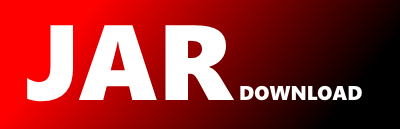
com.clickzetta.platform.metrics.MetricBase Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickzetta-java Show documentation
Show all versions of clickzetta-java Show documentation
The java SDK for clickzetta's Lakehouse
package com.clickzetta.platform.metrics;
import com.codahale.metrics.*;
import com.google.common.base.Preconditions;
import com.google.common.util.concurrent.ThreadFactoryBuilder;
import org.apache.commons.lang3.StringUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.IOException;
import java.util.*;
import java.util.concurrent.Executors;
import java.util.concurrent.ScheduledExecutorService;
import java.util.concurrent.TimeUnit;
public abstract class MetricBase implements MetricSet, AutoCloseable {
private final Logger LOG = LoggerFactory.getLogger(this.getClass().getSimpleName());
private final Map allMetrics;
private final Map namespaceMap;
private final String igsMetricsPrefix;
private ScheduledExecutorService executorService;
public MetricBase(Map namespaceMap) {
Preconditions.checkArgument(namespaceMap != null && namespaceMap.size() != 0,
"namespaceMap can not be null or empty.");
this.allMetrics = new LinkedHashMap<>();
this.namespaceMap = namespaceMap;
List list = new ArrayList<>();
namespaceMap.forEach((key, value) -> list.add(String.format("%s=%s", key, value)));
this.igsMetricsPrefix = StringUtils.join(list, "|");
}
public synchronized T open(MetricConf metricConf) {
if (metricConf != null && metricConf.isEnable()) {
if (executorService == null) {
executorService = Executors.newScheduledThreadPool(1, new ThreadFactoryBuilder()
.setNameFormat("igs-metrics-debugInfo")
.setDaemon(true).build());
executorService.scheduleWithFixedDelay(this::debugMetrics,
1 * 60 * 1000, metricConf.getInternalMs(), TimeUnit.MILLISECONDS);
}
}
return (T) this;
}
private String buildEventName(String eventName, Metric type) {
return String.format(igsMetricsPrefix + "#event.%s.%s",
eventName, type.getClass().getSimpleName().toLowerCase(Locale.ROOT));
}
protected T register(String eventName, Metric metric) {
allMetrics.put(buildEventName(eventName, metric), metric);
return (T) metric;
}
public T buildFromMetricsMap(Map metricMap) {
allMetrics.clear();
allMetrics.putAll(metricMap);
List metrics = new ArrayList<>(metricMap.values());
recoveryFromMetrics(metrics);
return (T) this;
}
protected abstract void recoveryFromMetrics(List metric);
public void debugMetrics() {
try {
if (!allMetrics.isEmpty()) {
LOG.info("Metrics All Details Info: <---------------------------------------------> \n{}",
MetricsInfo.toJsonString(new MetricsInfo(this)));
}
} catch (Throwable t) {
LOG.info("ignore debugMetrics error.", t);
}
}
public Map getNamespaceMap() {
return namespaceMap;
}
@Override
public Map getMetrics() {
return allMetrics;
}
@Override
public synchronized void close() throws IOException {
debugMetrics();
// allMetrics.clear();
if (executorService != null) {
try {
executorService.shutdownNow();
} catch (Throwable t) {
// ignore
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy