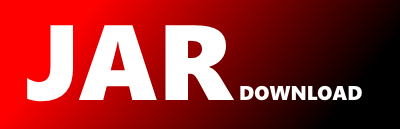
cz.proto.Distribution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickzetta-java Show documentation
Show all versions of clickzetta-java Show documentation
The java SDK for clickzetta's Lakehouse
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: operator.proto
package cz.proto;
/**
* Protobuf type {@code cz.proto.Distribution}
*/
public final class Distribution extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.Distribution)
DistributionOrBuilder {
private static final long serialVersionUID = 0L;
// Use Distribution.newBuilder() to construct.
private Distribution(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Distribution() {
type_ = 0;
keys_ = emptyLongList();
bucketType_ = 0;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Distribution();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Distribution(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int rawValue = input.readEnum();
type_ = rawValue;
break;
}
case 16: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
keys_ = newLongList();
mutable_bitField0_ |= 0x00000001;
}
keys_.addLong(input.readUInt64());
break;
}
case 18: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000001) != 0) && input.getBytesUntilLimit() > 0) {
keys_ = newLongList();
mutable_bitField0_ |= 0x00000001;
}
while (input.getBytesUntilLimit() > 0) {
keys_.addLong(input.readUInt64());
}
input.popLimit(limit);
break;
}
case 40: {
hasDop_ = input.readBool();
break;
}
case 48: {
dop_ = input.readUInt32();
break;
}
case 80: {
hasHashVersion_ = input.readBool();
break;
}
case 88: {
hashVersion_ = input.readUInt32();
break;
}
case 96: {
hasBucketType_ = input.readBool();
break;
}
case 104: {
int rawValue = input.readEnum();
bucketType_ = rawValue;
break;
}
case 160: {
rangeDistId_ = input.readUInt32();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
keys_.makeImmutable(); // C
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.OperatorProto.internal_static_cz_proto_Distribution_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.OperatorProto.internal_static_cz_proto_Distribution_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.Distribution.class, cz.proto.Distribution.Builder.class);
}
/**
* Protobuf enum {@code cz.proto.Distribution.Type}
*/
public enum Type
implements com.google.protobuf.ProtocolMessageEnum {
/**
* ANY = 0;
*/
ANY(0),
/**
* HASH = 1;
*/
HASH(1),
/**
* RANGE = 2;
*/
RANGE(2),
/**
* SINGLETON = 3;
*/
SINGLETON(3),
/**
* BROADCAST = 4;
*/
BROADCAST(4),
/**
* ROUND_ROBIN = 5;
*/
ROUND_ROBIN(5),
UNRECOGNIZED(-1),
;
/**
* ANY = 0;
*/
public static final int ANY_VALUE = 0;
/**
* HASH = 1;
*/
public static final int HASH_VALUE = 1;
/**
* RANGE = 2;
*/
public static final int RANGE_VALUE = 2;
/**
* SINGLETON = 3;
*/
public static final int SINGLETON_VALUE = 3;
/**
* BROADCAST = 4;
*/
public static final int BROADCAST_VALUE = 4;
/**
* ROUND_ROBIN = 5;
*/
public static final int ROUND_ROBIN_VALUE = 5;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Type valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static Type forNumber(int value) {
switch (value) {
case 0: return ANY;
case 1: return HASH;
case 2: return RANGE;
case 3: return SINGLETON;
case 4: return BROADCAST;
case 5: return ROUND_ROBIN;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
Type> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Type findValueByNumber(int number) {
return Type.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return cz.proto.Distribution.getDescriptor().getEnumTypes().get(0);
}
private static final Type[] VALUES = values();
public static Type valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Type(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:cz.proto.Distribution.Type)
}
public static final int TYPE_FIELD_NUMBER = 1;
private int type_;
/**
* .cz.proto.Distribution.Type type = 1;
* @return The enum numeric value on the wire for type.
*/
@java.lang.Override public int getTypeValue() {
return type_;
}
/**
* .cz.proto.Distribution.Type type = 1;
* @return The type.
*/
@java.lang.Override public cz.proto.Distribution.Type getType() {
@SuppressWarnings("deprecation")
cz.proto.Distribution.Type result = cz.proto.Distribution.Type.valueOf(type_);
return result == null ? cz.proto.Distribution.Type.UNRECOGNIZED : result;
}
public static final int KEYS_FIELD_NUMBER = 2;
private com.google.protobuf.Internal.LongList keys_;
/**
* repeated uint64 keys = 2;
* @return A list containing the keys.
*/
@java.lang.Override
public java.util.List
getKeysList() {
return keys_;
}
/**
* repeated uint64 keys = 2;
* @return The count of keys.
*/
public int getKeysCount() {
return keys_.size();
}
/**
* repeated uint64 keys = 2;
* @param index The index of the element to return.
* @return The keys at the given index.
*/
public long getKeys(int index) {
return keys_.getLong(index);
}
private int keysMemoizedSerializedSize = -1;
public static final int HAS_DOP_FIELD_NUMBER = 5;
private boolean hasDop_;
/**
* bool has_dop = 5;
* @return The hasDop.
*/
@java.lang.Override
public boolean getHasDop() {
return hasDop_;
}
public static final int DOP_FIELD_NUMBER = 6;
private int dop_;
/**
* uint32 dop = 6;
* @return The dop.
*/
@java.lang.Override
public int getDop() {
return dop_;
}
public static final int HAS_HASH_VERSION_FIELD_NUMBER = 10;
private boolean hasHashVersion_;
/**
* bool has_hash_version = 10;
* @return The hasHashVersion.
*/
@java.lang.Override
public boolean getHasHashVersion() {
return hasHashVersion_;
}
public static final int HASH_VERSION_FIELD_NUMBER = 11;
private int hashVersion_;
/**
* uint32 hash_version = 11;
* @return The hashVersion.
*/
@java.lang.Override
public int getHashVersion() {
return hashVersion_;
}
public static final int HAS_BUCKET_TYPE_FIELD_NUMBER = 12;
private boolean hasBucketType_;
/**
* bool has_bucket_type = 12;
* @return The hasBucketType.
*/
@java.lang.Override
public boolean getHasBucketType() {
return hasBucketType_;
}
public static final int BUCKET_TYPE_FIELD_NUMBER = 13;
private int bucketType_;
/**
* .cz.proto.HashBucketType bucket_type = 13;
* @return The enum numeric value on the wire for bucketType.
*/
@java.lang.Override public int getBucketTypeValue() {
return bucketType_;
}
/**
* .cz.proto.HashBucketType bucket_type = 13;
* @return The bucketType.
*/
@java.lang.Override public cz.proto.HashBucketType getBucketType() {
@SuppressWarnings("deprecation")
cz.proto.HashBucketType result = cz.proto.HashBucketType.valueOf(bucketType_);
return result == null ? cz.proto.HashBucketType.UNRECOGNIZED : result;
}
public static final int RANGE_DIST_ID_FIELD_NUMBER = 20;
private int rangeDistId_;
/**
*
* valid only when type = RANGE
*
*
* uint32 range_dist_id = 20;
* @return The rangeDistId.
*/
@java.lang.Override
public int getRangeDistId() {
return rangeDistId_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (type_ != cz.proto.Distribution.Type.ANY.getNumber()) {
output.writeEnum(1, type_);
}
if (getKeysList().size() > 0) {
output.writeUInt32NoTag(18);
output.writeUInt32NoTag(keysMemoizedSerializedSize);
}
for (int i = 0; i < keys_.size(); i++) {
output.writeUInt64NoTag(keys_.getLong(i));
}
if (hasDop_ != false) {
output.writeBool(5, hasDop_);
}
if (dop_ != 0) {
output.writeUInt32(6, dop_);
}
if (hasHashVersion_ != false) {
output.writeBool(10, hasHashVersion_);
}
if (hashVersion_ != 0) {
output.writeUInt32(11, hashVersion_);
}
if (hasBucketType_ != false) {
output.writeBool(12, hasBucketType_);
}
if (bucketType_ != cz.proto.HashBucketType.HASH_MOD.getNumber()) {
output.writeEnum(13, bucketType_);
}
if (rangeDistId_ != 0) {
output.writeUInt32(20, rangeDistId_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (type_ != cz.proto.Distribution.Type.ANY.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, type_);
}
{
int dataSize = 0;
for (int i = 0; i < keys_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeUInt64SizeNoTag(keys_.getLong(i));
}
size += dataSize;
if (!getKeysList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
keysMemoizedSerializedSize = dataSize;
}
if (hasDop_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, hasDop_);
}
if (dop_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(6, dop_);
}
if (hasHashVersion_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(10, hasHashVersion_);
}
if (hashVersion_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(11, hashVersion_);
}
if (hasBucketType_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(12, hasBucketType_);
}
if (bucketType_ != cz.proto.HashBucketType.HASH_MOD.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(13, bucketType_);
}
if (rangeDistId_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(20, rangeDistId_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.Distribution)) {
return super.equals(obj);
}
cz.proto.Distribution other = (cz.proto.Distribution) obj;
if (type_ != other.type_) return false;
if (!getKeysList()
.equals(other.getKeysList())) return false;
if (getHasDop()
!= other.getHasDop()) return false;
if (getDop()
!= other.getDop()) return false;
if (getHasHashVersion()
!= other.getHasHashVersion()) return false;
if (getHashVersion()
!= other.getHashVersion()) return false;
if (getHasBucketType()
!= other.getHasBucketType()) return false;
if (bucketType_ != other.bucketType_) return false;
if (getRangeDistId()
!= other.getRangeDistId()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + type_;
if (getKeysCount() > 0) {
hash = (37 * hash) + KEYS_FIELD_NUMBER;
hash = (53 * hash) + getKeysList().hashCode();
}
hash = (37 * hash) + HAS_DOP_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getHasDop());
hash = (37 * hash) + DOP_FIELD_NUMBER;
hash = (53 * hash) + getDop();
hash = (37 * hash) + HAS_HASH_VERSION_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getHasHashVersion());
hash = (37 * hash) + HASH_VERSION_FIELD_NUMBER;
hash = (53 * hash) + getHashVersion();
hash = (37 * hash) + HAS_BUCKET_TYPE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getHasBucketType());
hash = (37 * hash) + BUCKET_TYPE_FIELD_NUMBER;
hash = (53 * hash) + bucketType_;
hash = (37 * hash) + RANGE_DIST_ID_FIELD_NUMBER;
hash = (53 * hash) + getRangeDistId();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.Distribution parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Distribution parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Distribution parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Distribution parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Distribution parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Distribution parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Distribution parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.Distribution parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.Distribution parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.Distribution parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.Distribution parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.Distribution parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.Distribution prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.Distribution}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.Distribution)
cz.proto.DistributionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.OperatorProto.internal_static_cz_proto_Distribution_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.OperatorProto.internal_static_cz_proto_Distribution_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.Distribution.class, cz.proto.Distribution.Builder.class);
}
// Construct using cz.proto.Distribution.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
type_ = 0;
keys_ = emptyLongList();
bitField0_ = (bitField0_ & ~0x00000001);
hasDop_ = false;
dop_ = 0;
hasHashVersion_ = false;
hashVersion_ = 0;
hasBucketType_ = false;
bucketType_ = 0;
rangeDistId_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.OperatorProto.internal_static_cz_proto_Distribution_descriptor;
}
@java.lang.Override
public cz.proto.Distribution getDefaultInstanceForType() {
return cz.proto.Distribution.getDefaultInstance();
}
@java.lang.Override
public cz.proto.Distribution build() {
cz.proto.Distribution result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.Distribution buildPartial() {
cz.proto.Distribution result = new cz.proto.Distribution(this);
int from_bitField0_ = bitField0_;
result.type_ = type_;
if (((bitField0_ & 0x00000001) != 0)) {
keys_.makeImmutable();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.keys_ = keys_;
result.hasDop_ = hasDop_;
result.dop_ = dop_;
result.hasHashVersion_ = hasHashVersion_;
result.hashVersion_ = hashVersion_;
result.hasBucketType_ = hasBucketType_;
result.bucketType_ = bucketType_;
result.rangeDistId_ = rangeDistId_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.Distribution) {
return mergeFrom((cz.proto.Distribution)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.Distribution other) {
if (other == cz.proto.Distribution.getDefaultInstance()) return this;
if (other.type_ != 0) {
setTypeValue(other.getTypeValue());
}
if (!other.keys_.isEmpty()) {
if (keys_.isEmpty()) {
keys_ = other.keys_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureKeysIsMutable();
keys_.addAll(other.keys_);
}
onChanged();
}
if (other.getHasDop() != false) {
setHasDop(other.getHasDop());
}
if (other.getDop() != 0) {
setDop(other.getDop());
}
if (other.getHasHashVersion() != false) {
setHasHashVersion(other.getHasHashVersion());
}
if (other.getHashVersion() != 0) {
setHashVersion(other.getHashVersion());
}
if (other.getHasBucketType() != false) {
setHasBucketType(other.getHasBucketType());
}
if (other.bucketType_ != 0) {
setBucketTypeValue(other.getBucketTypeValue());
}
if (other.getRangeDistId() != 0) {
setRangeDistId(other.getRangeDistId());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.Distribution parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.Distribution) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int type_ = 0;
/**
* .cz.proto.Distribution.Type type = 1;
* @return The enum numeric value on the wire for type.
*/
@java.lang.Override public int getTypeValue() {
return type_;
}
/**
* .cz.proto.Distribution.Type type = 1;
* @param value The enum numeric value on the wire for type to set.
* @return This builder for chaining.
*/
public Builder setTypeValue(int value) {
type_ = value;
onChanged();
return this;
}
/**
* .cz.proto.Distribution.Type type = 1;
* @return The type.
*/
@java.lang.Override
public cz.proto.Distribution.Type getType() {
@SuppressWarnings("deprecation")
cz.proto.Distribution.Type result = cz.proto.Distribution.Type.valueOf(type_);
return result == null ? cz.proto.Distribution.Type.UNRECOGNIZED : result;
}
/**
* .cz.proto.Distribution.Type type = 1;
* @param value The type to set.
* @return This builder for chaining.
*/
public Builder setType(cz.proto.Distribution.Type value) {
if (value == null) {
throw new NullPointerException();
}
type_ = value.getNumber();
onChanged();
return this;
}
/**
* .cz.proto.Distribution.Type type = 1;
* @return This builder for chaining.
*/
public Builder clearType() {
type_ = 0;
onChanged();
return this;
}
private com.google.protobuf.Internal.LongList keys_ = emptyLongList();
private void ensureKeysIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
keys_ = mutableCopy(keys_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated uint64 keys = 2;
* @return A list containing the keys.
*/
public java.util.List
getKeysList() {
return ((bitField0_ & 0x00000001) != 0) ?
java.util.Collections.unmodifiableList(keys_) : keys_;
}
/**
* repeated uint64 keys = 2;
* @return The count of keys.
*/
public int getKeysCount() {
return keys_.size();
}
/**
* repeated uint64 keys = 2;
* @param index The index of the element to return.
* @return The keys at the given index.
*/
public long getKeys(int index) {
return keys_.getLong(index);
}
/**
* repeated uint64 keys = 2;
* @param index The index to set the value at.
* @param value The keys to set.
* @return This builder for chaining.
*/
public Builder setKeys(
int index, long value) {
ensureKeysIsMutable();
keys_.setLong(index, value);
onChanged();
return this;
}
/**
* repeated uint64 keys = 2;
* @param value The keys to add.
* @return This builder for chaining.
*/
public Builder addKeys(long value) {
ensureKeysIsMutable();
keys_.addLong(value);
onChanged();
return this;
}
/**
* repeated uint64 keys = 2;
* @param values The keys to add.
* @return This builder for chaining.
*/
public Builder addAllKeys(
java.lang.Iterable extends java.lang.Long> values) {
ensureKeysIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, keys_);
onChanged();
return this;
}
/**
* repeated uint64 keys = 2;
* @return This builder for chaining.
*/
public Builder clearKeys() {
keys_ = emptyLongList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
private boolean hasDop_ ;
/**
* bool has_dop = 5;
* @return The hasDop.
*/
@java.lang.Override
public boolean getHasDop() {
return hasDop_;
}
/**
* bool has_dop = 5;
* @param value The hasDop to set.
* @return This builder for chaining.
*/
public Builder setHasDop(boolean value) {
hasDop_ = value;
onChanged();
return this;
}
/**
* bool has_dop = 5;
* @return This builder for chaining.
*/
public Builder clearHasDop() {
hasDop_ = false;
onChanged();
return this;
}
private int dop_ ;
/**
* uint32 dop = 6;
* @return The dop.
*/
@java.lang.Override
public int getDop() {
return dop_;
}
/**
* uint32 dop = 6;
* @param value The dop to set.
* @return This builder for chaining.
*/
public Builder setDop(int value) {
dop_ = value;
onChanged();
return this;
}
/**
* uint32 dop = 6;
* @return This builder for chaining.
*/
public Builder clearDop() {
dop_ = 0;
onChanged();
return this;
}
private boolean hasHashVersion_ ;
/**
* bool has_hash_version = 10;
* @return The hasHashVersion.
*/
@java.lang.Override
public boolean getHasHashVersion() {
return hasHashVersion_;
}
/**
* bool has_hash_version = 10;
* @param value The hasHashVersion to set.
* @return This builder for chaining.
*/
public Builder setHasHashVersion(boolean value) {
hasHashVersion_ = value;
onChanged();
return this;
}
/**
* bool has_hash_version = 10;
* @return This builder for chaining.
*/
public Builder clearHasHashVersion() {
hasHashVersion_ = false;
onChanged();
return this;
}
private int hashVersion_ ;
/**
* uint32 hash_version = 11;
* @return The hashVersion.
*/
@java.lang.Override
public int getHashVersion() {
return hashVersion_;
}
/**
* uint32 hash_version = 11;
* @param value The hashVersion to set.
* @return This builder for chaining.
*/
public Builder setHashVersion(int value) {
hashVersion_ = value;
onChanged();
return this;
}
/**
* uint32 hash_version = 11;
* @return This builder for chaining.
*/
public Builder clearHashVersion() {
hashVersion_ = 0;
onChanged();
return this;
}
private boolean hasBucketType_ ;
/**
* bool has_bucket_type = 12;
* @return The hasBucketType.
*/
@java.lang.Override
public boolean getHasBucketType() {
return hasBucketType_;
}
/**
* bool has_bucket_type = 12;
* @param value The hasBucketType to set.
* @return This builder for chaining.
*/
public Builder setHasBucketType(boolean value) {
hasBucketType_ = value;
onChanged();
return this;
}
/**
* bool has_bucket_type = 12;
* @return This builder for chaining.
*/
public Builder clearHasBucketType() {
hasBucketType_ = false;
onChanged();
return this;
}
private int bucketType_ = 0;
/**
* .cz.proto.HashBucketType bucket_type = 13;
* @return The enum numeric value on the wire for bucketType.
*/
@java.lang.Override public int getBucketTypeValue() {
return bucketType_;
}
/**
* .cz.proto.HashBucketType bucket_type = 13;
* @param value The enum numeric value on the wire for bucketType to set.
* @return This builder for chaining.
*/
public Builder setBucketTypeValue(int value) {
bucketType_ = value;
onChanged();
return this;
}
/**
* .cz.proto.HashBucketType bucket_type = 13;
* @return The bucketType.
*/
@java.lang.Override
public cz.proto.HashBucketType getBucketType() {
@SuppressWarnings("deprecation")
cz.proto.HashBucketType result = cz.proto.HashBucketType.valueOf(bucketType_);
return result == null ? cz.proto.HashBucketType.UNRECOGNIZED : result;
}
/**
* .cz.proto.HashBucketType bucket_type = 13;
* @param value The bucketType to set.
* @return This builder for chaining.
*/
public Builder setBucketType(cz.proto.HashBucketType value) {
if (value == null) {
throw new NullPointerException();
}
bucketType_ = value.getNumber();
onChanged();
return this;
}
/**
* .cz.proto.HashBucketType bucket_type = 13;
* @return This builder for chaining.
*/
public Builder clearBucketType() {
bucketType_ = 0;
onChanged();
return this;
}
private int rangeDistId_ ;
/**
*
* valid only when type = RANGE
*
*
* uint32 range_dist_id = 20;
* @return The rangeDistId.
*/
@java.lang.Override
public int getRangeDistId() {
return rangeDistId_;
}
/**
*
* valid only when type = RANGE
*
*
* uint32 range_dist_id = 20;
* @param value The rangeDistId to set.
* @return This builder for chaining.
*/
public Builder setRangeDistId(int value) {
rangeDistId_ = value;
onChanged();
return this;
}
/**
*
* valid only when type = RANGE
*
*
* uint32 range_dist_id = 20;
* @return This builder for chaining.
*/
public Builder clearRangeDistId() {
rangeDistId_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.Distribution)
}
// @@protoc_insertion_point(class_scope:cz.proto.Distribution)
private static final cz.proto.Distribution DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.Distribution();
}
public static cz.proto.Distribution getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Distribution parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Distribution(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.Distribution getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy