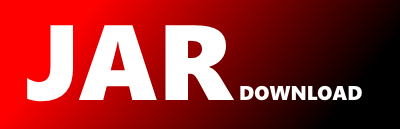
com.clickzetta.platform.operator.AbstractRow Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickzetta-java Show documentation
Show all versions of clickzetta-java Show documentation
The java SDK for clickzetta's Lakehouse
package com.clickzetta.platform.operator;
import com.clickzetta.platform.client.Table;
import com.clickzetta.platform.client.api.Row;
import com.google.common.base.Preconditions;
import cz.proto.ingestion.Ingestion;
import cz.proto.ingestion.v2.IngestionV2;
public abstract class AbstractRow implements WriteOperation, Row {
private WriteOperation.ChangeType changeType;
private Table table;
private PartialRow row;
public AbstractRow(Table table, WriteOperation.ChangeType changeType) {
if (table != null) {
// Table Operation Type Parameters Check First.
Preconditions.checkArgument(table.getIgsTableType() == Ingestion.IGSTableType.ACID ||
(changeType == WriteOperation.ChangeType.INSERT || changeType == WriteOperation.ChangeType.INSERT_IGNORE),
"Common or Cluster Table only support Insert Operation.");
this.table = table;
this.changeType = changeType;
this.row = table.getSchema().newPartialRow();
}
}
@Override
public Table getTable() {
return table;
}
@Override
public int getRowAllocSize() {
int fixedLength = this.row.getRowAlloc().length;
int varLength = this.row.getVarLengthData().stream()
.map(byteBuffer -> byteBuffer != null ? byteBuffer.remaining() : 0)
.reduce(Integer::sum).orElse(0);
return fixedLength + varLength;
}
@Override
public AbstractRow getRow() {
return this;
}
public PartialRow getPartialRow() {
return this.row;
}
public WriteOperation.ChangeType type() {
return this.changeType;
}
@Override
public void setValue(String columnName, Object value) {
row.addObject(columnName, value);
}
@Override
public void setValue(int columnIndex, Object value) {
// change columnIndex to original schema column position.
String columnName = table.getColumnNames().get(columnIndex);
setValue(columnName, value);
}
@Override
public AbstractRow getWriteOperation() {
return this;
}
/**
* row reuse support.
*/
private volatile boolean poolStatus = false;
@Override
public void setPoolStatus() {
poolStatus = true;
}
@Override
public boolean getPoolStatus() {
return poolStatus;
}
@Override
public Row resetRowMeta(Table table, ChangeType changeType) {
Preconditions.checkArgument(table.getIgsTableType() == Ingestion.IGSTableType.ACID ||
(changeType == WriteOperation.ChangeType.INSERT || changeType == WriteOperation.ChangeType.INSERT_IGNORE),
"Common or Cluster Table only support Insert Operation.");
this.changeType = changeType;
if (this.table == null || this.table != table) {
this.table = table;
this.row = table.getSchema().newPartialRow();
} else {
// table not change ignore reset.
this.row.reset();
}
return this;
}
@Override
public Row resetRowMeta(Table table, IngestionV2.OperationType operationType) {
throw new UnsupportedOperationException();
}
@Override
public void reset() {
this.changeType = null;
if (this.row != null) {
this.row.reset();
}
}
@Override
public String toString() {
return "AbstractRow{" +
"changeType=" + changeType +
", table=" + table +
", row=" + row +
'}';
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy