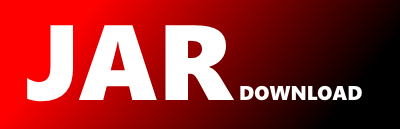
com.clickzetta.platform.client.proxy.AbstractRpcProxy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickzetta-java Show documentation
Show all versions of clickzetta-java Show documentation
The java SDK for clickzetta's Lakehouse
package com.clickzetta.platform.client.proxy;
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.JSONObject;
import com.clickzetta.platform.client.CZIgsContext;
import com.clickzetta.platform.client.api.ClientContext;
import com.clickzetta.platform.util.HttpClient;
import com.clickzetta.platform.util.StringUtil;
import com.google.common.cache.CacheBuilder;
import com.google.common.cache.CacheLoader;
import org.apache.commons.lang3.StringUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.IOException;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.concurrent.TimeUnit;
public abstract class AbstractRpcProxy implements RpcProxy {
protected Logger LOG = LoggerFactory.getLogger(getClass().getSimpleName());
// base target url to get token.
private static final String tokenTargetUrl = "/clickzetta-portal/user/loginSingle";
// base target url to parser region host.
private static final String regionTargetUrl = "/clickzetta-hornhub/hornhub/service/getInsideUrl";
protected final boolean localTest;
protected final ClientContext context;
protected final int httpTimeout = 120; // second;
protected HttpClient httpClient;
public AbstractRpcProxy(ClientContext context) {
this.context = context;
this.localTest = ((CZIgsContext) context).localTest();
}
@Override
public ClientContext getClientContext() {
return context;
}
@Override
public void open() {
this.httpClient = new HttpClient(httpTimeout, httpTimeout, context.isShowDebugLog());
Object obj = context.getProperties().getOrDefault("token_expire_time_ms", 2 * 60 * 60 * 1000);
int expireTimeMs = obj instanceof String ? Integer.parseInt((String) obj) : (int) obj;
((CZIgsContext) context).registerTokenCache(
CacheBuilder.newBuilder()
.expireAfterWrite(expireTimeMs, TimeUnit.MILLISECONDS)
.build(new CacheLoader() {
@Override
public String load(String s) throws Exception {
if (StringUtils.equals(s, "gateway-token")) {
return getToken();
} else {
throw new IOException(String.format("not support get token with %s", s));
}
}
})
);
}
public String getRegionHost() throws IOException {
String url = context.url();
String targetUrl = regionTargetUrl + "?instanceName=" + context.instanceName();
String response = httpClient.callRestAPI(url, targetUrl, "GET", null, "");
JSONObject jsonObject = JSON.parseObject(response);
JSONObject dataMap = jsonObject.getJSONObject("data");
if (dataMap == null || dataMap.getString("STREAMING_API") == null || dataMap.getString("STREAMING_API").isEmpty()) {
String errorMsg = String.format("Apply region url fail, url: %s, targetUrl: %s, " +
"response: %s", url, targetUrl, response);
LOG.error(errorMsg);
throw new IOException(errorMsg);
}
LOG.info("get region url success. {}", dataMap.getString("STREAMING_API"));
return dataMap.getString("STREAMING_API");
}
protected String getToken() throws IOException {
Map request = new LinkedHashMap() {{
put("username", context.userName());
put("password", context.password());
put("instanceName", context.instanceName());
}};
String url = context.url();
String targetUrl = tokenTargetUrl;
String response = httpClient.callRestAPI(url, targetUrl, "POST", null, JSON.toJSONString(request));
JSONObject jsonObject = JSON.parseObject(response);
JSONObject dataMap = jsonObject.getJSONObject("data");
if (dataMap == null || dataMap.getString("token") == null || dataMap.getString("token").isEmpty()) {
String errorMsg = String.format("Apply lakehouse token fail, request: %s, " +
"response: %s", StringUtil.replaceSensitiveInfo(JSON.toJSONString(request)), response);
LOG.error(errorMsg);
throw new IOException(errorMsg);
}
return dataMap.getString("token");
}
protected Map buildRequestHeaders() throws IOException {
String token = "mock-token";
if (!localTest) {
token = context.token();
}
String finalToken = token;
Map headers = new HashMap() {{
put("X-ClickZetta-Token", finalToken);
put("instanceName", context.instanceName());
}};
return headers;
}
protected String refineTargetUrl(String target) {
String endPoint = target + context.lakehouseInstance();
if (context.lakehouseInstance().equals(target)) {
endPoint = target;
}
return endPoint;
}
@Override
public void close(long wait_time_ms) throws IOException {
if (httpClient != null) {
httpClient.close();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy