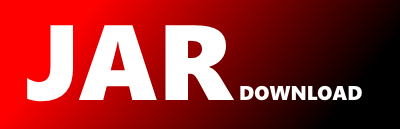
com.clickzetta.platform.tools.LonghaulConf Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickzetta-java Show documentation
Show all versions of clickzetta-java Show documentation
The java SDK for clickzetta's Lakehouse
package com.clickzetta.platform.tools;
import com.clickzetta.platform.client.api.ErrorTypeHandler;
import com.clickzetta.platform.client.api.FlushMode;
import com.clickzetta.platform.util.StringUtil;
import java.util.LinkedHashMap;
import java.util.List;
public class LonghaulConf {
public Long instanceId;
public String workspace;
public String schemaName;
public String tableName;
public Integer tabletNum;
public String crlHost;
public int crlPort;
public String streamUrl;
public LinkedHashMap properties;
public Options options;
public List fieldList;
public String operator;
public String dataGenMode;
public Long dataGenTimeMs;
public Long dataGenInternalMs;
public Long dataGenCount;
public boolean releaseResource;
public LonghaulConf() {}
public LonghaulConf(
Long instanceId,
String workspace,
String schemaName,
String tableName,
Integer tabletNum,
String crlHost,
int crlPort,
String streamUrl,
LinkedHashMap properties,
Options options,
List fieldList,
String operator,
String dataGenMode,
Long dataGenTimeMs,
Long dataGenCount,
boolean releaseResource) {
this.instanceId = instanceId;
this.workspace = workspace;
this.schemaName = schemaName;
this.tableName = tableName;
this.tabletNum = tabletNum;
this.crlHost = crlHost;
this.crlPort = crlPort;
this.streamUrl = streamUrl;
this.properties = properties;
this.options = options;
this.fieldList = fieldList;
this.operator = operator;
this.dataGenMode = dataGenMode;
this.dataGenTimeMs = dataGenTimeMs;
this.dataGenCount = dataGenCount;
this.releaseResource = releaseResource;
}
public Long getInstanceId() {
return instanceId;
}
public void setInstanceId(Long instanceId) {
this.instanceId = instanceId;
}
public String getWorkspace() {
return workspace;
}
public void setWorkspace(String workspace) {
this.workspace = workspace;
}
public String getSchemaName() {
return schemaName;
}
public void setSchemaName(String schemaName) {
this.schemaName = schemaName;
}
public String getTableName() {
return tableName;
}
public void setTableName(String tableName) {
this.tableName = tableName;
}
public Integer getTabletNum() {
return tabletNum;
}
public void setTabletNum(Integer tabletNum) {
this.tabletNum = tabletNum;
}
public String getCrlHost() {
return crlHost;
}
public void setCrlHost(String crlHost) {
this.crlHost = crlHost;
}
public int getCrlPort() {
return crlPort;
}
public void setCrlPort(int crlPort) {
this.crlPort = crlPort;
}
public String getStreamUrl() {
return streamUrl;
}
public void setStreamUrl(String streamUrl) {
this.streamUrl = streamUrl;
}
public LinkedHashMap getProperties() {
return properties;
}
public void setProperties(LinkedHashMap properties) {
this.properties = properties;
}
public Options getOptions() {
return options;
}
public void setOptions(Options options) {
this.options = options;
}
public List getFieldList() {
return fieldList;
}
public void setFieldList(List fieldList) {
this.fieldList = fieldList;
}
public String getOperator() {
return operator;
}
public void setOperator(String operator) {
this.operator = operator;
}
public String getDataGenMode() {
return dataGenMode;
}
public void setDataGenMode(String dataGenMode) {
this.dataGenMode = dataGenMode;
}
public Long getDataGenInternalMs() {
return dataGenInternalMs;
}
public void setDataGenInternalMs(Long dataGenInternalMs) {
this.dataGenInternalMs = dataGenInternalMs;
}
public Long getDataGenTimeMs() {
return dataGenTimeMs;
}
public void setDataGenTimeMs(Long dataGenTimeMs) {
this.dataGenTimeMs = dataGenTimeMs;
}
public Long getDataGenCount() {
return dataGenCount;
}
public void setDataGenCount(Long dataGenCount) {
this.dataGenCount = dataGenCount;
}
public boolean isReleaseResource() {
return releaseResource;
}
public void setReleaseResource(boolean releaseResource) {
this.releaseResource = releaseResource;
}
@Override
public String toString() {
return "LonghaulConf{" +
"instanceId=" + instanceId +
", workspace='" + workspace + '\'' +
", schemaName='" + schemaName + '\'' +
", tableName='" + tableName + '\'' +
", tabletNum=" + tabletNum +
", crlHost='" + crlHost + '\'' +
", crlPort=" + crlPort +
", streamUrl='" + StringUtil.replaceSensitiveInfo(streamUrl) + '\'' +
", properties=" + properties +
", options=" + options +
", fieldList=" + fieldList +
", operator='" + operator + '\'' +
", dataGenMode='" + dataGenMode + '\'' +
", dataGenTimeMs=" + dataGenTimeMs +
", dataGenInternalMs=" + dataGenInternalMs +
", dataGenCount=" + dataGenCount +
", releaseResource=" + releaseResource +
'}';
}
public static class Options {
public FlushMode flushMode;
public Long flushInterval;
public Integer mutationBufferSpace;
public Integer mutationBufferMaxNum;
public Integer mutationBufferLinesNum;
public Boolean requestFailedRetryEnable;
public Integer requestFailedRetryTimes;
public ErrorTypeHandler errorTypeHandler;
public Options() {}
public Options(
FlushMode flushMode,
Long flushInterval,
Integer mutationBufferSpace,
Integer mutationBufferMaxNum,
Integer mutationBufferLinesNum,
Boolean requestFailedRetryEnable,
Integer requestFailedRetryTimes) {
this.flushMode = flushMode;
this.flushInterval = flushInterval;
this.mutationBufferSpace = mutationBufferSpace;
this.mutationBufferMaxNum = mutationBufferMaxNum;
this.mutationBufferLinesNum = mutationBufferLinesNum;
this.requestFailedRetryEnable = requestFailedRetryEnable;
this.requestFailedRetryTimes = requestFailedRetryTimes;
}
public FlushMode getFlushMode() {
return flushMode;
}
public void setFlushMode(FlushMode flushMode) {
this.flushMode = flushMode;
}
public Long getFlushInterval() {
return flushInterval;
}
public void setFlushInterval(Long flushInterval) {
this.flushInterval = flushInterval;
}
public int getMutationBufferSpace() {
return mutationBufferSpace;
}
public void setMutationBufferSpace(int mutationBufferSpace) {
this.mutationBufferSpace = mutationBufferSpace;
}
public Integer getMutationBufferMaxNum() {
return mutationBufferMaxNum;
}
public void setMutationBufferMaxNum(Integer mutationBufferMaxNum) {
this.mutationBufferMaxNum = mutationBufferMaxNum;
}
public Integer getMutationBufferLinesNum() {
return mutationBufferLinesNum;
}
public void setMutationBufferLinesNum(Integer mutationBufferLinesNum) {
this.mutationBufferLinesNum = mutationBufferLinesNum;
}
public boolean isRequestFailedRetryEnable() {
return requestFailedRetryEnable;
}
public void setRequestFailedRetryEnable(boolean requestFailedRetryEnable) {
this.requestFailedRetryEnable = requestFailedRetryEnable;
}
public int getRequestFailedRetryTimes() {
return requestFailedRetryTimes;
}
public void setRequestFailedRetryTimes(int requestFailedRetryTimes) {
this.requestFailedRetryTimes = requestFailedRetryTimes;
}
public ErrorTypeHandler getErrorTypeHandler() {
return errorTypeHandler;
}
public void setErrorTypeHandler(ErrorTypeHandler errorTypeHandler) {
this.errorTypeHandler = errorTypeHandler;
}
public com.clickzetta.platform.client.api.Options toCZSessionOptions() {
com.clickzetta.platform.client.api.Options.Builder builder = com.clickzetta.platform.client.api.Options.builder();
if (flushMode != null) {
builder.withFlushMode(flushMode);
}
if (flushInterval != null) {
builder.withFlushInterval(flushInterval);
}
if (mutationBufferSpace != null) {
builder.withMutationBufferSpace(mutationBufferSpace);
}
if (mutationBufferMaxNum != null) {
builder.withMutationBufferMaxNum(mutationBufferMaxNum);
}
if (mutationBufferLinesNum != null) {
builder.withMutationBufferLinesNum(mutationBufferLinesNum);
}
if (requestFailedRetryEnable != null) {
builder.withRequestFailedRetryEnable(requestFailedRetryEnable);
}
if (requestFailedRetryTimes != null) {
builder.withRequestFailedRetryTimes(requestFailedRetryTimes);
}
if (errorTypeHandler != null) {
builder.withErrorTypeHandler(errorTypeHandler);
}
return builder.build();
}
@Override
public String toString() {
return "Options{" +
"flushMode=" + flushMode +
", flushInterval=" + flushInterval +
", mutationBufferSpace=" + mutationBufferSpace +
", mutationBufferMaxNum=" + mutationBufferMaxNum +
", mutationBufferLinesNum=" + mutationBufferLinesNum +
", requestFailedRetryEnable=" + requestFailedRetryEnable +
", requestFailedRetryTimes=" + requestFailedRetryTimes +
'}';
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy