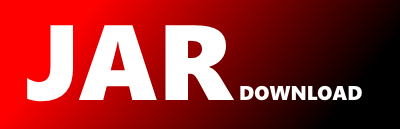
com.clickzetta.platform.example.CZClusterTableTest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickzetta-java Show documentation
Show all versions of clickzetta-java Show documentation
The java SDK for clickzetta's Lakehouse
package com.clickzetta.platform.example;
import com.clickzetta.platform.client.api.Client;
import com.clickzetta.platform.client.api.Options;
import com.clickzetta.platform.client.api.Row;
import com.clickzetta.platform.client.api.Stream;
import com.clickzetta.platform.client.api.FlushMode;
import java.io.*;
import java.nio.charset.StandardCharsets;
import java.nio.file.Files;
import java.nio.file.Paths;
public class CZClusterTableTest {
public static void main(String[] args) throws Exception {
String datafile = args[0];
String coordinator_host = args[1];
String cz_schema_name = args[2];
String cz_table_name = args[3];
Client client = Client.getBuilder()
.authenticate(false)
.crlAddr(coordinator_host, 10086)
.build();
Options options = Options.builder()
.withFlushMode(FlushMode.AUTO_FLUSH_BACKGROUND)
.withFlushInterval(1 * 1000)
.withMutationBufferSpace(20 * 1024 * 1024)
.withMutationBufferMaxNum(3)
.withMutationBufferLinesNum(1000).build();
Stream stream = client.createStream(cz_schema_name, cz_table_name, options);
BufferedReader br = null;
try {
br = new BufferedReader(new InputStreamReader(Files.newInputStream(Paths.get(datafile)), StandardCharsets.UTF_8));
} catch (Exception e) {
throw new RuntimeException(e);
}
String line = null;
try {
while ((line = br.readLine()) != null){
//CREATE TABLE lakehouse_forpd_tpch2g.supplier_cluster_with_sort (S_SUPPKEY int,S_NAME String,S_ADDRESS String,S_CITY String,S_NATION String,S_REGION String,S_PHONE String) clustered by (S_SUPPKEY) sorted by (S_CITY) into 16 buckets ROW FORMAT DELIMITED FIELDS TERMINATED BY '|';
System.out.println(line);
String [] values = line.split("\\|");
Row row = stream.createInsertRow();
row.setValue("s_suppkey", Integer.parseInt(values[0]));
row.setValue("s_name", values[1]);
row.setValue("s_address", values[2]);
row.setValue("s_city", values[3]);
row.setValue("s_nation", values[4]);
row.setValue("s_region", values[5]);
row.setValue("s_phone", values[6]);
stream.apply(row);
}
stream.close();
client.close();
} finally {
if (br != null) {
br.close();
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy