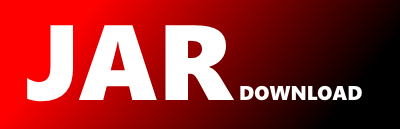
com.clickzetta.platform.common.ColumnSchema Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickzetta-java Show documentation
Show all versions of clickzetta-java Show documentation
The java SDK for clickzetta's Lakehouse
package com.clickzetta.platform.common;
import com.clickzetta.platform.util.CharUtil;
import org.apache.kudu.KuduCommon;
import org.apache.kudu.KuduCommon.EncodingType;
import org.apache.kudu.Compression;
import org.apache.kudu.Compression.CompressionType;
import java.util.Arrays;
import java.util.List;
import java.util.Objects;
public class ColumnSchema {
private final String name;
private final Type type;
private final boolean key;
private final boolean nullable;
private final Object defaultValue;
private final int desiredBlockSize;
private final Encoding encoding;
private final CompressionAlgorithm compressionAlgorithm;
private final ColumnTypeAttributes typeAttributes;
private final int typeSize;
private final KuduCommon.DataType wireType;
private final String comment;
public enum Encoding {
UNKNOWN(EncodingType.UNKNOWN_ENCODING),
AUTO_ENCODING(EncodingType.AUTO_ENCODING),
PLAIN_ENCODING(EncodingType.PLAIN_ENCODING),
PREFIX_ENCODING(EncodingType.PREFIX_ENCODING),
GROUP_VARINT(EncodingType.GROUP_VARINT),
RLE(EncodingType.RLE),
DICT_ENCODING(EncodingType.DICT_ENCODING),
BIT_SHUFFLE(EncodingType.BIT_SHUFFLE);
final EncodingType internalPbType;
Encoding(EncodingType internalPbType) {
this.internalPbType = internalPbType;
}
public EncodingType getInternalPbType() {
return internalPbType;
}
}
public enum CompressionAlgorithm {
UNKNOWN(CompressionType.UNKNOWN_COMPRESSION),
DEFAULT_COMPRESSION(CompressionType.DEFAULT_COMPRESSION),
NO_COMPRESSION(CompressionType.NO_COMPRESSION),
SNAPPY(CompressionType.SNAPPY),
LZ4(CompressionType.LZ4),
ZLIB(CompressionType.ZLIB);
final CompressionType internalPbType;
CompressionAlgorithm(CompressionType internalPbType) {
this.internalPbType = internalPbType;
}
public Compression.CompressionType getInternalPbType() {
return internalPbType;
}
}
private ColumnSchema(String name, Type type, boolean key, boolean nullable,
Object defaultValue, int desiredBlockSize, Encoding encoding,
CompressionAlgorithm compressionAlgorithm,
ColumnTypeAttributes typeAttributes, KuduCommon.DataType wireType,
String comment) {
this.name = name;
this.type = type;
this.key = key;
this.nullable = nullable;
this.defaultValue = defaultValue;
this.desiredBlockSize = desiredBlockSize;
this.encoding = encoding;
this.compressionAlgorithm = compressionAlgorithm;
this.typeAttributes = typeAttributes;
this.typeSize = type.getSize(typeAttributes);
this.wireType = wireType;
this.comment = comment;
}
/**
* Get the column's Type
* @return the type
*/
public Type getType() {
return type;
}
/**
* Get the column's name
* @return A string representation of the name
*/
public String getName() {
return name;
}
/**
* Answers if the column part of the key
* @return true if the column is part of the key, else false
*/
public boolean isKey() {
return key;
}
/**
* Answers if the column can be set to null
* @return true if it can be set to null, else false
*/
public boolean isNullable() {
return nullable;
}
/**
* The Java object representation of the default value that's read
* @return the default read value
*/
public Object getDefaultValue() {
return defaultValue;
}
/**
* Gets the desired block size for this column.
* If no block size has been explicitly specified for this column,
* returns 0 to indicate that the server-side default will be used.
*
* @return the block size, in bytes, or 0 if none has been configured.
*/
public int getDesiredBlockSize() {
return desiredBlockSize;
}
/**
* Return the encoding of this column, or null if it is not known.
*/
public Encoding getEncoding() {
return encoding;
}
/**
* Return the compression algorithm of this column, or null if it is not known.
*/
public CompressionAlgorithm getCompressionAlgorithm() {
return compressionAlgorithm;
}
/**
* Return the column type attributes for the column, or null if it is not known.
*/
public ColumnTypeAttributes getTypeAttributes() {
return typeAttributes;
}
/**
* Get the column's underlying DataType.
*/
public KuduCommon.DataType getWireType() {
return wireType;
}
/**
* The size of this type in bytes on the wire.
* @return A size
*/
public int getTypeSize() {
return typeSize;
}
/**
* Return the comment for the column. An empty string means there is no comment.
*/
public String getComment() {
return comment;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof ColumnSchema)) {
return false;
}
ColumnSchema that = (ColumnSchema) o;
return Objects.equals(name, that.name) &&
Objects.equals(type, that.type) &&
Objects.equals(key, that.key) &&
Objects.equals(typeAttributes, that.typeAttributes) &&
Objects.equals(comment, that.comment);
}
@Override
public int hashCode() {
return Objects.hash(name, type, key, typeAttributes, comment);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("Column name: ");
sb.append(name);
sb.append(", type: ");
sb.append(type.getName());
if (typeAttributes != null) {
sb.append(typeAttributes.toStringForType(type));
}
if (!comment.isEmpty()) {
sb.append(", comment: ");
sb.append(comment);
}
return sb.toString();
}
/**
* Builder for ColumnSchema.
*/
public static class ColumnSchemaBuilder {
private static final List TYPES_WITH_ATTRIBUTES = Arrays.asList(Type.DECIMAL,
Type.VARCHAR);
private final String name;
private final Type type;
private boolean key = false;
private boolean nullable = false;
private Object defaultValue = null;
private int desiredBlockSize = 0;
private Encoding encoding = null;
private CompressionAlgorithm compressionAlgorithm = null;
private ColumnTypeAttributes typeAttributes = null;
private KuduCommon.DataType wireType = null;
private String comment = "";
/**
* Constructor for the required parameters.
* @param name column's name
* @param type column's type
*/
public ColumnSchemaBuilder(String name, Type type) {
this.name = name;
this.type = type;
}
/**
* Constructor to copy an existing columnSchema
* @param that the columnSchema to copy
*/
public ColumnSchemaBuilder(ColumnSchema that) {
this.name = that.name;
this.type = that.type;
this.key = that.key;
this.nullable = that.nullable;
this.defaultValue = that.defaultValue;
this.desiredBlockSize = that.desiredBlockSize;
this.encoding = that.encoding;
this.compressionAlgorithm = that.compressionAlgorithm;
this.typeAttributes = that.typeAttributes;
this.wireType = that.wireType;
this.comment = that.comment;
}
public ColumnSchemaBuilder key(boolean key) {
this.key = key;
return this;
}
public ColumnSchemaBuilder nullable(boolean nullable) {
this.nullable = nullable;
return this;
}
public ColumnSchemaBuilder defaultValue(Object defaultValue) {
this.defaultValue = defaultValue;
return this;
}
public ColumnSchemaBuilder desiredBlockSize(int desiredBlockSize) {
this.desiredBlockSize = desiredBlockSize;
return this;
}
public ColumnSchemaBuilder encoding(Encoding encoding) {
this.encoding = encoding;
return this;
}
public ColumnSchemaBuilder compressionAlgorithm(CompressionAlgorithm compressionAlgorithm) {
this.compressionAlgorithm = compressionAlgorithm;
return this;
}
public ColumnSchemaBuilder typeAttributes(ColumnTypeAttributes typeAttributes) {
if (typeAttributes != null && !TYPES_WITH_ATTRIBUTES.contains(type)) {
throw new IllegalArgumentException(
"ColumnTypeAttributes are not used on " + type + " columns");
}
this.typeAttributes = typeAttributes;
return this;
}
public ColumnSchemaBuilder wireType(KuduCommon.DataType wireType) {
this.wireType = wireType;
return this;
}
/**
* Set the comment for this column.
*/
public ColumnSchemaBuilder comment(String comment) {
this.comment = comment;
return this;
}
public ColumnSchema build() {
if (wireType == null) {
this.wireType = type.getDataType(typeAttributes);
}
if (type == Type.VARCHAR) {
if (typeAttributes == null || !typeAttributes.hasLength() ||
typeAttributes.getLength() < CharUtil.MIN_VARCHAR_LENGTH ||
typeAttributes.getLength() > CharUtil.MAX_VARCHAR_LENGTH) {
throw new IllegalArgumentException(
String.format("VARCHAR's length must be set and between %d and %d",
CharUtil.MIN_VARCHAR_LENGTH, CharUtil.MAX_VARCHAR_LENGTH));
}
}
return new ColumnSchema(name, type,
key, nullable, defaultValue,
desiredBlockSize, encoding, compressionAlgorithm,
typeAttributes, wireType, comment);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy