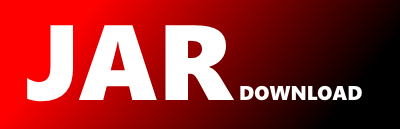
com.clickzetta.platform.client.proxy.GatewayRpcProxy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickzetta-java Show documentation
Show all versions of clickzetta-java Show documentation
The java SDK for clickzetta's Lakehouse
package com.clickzetta.platform.client.proxy;
import com.clickzetta.platform.client.api.ClientContext;
import com.clickzetta.platform.common.Constant;
import com.clickzetta.platform.util.StringUtil;
import com.google.common.util.concurrent.ThreadFactoryBuilder;
import com.google.protobuf.AbstractMessage;
import cz.proto.ingestion.Ingestion;
import java.io.IOException;
import java.util.Map;
import java.util.concurrent.*;
public class GatewayRpcProxy extends AbstractRpcProxy {
private static final String gatewayEndpoint = "/igs/gatewayEndpoint";
private static final String getDummyValidEndpoint = "/igs/dummyValid"; // NOLIMIT
private ScheduledExecutorService scheduledExecutorService;
public GatewayRpcProxy(ClientContext context) {
super(context);
}
@Override
public void open() {
super.open();
this.scheduledExecutorService = Executors.newScheduledThreadPool(4, new ThreadFactoryBuilder()
.setNameFormat("http-timeout-thread-%d")
.setDaemon(true).build());
}
@Override
public
CompletableFuture rpcProxyCallAsync(Ingestion.MethodEnum method, T request) throws IOException {
/**
* new interface wrap for all rest rpc call.
*/
String jsonString = RequestConstructor.toReqString(
Ingestion.GatewayRequest.newBuilder()
.setMethodEnumValue(method.getNumber())
.setMessage(RequestConstructor.toReqString(request))
.setVersionInfo(Ingestion.VersionInfo.newBuilder()
.setName(Constant.VERSION_NAME)
.setVersion(Constant.VERSION_NUMBER).build())
.build());
Map headers = buildRequestHeaders();
CompletableFuture future = new CompletableFuture<>();
CompletableFuture httpFuture = (CompletableFuture)
this.httpClient.callRestAPIAsync(this.context.url(), refineTargetUrl(gatewayEndpoint), "POST", headers, jsonString);
ScheduledFuture> timeoutFuture = scheduledExecutorService.schedule(() -> {
httpFuture.completeExceptionally(new TimeoutException(
String.format("get http response timeout with request \n%s method %s\n.", StringUtil.replaceSensitiveInfo(jsonString), method)));
}, httpTimeout, TimeUnit.SECONDS);
httpFuture.whenCompleteAsync((s, throwable) -> {
try {
if (throwable != null) {
future.completeExceptionally(throwable);
} else {
try {
Ingestion.GatewayResponse gatewayResponse = ResponseConstructor.getResponse(Ingestion.MethodEnum.GATEWAY_RPC_CALL, s);
if (gatewayResponse.hasStatus() && gatewayResponse.getStatus().getCode() != Ingestion.Code.SUCCESS) {
throw new IOException(gatewayResponse.getStatus().getMessage());
}
R response = ResponseConstructor.getResponse(method, gatewayResponse.getMessage());
future.complete(response);
} catch (Throwable t) {
future.completeExceptionally(t);
}
}
} finally {
if (!timeoutFuture.isCancelled() && !timeoutFuture.isDone()) {
timeoutFuture.cancel(true);
}
}
});
return future;
}
@Override
public CompletableFuture dummyValidAsync(String request) throws IOException {
String jsonString = request;
Map headers = buildRequestHeaders();
CompletableFuture future = new CompletableFuture<>();
CompletableFuture httpFuture = (CompletableFuture)
this.httpClient.callRestAPIAsync(this.context.url(), refineTargetUrl(getDummyValidEndpoint), "POST", headers, jsonString);
ScheduledFuture> timeoutFuture = scheduledExecutorService.schedule(() -> {
httpFuture.completeExceptionally(new TimeoutException(
String.format("get http response timeout. %s", request)));
}, httpTimeout, TimeUnit.SECONDS);
httpFuture.whenCompleteAsync((s, throwable) -> {
try {
if (throwable != null) {
future.completeExceptionally(throwable);
} else {
try {
future.complete(s);
} catch (Throwable t) {
future.completeExceptionally(t);
}
}
} finally {
if (!timeoutFuture.isCancelled() && !timeoutFuture.isDone()) {
timeoutFuture.cancel(true);
}
}
});
return future;
}
@Override
public void close(long wait_time_ms) throws IOException {
if (scheduledExecutorService != null) {
scheduledExecutorService.shutdown();
try {
boolean closed = scheduledExecutorService.awaitTermination(wait_time_ms, TimeUnit.MILLISECONDS);
if (!closed) {
scheduledExecutorService.shutdownNow();
}
} catch (InterruptedException ite) {
LOG.warn("ignore executor termination.", ite);
} finally {
scheduledExecutorService = null;
}
}
super.close(wait_time_ms);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy