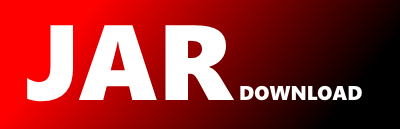
com.clickzetta.platform.util.Util Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickzetta-java Show documentation
Show all versions of clickzetta-java Show documentation
The java SDK for clickzetta's Lakehouse
package com.clickzetta.platform.util;
import org.apache.commons.lang3.StringUtils;
import org.apache.commons.lang3.SystemUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.Closeable;
import java.io.IOException;
import java.net.Inet4Address;
import java.net.InetAddress;
import java.net.InetSocketAddress;
import java.net.NetworkInterface;
import java.util.*;
import java.util.stream.Collectors;
public class Util {
private static final Logger LOG = LoggerFactory.getLogger(Util.class);
public interface InternalRunnable {
void run() throws IOException;
}
public static void tryWithFinally(InternalRunnable run, Closeable closeable) throws IOException {
try {
if (run != null) {
run.run();
}
} catch (Throwable t) {
IOException ioe;
if (t instanceof IOException) {
ioe = (IOException) t;
} else {
ioe = new IOException(t);
}
throw ioe;
} finally {
if (closeable != null) {
closeable.close();
}
}
}
public static String generatorStreamId(String sessionId, String... args) {
String prefix = String.format("%s-%s", sessionId, localIpAddress.getHostAddress());
if (args != null && args.length != 0) {
prefix = String.format("%s-%s", prefix, StringUtils.join(args, "-"));
}
return prefix;
}
/**
* Whether the underlying operating system is Windows.
*/
private static final boolean isWindows = SystemUtils.IS_OS_WINDOWS;
/**
* Get the local host's IP address in dotted-quad format (e.g. 1.2.3.4).
*/
public static InetAddress localIpAddress;
static {
try {
localIpAddress = findLocalInetAddress();
} catch (Throwable e) {
LOG.error("ignore findLocalInetAddress error.", e);
// mock once.
localIpAddress = new InetSocketAddress(10186).getAddress();
}
}
private static InetAddress findLocalInetAddress() throws IOException {
String defaultIpOverride = System.getenv("IGS_LOCAL_IP");
if (defaultIpOverride != null) {
return InetAddress.getByName(defaultIpOverride);
} else {
InetAddress address = InetAddress.getLocalHost();
if (address.isLoopbackAddress()) {
// Address resolves to something like 127.0.1.1, which happens on Debian; try to find
// a better address using the local network interfaces
// getNetworkInterfaces returns ifs in reverse order compared to ifconfig output order
// on unix-like system. On windows, it returns in index order.
// It's more proper to pick ip address following system output order.
Enumeration activeNetworkIFs = NetworkInterface.getNetworkInterfaces();
List reOrderedNetworkIFs = new ArrayList<>();
while (activeNetworkIFs.hasMoreElements()) {
reOrderedNetworkIFs.add(activeNetworkIFs.nextElement());
}
if (!isWindows) {
Collections.reverse(reOrderedNetworkIFs);
}
for (NetworkInterface ni : reOrderedNetworkIFs) {
Enumeration inetAddresses = ni.getInetAddresses();
List tmpList = new ArrayList<>();
while (inetAddresses.hasMoreElements()) {
tmpList.add(inetAddresses.nextElement());
}
List addresses = tmpList.stream()
.filter(addr -> !(addr.isLinkLocalAddress() || addr.isLoopbackAddress())).collect(Collectors.toList());
if (!addresses.isEmpty()) {
InetAddress addr = addresses.stream().filter(
x -> x instanceof Inet4Address).findFirst().orElse(addresses.get(0));
// because of Inet6Address.toHostName may add interface at the end if it knows about it
InetAddress strippedAddress = InetAddress.getByAddress(addr.getAddress());
// We've found an address that looks reasonable!
LOG.info("Your hostname, " + InetAddress.getLocalHost().getHostName() + " resolves to" +
" a loopback address: " + address.getHostAddress() + "; using " +
strippedAddress.getHostAddress() + " instead (on interface " + ni.getName() + ")");
LOG.info("Set IGS_LOCAL_IP if you need to bind to another address");
return strippedAddress;
}
}
LOG.info("Your hostname, " + InetAddress.getLocalHost().getHostName() + " resolves to" +
" a loopback address: " + address.getHostAddress() + ", but we couldn't find any" +
" external IP address!");
LOG.info("Set IGS_LOCAL_IP if you need to bind to another address");
}
return address;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy