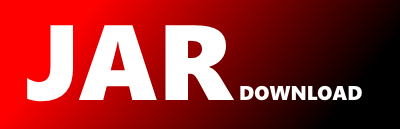
com.clickzetta.platform.client.pool.AbstractRowPoolSupport Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickzetta-java Show documentation
Show all versions of clickzetta-java Show documentation
The java SDK for clickzetta's Lakehouse
package com.clickzetta.platform.client.pool;
import com.clickzetta.platform.client.api.Options;
import com.clickzetta.platform.client.api.Row;
import com.clickzetta.platform.common.Constant;
import org.apache.commons.lang3.StringUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* not thread-safe. not support concurrent operation.
*/
public abstract class AbstractRowPoolSupport implements RowPoolSupport {
protected final Logger LOG = LoggerFactory.getLogger(this.getClass());
private RowPool rowPool;
private boolean poolSupport;
@Override
public void initRowPool(Options options) {
// row pool support.
{
Object obj = options.getProperties().getOrDefault(Constant.ROW_POOL_SUPPORT, false);
this.poolSupport = obj instanceof String ? Boolean.parseBoolean((String) obj) : (boolean) obj;
}
if (this.poolSupport) {
Object obj = options.getProperties().getOrDefault(Constant.ROW_POOL_MAX_SIZE, 20000);
int configPoolSize = obj instanceof String ? Integer.parseInt((String) obj) : (int) obj;
int poolSize = Math.min(configPoolSize, options.getMutationBufferLinesNum() * options.getMutationBufferMaxNum() + 1);
RowPool.Type type = RowPool.Type.valueOf(StringUtils.upperCase(
options.getProperties().getProperty(Constant.ROW_POOL_TYPE, "array")));
this.rowPool = RowPool.Builder.build(type, poolSize);
this.rowPool.initLoader(this::buildEmptyRow);
LOG.info("init row pool with type {} poolSize {} success.", type, poolSize);
}
}
@Override
public boolean poolSupport() {
return this.poolSupport;
}
@Override
public Row getRowInPool() {
Row row = rowPool.acquireRow();
if (row == null) {
row = buildEmptyRow();
row.getWriteOperation().reset();
}
return row;
}
@Override
public void returnRow(Row... rows) {
for (Row row : rows) {
rowPool.releaseRow(row);
}
}
/**
* build empty row & will lazy put to row pool after row used.
*
* @return
*/
protected abstract Row buildEmptyRow();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy