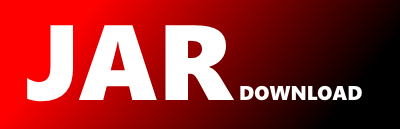
com.clickzetta.platform.flusher.SyncFlusher Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickzetta-java Show documentation
Show all versions of clickzetta-java Show documentation
The java SDK for clickzetta's Lakehouse
package com.clickzetta.platform.flusher;
import com.clickzetta.platform.client.api.Options;
import com.clickzetta.platform.util.Util;
import java.io.IOException;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.TimeUnit;
public class SyncFlusher extends BaseFlusher {
private Thread flushThread;
public SyncFlusher(Options options, Buffer.Type bufferType) {
super(options, bufferType);
}
@Override
public void init() {
LOG.info("SyncFlusher init with nThreads {}", 1);
this.flushThread = new Thread(() -> {
while (!Thread.currentThread().isInterrupted()) {
try {
Task task = flushQueue.poll(200, TimeUnit.MILLISECONDS);
if (task != null) {
try {
CompletableFuture> future;
// no need to do task call when hit exception in preTask.call.
if (getException().get() != null) {
future = task.skipCall(getException().get());
} else {
future = task.call();
}
future.whenComplete((o, t) -> {
if (t != null) {
setException(t);
}
returnBuffer(task.getBuffer());
});
future.get();
} catch (Exception e) {
setException(e);
}
}
} catch (InterruptedException ite) {
Thread.currentThread().interrupt();
}
}
});
this.flushThread.setName("Session-Flush-Thread");
this.flushThread.setDaemon(true);
this.flushThread.start();
super.init();
}
@Override
public void executorTask(Task task) throws IOException {
try {
validCallException();
flushQueue.put(task);
} catch (InterruptedException ite) {
throw new IOException(ite);
}
}
@Override
public void close(boolean wait) throws IOException {
Util.tryWithFinally(() -> {
try {
if (wait) {
synchronized (SyncFlusher.this) {
while (flushQueue.size() != 0) {
validCallException();
wait(200);
}
}
}
} catch (InterruptedException ite) {
throw new IOException(ite);
}
validCallException();
super.close(wait);
}, () -> {
if (this.flushThread != null) {
this.flushThread.interrupt();
}
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy