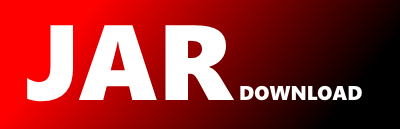
com.clickzetta.platform.client.api.Client Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickzetta-java Show documentation
Show all versions of clickzetta-java Show documentation
The java SDK for clickzetta's Lakehouse
package com.clickzetta.platform.client.api;
import com.clickzetta.platform.bulkload.BulkLoadMetaData;
import com.clickzetta.platform.client.CZClientBuilder;
import com.clickzetta.platform.client.CloseableInternal;
import com.clickzetta.platform.client.api.multi.MultiStream;
import com.clickzetta.platform.client.api.multi.MultiTablesOptions;
import java.io.IOException;
public interface Client extends CloseableInternal {
/**
* static method to get Client Builder.
*
* @return
*/
static ClientBuilder getBuilder() {
return CZClientBuilder.getBuilder();
}
/**
* get igs client connect context info.
*
* @return
*/
ClientContext getClientContext();
/**
* create io stream to target table.
*
* @param schemaName
* @param tableName
* @return
*/
default Stream createStream(String schemaName, String tableName) throws IOException {
return createStream(schemaName, tableName, null, Options.DEFAULT);
}
/**
* create io stream to target table with target tabletNum.
*
* @param schemaName
* @param tableName
* @return
*/
default Stream createStream(String schemaName, String tableName, Integer tabletNum) throws IOException {
return createStream(schemaName, tableName, tabletNum, Options.DEFAULT);
}
/**
* create io stream to target table with target options.
*
* @param schemaName
* @param tableName
* @return
*/
default Stream createStream(String schemaName, String tableName, Options options) throws IOException {
return createStream(schemaName, tableName, null, options);
}
/**
* create io stream to target table with all parameters.
*
* @param schemaName
* @param tableName
* @param options
* @return
*/
Stream createStream(String schemaName, String tableName, Integer tabletNum, Options options) throws IOException;
/**
* create io stream for multi tables. with target options.
*
* @param multiTablesOptions
* @param options
* @return
* @throws IOException
*/
MultiStream createMultiStream(MultiTablesOptions multiTablesOptions, Options options) throws IOException;
/**
* append single stream to target multiStream.
*
* @param multiStream
* @param schemaName
* @param tableName
* @return
*/
MultiStream appendStream(MultiStream multiStream, String schemaName, String tableName) throws IOException;
/**
* append a batch of streams to target multiStream.
*
* @param multiStream
* @param newTableOptions
* @return
*/
MultiStream batchAppendStream(MultiStream multiStream, MultiTablesOptions newTableOptions) throws IOException;
/**
* Create a new BulkLoadStream.
*
* @param schemaName
* @param tableName
* @param options
* @return
*/
BulkLoadStream createBulkLoadStream(String schemaName, String tableName, BulkLoadOptions options) throws IOException;
/**
* Get a BulkLoadStream to check status or write data.
*
* @param schemaName
* @param tableName
* @param streamId
* @return
*/
BulkLoadStream getBulkLoadStream(String schemaName, String tableName, String streamId) throws IOException;
/**
* Get a BulkLoadStream's metadata
*
* @param schemaName
* @param tableName
* @param streamId
* @return
* @throws IOException
*/
BulkLoadMetaData getBulkLoadStreamMetaData(String schemaName, String tableName, String streamId) throws IOException;
/**
* close this client.
*/
@Override
void close(long wait_time_ms) throws IOException;
/**
* release all server resource in current stream.
*/
void releaseResource(Stream stream) throws IOException;
/**
* release all server resource with target schema & table.
*
* only used by multiStream.
*/
default void releaseResource(MultiStream multiStream, String schemaName, String tableName) throws IOException {
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy