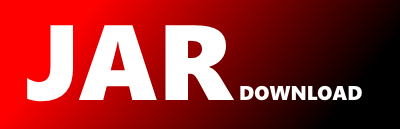
com.clickzetta.platform.example.ClientExample Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickzetta-java Show documentation
Show all versions of clickzetta-java Show documentation
The java SDK for clickzetta's Lakehouse
package com.clickzetta.platform.example;
import com.clickzetta.platform.client.api.*;
import io.grpc.CallOptions;
import io.grpc.Deadline;
import org.apache.commons.lang3.RandomStringUtils;
import org.apache.commons.lang3.StringUtils;
import java.io.IOException;
import java.util.concurrent.TimeUnit;
public class ClientExample {
public static void main(String[] args) throws IOException, InterruptedException {
Client client = Client.getBuilder()
.authenticate(false)
.crlAddr("localhost", 10086)
.workspace("default")
.addProperty("instanceId", 1)
.addProperty("username", "mock-user")
.addProperty("password", "mock-pwd")
.addProperty("localTest", true)
.build();
Options options = Options.builder()
.withFlushMode(FlushMode.AUTO_FLUSH_SYNC)
.withFlushInterval(60 * 1000)
.withMutationBufferSpace(20 * 1024 * 1024)
.withMutationBufferMaxNum(5)
.withMutationBufferLinesNum(10)
.withRpcCallOptions(CallOptions.DEFAULT
.withDeadline(Deadline.after(100, TimeUnit.SECONDS))
.withMaxInboundMessageSize(1000000)
.withMaxOutboundMessageSize(1000000)).build();
Stream stream = client.createStream("lakehouse_mvp", "mvp_igs_05191", 4, options);
long startTime = System.currentTimeMillis();
System.out.println("start to feed data");
for (int t = 0; t < 10000; t++) {
Row row = stream.createInsertRow();
row.setValue("col1", t);
row.setValue("col2", t);
row.setValue("col3", RandomStringUtils.randomAlphanumeric(20));
stream.apply(row);
}
stream.close();
// client.releaseResource(stream);
client.close();
System.out.println("end to feed data cost " + (System.currentTimeMillis() - startTime) + " ms");
Thread.sleep(5 * 1000);
System.out.println("finish success");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy