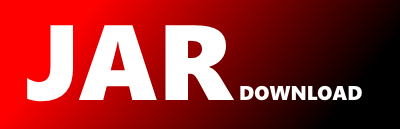
com.clickzetta.platform.client.schemachange.SchemaChange Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickzetta-java Show documentation
Show all versions of clickzetta-java Show documentation
The java SDK for clickzetta's Lakehouse
package com.clickzetta.platform.client.schemachange;
import com.clickzetta.platform.client.Table;
import cz.proto.DataType;
import java.io.Serializable;
import java.util.Arrays;
import java.util.List;
import java.util.Objects;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.Future;
/**
* Schema change to table.
*/
public interface SchemaChange extends Serializable {
Callback DEFAULT_INSTANCE = new DefaultCallback();
/**
* schema change callback.
*/
class DefaultCallback implements SchemaChange.Callback {
@Override
public Future call(String schemaName, String tableName, Table table, List schemaChanges) {
CompletableFuture result = new CompletableFuture<>();
try {
table.applySchemaChange(schemaName, tableName, table, schemaChanges);
result.complete(true);
} catch (Throwable t) {
result.completeExceptionally(t);
}
return result;
}
}
interface Callback {
Future call(String schemaName, String tableName, Table table, List schemaChanges);
}
/**
* schema change operators like (add | rename | drop | update).
*/
static SchemaChange addColumn(String fieldName, DataType dataType) {
return new AddColumn(fieldName, dataType);
}
static SchemaChange renameColumn(String fieldName, String newName) {
return new RenameColumn(fieldName, newName);
}
static SchemaChange dropColumn(String fieldName) {
return new DropColumn(fieldName);
}
static SchemaChange updateColumnType(String fieldName, DataType newDataType) {
return new UpdateColumnType(fieldName, newDataType);
}
static SchemaChange updateColumnNullability(String fieldName, boolean newNullability) {
return new UpdateColumnNullability(new String[]{fieldName}, newNullability);
}
static SchemaChange updateColumnNullability(String[] fieldNames, boolean newNullability) {
return new UpdateColumnNullability(fieldNames, newNullability);
}
static SchemaChange updateColumnPosition(Move move) {
return new UpdateColumnPosition(move);
}
/**
* A SchemaChange to add a field.
*/
final class AddColumn implements SchemaChange {
private static final long serialVersionUID = 1L;
private final String fieldName;
private final DataType dataType;
private AddColumn(String fieldName, DataType dataType) {
this.fieldName = fieldName;
this.dataType = dataType;
}
public String fieldName() {
return fieldName;
}
public DataType dataType() {
return dataType;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
AddColumn addColumn = (AddColumn) o;
return Objects.equals(fieldName, addColumn.fieldName)
&& dataType.equals(addColumn.dataType);
}
@Override
public int hashCode() {
int result = Objects.hash(dataType);
result = 31 * result + Objects.hashCode(fieldName);
return result;
}
@Override
public String toString() {
return "AddColumn{" +
"fieldName='" + fieldName + '\'' +
", dataType=" + dataType +
'}';
}
}
/**
* A SchemaChange to rename a field.
*/
final class RenameColumn implements SchemaChange {
private static final long serialVersionUID = 1L;
private final String fieldName;
private final String newName;
private RenameColumn(String fieldName, String newName) {
this.fieldName = fieldName;
this.newName = newName;
}
public String fieldName() {
return fieldName;
}
public String newName() {
return newName;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
RenameColumn that = (RenameColumn) o;
return Objects.equals(fieldName, that.fieldName)
&& Objects.equals(newName, that.newName);
}
@Override
public int hashCode() {
int result = Objects.hash(newName);
result = 31 * result + Objects.hashCode(fieldName);
return result;
}
@Override
public String toString() {
return "RenameColumn{" +
"fieldName='" + fieldName + '\'' +
", newName='" + newName + '\'' +
'}';
}
}
/**
* A SchemaChange to drop a field.
*/
final class DropColumn implements SchemaChange {
private static final long serialVersionUID = 1L;
private final String fieldName;
private DropColumn(String fieldName) {
this.fieldName = fieldName;
}
public String fieldName() {
return fieldName;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DropColumn that = (DropColumn) o;
return Objects.equals(fieldName, that.fieldName);
}
@Override
public int hashCode() {
return Objects.hashCode(fieldName);
}
@Override
public String toString() {
return "DropColumn{" +
"fieldName='" + fieldName + '\'' +
'}';
}
}
/**
* A SchemaChange to update the field type.
*/
final class UpdateColumnType implements SchemaChange {
private static final long serialVersionUID = 1L;
private final String fieldName;
private final DataType newDataType;
private UpdateColumnType(String fieldName, DataType newDataType) {
this.fieldName = fieldName;
this.newDataType = newDataType;
}
public String fieldName() {
return fieldName;
}
public DataType newDataType() {
return newDataType;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
UpdateColumnType that = (UpdateColumnType) o;
return Objects.equals(fieldName, that.fieldName)
&& newDataType.equals(that.newDataType);
}
@Override
public int hashCode() {
int result = Objects.hash(newDataType);
result = 31 * result + Objects.hashCode(fieldName);
return result;
}
@Override
public String toString() {
return "UpdateColumnType{" +
"fieldName='" + fieldName + '\'' +
", newDataType=" + newDataType +
'}';
}
}
/**
* A SchemaChange to update the field position.
*/
final class UpdateColumnPosition implements SchemaChange {
private static final long serialVersionUID = 1L;
private final Move move;
private UpdateColumnPosition(Move move) {
this.move = move;
}
public Move move() {
return move;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
UpdateColumnPosition updateColumnPosition = (UpdateColumnPosition) o;
return Objects.equals(move, updateColumnPosition.move);
}
@Override
public int hashCode() {
return Objects.hash(move);
}
@Override
public String toString() {
return "UpdateColumnPosition{" +
"move=" + move +
'}';
}
}
/**
* Represents a requested column move in a struct.
*/
class Move implements Serializable {
public enum MoveType {
FIRST,
AFTER
}
public static Move first(String fieldName) {
return new Move(fieldName, null, MoveType.FIRST);
}
public static Move after(String fieldName, String referenceFieldName) {
return new Move(fieldName, referenceFieldName, MoveType.AFTER);
}
private static final long serialVersionUID = 1L;
private final String fieldName;
private final String referenceFieldName;
private final MoveType type;
public Move(String fieldName, String referenceFieldName, MoveType type) {
this.fieldName = fieldName;
this.referenceFieldName = referenceFieldName;
this.type = type;
}
public String fieldName() {
return fieldName;
}
public String referenceFieldName() {
return referenceFieldName;
}
public MoveType type() {
return type;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Move move = (Move) o;
return Objects.equals(fieldName, move.fieldName)
&& Objects.equals(referenceFieldName, move.referenceFieldName)
&& Objects.equals(type, move.type);
}
@Override
public int hashCode() {
return Objects.hash(fieldName, referenceFieldName, type);
}
@Override
public String toString() {
return "Move{" +
"fieldName='" + fieldName + '\'' +
", referenceFieldName='" + referenceFieldName + '\'' +
", type=" + type +
'}';
}
}
/**
* A SchemaChange to update the (nested) field nullability.
*/
final class UpdateColumnNullability implements SchemaChange {
private static final long serialVersionUID = 1L;
private final String[] fieldNames;
private final boolean newNullability;
public UpdateColumnNullability(String[] fieldNames, boolean newNullability) {
this.fieldNames = fieldNames;
this.newNullability = newNullability;
}
public String[] fieldNames() {
return fieldNames;
}
public boolean newNullability() {
return newNullability;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof UpdateColumnNullability)) {
return false;
}
UpdateColumnNullability that = (UpdateColumnNullability) o;
return newNullability == that.newNullability
&& Arrays.equals(fieldNames, that.fieldNames);
}
@Override
public int hashCode() {
int result = Objects.hash(newNullability);
result = 31 * result + Arrays.hashCode(fieldNames);
return result;
}
@Override
public String toString() {
return "UpdateColumnNullability{" +
"fieldNames=" + Arrays.toString(fieldNames) +
", newNullability=" + newNullability +
'}';
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy