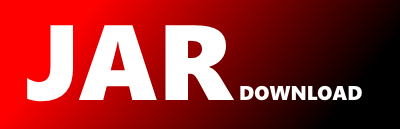
com.clickzetta.platform.client.pool.RowArrayPool Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickzetta-java Show documentation
Show all versions of clickzetta-java Show documentation
The java SDK for clickzetta's Lakehouse
package com.clickzetta.platform.client.pool;
import com.clickzetta.platform.client.api.Row;
import java.util.concurrent.ArrayBlockingQueue;
import java.util.concurrent.BlockingQueue;
public class RowArrayPool implements RowPool {
private int currentSize;
private int poolSize;
private BlockingQueue queue;
private Loader loader;
public RowArrayPool(int poolSize) {
this.poolSize = poolSize;
this.queue = new ArrayBlockingQueue<>(poolSize);
}
@Override
public void initLoader(Loader loader) {
this.loader = loader;
}
@Override
public Row acquireRow() {
if (this.queue.isEmpty() && currentSize < poolSize) {
currentSize++;
Row row = this.loader.load();
row.getWriteOperation().setPoolStatus();
return row;
} else {
return this.queue.poll();
}
}
@Override
public void releaseRow(Row row) {
if (row.getWriteOperation().getPoolStatus()) {
this.queue.offer(row);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy