getOrCreateRow(WriteOperation.ChangeType.INSERT_IGNORE);
}
@Override
public Row createUpdateRow() {
return getOrCreateRow(WriteOperation.ChangeType.UPDATE);
}
@Override
public Row createUpsertRow() {
return getOrCreateRow(WriteOperation.ChangeType.UPSERT);
}
@Override
public Row createDeleteRow() {
return getOrCreateRow(WriteOperation.ChangeType.DELETE);
}
@Override
public Row createDeleteIgnoreRow() {
return getOrCreateRow(WriteOperation.ChangeType.DELETE_IGNORE);
}
@Override
protected Row buildEmptyRow() {
WriteOperation operation = KuduRow.buildReuseRow();
return operation.getRow();
}
private Row getOrCreateRow(WriteOperation.ChangeType changeType) {
WriteOperation operation = null;
if (poolSupport()) {
operation = getRowInPool().getWriteOperation();
operation.resetRowMeta(table, changeType);
} else {
operation = new KuduRow(table, changeType);
}
return operation.getRow();
}
@Override
public ProtocolType getProtocolType() {
return ProtocolType.V1;
}
@Override
public Table getTable() {
return this.table;
}
@Override
public EvolutionManager getEvolutionManager() {
return NoopEvolutionManager.NOOP_EVOLUTION_INSTANCE;
}
public CZSession getSession() {
return czSession;
}
@Override
public void bindListener(Listener listener) {
this.czSession.bindListener(listener);
}
@Override
public void apply(Row... rows) throws IOException {
this.czSession.apply(rows);
}
@Override
public void flush() throws IOException {
this.czSession.flush();
}
@Override
public MetricBase getMetrics() {
return this.czSession.getMetrics();
}
@Override
public void close() throws IOException {
if (this.czSession != null) {
this.czSession.close();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy