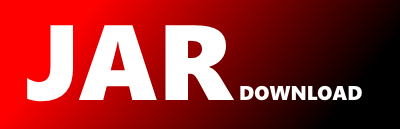
com.clickzetta.platform.util.AsyncUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickzetta-java Show documentation
Show all versions of clickzetta-java Show documentation
The java SDK for clickzetta's Lakehouse
package com.clickzetta.platform.util;
import com.clickzetta.platform.client.CloseableInternal;
import com.stumbleupon.async.Callback;
import com.stumbleupon.async.Deferred;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.IOException;
import java.util.concurrent.ForkJoinPool;
import java.util.concurrent.TimeUnit;
public class AsyncUtil implements CloseableInternal {
private static final Logger LOG = LoggerFactory.getLogger(AsyncUtil.class);
private AsyncUtil() {
}
private static ForkJoinPool customThreadPool;
public static ForkJoinPool getForkJoinPool() {
if (customThreadPool == null) {
synchronized (AsyncUtil.class) {
if (customThreadPool == null) {
customThreadPool = new ForkJoinPool(16);
}
}
}
return customThreadPool;
}
@Override
public void close(long wait_time_ms) throws IOException {
if (customThreadPool != null) {
synchronized (AsyncUtil.class) {
if (customThreadPool != null) {
customThreadPool.shutdown();
try {
boolean closed = customThreadPool.awaitTermination(wait_time_ms, TimeUnit.MILLISECONDS);
if (!closed) {
customThreadPool.shutdownNow();
}
} catch (InterruptedException ite) {
LOG.warn("ignore executor termination.", ite);
} finally {
customThreadPool = null;
}
}
}
}
}
public static , E> Deferred addCallbacksDeferring(final Deferred d,
final Callback cb,
final Callback eb) {
return d.addCallbacks((Callback) cb, eb);
}
public static Deferred addBoth(final Deferred deferred,
final Callback extends U, Object> callback) {
return ((Deferred) deferred).addBoth(callback);
}
public static Deferred addBothDeferring(final Deferred deferred,
final Callback, Object> callback) {
return ((Deferred) deferred).addBothDeferring(callback);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy