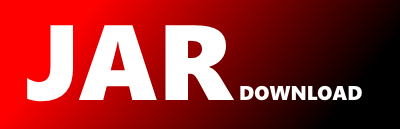
cz.proto.Calc Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickzetta-java Show documentation
Show all versions of clickzetta-java Show documentation
The java SDK for clickzetta's Lakehouse
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: operator.proto
package cz.proto;
/**
* Protobuf type {@code cz.proto.Calc}
*/
public final class Calc extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.Calc)
CalcOrBuilder {
private static final long serialVersionUID = 0L;
// Use Calc.newBuilder() to construct.
private Calc(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Calc() {
expressions_ = java.util.Collections.emptyList();
projects_ = emptyLongList();
lazy_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Calc();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Calc(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
expressions_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
expressions_.add(
input.readMessage(cz.proto.Expression.ScalarExpression.parser(), extensionRegistry));
break;
}
case 16: {
optionalFilter_ = input.readBool();
optionalFilterCase_ = 2;
break;
}
case 24: {
optionalFilter_ = input.readUInt64();
optionalFilterCase_ = 3;
break;
}
case 32: {
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
projects_ = newLongList();
mutable_bitField0_ |= 0x00000002;
}
projects_.addLong(input.readUInt64());
break;
}
case 34: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000002) != 0) && input.getBytesUntilLimit() > 0) {
projects_ = newLongList();
mutable_bitField0_ |= 0x00000002;
}
while (input.getBytesUntilLimit() > 0) {
projects_.addLong(input.readUInt64());
}
input.popLimit(limit);
break;
}
case 40: {
int rawValue = input.readEnum();
if (!((mutable_bitField0_ & 0x00000004) != 0)) {
lazy_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
lazy_.add(rawValue);
break;
}
case 42: {
int length = input.readRawVarint32();
int oldLimit = input.pushLimit(length);
while(input.getBytesUntilLimit() > 0) {
int rawValue = input.readEnum();
if (!((mutable_bitField0_ & 0x00000004) != 0)) {
lazy_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
lazy_.add(rawValue);
}
input.popLimit(oldLimit);
break;
}
case 49: {
bitField0_ |= 0x00000001;
partialWindowFilterSelectivity_ = input.readDouble();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
expressions_ = java.util.Collections.unmodifiableList(expressions_);
}
if (((mutable_bitField0_ & 0x00000002) != 0)) {
projects_.makeImmutable(); // C
}
if (((mutable_bitField0_ & 0x00000004) != 0)) {
lazy_ = java.util.Collections.unmodifiableList(lazy_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.OperatorProto.internal_static_cz_proto_Calc_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.OperatorProto.internal_static_cz_proto_Calc_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.Calc.class, cz.proto.Calc.Builder.class);
}
private int bitField0_;
private int optionalFilterCase_ = 0;
private java.lang.Object optionalFilter_;
public enum OptionalFilterCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
NO_FILTER(2),
FILTER(3),
OPTIONALFILTER_NOT_SET(0);
private final int value;
private OptionalFilterCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static OptionalFilterCase valueOf(int value) {
return forNumber(value);
}
public static OptionalFilterCase forNumber(int value) {
switch (value) {
case 2: return NO_FILTER;
case 3: return FILTER;
case 0: return OPTIONALFILTER_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public OptionalFilterCase
getOptionalFilterCase() {
return OptionalFilterCase.forNumber(
optionalFilterCase_);
}
public static final int EXPRESSIONS_FIELD_NUMBER = 1;
private java.util.List expressions_;
/**
* repeated .cz.proto.ScalarExpression expressions = 1;
*/
@java.lang.Override
public java.util.List getExpressionsList() {
return expressions_;
}
/**
* repeated .cz.proto.ScalarExpression expressions = 1;
*/
@java.lang.Override
public java.util.List extends cz.proto.Expression.ScalarExpressionOrBuilder>
getExpressionsOrBuilderList() {
return expressions_;
}
/**
* repeated .cz.proto.ScalarExpression expressions = 1;
*/
@java.lang.Override
public int getExpressionsCount() {
return expressions_.size();
}
/**
* repeated .cz.proto.ScalarExpression expressions = 1;
*/
@java.lang.Override
public cz.proto.Expression.ScalarExpression getExpressions(int index) {
return expressions_.get(index);
}
/**
* repeated .cz.proto.ScalarExpression expressions = 1;
*/
@java.lang.Override
public cz.proto.Expression.ScalarExpressionOrBuilder getExpressionsOrBuilder(
int index) {
return expressions_.get(index);
}
public static final int NO_FILTER_FIELD_NUMBER = 2;
/**
* bool no_filter = 2;
* @return Whether the noFilter field is set.
*/
@java.lang.Override
public boolean hasNoFilter() {
return optionalFilterCase_ == 2;
}
/**
* bool no_filter = 2;
* @return The noFilter.
*/
@java.lang.Override
public boolean getNoFilter() {
if (optionalFilterCase_ == 2) {
return (java.lang.Boolean) optionalFilter_;
}
return false;
}
public static final int FILTER_FIELD_NUMBER = 3;
/**
* uint64 filter = 3;
* @return Whether the filter field is set.
*/
@java.lang.Override
public boolean hasFilter() {
return optionalFilterCase_ == 3;
}
/**
* uint64 filter = 3;
* @return The filter.
*/
@java.lang.Override
public long getFilter() {
if (optionalFilterCase_ == 3) {
return (java.lang.Long) optionalFilter_;
}
return 0L;
}
public static final int PROJECTS_FIELD_NUMBER = 4;
private com.google.protobuf.Internal.LongList projects_;
/**
* repeated uint64 projects = 4;
* @return A list containing the projects.
*/
@java.lang.Override
public java.util.List
getProjectsList() {
return projects_;
}
/**
* repeated uint64 projects = 4;
* @return The count of projects.
*/
public int getProjectsCount() {
return projects_.size();
}
/**
* repeated uint64 projects = 4;
* @param index The index of the element to return.
* @return The projects at the given index.
*/
public long getProjects(int index) {
return projects_.getLong(index);
}
private int projectsMemoizedSerializedSize = -1;
public static final int LAZY_FIELD_NUMBER = 5;
private java.util.List lazy_;
private static final com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, cz.proto.LazyEval> lazy_converter_ =
new com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, cz.proto.LazyEval>() {
public cz.proto.LazyEval convert(java.lang.Integer from) {
@SuppressWarnings("deprecation")
cz.proto.LazyEval result = cz.proto.LazyEval.valueOf(from);
return result == null ? cz.proto.LazyEval.UNRECOGNIZED : result;
}
};
/**
* repeated .cz.proto.LazyEval lazy = 5;
* @return A list containing the lazy.
*/
@java.lang.Override
public java.util.List getLazyList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, cz.proto.LazyEval>(lazy_, lazy_converter_);
}
/**
* repeated .cz.proto.LazyEval lazy = 5;
* @return The count of lazy.
*/
@java.lang.Override
public int getLazyCount() {
return lazy_.size();
}
/**
* repeated .cz.proto.LazyEval lazy = 5;
* @param index The index of the element to return.
* @return The lazy at the given index.
*/
@java.lang.Override
public cz.proto.LazyEval getLazy(int index) {
return lazy_converter_.convert(lazy_.get(index));
}
/**
* repeated .cz.proto.LazyEval lazy = 5;
* @return A list containing the enum numeric values on the wire for lazy.
*/
@java.lang.Override
public java.util.List
getLazyValueList() {
return lazy_;
}
/**
* repeated .cz.proto.LazyEval lazy = 5;
* @param index The index of the value to return.
* @return The enum numeric value on the wire of lazy at the given index.
*/
@java.lang.Override
public int getLazyValue(int index) {
return lazy_.get(index);
}
private int lazyMemoizedSerializedSize;
public static final int PARTIAL_WINDOW_FILTER_SELECTIVITY_FIELD_NUMBER = 6;
private double partialWindowFilterSelectivity_;
/**
* optional double partial_window_filter_selectivity = 6;
* @return Whether the partialWindowFilterSelectivity field is set.
*/
@java.lang.Override
public boolean hasPartialWindowFilterSelectivity() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional double partial_window_filter_selectivity = 6;
* @return The partialWindowFilterSelectivity.
*/
@java.lang.Override
public double getPartialWindowFilterSelectivity() {
return partialWindowFilterSelectivity_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (int i = 0; i < expressions_.size(); i++) {
output.writeMessage(1, expressions_.get(i));
}
if (optionalFilterCase_ == 2) {
output.writeBool(
2, (boolean)((java.lang.Boolean) optionalFilter_));
}
if (optionalFilterCase_ == 3) {
output.writeUInt64(
3, (long)((java.lang.Long) optionalFilter_));
}
if (getProjectsList().size() > 0) {
output.writeUInt32NoTag(34);
output.writeUInt32NoTag(projectsMemoizedSerializedSize);
}
for (int i = 0; i < projects_.size(); i++) {
output.writeUInt64NoTag(projects_.getLong(i));
}
if (getLazyList().size() > 0) {
output.writeUInt32NoTag(42);
output.writeUInt32NoTag(lazyMemoizedSerializedSize);
}
for (int i = 0; i < lazy_.size(); i++) {
output.writeEnumNoTag(lazy_.get(i));
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeDouble(6, partialWindowFilterSelectivity_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < expressions_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, expressions_.get(i));
}
if (optionalFilterCase_ == 2) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(
2, (boolean)((java.lang.Boolean) optionalFilter_));
}
if (optionalFilterCase_ == 3) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(
3, (long)((java.lang.Long) optionalFilter_));
}
{
int dataSize = 0;
for (int i = 0; i < projects_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeUInt64SizeNoTag(projects_.getLong(i));
}
size += dataSize;
if (!getProjectsList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
projectsMemoizedSerializedSize = dataSize;
}
{
int dataSize = 0;
for (int i = 0; i < lazy_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeEnumSizeNoTag(lazy_.get(i));
}
size += dataSize;
if (!getLazyList().isEmpty()) { size += 1;
size += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(dataSize);
}lazyMemoizedSerializedSize = dataSize;
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(6, partialWindowFilterSelectivity_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.Calc)) {
return super.equals(obj);
}
cz.proto.Calc other = (cz.proto.Calc) obj;
if (!getExpressionsList()
.equals(other.getExpressionsList())) return false;
if (!getProjectsList()
.equals(other.getProjectsList())) return false;
if (!lazy_.equals(other.lazy_)) return false;
if (hasPartialWindowFilterSelectivity() != other.hasPartialWindowFilterSelectivity()) return false;
if (hasPartialWindowFilterSelectivity()) {
if (java.lang.Double.doubleToLongBits(getPartialWindowFilterSelectivity())
!= java.lang.Double.doubleToLongBits(
other.getPartialWindowFilterSelectivity())) return false;
}
if (!getOptionalFilterCase().equals(other.getOptionalFilterCase())) return false;
switch (optionalFilterCase_) {
case 2:
if (getNoFilter()
!= other.getNoFilter()) return false;
break;
case 3:
if (getFilter()
!= other.getFilter()) return false;
break;
case 0:
default:
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getExpressionsCount() > 0) {
hash = (37 * hash) + EXPRESSIONS_FIELD_NUMBER;
hash = (53 * hash) + getExpressionsList().hashCode();
}
if (getProjectsCount() > 0) {
hash = (37 * hash) + PROJECTS_FIELD_NUMBER;
hash = (53 * hash) + getProjectsList().hashCode();
}
if (getLazyCount() > 0) {
hash = (37 * hash) + LAZY_FIELD_NUMBER;
hash = (53 * hash) + lazy_.hashCode();
}
if (hasPartialWindowFilterSelectivity()) {
hash = (37 * hash) + PARTIAL_WINDOW_FILTER_SELECTIVITY_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getPartialWindowFilterSelectivity()));
}
switch (optionalFilterCase_) {
case 2:
hash = (37 * hash) + NO_FILTER_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getNoFilter());
break;
case 3:
hash = (37 * hash) + FILTER_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getFilter());
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.Calc parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Calc parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Calc parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Calc parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Calc parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Calc parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Calc parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.Calc parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.Calc parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.Calc parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.Calc parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.Calc parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.Calc prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.Calc}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.Calc)
cz.proto.CalcOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.OperatorProto.internal_static_cz_proto_Calc_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.OperatorProto.internal_static_cz_proto_Calc_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.Calc.class, cz.proto.Calc.Builder.class);
}
// Construct using cz.proto.Calc.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getExpressionsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (expressionsBuilder_ == null) {
expressions_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
expressionsBuilder_.clear();
}
projects_ = emptyLongList();
bitField0_ = (bitField0_ & ~0x00000002);
lazy_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
partialWindowFilterSelectivity_ = 0D;
bitField0_ = (bitField0_ & ~0x00000008);
optionalFilterCase_ = 0;
optionalFilter_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.OperatorProto.internal_static_cz_proto_Calc_descriptor;
}
@java.lang.Override
public cz.proto.Calc getDefaultInstanceForType() {
return cz.proto.Calc.getDefaultInstance();
}
@java.lang.Override
public cz.proto.Calc build() {
cz.proto.Calc result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.Calc buildPartial() {
cz.proto.Calc result = new cz.proto.Calc(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (expressionsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
expressions_ = java.util.Collections.unmodifiableList(expressions_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.expressions_ = expressions_;
} else {
result.expressions_ = expressionsBuilder_.build();
}
if (optionalFilterCase_ == 2) {
result.optionalFilter_ = optionalFilter_;
}
if (optionalFilterCase_ == 3) {
result.optionalFilter_ = optionalFilter_;
}
if (((bitField0_ & 0x00000002) != 0)) {
projects_.makeImmutable();
bitField0_ = (bitField0_ & ~0x00000002);
}
result.projects_ = projects_;
if (((bitField0_ & 0x00000004) != 0)) {
lazy_ = java.util.Collections.unmodifiableList(lazy_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.lazy_ = lazy_;
if (((from_bitField0_ & 0x00000008) != 0)) {
result.partialWindowFilterSelectivity_ = partialWindowFilterSelectivity_;
to_bitField0_ |= 0x00000001;
}
result.bitField0_ = to_bitField0_;
result.optionalFilterCase_ = optionalFilterCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.Calc) {
return mergeFrom((cz.proto.Calc)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.Calc other) {
if (other == cz.proto.Calc.getDefaultInstance()) return this;
if (expressionsBuilder_ == null) {
if (!other.expressions_.isEmpty()) {
if (expressions_.isEmpty()) {
expressions_ = other.expressions_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureExpressionsIsMutable();
expressions_.addAll(other.expressions_);
}
onChanged();
}
} else {
if (!other.expressions_.isEmpty()) {
if (expressionsBuilder_.isEmpty()) {
expressionsBuilder_.dispose();
expressionsBuilder_ = null;
expressions_ = other.expressions_;
bitField0_ = (bitField0_ & ~0x00000001);
expressionsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getExpressionsFieldBuilder() : null;
} else {
expressionsBuilder_.addAllMessages(other.expressions_);
}
}
}
if (!other.projects_.isEmpty()) {
if (projects_.isEmpty()) {
projects_ = other.projects_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureProjectsIsMutable();
projects_.addAll(other.projects_);
}
onChanged();
}
if (!other.lazy_.isEmpty()) {
if (lazy_.isEmpty()) {
lazy_ = other.lazy_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureLazyIsMutable();
lazy_.addAll(other.lazy_);
}
onChanged();
}
if (other.hasPartialWindowFilterSelectivity()) {
setPartialWindowFilterSelectivity(other.getPartialWindowFilterSelectivity());
}
switch (other.getOptionalFilterCase()) {
case NO_FILTER: {
setNoFilter(other.getNoFilter());
break;
}
case FILTER: {
setFilter(other.getFilter());
break;
}
case OPTIONALFILTER_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.Calc parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.Calc) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int optionalFilterCase_ = 0;
private java.lang.Object optionalFilter_;
public OptionalFilterCase
getOptionalFilterCase() {
return OptionalFilterCase.forNumber(
optionalFilterCase_);
}
public Builder clearOptionalFilter() {
optionalFilterCase_ = 0;
optionalFilter_ = null;
onChanged();
return this;
}
private int bitField0_;
private java.util.List expressions_ =
java.util.Collections.emptyList();
private void ensureExpressionsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
expressions_ = new java.util.ArrayList(expressions_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.Expression.ScalarExpression, cz.proto.Expression.ScalarExpression.Builder, cz.proto.Expression.ScalarExpressionOrBuilder> expressionsBuilder_;
/**
* repeated .cz.proto.ScalarExpression expressions = 1;
*/
public java.util.List getExpressionsList() {
if (expressionsBuilder_ == null) {
return java.util.Collections.unmodifiableList(expressions_);
} else {
return expressionsBuilder_.getMessageList();
}
}
/**
* repeated .cz.proto.ScalarExpression expressions = 1;
*/
public int getExpressionsCount() {
if (expressionsBuilder_ == null) {
return expressions_.size();
} else {
return expressionsBuilder_.getCount();
}
}
/**
* repeated .cz.proto.ScalarExpression expressions = 1;
*/
public cz.proto.Expression.ScalarExpression getExpressions(int index) {
if (expressionsBuilder_ == null) {
return expressions_.get(index);
} else {
return expressionsBuilder_.getMessage(index);
}
}
/**
* repeated .cz.proto.ScalarExpression expressions = 1;
*/
public Builder setExpressions(
int index, cz.proto.Expression.ScalarExpression value) {
if (expressionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureExpressionsIsMutable();
expressions_.set(index, value);
onChanged();
} else {
expressionsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .cz.proto.ScalarExpression expressions = 1;
*/
public Builder setExpressions(
int index, cz.proto.Expression.ScalarExpression.Builder builderForValue) {
if (expressionsBuilder_ == null) {
ensureExpressionsIsMutable();
expressions_.set(index, builderForValue.build());
onChanged();
} else {
expressionsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.ScalarExpression expressions = 1;
*/
public Builder addExpressions(cz.proto.Expression.ScalarExpression value) {
if (expressionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureExpressionsIsMutable();
expressions_.add(value);
onChanged();
} else {
expressionsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .cz.proto.ScalarExpression expressions = 1;
*/
public Builder addExpressions(
int index, cz.proto.Expression.ScalarExpression value) {
if (expressionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureExpressionsIsMutable();
expressions_.add(index, value);
onChanged();
} else {
expressionsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .cz.proto.ScalarExpression expressions = 1;
*/
public Builder addExpressions(
cz.proto.Expression.ScalarExpression.Builder builderForValue) {
if (expressionsBuilder_ == null) {
ensureExpressionsIsMutable();
expressions_.add(builderForValue.build());
onChanged();
} else {
expressionsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.ScalarExpression expressions = 1;
*/
public Builder addExpressions(
int index, cz.proto.Expression.ScalarExpression.Builder builderForValue) {
if (expressionsBuilder_ == null) {
ensureExpressionsIsMutable();
expressions_.add(index, builderForValue.build());
onChanged();
} else {
expressionsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.ScalarExpression expressions = 1;
*/
public Builder addAllExpressions(
java.lang.Iterable extends cz.proto.Expression.ScalarExpression> values) {
if (expressionsBuilder_ == null) {
ensureExpressionsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, expressions_);
onChanged();
} else {
expressionsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .cz.proto.ScalarExpression expressions = 1;
*/
public Builder clearExpressions() {
if (expressionsBuilder_ == null) {
expressions_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
expressionsBuilder_.clear();
}
return this;
}
/**
* repeated .cz.proto.ScalarExpression expressions = 1;
*/
public Builder removeExpressions(int index) {
if (expressionsBuilder_ == null) {
ensureExpressionsIsMutable();
expressions_.remove(index);
onChanged();
} else {
expressionsBuilder_.remove(index);
}
return this;
}
/**
* repeated .cz.proto.ScalarExpression expressions = 1;
*/
public cz.proto.Expression.ScalarExpression.Builder getExpressionsBuilder(
int index) {
return getExpressionsFieldBuilder().getBuilder(index);
}
/**
* repeated .cz.proto.ScalarExpression expressions = 1;
*/
public cz.proto.Expression.ScalarExpressionOrBuilder getExpressionsOrBuilder(
int index) {
if (expressionsBuilder_ == null) {
return expressions_.get(index); } else {
return expressionsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .cz.proto.ScalarExpression expressions = 1;
*/
public java.util.List extends cz.proto.Expression.ScalarExpressionOrBuilder>
getExpressionsOrBuilderList() {
if (expressionsBuilder_ != null) {
return expressionsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(expressions_);
}
}
/**
* repeated .cz.proto.ScalarExpression expressions = 1;
*/
public cz.proto.Expression.ScalarExpression.Builder addExpressionsBuilder() {
return getExpressionsFieldBuilder().addBuilder(
cz.proto.Expression.ScalarExpression.getDefaultInstance());
}
/**
* repeated .cz.proto.ScalarExpression expressions = 1;
*/
public cz.proto.Expression.ScalarExpression.Builder addExpressionsBuilder(
int index) {
return getExpressionsFieldBuilder().addBuilder(
index, cz.proto.Expression.ScalarExpression.getDefaultInstance());
}
/**
* repeated .cz.proto.ScalarExpression expressions = 1;
*/
public java.util.List
getExpressionsBuilderList() {
return getExpressionsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.Expression.ScalarExpression, cz.proto.Expression.ScalarExpression.Builder, cz.proto.Expression.ScalarExpressionOrBuilder>
getExpressionsFieldBuilder() {
if (expressionsBuilder_ == null) {
expressionsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.Expression.ScalarExpression, cz.proto.Expression.ScalarExpression.Builder, cz.proto.Expression.ScalarExpressionOrBuilder>(
expressions_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
expressions_ = null;
}
return expressionsBuilder_;
}
/**
* bool no_filter = 2;
* @return Whether the noFilter field is set.
*/
public boolean hasNoFilter() {
return optionalFilterCase_ == 2;
}
/**
* bool no_filter = 2;
* @return The noFilter.
*/
public boolean getNoFilter() {
if (optionalFilterCase_ == 2) {
return (java.lang.Boolean) optionalFilter_;
}
return false;
}
/**
* bool no_filter = 2;
* @param value The noFilter to set.
* @return This builder for chaining.
*/
public Builder setNoFilter(boolean value) {
optionalFilterCase_ = 2;
optionalFilter_ = value;
onChanged();
return this;
}
/**
* bool no_filter = 2;
* @return This builder for chaining.
*/
public Builder clearNoFilter() {
if (optionalFilterCase_ == 2) {
optionalFilterCase_ = 0;
optionalFilter_ = null;
onChanged();
}
return this;
}
/**
* uint64 filter = 3;
* @return Whether the filter field is set.
*/
public boolean hasFilter() {
return optionalFilterCase_ == 3;
}
/**
* uint64 filter = 3;
* @return The filter.
*/
public long getFilter() {
if (optionalFilterCase_ == 3) {
return (java.lang.Long) optionalFilter_;
}
return 0L;
}
/**
* uint64 filter = 3;
* @param value The filter to set.
* @return This builder for chaining.
*/
public Builder setFilter(long value) {
optionalFilterCase_ = 3;
optionalFilter_ = value;
onChanged();
return this;
}
/**
* uint64 filter = 3;
* @return This builder for chaining.
*/
public Builder clearFilter() {
if (optionalFilterCase_ == 3) {
optionalFilterCase_ = 0;
optionalFilter_ = null;
onChanged();
}
return this;
}
private com.google.protobuf.Internal.LongList projects_ = emptyLongList();
private void ensureProjectsIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
projects_ = mutableCopy(projects_);
bitField0_ |= 0x00000002;
}
}
/**
* repeated uint64 projects = 4;
* @return A list containing the projects.
*/
public java.util.List
getProjectsList() {
return ((bitField0_ & 0x00000002) != 0) ?
java.util.Collections.unmodifiableList(projects_) : projects_;
}
/**
* repeated uint64 projects = 4;
* @return The count of projects.
*/
public int getProjectsCount() {
return projects_.size();
}
/**
* repeated uint64 projects = 4;
* @param index The index of the element to return.
* @return The projects at the given index.
*/
public long getProjects(int index) {
return projects_.getLong(index);
}
/**
* repeated uint64 projects = 4;
* @param index The index to set the value at.
* @param value The projects to set.
* @return This builder for chaining.
*/
public Builder setProjects(
int index, long value) {
ensureProjectsIsMutable();
projects_.setLong(index, value);
onChanged();
return this;
}
/**
* repeated uint64 projects = 4;
* @param value The projects to add.
* @return This builder for chaining.
*/
public Builder addProjects(long value) {
ensureProjectsIsMutable();
projects_.addLong(value);
onChanged();
return this;
}
/**
* repeated uint64 projects = 4;
* @param values The projects to add.
* @return This builder for chaining.
*/
public Builder addAllProjects(
java.lang.Iterable extends java.lang.Long> values) {
ensureProjectsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, projects_);
onChanged();
return this;
}
/**
* repeated uint64 projects = 4;
* @return This builder for chaining.
*/
public Builder clearProjects() {
projects_ = emptyLongList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
private java.util.List lazy_ =
java.util.Collections.emptyList();
private void ensureLazyIsMutable() {
if (!((bitField0_ & 0x00000004) != 0)) {
lazy_ = new java.util.ArrayList(lazy_);
bitField0_ |= 0x00000004;
}
}
/**
* repeated .cz.proto.LazyEval lazy = 5;
* @return A list containing the lazy.
*/
public java.util.List getLazyList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, cz.proto.LazyEval>(lazy_, lazy_converter_);
}
/**
* repeated .cz.proto.LazyEval lazy = 5;
* @return The count of lazy.
*/
public int getLazyCount() {
return lazy_.size();
}
/**
* repeated .cz.proto.LazyEval lazy = 5;
* @param index The index of the element to return.
* @return The lazy at the given index.
*/
public cz.proto.LazyEval getLazy(int index) {
return lazy_converter_.convert(lazy_.get(index));
}
/**
* repeated .cz.proto.LazyEval lazy = 5;
* @param index The index to set the value at.
* @param value The lazy to set.
* @return This builder for chaining.
*/
public Builder setLazy(
int index, cz.proto.LazyEval value) {
if (value == null) {
throw new NullPointerException();
}
ensureLazyIsMutable();
lazy_.set(index, value.getNumber());
onChanged();
return this;
}
/**
* repeated .cz.proto.LazyEval lazy = 5;
* @param value The lazy to add.
* @return This builder for chaining.
*/
public Builder addLazy(cz.proto.LazyEval value) {
if (value == null) {
throw new NullPointerException();
}
ensureLazyIsMutable();
lazy_.add(value.getNumber());
onChanged();
return this;
}
/**
* repeated .cz.proto.LazyEval lazy = 5;
* @param values The lazy to add.
* @return This builder for chaining.
*/
public Builder addAllLazy(
java.lang.Iterable extends cz.proto.LazyEval> values) {
ensureLazyIsMutable();
for (cz.proto.LazyEval value : values) {
lazy_.add(value.getNumber());
}
onChanged();
return this;
}
/**
* repeated .cz.proto.LazyEval lazy = 5;
* @return This builder for chaining.
*/
public Builder clearLazy() {
lazy_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
* repeated .cz.proto.LazyEval lazy = 5;
* @return A list containing the enum numeric values on the wire for lazy.
*/
public java.util.List
getLazyValueList() {
return java.util.Collections.unmodifiableList(lazy_);
}
/**
* repeated .cz.proto.LazyEval lazy = 5;
* @param index The index of the value to return.
* @return The enum numeric value on the wire of lazy at the given index.
*/
public int getLazyValue(int index) {
return lazy_.get(index);
}
/**
* repeated .cz.proto.LazyEval lazy = 5;
* @param index The index of the value to return.
* @return The enum numeric value on the wire of lazy at the given index.
* @return This builder for chaining.
*/
public Builder setLazyValue(
int index, int value) {
ensureLazyIsMutable();
lazy_.set(index, value);
onChanged();
return this;
}
/**
* repeated .cz.proto.LazyEval lazy = 5;
* @param value The enum numeric value on the wire for lazy to add.
* @return This builder for chaining.
*/
public Builder addLazyValue(int value) {
ensureLazyIsMutable();
lazy_.add(value);
onChanged();
return this;
}
/**
* repeated .cz.proto.LazyEval lazy = 5;
* @param values The enum numeric values on the wire for lazy to add.
* @return This builder for chaining.
*/
public Builder addAllLazyValue(
java.lang.Iterable values) {
ensureLazyIsMutable();
for (int value : values) {
lazy_.add(value);
}
onChanged();
return this;
}
private double partialWindowFilterSelectivity_ ;
/**
* optional double partial_window_filter_selectivity = 6;
* @return Whether the partialWindowFilterSelectivity field is set.
*/
@java.lang.Override
public boolean hasPartialWindowFilterSelectivity() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional double partial_window_filter_selectivity = 6;
* @return The partialWindowFilterSelectivity.
*/
@java.lang.Override
public double getPartialWindowFilterSelectivity() {
return partialWindowFilterSelectivity_;
}
/**
* optional double partial_window_filter_selectivity = 6;
* @param value The partialWindowFilterSelectivity to set.
* @return This builder for chaining.
*/
public Builder setPartialWindowFilterSelectivity(double value) {
bitField0_ |= 0x00000008;
partialWindowFilterSelectivity_ = value;
onChanged();
return this;
}
/**
* optional double partial_window_filter_selectivity = 6;
* @return This builder for chaining.
*/
public Builder clearPartialWindowFilterSelectivity() {
bitField0_ = (bitField0_ & ~0x00000008);
partialWindowFilterSelectivity_ = 0D;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.Calc)
}
// @@protoc_insertion_point(class_scope:cz.proto.Calc)
private static final cz.proto.Calc DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.Calc();
}
public static cz.proto.Calc getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Calc parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Calc(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.Calc getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy