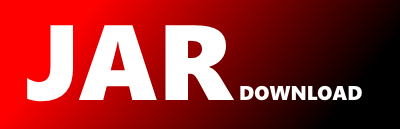
cz.proto.Expression Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickzetta-java Show documentation
Show all versions of clickzetta-java Show documentation
The java SDK for clickzetta's Lakehouse
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: expression.proto
package cz.proto;
public final class Expression {
private Expression() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
* Protobuf enum {@code cz.proto.ReferenceType}
*/
public enum ReferenceType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* LOGICAL_FIELD = 0;
*/
LOGICAL_FIELD(0),
/**
* REF_LOCAL = 1;
*/
REF_LOCAL(1),
/**
* PHYSICAL_FIELD = 2;
*/
PHYSICAL_FIELD(2),
/**
* REF_VARIABLE = 3;
*/
REF_VARIABLE(3),
UNRECOGNIZED(-1),
;
/**
* LOGICAL_FIELD = 0;
*/
public static final int LOGICAL_FIELD_VALUE = 0;
/**
* REF_LOCAL = 1;
*/
public static final int REF_LOCAL_VALUE = 1;
/**
* PHYSICAL_FIELD = 2;
*/
public static final int PHYSICAL_FIELD_VALUE = 2;
/**
* REF_VARIABLE = 3;
*/
public static final int REF_VARIABLE_VALUE = 3;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ReferenceType valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static ReferenceType forNumber(int value) {
switch (value) {
case 0: return LOGICAL_FIELD;
case 1: return REF_LOCAL;
case 2: return PHYSICAL_FIELD;
case 3: return REF_VARIABLE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
ReferenceType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public ReferenceType findValueByNumber(int number) {
return ReferenceType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return cz.proto.Expression.getDescriptor().getEnumTypes().get(0);
}
private static final ReferenceType[] VALUES = values();
public static ReferenceType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private ReferenceType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:cz.proto.ReferenceType)
}
public interface ParseTreeInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ParseTreeInfo)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.ParseTreeInfo.LocationInfo start = 1;
* @return Whether the start field is set.
*/
boolean hasStart();
/**
* .cz.proto.ParseTreeInfo.LocationInfo start = 1;
* @return The start.
*/
cz.proto.Expression.ParseTreeInfo.LocationInfo getStart();
/**
* .cz.proto.ParseTreeInfo.LocationInfo start = 1;
*/
cz.proto.Expression.ParseTreeInfo.LocationInfoOrBuilder getStartOrBuilder();
/**
* .cz.proto.ParseTreeInfo.LocationInfo end = 2;
* @return Whether the end field is set.
*/
boolean hasEnd();
/**
* .cz.proto.ParseTreeInfo.LocationInfo end = 2;
* @return The end.
*/
cz.proto.Expression.ParseTreeInfo.LocationInfo getEnd();
/**
* .cz.proto.ParseTreeInfo.LocationInfo end = 2;
*/
cz.proto.Expression.ParseTreeInfo.LocationInfoOrBuilder getEndOrBuilder();
}
/**
* Protobuf type {@code cz.proto.ParseTreeInfo}
*/
public static final class ParseTreeInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ParseTreeInfo)
ParseTreeInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use ParseTreeInfo.newBuilder() to construct.
private ParseTreeInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ParseTreeInfo() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ParseTreeInfo();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ParseTreeInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.Expression.ParseTreeInfo.LocationInfo.Builder subBuilder = null;
if (start_ != null) {
subBuilder = start_.toBuilder();
}
start_ = input.readMessage(cz.proto.Expression.ParseTreeInfo.LocationInfo.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(start_);
start_ = subBuilder.buildPartial();
}
break;
}
case 18: {
cz.proto.Expression.ParseTreeInfo.LocationInfo.Builder subBuilder = null;
if (end_ != null) {
subBuilder = end_.toBuilder();
}
end_ = input.readMessage(cz.proto.Expression.ParseTreeInfo.LocationInfo.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(end_);
end_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.Expression.internal_static_cz_proto_ParseTreeInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.Expression.internal_static_cz_proto_ParseTreeInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.Expression.ParseTreeInfo.class, cz.proto.Expression.ParseTreeInfo.Builder.class);
}
public interface LocationInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ParseTreeInfo.LocationInfo)
com.google.protobuf.MessageOrBuilder {
/**
* uint32 line = 1;
* @return The line.
*/
int getLine();
/**
* uint32 col = 2;
* @return The col.
*/
int getCol();
/**
* uint32 pos = 3;
* @return The pos.
*/
int getPos();
}
/**
* Protobuf type {@code cz.proto.ParseTreeInfo.LocationInfo}
*/
public static final class LocationInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ParseTreeInfo.LocationInfo)
LocationInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use LocationInfo.newBuilder() to construct.
private LocationInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private LocationInfo() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new LocationInfo();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private LocationInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
line_ = input.readUInt32();
break;
}
case 16: {
col_ = input.readUInt32();
break;
}
case 24: {
pos_ = input.readUInt32();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.Expression.internal_static_cz_proto_ParseTreeInfo_LocationInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.Expression.internal_static_cz_proto_ParseTreeInfo_LocationInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.Expression.ParseTreeInfo.LocationInfo.class, cz.proto.Expression.ParseTreeInfo.LocationInfo.Builder.class);
}
public static final int LINE_FIELD_NUMBER = 1;
private int line_;
/**
* uint32 line = 1;
* @return The line.
*/
@java.lang.Override
public int getLine() {
return line_;
}
public static final int COL_FIELD_NUMBER = 2;
private int col_;
/**
* uint32 col = 2;
* @return The col.
*/
@java.lang.Override
public int getCol() {
return col_;
}
public static final int POS_FIELD_NUMBER = 3;
private int pos_;
/**
* uint32 pos = 3;
* @return The pos.
*/
@java.lang.Override
public int getPos() {
return pos_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (line_ != 0) {
output.writeUInt32(1, line_);
}
if (col_ != 0) {
output.writeUInt32(2, col_);
}
if (pos_ != 0) {
output.writeUInt32(3, pos_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (line_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, line_);
}
if (col_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(2, col_);
}
if (pos_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(3, pos_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.Expression.ParseTreeInfo.LocationInfo)) {
return super.equals(obj);
}
cz.proto.Expression.ParseTreeInfo.LocationInfo other = (cz.proto.Expression.ParseTreeInfo.LocationInfo) obj;
if (getLine()
!= other.getLine()) return false;
if (getCol()
!= other.getCol()) return false;
if (getPos()
!= other.getPos()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + LINE_FIELD_NUMBER;
hash = (53 * hash) + getLine();
hash = (37 * hash) + COL_FIELD_NUMBER;
hash = (53 * hash) + getCol();
hash = (37 * hash) + POS_FIELD_NUMBER;
hash = (53 * hash) + getPos();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.Expression.ParseTreeInfo.LocationInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.ParseTreeInfo.LocationInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.ParseTreeInfo.LocationInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.ParseTreeInfo.LocationInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.ParseTreeInfo.LocationInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.ParseTreeInfo.LocationInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.ParseTreeInfo.LocationInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.Expression.ParseTreeInfo.LocationInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.Expression.ParseTreeInfo.LocationInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.Expression.ParseTreeInfo.LocationInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.Expression.ParseTreeInfo.LocationInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.Expression.ParseTreeInfo.LocationInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.Expression.ParseTreeInfo.LocationInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ParseTreeInfo.LocationInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ParseTreeInfo.LocationInfo)
cz.proto.Expression.ParseTreeInfo.LocationInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.Expression.internal_static_cz_proto_ParseTreeInfo_LocationInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.Expression.internal_static_cz_proto_ParseTreeInfo_LocationInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.Expression.ParseTreeInfo.LocationInfo.class, cz.proto.Expression.ParseTreeInfo.LocationInfo.Builder.class);
}
// Construct using cz.proto.Expression.ParseTreeInfo.LocationInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
line_ = 0;
col_ = 0;
pos_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.Expression.internal_static_cz_proto_ParseTreeInfo_LocationInfo_descriptor;
}
@java.lang.Override
public cz.proto.Expression.ParseTreeInfo.LocationInfo getDefaultInstanceForType() {
return cz.proto.Expression.ParseTreeInfo.LocationInfo.getDefaultInstance();
}
@java.lang.Override
public cz.proto.Expression.ParseTreeInfo.LocationInfo build() {
cz.proto.Expression.ParseTreeInfo.LocationInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.Expression.ParseTreeInfo.LocationInfo buildPartial() {
cz.proto.Expression.ParseTreeInfo.LocationInfo result = new cz.proto.Expression.ParseTreeInfo.LocationInfo(this);
result.line_ = line_;
result.col_ = col_;
result.pos_ = pos_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.Expression.ParseTreeInfo.LocationInfo) {
return mergeFrom((cz.proto.Expression.ParseTreeInfo.LocationInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.Expression.ParseTreeInfo.LocationInfo other) {
if (other == cz.proto.Expression.ParseTreeInfo.LocationInfo.getDefaultInstance()) return this;
if (other.getLine() != 0) {
setLine(other.getLine());
}
if (other.getCol() != 0) {
setCol(other.getCol());
}
if (other.getPos() != 0) {
setPos(other.getPos());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.Expression.ParseTreeInfo.LocationInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.Expression.ParseTreeInfo.LocationInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int line_ ;
/**
* uint32 line = 1;
* @return The line.
*/
@java.lang.Override
public int getLine() {
return line_;
}
/**
* uint32 line = 1;
* @param value The line to set.
* @return This builder for chaining.
*/
public Builder setLine(int value) {
line_ = value;
onChanged();
return this;
}
/**
* uint32 line = 1;
* @return This builder for chaining.
*/
public Builder clearLine() {
line_ = 0;
onChanged();
return this;
}
private int col_ ;
/**
* uint32 col = 2;
* @return The col.
*/
@java.lang.Override
public int getCol() {
return col_;
}
/**
* uint32 col = 2;
* @param value The col to set.
* @return This builder for chaining.
*/
public Builder setCol(int value) {
col_ = value;
onChanged();
return this;
}
/**
* uint32 col = 2;
* @return This builder for chaining.
*/
public Builder clearCol() {
col_ = 0;
onChanged();
return this;
}
private int pos_ ;
/**
* uint32 pos = 3;
* @return The pos.
*/
@java.lang.Override
public int getPos() {
return pos_;
}
/**
* uint32 pos = 3;
* @param value The pos to set.
* @return This builder for chaining.
*/
public Builder setPos(int value) {
pos_ = value;
onChanged();
return this;
}
/**
* uint32 pos = 3;
* @return This builder for chaining.
*/
public Builder clearPos() {
pos_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ParseTreeInfo.LocationInfo)
}
// @@protoc_insertion_point(class_scope:cz.proto.ParseTreeInfo.LocationInfo)
private static final cz.proto.Expression.ParseTreeInfo.LocationInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.Expression.ParseTreeInfo.LocationInfo();
}
public static cz.proto.Expression.ParseTreeInfo.LocationInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public LocationInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new LocationInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.Expression.ParseTreeInfo.LocationInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public static final int START_FIELD_NUMBER = 1;
private cz.proto.Expression.ParseTreeInfo.LocationInfo start_;
/**
* .cz.proto.ParseTreeInfo.LocationInfo start = 1;
* @return Whether the start field is set.
*/
@java.lang.Override
public boolean hasStart() {
return start_ != null;
}
/**
* .cz.proto.ParseTreeInfo.LocationInfo start = 1;
* @return The start.
*/
@java.lang.Override
public cz.proto.Expression.ParseTreeInfo.LocationInfo getStart() {
return start_ == null ? cz.proto.Expression.ParseTreeInfo.LocationInfo.getDefaultInstance() : start_;
}
/**
* .cz.proto.ParseTreeInfo.LocationInfo start = 1;
*/
@java.lang.Override
public cz.proto.Expression.ParseTreeInfo.LocationInfoOrBuilder getStartOrBuilder() {
return getStart();
}
public static final int END_FIELD_NUMBER = 2;
private cz.proto.Expression.ParseTreeInfo.LocationInfo end_;
/**
* .cz.proto.ParseTreeInfo.LocationInfo end = 2;
* @return Whether the end field is set.
*/
@java.lang.Override
public boolean hasEnd() {
return end_ != null;
}
/**
* .cz.proto.ParseTreeInfo.LocationInfo end = 2;
* @return The end.
*/
@java.lang.Override
public cz.proto.Expression.ParseTreeInfo.LocationInfo getEnd() {
return end_ == null ? cz.proto.Expression.ParseTreeInfo.LocationInfo.getDefaultInstance() : end_;
}
/**
* .cz.proto.ParseTreeInfo.LocationInfo end = 2;
*/
@java.lang.Override
public cz.proto.Expression.ParseTreeInfo.LocationInfoOrBuilder getEndOrBuilder() {
return getEnd();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (start_ != null) {
output.writeMessage(1, getStart());
}
if (end_ != null) {
output.writeMessage(2, getEnd());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (start_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getStart());
}
if (end_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getEnd());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.Expression.ParseTreeInfo)) {
return super.equals(obj);
}
cz.proto.Expression.ParseTreeInfo other = (cz.proto.Expression.ParseTreeInfo) obj;
if (hasStart() != other.hasStart()) return false;
if (hasStart()) {
if (!getStart()
.equals(other.getStart())) return false;
}
if (hasEnd() != other.hasEnd()) return false;
if (hasEnd()) {
if (!getEnd()
.equals(other.getEnd())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasStart()) {
hash = (37 * hash) + START_FIELD_NUMBER;
hash = (53 * hash) + getStart().hashCode();
}
if (hasEnd()) {
hash = (37 * hash) + END_FIELD_NUMBER;
hash = (53 * hash) + getEnd().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.Expression.ParseTreeInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.ParseTreeInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.ParseTreeInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.ParseTreeInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.ParseTreeInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.ParseTreeInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.ParseTreeInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.Expression.ParseTreeInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.Expression.ParseTreeInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.Expression.ParseTreeInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.Expression.ParseTreeInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.Expression.ParseTreeInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.Expression.ParseTreeInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ParseTreeInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ParseTreeInfo)
cz.proto.Expression.ParseTreeInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.Expression.internal_static_cz_proto_ParseTreeInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.Expression.internal_static_cz_proto_ParseTreeInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.Expression.ParseTreeInfo.class, cz.proto.Expression.ParseTreeInfo.Builder.class);
}
// Construct using cz.proto.Expression.ParseTreeInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (startBuilder_ == null) {
start_ = null;
} else {
start_ = null;
startBuilder_ = null;
}
if (endBuilder_ == null) {
end_ = null;
} else {
end_ = null;
endBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.Expression.internal_static_cz_proto_ParseTreeInfo_descriptor;
}
@java.lang.Override
public cz.proto.Expression.ParseTreeInfo getDefaultInstanceForType() {
return cz.proto.Expression.ParseTreeInfo.getDefaultInstance();
}
@java.lang.Override
public cz.proto.Expression.ParseTreeInfo build() {
cz.proto.Expression.ParseTreeInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.Expression.ParseTreeInfo buildPartial() {
cz.proto.Expression.ParseTreeInfo result = new cz.proto.Expression.ParseTreeInfo(this);
if (startBuilder_ == null) {
result.start_ = start_;
} else {
result.start_ = startBuilder_.build();
}
if (endBuilder_ == null) {
result.end_ = end_;
} else {
result.end_ = endBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.Expression.ParseTreeInfo) {
return mergeFrom((cz.proto.Expression.ParseTreeInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.Expression.ParseTreeInfo other) {
if (other == cz.proto.Expression.ParseTreeInfo.getDefaultInstance()) return this;
if (other.hasStart()) {
mergeStart(other.getStart());
}
if (other.hasEnd()) {
mergeEnd(other.getEnd());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.Expression.ParseTreeInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.Expression.ParseTreeInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cz.proto.Expression.ParseTreeInfo.LocationInfo start_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.ParseTreeInfo.LocationInfo, cz.proto.Expression.ParseTreeInfo.LocationInfo.Builder, cz.proto.Expression.ParseTreeInfo.LocationInfoOrBuilder> startBuilder_;
/**
* .cz.proto.ParseTreeInfo.LocationInfo start = 1;
* @return Whether the start field is set.
*/
public boolean hasStart() {
return startBuilder_ != null || start_ != null;
}
/**
* .cz.proto.ParseTreeInfo.LocationInfo start = 1;
* @return The start.
*/
public cz.proto.Expression.ParseTreeInfo.LocationInfo getStart() {
if (startBuilder_ == null) {
return start_ == null ? cz.proto.Expression.ParseTreeInfo.LocationInfo.getDefaultInstance() : start_;
} else {
return startBuilder_.getMessage();
}
}
/**
* .cz.proto.ParseTreeInfo.LocationInfo start = 1;
*/
public Builder setStart(cz.proto.Expression.ParseTreeInfo.LocationInfo value) {
if (startBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
start_ = value;
onChanged();
} else {
startBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ParseTreeInfo.LocationInfo start = 1;
*/
public Builder setStart(
cz.proto.Expression.ParseTreeInfo.LocationInfo.Builder builderForValue) {
if (startBuilder_ == null) {
start_ = builderForValue.build();
onChanged();
} else {
startBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ParseTreeInfo.LocationInfo start = 1;
*/
public Builder mergeStart(cz.proto.Expression.ParseTreeInfo.LocationInfo value) {
if (startBuilder_ == null) {
if (start_ != null) {
start_ =
cz.proto.Expression.ParseTreeInfo.LocationInfo.newBuilder(start_).mergeFrom(value).buildPartial();
} else {
start_ = value;
}
onChanged();
} else {
startBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ParseTreeInfo.LocationInfo start = 1;
*/
public Builder clearStart() {
if (startBuilder_ == null) {
start_ = null;
onChanged();
} else {
start_ = null;
startBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ParseTreeInfo.LocationInfo start = 1;
*/
public cz.proto.Expression.ParseTreeInfo.LocationInfo.Builder getStartBuilder() {
onChanged();
return getStartFieldBuilder().getBuilder();
}
/**
* .cz.proto.ParseTreeInfo.LocationInfo start = 1;
*/
public cz.proto.Expression.ParseTreeInfo.LocationInfoOrBuilder getStartOrBuilder() {
if (startBuilder_ != null) {
return startBuilder_.getMessageOrBuilder();
} else {
return start_ == null ?
cz.proto.Expression.ParseTreeInfo.LocationInfo.getDefaultInstance() : start_;
}
}
/**
* .cz.proto.ParseTreeInfo.LocationInfo start = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.ParseTreeInfo.LocationInfo, cz.proto.Expression.ParseTreeInfo.LocationInfo.Builder, cz.proto.Expression.ParseTreeInfo.LocationInfoOrBuilder>
getStartFieldBuilder() {
if (startBuilder_ == null) {
startBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.ParseTreeInfo.LocationInfo, cz.proto.Expression.ParseTreeInfo.LocationInfo.Builder, cz.proto.Expression.ParseTreeInfo.LocationInfoOrBuilder>(
getStart(),
getParentForChildren(),
isClean());
start_ = null;
}
return startBuilder_;
}
private cz.proto.Expression.ParseTreeInfo.LocationInfo end_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.ParseTreeInfo.LocationInfo, cz.proto.Expression.ParseTreeInfo.LocationInfo.Builder, cz.proto.Expression.ParseTreeInfo.LocationInfoOrBuilder> endBuilder_;
/**
* .cz.proto.ParseTreeInfo.LocationInfo end = 2;
* @return Whether the end field is set.
*/
public boolean hasEnd() {
return endBuilder_ != null || end_ != null;
}
/**
* .cz.proto.ParseTreeInfo.LocationInfo end = 2;
* @return The end.
*/
public cz.proto.Expression.ParseTreeInfo.LocationInfo getEnd() {
if (endBuilder_ == null) {
return end_ == null ? cz.proto.Expression.ParseTreeInfo.LocationInfo.getDefaultInstance() : end_;
} else {
return endBuilder_.getMessage();
}
}
/**
* .cz.proto.ParseTreeInfo.LocationInfo end = 2;
*/
public Builder setEnd(cz.proto.Expression.ParseTreeInfo.LocationInfo value) {
if (endBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
end_ = value;
onChanged();
} else {
endBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ParseTreeInfo.LocationInfo end = 2;
*/
public Builder setEnd(
cz.proto.Expression.ParseTreeInfo.LocationInfo.Builder builderForValue) {
if (endBuilder_ == null) {
end_ = builderForValue.build();
onChanged();
} else {
endBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ParseTreeInfo.LocationInfo end = 2;
*/
public Builder mergeEnd(cz.proto.Expression.ParseTreeInfo.LocationInfo value) {
if (endBuilder_ == null) {
if (end_ != null) {
end_ =
cz.proto.Expression.ParseTreeInfo.LocationInfo.newBuilder(end_).mergeFrom(value).buildPartial();
} else {
end_ = value;
}
onChanged();
} else {
endBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ParseTreeInfo.LocationInfo end = 2;
*/
public Builder clearEnd() {
if (endBuilder_ == null) {
end_ = null;
onChanged();
} else {
end_ = null;
endBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ParseTreeInfo.LocationInfo end = 2;
*/
public cz.proto.Expression.ParseTreeInfo.LocationInfo.Builder getEndBuilder() {
onChanged();
return getEndFieldBuilder().getBuilder();
}
/**
* .cz.proto.ParseTreeInfo.LocationInfo end = 2;
*/
public cz.proto.Expression.ParseTreeInfo.LocationInfoOrBuilder getEndOrBuilder() {
if (endBuilder_ != null) {
return endBuilder_.getMessageOrBuilder();
} else {
return end_ == null ?
cz.proto.Expression.ParseTreeInfo.LocationInfo.getDefaultInstance() : end_;
}
}
/**
* .cz.proto.ParseTreeInfo.LocationInfo end = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.ParseTreeInfo.LocationInfo, cz.proto.Expression.ParseTreeInfo.LocationInfo.Builder, cz.proto.Expression.ParseTreeInfo.LocationInfoOrBuilder>
getEndFieldBuilder() {
if (endBuilder_ == null) {
endBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.ParseTreeInfo.LocationInfo, cz.proto.Expression.ParseTreeInfo.LocationInfo.Builder, cz.proto.Expression.ParseTreeInfo.LocationInfoOrBuilder>(
getEnd(),
getParentForChildren(),
isClean());
end_ = null;
}
return endBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ParseTreeInfo)
}
// @@protoc_insertion_point(class_scope:cz.proto.ParseTreeInfo)
private static final cz.proto.Expression.ParseTreeInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.Expression.ParseTreeInfo();
}
public static cz.proto.Expression.ParseTreeInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ParseTreeInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ParseTreeInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.Expression.ParseTreeInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface IntervalDayTimeOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.IntervalDayTime)
com.google.protobuf.MessageOrBuilder {
/**
* int64 seconds = 1;
* @return The seconds.
*/
long getSeconds();
/**
* int32 nanos = 2;
* @return The nanos.
*/
int getNanos();
}
/**
* Protobuf type {@code cz.proto.IntervalDayTime}
*/
public static final class IntervalDayTime extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.IntervalDayTime)
IntervalDayTimeOrBuilder {
private static final long serialVersionUID = 0L;
// Use IntervalDayTime.newBuilder() to construct.
private IntervalDayTime(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private IntervalDayTime() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new IntervalDayTime();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private IntervalDayTime(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
seconds_ = input.readInt64();
break;
}
case 16: {
nanos_ = input.readInt32();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.Expression.internal_static_cz_proto_IntervalDayTime_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.Expression.internal_static_cz_proto_IntervalDayTime_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.Expression.IntervalDayTime.class, cz.proto.Expression.IntervalDayTime.Builder.class);
}
public static final int SECONDS_FIELD_NUMBER = 1;
private long seconds_;
/**
* int64 seconds = 1;
* @return The seconds.
*/
@java.lang.Override
public long getSeconds() {
return seconds_;
}
public static final int NANOS_FIELD_NUMBER = 2;
private int nanos_;
/**
* int32 nanos = 2;
* @return The nanos.
*/
@java.lang.Override
public int getNanos() {
return nanos_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (seconds_ != 0L) {
output.writeInt64(1, seconds_);
}
if (nanos_ != 0) {
output.writeInt32(2, nanos_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (seconds_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, seconds_);
}
if (nanos_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, nanos_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.Expression.IntervalDayTime)) {
return super.equals(obj);
}
cz.proto.Expression.IntervalDayTime other = (cz.proto.Expression.IntervalDayTime) obj;
if (getSeconds()
!= other.getSeconds()) return false;
if (getNanos()
!= other.getNanos()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + SECONDS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getSeconds());
hash = (37 * hash) + NANOS_FIELD_NUMBER;
hash = (53 * hash) + getNanos();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.Expression.IntervalDayTime parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.IntervalDayTime parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.IntervalDayTime parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.IntervalDayTime parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.IntervalDayTime parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.IntervalDayTime parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.IntervalDayTime parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.Expression.IntervalDayTime parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.Expression.IntervalDayTime parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.Expression.IntervalDayTime parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.Expression.IntervalDayTime parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.Expression.IntervalDayTime parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.Expression.IntervalDayTime prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.IntervalDayTime}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.IntervalDayTime)
cz.proto.Expression.IntervalDayTimeOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.Expression.internal_static_cz_proto_IntervalDayTime_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.Expression.internal_static_cz_proto_IntervalDayTime_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.Expression.IntervalDayTime.class, cz.proto.Expression.IntervalDayTime.Builder.class);
}
// Construct using cz.proto.Expression.IntervalDayTime.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
seconds_ = 0L;
nanos_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.Expression.internal_static_cz_proto_IntervalDayTime_descriptor;
}
@java.lang.Override
public cz.proto.Expression.IntervalDayTime getDefaultInstanceForType() {
return cz.proto.Expression.IntervalDayTime.getDefaultInstance();
}
@java.lang.Override
public cz.proto.Expression.IntervalDayTime build() {
cz.proto.Expression.IntervalDayTime result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.Expression.IntervalDayTime buildPartial() {
cz.proto.Expression.IntervalDayTime result = new cz.proto.Expression.IntervalDayTime(this);
result.seconds_ = seconds_;
result.nanos_ = nanos_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.Expression.IntervalDayTime) {
return mergeFrom((cz.proto.Expression.IntervalDayTime)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.Expression.IntervalDayTime other) {
if (other == cz.proto.Expression.IntervalDayTime.getDefaultInstance()) return this;
if (other.getSeconds() != 0L) {
setSeconds(other.getSeconds());
}
if (other.getNanos() != 0) {
setNanos(other.getNanos());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.Expression.IntervalDayTime parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.Expression.IntervalDayTime) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long seconds_ ;
/**
* int64 seconds = 1;
* @return The seconds.
*/
@java.lang.Override
public long getSeconds() {
return seconds_;
}
/**
* int64 seconds = 1;
* @param value The seconds to set.
* @return This builder for chaining.
*/
public Builder setSeconds(long value) {
seconds_ = value;
onChanged();
return this;
}
/**
* int64 seconds = 1;
* @return This builder for chaining.
*/
public Builder clearSeconds() {
seconds_ = 0L;
onChanged();
return this;
}
private int nanos_ ;
/**
* int32 nanos = 2;
* @return The nanos.
*/
@java.lang.Override
public int getNanos() {
return nanos_;
}
/**
* int32 nanos = 2;
* @param value The nanos to set.
* @return This builder for chaining.
*/
public Builder setNanos(int value) {
nanos_ = value;
onChanged();
return this;
}
/**
* int32 nanos = 2;
* @return This builder for chaining.
*/
public Builder clearNanos() {
nanos_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.IntervalDayTime)
}
// @@protoc_insertion_point(class_scope:cz.proto.IntervalDayTime)
private static final cz.proto.Expression.IntervalDayTime DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.Expression.IntervalDayTime();
}
public static cz.proto.Expression.IntervalDayTime getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public IntervalDayTime parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new IntervalDayTime(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.Expression.IntervalDayTime getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ArrayValueOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ArrayValue)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .cz.proto.Constant elements = 1;
*/
java.util.List
getElementsList();
/**
* repeated .cz.proto.Constant elements = 1;
*/
cz.proto.Expression.Constant getElements(int index);
/**
* repeated .cz.proto.Constant elements = 1;
*/
int getElementsCount();
/**
* repeated .cz.proto.Constant elements = 1;
*/
java.util.List extends cz.proto.Expression.ConstantOrBuilder>
getElementsOrBuilderList();
/**
* repeated .cz.proto.Constant elements = 1;
*/
cz.proto.Expression.ConstantOrBuilder getElementsOrBuilder(
int index);
}
/**
* Protobuf type {@code cz.proto.ArrayValue}
*/
public static final class ArrayValue extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ArrayValue)
ArrayValueOrBuilder {
private static final long serialVersionUID = 0L;
// Use ArrayValue.newBuilder() to construct.
private ArrayValue(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ArrayValue() {
elements_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ArrayValue();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ArrayValue(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
elements_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
elements_.add(
input.readMessage(cz.proto.Expression.Constant.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
elements_ = java.util.Collections.unmodifiableList(elements_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.Expression.internal_static_cz_proto_ArrayValue_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.Expression.internal_static_cz_proto_ArrayValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.Expression.ArrayValue.class, cz.proto.Expression.ArrayValue.Builder.class);
}
public static final int ELEMENTS_FIELD_NUMBER = 1;
private java.util.List elements_;
/**
* repeated .cz.proto.Constant elements = 1;
*/
@java.lang.Override
public java.util.List getElementsList() {
return elements_;
}
/**
* repeated .cz.proto.Constant elements = 1;
*/
@java.lang.Override
public java.util.List extends cz.proto.Expression.ConstantOrBuilder>
getElementsOrBuilderList() {
return elements_;
}
/**
* repeated .cz.proto.Constant elements = 1;
*/
@java.lang.Override
public int getElementsCount() {
return elements_.size();
}
/**
* repeated .cz.proto.Constant elements = 1;
*/
@java.lang.Override
public cz.proto.Expression.Constant getElements(int index) {
return elements_.get(index);
}
/**
* repeated .cz.proto.Constant elements = 1;
*/
@java.lang.Override
public cz.proto.Expression.ConstantOrBuilder getElementsOrBuilder(
int index) {
return elements_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < elements_.size(); i++) {
output.writeMessage(1, elements_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < elements_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, elements_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.Expression.ArrayValue)) {
return super.equals(obj);
}
cz.proto.Expression.ArrayValue other = (cz.proto.Expression.ArrayValue) obj;
if (!getElementsList()
.equals(other.getElementsList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getElementsCount() > 0) {
hash = (37 * hash) + ELEMENTS_FIELD_NUMBER;
hash = (53 * hash) + getElementsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.Expression.ArrayValue parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.ArrayValue parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.ArrayValue parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.ArrayValue parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.ArrayValue parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.ArrayValue parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.ArrayValue parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.Expression.ArrayValue parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.Expression.ArrayValue parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.Expression.ArrayValue parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.Expression.ArrayValue parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.Expression.ArrayValue parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.Expression.ArrayValue prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ArrayValue}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ArrayValue)
cz.proto.Expression.ArrayValueOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.Expression.internal_static_cz_proto_ArrayValue_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.Expression.internal_static_cz_proto_ArrayValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.Expression.ArrayValue.class, cz.proto.Expression.ArrayValue.Builder.class);
}
// Construct using cz.proto.Expression.ArrayValue.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getElementsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (elementsBuilder_ == null) {
elements_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
elementsBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.Expression.internal_static_cz_proto_ArrayValue_descriptor;
}
@java.lang.Override
public cz.proto.Expression.ArrayValue getDefaultInstanceForType() {
return cz.proto.Expression.ArrayValue.getDefaultInstance();
}
@java.lang.Override
public cz.proto.Expression.ArrayValue build() {
cz.proto.Expression.ArrayValue result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.Expression.ArrayValue buildPartial() {
cz.proto.Expression.ArrayValue result = new cz.proto.Expression.ArrayValue(this);
int from_bitField0_ = bitField0_;
if (elementsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
elements_ = java.util.Collections.unmodifiableList(elements_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.elements_ = elements_;
} else {
result.elements_ = elementsBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.Expression.ArrayValue) {
return mergeFrom((cz.proto.Expression.ArrayValue)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.Expression.ArrayValue other) {
if (other == cz.proto.Expression.ArrayValue.getDefaultInstance()) return this;
if (elementsBuilder_ == null) {
if (!other.elements_.isEmpty()) {
if (elements_.isEmpty()) {
elements_ = other.elements_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureElementsIsMutable();
elements_.addAll(other.elements_);
}
onChanged();
}
} else {
if (!other.elements_.isEmpty()) {
if (elementsBuilder_.isEmpty()) {
elementsBuilder_.dispose();
elementsBuilder_ = null;
elements_ = other.elements_;
bitField0_ = (bitField0_ & ~0x00000001);
elementsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getElementsFieldBuilder() : null;
} else {
elementsBuilder_.addAllMessages(other.elements_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.Expression.ArrayValue parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.Expression.ArrayValue) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List elements_ =
java.util.Collections.emptyList();
private void ensureElementsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
elements_ = new java.util.ArrayList(elements_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.Expression.Constant, cz.proto.Expression.Constant.Builder, cz.proto.Expression.ConstantOrBuilder> elementsBuilder_;
/**
* repeated .cz.proto.Constant elements = 1;
*/
public java.util.List getElementsList() {
if (elementsBuilder_ == null) {
return java.util.Collections.unmodifiableList(elements_);
} else {
return elementsBuilder_.getMessageList();
}
}
/**
* repeated .cz.proto.Constant elements = 1;
*/
public int getElementsCount() {
if (elementsBuilder_ == null) {
return elements_.size();
} else {
return elementsBuilder_.getCount();
}
}
/**
* repeated .cz.proto.Constant elements = 1;
*/
public cz.proto.Expression.Constant getElements(int index) {
if (elementsBuilder_ == null) {
return elements_.get(index);
} else {
return elementsBuilder_.getMessage(index);
}
}
/**
* repeated .cz.proto.Constant elements = 1;
*/
public Builder setElements(
int index, cz.proto.Expression.Constant value) {
if (elementsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureElementsIsMutable();
elements_.set(index, value);
onChanged();
} else {
elementsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .cz.proto.Constant elements = 1;
*/
public Builder setElements(
int index, cz.proto.Expression.Constant.Builder builderForValue) {
if (elementsBuilder_ == null) {
ensureElementsIsMutable();
elements_.set(index, builderForValue.build());
onChanged();
} else {
elementsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.Constant elements = 1;
*/
public Builder addElements(cz.proto.Expression.Constant value) {
if (elementsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureElementsIsMutable();
elements_.add(value);
onChanged();
} else {
elementsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .cz.proto.Constant elements = 1;
*/
public Builder addElements(
int index, cz.proto.Expression.Constant value) {
if (elementsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureElementsIsMutable();
elements_.add(index, value);
onChanged();
} else {
elementsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .cz.proto.Constant elements = 1;
*/
public Builder addElements(
cz.proto.Expression.Constant.Builder builderForValue) {
if (elementsBuilder_ == null) {
ensureElementsIsMutable();
elements_.add(builderForValue.build());
onChanged();
} else {
elementsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.Constant elements = 1;
*/
public Builder addElements(
int index, cz.proto.Expression.Constant.Builder builderForValue) {
if (elementsBuilder_ == null) {
ensureElementsIsMutable();
elements_.add(index, builderForValue.build());
onChanged();
} else {
elementsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.Constant elements = 1;
*/
public Builder addAllElements(
java.lang.Iterable extends cz.proto.Expression.Constant> values) {
if (elementsBuilder_ == null) {
ensureElementsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, elements_);
onChanged();
} else {
elementsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .cz.proto.Constant elements = 1;
*/
public Builder clearElements() {
if (elementsBuilder_ == null) {
elements_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
elementsBuilder_.clear();
}
return this;
}
/**
* repeated .cz.proto.Constant elements = 1;
*/
public Builder removeElements(int index) {
if (elementsBuilder_ == null) {
ensureElementsIsMutable();
elements_.remove(index);
onChanged();
} else {
elementsBuilder_.remove(index);
}
return this;
}
/**
* repeated .cz.proto.Constant elements = 1;
*/
public cz.proto.Expression.Constant.Builder getElementsBuilder(
int index) {
return getElementsFieldBuilder().getBuilder(index);
}
/**
* repeated .cz.proto.Constant elements = 1;
*/
public cz.proto.Expression.ConstantOrBuilder getElementsOrBuilder(
int index) {
if (elementsBuilder_ == null) {
return elements_.get(index); } else {
return elementsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .cz.proto.Constant elements = 1;
*/
public java.util.List extends cz.proto.Expression.ConstantOrBuilder>
getElementsOrBuilderList() {
if (elementsBuilder_ != null) {
return elementsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(elements_);
}
}
/**
* repeated .cz.proto.Constant elements = 1;
*/
public cz.proto.Expression.Constant.Builder addElementsBuilder() {
return getElementsFieldBuilder().addBuilder(
cz.proto.Expression.Constant.getDefaultInstance());
}
/**
* repeated .cz.proto.Constant elements = 1;
*/
public cz.proto.Expression.Constant.Builder addElementsBuilder(
int index) {
return getElementsFieldBuilder().addBuilder(
index, cz.proto.Expression.Constant.getDefaultInstance());
}
/**
* repeated .cz.proto.Constant elements = 1;
*/
public java.util.List
getElementsBuilderList() {
return getElementsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.Expression.Constant, cz.proto.Expression.Constant.Builder, cz.proto.Expression.ConstantOrBuilder>
getElementsFieldBuilder() {
if (elementsBuilder_ == null) {
elementsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.Expression.Constant, cz.proto.Expression.Constant.Builder, cz.proto.Expression.ConstantOrBuilder>(
elements_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
elements_ = null;
}
return elementsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ArrayValue)
}
// @@protoc_insertion_point(class_scope:cz.proto.ArrayValue)
private static final cz.proto.Expression.ArrayValue DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.Expression.ArrayValue();
}
public static cz.proto.Expression.ArrayValue getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ArrayValue parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ArrayValue(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.Expression.ArrayValue getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MapValueOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.MapValue)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .cz.proto.Constant keys = 1;
*/
java.util.List
getKeysList();
/**
* repeated .cz.proto.Constant keys = 1;
*/
cz.proto.Expression.Constant getKeys(int index);
/**
* repeated .cz.proto.Constant keys = 1;
*/
int getKeysCount();
/**
* repeated .cz.proto.Constant keys = 1;
*/
java.util.List extends cz.proto.Expression.ConstantOrBuilder>
getKeysOrBuilderList();
/**
* repeated .cz.proto.Constant keys = 1;
*/
cz.proto.Expression.ConstantOrBuilder getKeysOrBuilder(
int index);
/**
* repeated .cz.proto.Constant values = 2;
*/
java.util.List
getValuesList();
/**
* repeated .cz.proto.Constant values = 2;
*/
cz.proto.Expression.Constant getValues(int index);
/**
* repeated .cz.proto.Constant values = 2;
*/
int getValuesCount();
/**
* repeated .cz.proto.Constant values = 2;
*/
java.util.List extends cz.proto.Expression.ConstantOrBuilder>
getValuesOrBuilderList();
/**
* repeated .cz.proto.Constant values = 2;
*/
cz.proto.Expression.ConstantOrBuilder getValuesOrBuilder(
int index);
}
/**
* Protobuf type {@code cz.proto.MapValue}
*/
public static final class MapValue extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.MapValue)
MapValueOrBuilder {
private static final long serialVersionUID = 0L;
// Use MapValue.newBuilder() to construct.
private MapValue(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MapValue() {
keys_ = java.util.Collections.emptyList();
values_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new MapValue();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private MapValue(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
keys_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
keys_.add(
input.readMessage(cz.proto.Expression.Constant.parser(), extensionRegistry));
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
values_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
values_.add(
input.readMessage(cz.proto.Expression.Constant.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
keys_ = java.util.Collections.unmodifiableList(keys_);
}
if (((mutable_bitField0_ & 0x00000002) != 0)) {
values_ = java.util.Collections.unmodifiableList(values_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.Expression.internal_static_cz_proto_MapValue_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.Expression.internal_static_cz_proto_MapValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.Expression.MapValue.class, cz.proto.Expression.MapValue.Builder.class);
}
public static final int KEYS_FIELD_NUMBER = 1;
private java.util.List keys_;
/**
* repeated .cz.proto.Constant keys = 1;
*/
@java.lang.Override
public java.util.List getKeysList() {
return keys_;
}
/**
* repeated .cz.proto.Constant keys = 1;
*/
@java.lang.Override
public java.util.List extends cz.proto.Expression.ConstantOrBuilder>
getKeysOrBuilderList() {
return keys_;
}
/**
* repeated .cz.proto.Constant keys = 1;
*/
@java.lang.Override
public int getKeysCount() {
return keys_.size();
}
/**
* repeated .cz.proto.Constant keys = 1;
*/
@java.lang.Override
public cz.proto.Expression.Constant getKeys(int index) {
return keys_.get(index);
}
/**
* repeated .cz.proto.Constant keys = 1;
*/
@java.lang.Override
public cz.proto.Expression.ConstantOrBuilder getKeysOrBuilder(
int index) {
return keys_.get(index);
}
public static final int VALUES_FIELD_NUMBER = 2;
private java.util.List values_;
/**
* repeated .cz.proto.Constant values = 2;
*/
@java.lang.Override
public java.util.List getValuesList() {
return values_;
}
/**
* repeated .cz.proto.Constant values = 2;
*/
@java.lang.Override
public java.util.List extends cz.proto.Expression.ConstantOrBuilder>
getValuesOrBuilderList() {
return values_;
}
/**
* repeated .cz.proto.Constant values = 2;
*/
@java.lang.Override
public int getValuesCount() {
return values_.size();
}
/**
* repeated .cz.proto.Constant values = 2;
*/
@java.lang.Override
public cz.proto.Expression.Constant getValues(int index) {
return values_.get(index);
}
/**
* repeated .cz.proto.Constant values = 2;
*/
@java.lang.Override
public cz.proto.Expression.ConstantOrBuilder getValuesOrBuilder(
int index) {
return values_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < keys_.size(); i++) {
output.writeMessage(1, keys_.get(i));
}
for (int i = 0; i < values_.size(); i++) {
output.writeMessage(2, values_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < keys_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, keys_.get(i));
}
for (int i = 0; i < values_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, values_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.Expression.MapValue)) {
return super.equals(obj);
}
cz.proto.Expression.MapValue other = (cz.proto.Expression.MapValue) obj;
if (!getKeysList()
.equals(other.getKeysList())) return false;
if (!getValuesList()
.equals(other.getValuesList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getKeysCount() > 0) {
hash = (37 * hash) + KEYS_FIELD_NUMBER;
hash = (53 * hash) + getKeysList().hashCode();
}
if (getValuesCount() > 0) {
hash = (37 * hash) + VALUES_FIELD_NUMBER;
hash = (53 * hash) + getValuesList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.Expression.MapValue parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.MapValue parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.MapValue parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.MapValue parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.MapValue parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.MapValue parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.MapValue parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.Expression.MapValue parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.Expression.MapValue parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.Expression.MapValue parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.Expression.MapValue parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.Expression.MapValue parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.Expression.MapValue prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.MapValue}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.MapValue)
cz.proto.Expression.MapValueOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.Expression.internal_static_cz_proto_MapValue_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.Expression.internal_static_cz_proto_MapValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.Expression.MapValue.class, cz.proto.Expression.MapValue.Builder.class);
}
// Construct using cz.proto.Expression.MapValue.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getKeysFieldBuilder();
getValuesFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (keysBuilder_ == null) {
keys_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
keysBuilder_.clear();
}
if (valuesBuilder_ == null) {
values_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
valuesBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.Expression.internal_static_cz_proto_MapValue_descriptor;
}
@java.lang.Override
public cz.proto.Expression.MapValue getDefaultInstanceForType() {
return cz.proto.Expression.MapValue.getDefaultInstance();
}
@java.lang.Override
public cz.proto.Expression.MapValue build() {
cz.proto.Expression.MapValue result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.Expression.MapValue buildPartial() {
cz.proto.Expression.MapValue result = new cz.proto.Expression.MapValue(this);
int from_bitField0_ = bitField0_;
if (keysBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
keys_ = java.util.Collections.unmodifiableList(keys_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.keys_ = keys_;
} else {
result.keys_ = keysBuilder_.build();
}
if (valuesBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)) {
values_ = java.util.Collections.unmodifiableList(values_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.values_ = values_;
} else {
result.values_ = valuesBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.Expression.MapValue) {
return mergeFrom((cz.proto.Expression.MapValue)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.Expression.MapValue other) {
if (other == cz.proto.Expression.MapValue.getDefaultInstance()) return this;
if (keysBuilder_ == null) {
if (!other.keys_.isEmpty()) {
if (keys_.isEmpty()) {
keys_ = other.keys_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureKeysIsMutable();
keys_.addAll(other.keys_);
}
onChanged();
}
} else {
if (!other.keys_.isEmpty()) {
if (keysBuilder_.isEmpty()) {
keysBuilder_.dispose();
keysBuilder_ = null;
keys_ = other.keys_;
bitField0_ = (bitField0_ & ~0x00000001);
keysBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getKeysFieldBuilder() : null;
} else {
keysBuilder_.addAllMessages(other.keys_);
}
}
}
if (valuesBuilder_ == null) {
if (!other.values_.isEmpty()) {
if (values_.isEmpty()) {
values_ = other.values_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureValuesIsMutable();
values_.addAll(other.values_);
}
onChanged();
}
} else {
if (!other.values_.isEmpty()) {
if (valuesBuilder_.isEmpty()) {
valuesBuilder_.dispose();
valuesBuilder_ = null;
values_ = other.values_;
bitField0_ = (bitField0_ & ~0x00000002);
valuesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getValuesFieldBuilder() : null;
} else {
valuesBuilder_.addAllMessages(other.values_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.Expression.MapValue parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.Expression.MapValue) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List keys_ =
java.util.Collections.emptyList();
private void ensureKeysIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
keys_ = new java.util.ArrayList(keys_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.Expression.Constant, cz.proto.Expression.Constant.Builder, cz.proto.Expression.ConstantOrBuilder> keysBuilder_;
/**
* repeated .cz.proto.Constant keys = 1;
*/
public java.util.List getKeysList() {
if (keysBuilder_ == null) {
return java.util.Collections.unmodifiableList(keys_);
} else {
return keysBuilder_.getMessageList();
}
}
/**
* repeated .cz.proto.Constant keys = 1;
*/
public int getKeysCount() {
if (keysBuilder_ == null) {
return keys_.size();
} else {
return keysBuilder_.getCount();
}
}
/**
* repeated .cz.proto.Constant keys = 1;
*/
public cz.proto.Expression.Constant getKeys(int index) {
if (keysBuilder_ == null) {
return keys_.get(index);
} else {
return keysBuilder_.getMessage(index);
}
}
/**
* repeated .cz.proto.Constant keys = 1;
*/
public Builder setKeys(
int index, cz.proto.Expression.Constant value) {
if (keysBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureKeysIsMutable();
keys_.set(index, value);
onChanged();
} else {
keysBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .cz.proto.Constant keys = 1;
*/
public Builder setKeys(
int index, cz.proto.Expression.Constant.Builder builderForValue) {
if (keysBuilder_ == null) {
ensureKeysIsMutable();
keys_.set(index, builderForValue.build());
onChanged();
} else {
keysBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.Constant keys = 1;
*/
public Builder addKeys(cz.proto.Expression.Constant value) {
if (keysBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureKeysIsMutable();
keys_.add(value);
onChanged();
} else {
keysBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .cz.proto.Constant keys = 1;
*/
public Builder addKeys(
int index, cz.proto.Expression.Constant value) {
if (keysBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureKeysIsMutable();
keys_.add(index, value);
onChanged();
} else {
keysBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .cz.proto.Constant keys = 1;
*/
public Builder addKeys(
cz.proto.Expression.Constant.Builder builderForValue) {
if (keysBuilder_ == null) {
ensureKeysIsMutable();
keys_.add(builderForValue.build());
onChanged();
} else {
keysBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.Constant keys = 1;
*/
public Builder addKeys(
int index, cz.proto.Expression.Constant.Builder builderForValue) {
if (keysBuilder_ == null) {
ensureKeysIsMutable();
keys_.add(index, builderForValue.build());
onChanged();
} else {
keysBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.Constant keys = 1;
*/
public Builder addAllKeys(
java.lang.Iterable extends cz.proto.Expression.Constant> values) {
if (keysBuilder_ == null) {
ensureKeysIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, keys_);
onChanged();
} else {
keysBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .cz.proto.Constant keys = 1;
*/
public Builder clearKeys() {
if (keysBuilder_ == null) {
keys_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
keysBuilder_.clear();
}
return this;
}
/**
* repeated .cz.proto.Constant keys = 1;
*/
public Builder removeKeys(int index) {
if (keysBuilder_ == null) {
ensureKeysIsMutable();
keys_.remove(index);
onChanged();
} else {
keysBuilder_.remove(index);
}
return this;
}
/**
* repeated .cz.proto.Constant keys = 1;
*/
public cz.proto.Expression.Constant.Builder getKeysBuilder(
int index) {
return getKeysFieldBuilder().getBuilder(index);
}
/**
* repeated .cz.proto.Constant keys = 1;
*/
public cz.proto.Expression.ConstantOrBuilder getKeysOrBuilder(
int index) {
if (keysBuilder_ == null) {
return keys_.get(index); } else {
return keysBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .cz.proto.Constant keys = 1;
*/
public java.util.List extends cz.proto.Expression.ConstantOrBuilder>
getKeysOrBuilderList() {
if (keysBuilder_ != null) {
return keysBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(keys_);
}
}
/**
* repeated .cz.proto.Constant keys = 1;
*/
public cz.proto.Expression.Constant.Builder addKeysBuilder() {
return getKeysFieldBuilder().addBuilder(
cz.proto.Expression.Constant.getDefaultInstance());
}
/**
* repeated .cz.proto.Constant keys = 1;
*/
public cz.proto.Expression.Constant.Builder addKeysBuilder(
int index) {
return getKeysFieldBuilder().addBuilder(
index, cz.proto.Expression.Constant.getDefaultInstance());
}
/**
* repeated .cz.proto.Constant keys = 1;
*/
public java.util.List
getKeysBuilderList() {
return getKeysFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.Expression.Constant, cz.proto.Expression.Constant.Builder, cz.proto.Expression.ConstantOrBuilder>
getKeysFieldBuilder() {
if (keysBuilder_ == null) {
keysBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.Expression.Constant, cz.proto.Expression.Constant.Builder, cz.proto.Expression.ConstantOrBuilder>(
keys_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
keys_ = null;
}
return keysBuilder_;
}
private java.util.List values_ =
java.util.Collections.emptyList();
private void ensureValuesIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
values_ = new java.util.ArrayList(values_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.Expression.Constant, cz.proto.Expression.Constant.Builder, cz.proto.Expression.ConstantOrBuilder> valuesBuilder_;
/**
* repeated .cz.proto.Constant values = 2;
*/
public java.util.List getValuesList() {
if (valuesBuilder_ == null) {
return java.util.Collections.unmodifiableList(values_);
} else {
return valuesBuilder_.getMessageList();
}
}
/**
* repeated .cz.proto.Constant values = 2;
*/
public int getValuesCount() {
if (valuesBuilder_ == null) {
return values_.size();
} else {
return valuesBuilder_.getCount();
}
}
/**
* repeated .cz.proto.Constant values = 2;
*/
public cz.proto.Expression.Constant getValues(int index) {
if (valuesBuilder_ == null) {
return values_.get(index);
} else {
return valuesBuilder_.getMessage(index);
}
}
/**
* repeated .cz.proto.Constant values = 2;
*/
public Builder setValues(
int index, cz.proto.Expression.Constant value) {
if (valuesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureValuesIsMutable();
values_.set(index, value);
onChanged();
} else {
valuesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .cz.proto.Constant values = 2;
*/
public Builder setValues(
int index, cz.proto.Expression.Constant.Builder builderForValue) {
if (valuesBuilder_ == null) {
ensureValuesIsMutable();
values_.set(index, builderForValue.build());
onChanged();
} else {
valuesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.Constant values = 2;
*/
public Builder addValues(cz.proto.Expression.Constant value) {
if (valuesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureValuesIsMutable();
values_.add(value);
onChanged();
} else {
valuesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .cz.proto.Constant values = 2;
*/
public Builder addValues(
int index, cz.proto.Expression.Constant value) {
if (valuesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureValuesIsMutable();
values_.add(index, value);
onChanged();
} else {
valuesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .cz.proto.Constant values = 2;
*/
public Builder addValues(
cz.proto.Expression.Constant.Builder builderForValue) {
if (valuesBuilder_ == null) {
ensureValuesIsMutable();
values_.add(builderForValue.build());
onChanged();
} else {
valuesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.Constant values = 2;
*/
public Builder addValues(
int index, cz.proto.Expression.Constant.Builder builderForValue) {
if (valuesBuilder_ == null) {
ensureValuesIsMutable();
values_.add(index, builderForValue.build());
onChanged();
} else {
valuesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.Constant values = 2;
*/
public Builder addAllValues(
java.lang.Iterable extends cz.proto.Expression.Constant> values) {
if (valuesBuilder_ == null) {
ensureValuesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, values_);
onChanged();
} else {
valuesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .cz.proto.Constant values = 2;
*/
public Builder clearValues() {
if (valuesBuilder_ == null) {
values_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
valuesBuilder_.clear();
}
return this;
}
/**
* repeated .cz.proto.Constant values = 2;
*/
public Builder removeValues(int index) {
if (valuesBuilder_ == null) {
ensureValuesIsMutable();
values_.remove(index);
onChanged();
} else {
valuesBuilder_.remove(index);
}
return this;
}
/**
* repeated .cz.proto.Constant values = 2;
*/
public cz.proto.Expression.Constant.Builder getValuesBuilder(
int index) {
return getValuesFieldBuilder().getBuilder(index);
}
/**
* repeated .cz.proto.Constant values = 2;
*/
public cz.proto.Expression.ConstantOrBuilder getValuesOrBuilder(
int index) {
if (valuesBuilder_ == null) {
return values_.get(index); } else {
return valuesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .cz.proto.Constant values = 2;
*/
public java.util.List extends cz.proto.Expression.ConstantOrBuilder>
getValuesOrBuilderList() {
if (valuesBuilder_ != null) {
return valuesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(values_);
}
}
/**
* repeated .cz.proto.Constant values = 2;
*/
public cz.proto.Expression.Constant.Builder addValuesBuilder() {
return getValuesFieldBuilder().addBuilder(
cz.proto.Expression.Constant.getDefaultInstance());
}
/**
* repeated .cz.proto.Constant values = 2;
*/
public cz.proto.Expression.Constant.Builder addValuesBuilder(
int index) {
return getValuesFieldBuilder().addBuilder(
index, cz.proto.Expression.Constant.getDefaultInstance());
}
/**
* repeated .cz.proto.Constant values = 2;
*/
public java.util.List
getValuesBuilderList() {
return getValuesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.Expression.Constant, cz.proto.Expression.Constant.Builder, cz.proto.Expression.ConstantOrBuilder>
getValuesFieldBuilder() {
if (valuesBuilder_ == null) {
valuesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.Expression.Constant, cz.proto.Expression.Constant.Builder, cz.proto.Expression.ConstantOrBuilder>(
values_,
((bitField0_ & 0x00000002) != 0),
getParentForChildren(),
isClean());
values_ = null;
}
return valuesBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.MapValue)
}
// @@protoc_insertion_point(class_scope:cz.proto.MapValue)
private static final cz.proto.Expression.MapValue DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.Expression.MapValue();
}
public static cz.proto.Expression.MapValue getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MapValue parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new MapValue(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.Expression.MapValue getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface StructValueOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.StructValue)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .cz.proto.Constant fields = 1;
*/
java.util.List
getFieldsList();
/**
* repeated .cz.proto.Constant fields = 1;
*/
cz.proto.Expression.Constant getFields(int index);
/**
* repeated .cz.proto.Constant fields = 1;
*/
int getFieldsCount();
/**
* repeated .cz.proto.Constant fields = 1;
*/
java.util.List extends cz.proto.Expression.ConstantOrBuilder>
getFieldsOrBuilderList();
/**
* repeated .cz.proto.Constant fields = 1;
*/
cz.proto.Expression.ConstantOrBuilder getFieldsOrBuilder(
int index);
}
/**
* Protobuf type {@code cz.proto.StructValue}
*/
public static final class StructValue extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.StructValue)
StructValueOrBuilder {
private static final long serialVersionUID = 0L;
// Use StructValue.newBuilder() to construct.
private StructValue(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private StructValue() {
fields_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new StructValue();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private StructValue(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
fields_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
fields_.add(
input.readMessage(cz.proto.Expression.Constant.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
fields_ = java.util.Collections.unmodifiableList(fields_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.Expression.internal_static_cz_proto_StructValue_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.Expression.internal_static_cz_proto_StructValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.Expression.StructValue.class, cz.proto.Expression.StructValue.Builder.class);
}
public static final int FIELDS_FIELD_NUMBER = 1;
private java.util.List fields_;
/**
* repeated .cz.proto.Constant fields = 1;
*/
@java.lang.Override
public java.util.List getFieldsList() {
return fields_;
}
/**
* repeated .cz.proto.Constant fields = 1;
*/
@java.lang.Override
public java.util.List extends cz.proto.Expression.ConstantOrBuilder>
getFieldsOrBuilderList() {
return fields_;
}
/**
* repeated .cz.proto.Constant fields = 1;
*/
@java.lang.Override
public int getFieldsCount() {
return fields_.size();
}
/**
* repeated .cz.proto.Constant fields = 1;
*/
@java.lang.Override
public cz.proto.Expression.Constant getFields(int index) {
return fields_.get(index);
}
/**
* repeated .cz.proto.Constant fields = 1;
*/
@java.lang.Override
public cz.proto.Expression.ConstantOrBuilder getFieldsOrBuilder(
int index) {
return fields_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < fields_.size(); i++) {
output.writeMessage(1, fields_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < fields_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, fields_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.Expression.StructValue)) {
return super.equals(obj);
}
cz.proto.Expression.StructValue other = (cz.proto.Expression.StructValue) obj;
if (!getFieldsList()
.equals(other.getFieldsList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getFieldsCount() > 0) {
hash = (37 * hash) + FIELDS_FIELD_NUMBER;
hash = (53 * hash) + getFieldsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.Expression.StructValue parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.StructValue parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.StructValue parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.StructValue parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.StructValue parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.StructValue parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.StructValue parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.Expression.StructValue parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.Expression.StructValue parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.Expression.StructValue parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.Expression.StructValue parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.Expression.StructValue parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.Expression.StructValue prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.StructValue}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.StructValue)
cz.proto.Expression.StructValueOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.Expression.internal_static_cz_proto_StructValue_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.Expression.internal_static_cz_proto_StructValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.Expression.StructValue.class, cz.proto.Expression.StructValue.Builder.class);
}
// Construct using cz.proto.Expression.StructValue.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getFieldsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (fieldsBuilder_ == null) {
fields_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
fieldsBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.Expression.internal_static_cz_proto_StructValue_descriptor;
}
@java.lang.Override
public cz.proto.Expression.StructValue getDefaultInstanceForType() {
return cz.proto.Expression.StructValue.getDefaultInstance();
}
@java.lang.Override
public cz.proto.Expression.StructValue build() {
cz.proto.Expression.StructValue result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.Expression.StructValue buildPartial() {
cz.proto.Expression.StructValue result = new cz.proto.Expression.StructValue(this);
int from_bitField0_ = bitField0_;
if (fieldsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
fields_ = java.util.Collections.unmodifiableList(fields_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.fields_ = fields_;
} else {
result.fields_ = fieldsBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.Expression.StructValue) {
return mergeFrom((cz.proto.Expression.StructValue)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.Expression.StructValue other) {
if (other == cz.proto.Expression.StructValue.getDefaultInstance()) return this;
if (fieldsBuilder_ == null) {
if (!other.fields_.isEmpty()) {
if (fields_.isEmpty()) {
fields_ = other.fields_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureFieldsIsMutable();
fields_.addAll(other.fields_);
}
onChanged();
}
} else {
if (!other.fields_.isEmpty()) {
if (fieldsBuilder_.isEmpty()) {
fieldsBuilder_.dispose();
fieldsBuilder_ = null;
fields_ = other.fields_;
bitField0_ = (bitField0_ & ~0x00000001);
fieldsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getFieldsFieldBuilder() : null;
} else {
fieldsBuilder_.addAllMessages(other.fields_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.Expression.StructValue parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.Expression.StructValue) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List fields_ =
java.util.Collections.emptyList();
private void ensureFieldsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
fields_ = new java.util.ArrayList(fields_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.Expression.Constant, cz.proto.Expression.Constant.Builder, cz.proto.Expression.ConstantOrBuilder> fieldsBuilder_;
/**
* repeated .cz.proto.Constant fields = 1;
*/
public java.util.List getFieldsList() {
if (fieldsBuilder_ == null) {
return java.util.Collections.unmodifiableList(fields_);
} else {
return fieldsBuilder_.getMessageList();
}
}
/**
* repeated .cz.proto.Constant fields = 1;
*/
public int getFieldsCount() {
if (fieldsBuilder_ == null) {
return fields_.size();
} else {
return fieldsBuilder_.getCount();
}
}
/**
* repeated .cz.proto.Constant fields = 1;
*/
public cz.proto.Expression.Constant getFields(int index) {
if (fieldsBuilder_ == null) {
return fields_.get(index);
} else {
return fieldsBuilder_.getMessage(index);
}
}
/**
* repeated .cz.proto.Constant fields = 1;
*/
public Builder setFields(
int index, cz.proto.Expression.Constant value) {
if (fieldsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFieldsIsMutable();
fields_.set(index, value);
onChanged();
} else {
fieldsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .cz.proto.Constant fields = 1;
*/
public Builder setFields(
int index, cz.proto.Expression.Constant.Builder builderForValue) {
if (fieldsBuilder_ == null) {
ensureFieldsIsMutable();
fields_.set(index, builderForValue.build());
onChanged();
} else {
fieldsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.Constant fields = 1;
*/
public Builder addFields(cz.proto.Expression.Constant value) {
if (fieldsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFieldsIsMutable();
fields_.add(value);
onChanged();
} else {
fieldsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .cz.proto.Constant fields = 1;
*/
public Builder addFields(
int index, cz.proto.Expression.Constant value) {
if (fieldsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFieldsIsMutable();
fields_.add(index, value);
onChanged();
} else {
fieldsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .cz.proto.Constant fields = 1;
*/
public Builder addFields(
cz.proto.Expression.Constant.Builder builderForValue) {
if (fieldsBuilder_ == null) {
ensureFieldsIsMutable();
fields_.add(builderForValue.build());
onChanged();
} else {
fieldsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.Constant fields = 1;
*/
public Builder addFields(
int index, cz.proto.Expression.Constant.Builder builderForValue) {
if (fieldsBuilder_ == null) {
ensureFieldsIsMutable();
fields_.add(index, builderForValue.build());
onChanged();
} else {
fieldsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.Constant fields = 1;
*/
public Builder addAllFields(
java.lang.Iterable extends cz.proto.Expression.Constant> values) {
if (fieldsBuilder_ == null) {
ensureFieldsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, fields_);
onChanged();
} else {
fieldsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .cz.proto.Constant fields = 1;
*/
public Builder clearFields() {
if (fieldsBuilder_ == null) {
fields_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
fieldsBuilder_.clear();
}
return this;
}
/**
* repeated .cz.proto.Constant fields = 1;
*/
public Builder removeFields(int index) {
if (fieldsBuilder_ == null) {
ensureFieldsIsMutable();
fields_.remove(index);
onChanged();
} else {
fieldsBuilder_.remove(index);
}
return this;
}
/**
* repeated .cz.proto.Constant fields = 1;
*/
public cz.proto.Expression.Constant.Builder getFieldsBuilder(
int index) {
return getFieldsFieldBuilder().getBuilder(index);
}
/**
* repeated .cz.proto.Constant fields = 1;
*/
public cz.proto.Expression.ConstantOrBuilder getFieldsOrBuilder(
int index) {
if (fieldsBuilder_ == null) {
return fields_.get(index); } else {
return fieldsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .cz.proto.Constant fields = 1;
*/
public java.util.List extends cz.proto.Expression.ConstantOrBuilder>
getFieldsOrBuilderList() {
if (fieldsBuilder_ != null) {
return fieldsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(fields_);
}
}
/**
* repeated .cz.proto.Constant fields = 1;
*/
public cz.proto.Expression.Constant.Builder addFieldsBuilder() {
return getFieldsFieldBuilder().addBuilder(
cz.proto.Expression.Constant.getDefaultInstance());
}
/**
* repeated .cz.proto.Constant fields = 1;
*/
public cz.proto.Expression.Constant.Builder addFieldsBuilder(
int index) {
return getFieldsFieldBuilder().addBuilder(
index, cz.proto.Expression.Constant.getDefaultInstance());
}
/**
* repeated .cz.proto.Constant fields = 1;
*/
public java.util.List
getFieldsBuilderList() {
return getFieldsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.Expression.Constant, cz.proto.Expression.Constant.Builder, cz.proto.Expression.ConstantOrBuilder>
getFieldsFieldBuilder() {
if (fieldsBuilder_ == null) {
fieldsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.Expression.Constant, cz.proto.Expression.Constant.Builder, cz.proto.Expression.ConstantOrBuilder>(
fields_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
fields_ = null;
}
return fieldsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.StructValue)
}
// @@protoc_insertion_point(class_scope:cz.proto.StructValue)
private static final cz.proto.Expression.StructValue DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.Expression.StructValue();
}
public static cz.proto.Expression.StructValue getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public StructValue parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new StructValue(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.Expression.StructValue getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface BitmapValueOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.BitmapValue)
com.google.protobuf.MessageOrBuilder {
/**
* bytes value = 1;
* @return The value.
*/
com.google.protobuf.ByteString getValue();
}
/**
* Protobuf type {@code cz.proto.BitmapValue}
*/
public static final class BitmapValue extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.BitmapValue)
BitmapValueOrBuilder {
private static final long serialVersionUID = 0L;
// Use BitmapValue.newBuilder() to construct.
private BitmapValue(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private BitmapValue() {
value_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new BitmapValue();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private BitmapValue(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
value_ = input.readBytes();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.Expression.internal_static_cz_proto_BitmapValue_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.Expression.internal_static_cz_proto_BitmapValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.Expression.BitmapValue.class, cz.proto.Expression.BitmapValue.Builder.class);
}
public static final int VALUE_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString value_;
/**
* bytes value = 1;
* @return The value.
*/
@java.lang.Override
public com.google.protobuf.ByteString getValue() {
return value_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!value_.isEmpty()) {
output.writeBytes(1, value_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!value_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, value_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.Expression.BitmapValue)) {
return super.equals(obj);
}
cz.proto.Expression.BitmapValue other = (cz.proto.Expression.BitmapValue) obj;
if (!getValue()
.equals(other.getValue())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + VALUE_FIELD_NUMBER;
hash = (53 * hash) + getValue().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.Expression.BitmapValue parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.BitmapValue parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.BitmapValue parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.BitmapValue parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.BitmapValue parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.BitmapValue parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.BitmapValue parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.Expression.BitmapValue parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.Expression.BitmapValue parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.Expression.BitmapValue parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.Expression.BitmapValue parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.Expression.BitmapValue parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.Expression.BitmapValue prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.BitmapValue}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.BitmapValue)
cz.proto.Expression.BitmapValueOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.Expression.internal_static_cz_proto_BitmapValue_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.Expression.internal_static_cz_proto_BitmapValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.Expression.BitmapValue.class, cz.proto.Expression.BitmapValue.Builder.class);
}
// Construct using cz.proto.Expression.BitmapValue.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
value_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.Expression.internal_static_cz_proto_BitmapValue_descriptor;
}
@java.lang.Override
public cz.proto.Expression.BitmapValue getDefaultInstanceForType() {
return cz.proto.Expression.BitmapValue.getDefaultInstance();
}
@java.lang.Override
public cz.proto.Expression.BitmapValue build() {
cz.proto.Expression.BitmapValue result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.Expression.BitmapValue buildPartial() {
cz.proto.Expression.BitmapValue result = new cz.proto.Expression.BitmapValue(this);
result.value_ = value_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.Expression.BitmapValue) {
return mergeFrom((cz.proto.Expression.BitmapValue)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.Expression.BitmapValue other) {
if (other == cz.proto.Expression.BitmapValue.getDefaultInstance()) return this;
if (other.getValue() != com.google.protobuf.ByteString.EMPTY) {
setValue(other.getValue());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.Expression.BitmapValue parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.Expression.BitmapValue) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private com.google.protobuf.ByteString value_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes value = 1;
* @return The value.
*/
@java.lang.Override
public com.google.protobuf.ByteString getValue() {
return value_;
}
/**
* bytes value = 1;
* @param value The value to set.
* @return This builder for chaining.
*/
public Builder setValue(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
value_ = value;
onChanged();
return this;
}
/**
* bytes value = 1;
* @return This builder for chaining.
*/
public Builder clearValue() {
value_ = getDefaultInstance().getValue();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.BitmapValue)
}
// @@protoc_insertion_point(class_scope:cz.proto.BitmapValue)
private static final cz.proto.Expression.BitmapValue DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.Expression.BitmapValue();
}
public static cz.proto.Expression.BitmapValue getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public BitmapValue parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new BitmapValue(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.Expression.BitmapValue getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ConstantOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.Constant)
com.google.protobuf.MessageOrBuilder {
/**
* bool null = 1;
* @return Whether the null field is set.
*/
boolean hasNull();
/**
* bool null = 1;
* @return The null.
*/
boolean getNull();
/**
* int32 tinyint = 2;
* @return Whether the tinyint field is set.
*/
boolean hasTinyint();
/**
* int32 tinyint = 2;
* @return The tinyint.
*/
int getTinyint();
/**
* int32 smallInt = 3;
* @return Whether the smallInt field is set.
*/
boolean hasSmallInt();
/**
* int32 smallInt = 3;
* @return The smallInt.
*/
int getSmallInt();
/**
* int32 int = 4;
* @return Whether the int field is set.
*/
boolean hasInt();
/**
* int32 int = 4;
* @return The int.
*/
int getInt();
/**
* int64 bigint = 5;
* @return Whether the bigint field is set.
*/
boolean hasBigint();
/**
* int64 bigint = 5;
* @return The bigint.
*/
long getBigint();
/**
* float float = 6;
* @return Whether the float field is set.
*/
boolean hasFloat();
/**
* float float = 6;
* @return The float.
*/
float getFloat();
/**
* double double = 7;
* @return Whether the double field is set.
*/
boolean hasDouble();
/**
* double double = 7;
* @return The double.
*/
double getDouble();
/**
* string decimal = 8;
* @return Whether the decimal field is set.
*/
boolean hasDecimal();
/**
* string decimal = 8;
* @return The decimal.
*/
java.lang.String getDecimal();
/**
* string decimal = 8;
* @return The bytes for decimal.
*/
com.google.protobuf.ByteString
getDecimalBytes();
/**
* bool boolean = 9;
* @return Whether the boolean field is set.
*/
boolean hasBoolean();
/**
* bool boolean = 9;
* @return The boolean.
*/
boolean getBoolean();
/**
* string char = 10;
* @return Whether the char field is set.
*/
boolean hasChar();
/**
* string char = 10;
* @return The char.
*/
java.lang.String getChar();
/**
* string char = 10;
* @return The bytes for char.
*/
com.google.protobuf.ByteString
getCharBytes();
/**
* string varchar = 11;
* @return Whether the varchar field is set.
*/
boolean hasVarchar();
/**
* string varchar = 11;
* @return The varchar.
*/
java.lang.String getVarchar();
/**
* string varchar = 11;
* @return The bytes for varchar.
*/
com.google.protobuf.ByteString
getVarcharBytes();
/**
* string string = 12;
* @return Whether the string field is set.
*/
boolean hasString();
/**
* string string = 12;
* @return The string.
*/
java.lang.String getString();
/**
* string string = 12;
* @return The bytes for string.
*/
com.google.protobuf.ByteString
getStringBytes();
/**
* bytes binary = 13;
* @return Whether the binary field is set.
*/
boolean hasBinary();
/**
* bytes binary = 13;
* @return The binary.
*/
com.google.protobuf.ByteString getBinary();
/**
* int32 date = 14;
* @return Whether the date field is set.
*/
boolean hasDate();
/**
* int32 date = 14;
* @return The date.
*/
int getDate();
/**
* int64 timestamp = 15;
* @return Whether the timestamp field is set.
*/
boolean hasTimestamp();
/**
* int64 timestamp = 15;
* @return The timestamp.
*/
long getTimestamp();
/**
* int64 IntervalYearMonth = 16;
* @return Whether the intervalYearMonth field is set.
*/
boolean hasIntervalYearMonth();
/**
* int64 IntervalYearMonth = 16;
* @return The intervalYearMonth.
*/
long getIntervalYearMonth();
/**
* .cz.proto.IntervalDayTime IntervalDayTime = 17;
* @return Whether the intervalDayTime field is set.
*/
boolean hasIntervalDayTime();
/**
* .cz.proto.IntervalDayTime IntervalDayTime = 17;
* @return The intervalDayTime.
*/
cz.proto.Expression.IntervalDayTime getIntervalDayTime();
/**
* .cz.proto.IntervalDayTime IntervalDayTime = 17;
*/
cz.proto.Expression.IntervalDayTimeOrBuilder getIntervalDayTimeOrBuilder();
/**
* .cz.proto.ArrayValue array = 100;
* @return Whether the array field is set.
*/
boolean hasArray();
/**
* .cz.proto.ArrayValue array = 100;
* @return The array.
*/
cz.proto.Expression.ArrayValue getArray();
/**
* .cz.proto.ArrayValue array = 100;
*/
cz.proto.Expression.ArrayValueOrBuilder getArrayOrBuilder();
/**
* .cz.proto.MapValue map = 101;
* @return Whether the map field is set.
*/
boolean hasMap();
/**
* .cz.proto.MapValue map = 101;
* @return The map.
*/
cz.proto.Expression.MapValue getMap();
/**
* .cz.proto.MapValue map = 101;
*/
cz.proto.Expression.MapValueOrBuilder getMapOrBuilder();
/**
* .cz.proto.StructValue struct = 102;
* @return Whether the struct field is set.
*/
boolean hasStruct();
/**
* .cz.proto.StructValue struct = 102;
* @return The struct.
*/
cz.proto.Expression.StructValue getStruct();
/**
* .cz.proto.StructValue struct = 102;
*/
cz.proto.Expression.StructValueOrBuilder getStructOrBuilder();
/**
* .cz.proto.BitmapValue bitmap = 103;
* @return Whether the bitmap field is set.
*/
boolean hasBitmap();
/**
* .cz.proto.BitmapValue bitmap = 103;
* @return The bitmap.
*/
cz.proto.Expression.BitmapValue getBitmap();
/**
* .cz.proto.BitmapValue bitmap = 103;
*/
cz.proto.Expression.BitmapValueOrBuilder getBitmapOrBuilder();
public cz.proto.Expression.Constant.ValueCase getValueCase();
}
/**
* Protobuf type {@code cz.proto.Constant}
*/
public static final class Constant extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.Constant)
ConstantOrBuilder {
private static final long serialVersionUID = 0L;
// Use Constant.newBuilder() to construct.
private Constant(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Constant() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Constant();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Constant(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
value_ = input.readBool();
valueCase_ = 1;
break;
}
case 16: {
value_ = input.readInt32();
valueCase_ = 2;
break;
}
case 24: {
value_ = input.readInt32();
valueCase_ = 3;
break;
}
case 32: {
value_ = input.readInt32();
valueCase_ = 4;
break;
}
case 40: {
value_ = input.readInt64();
valueCase_ = 5;
break;
}
case 53: {
value_ = input.readFloat();
valueCase_ = 6;
break;
}
case 57: {
value_ = input.readDouble();
valueCase_ = 7;
break;
}
case 66: {
java.lang.String s = input.readStringRequireUtf8();
valueCase_ = 8;
value_ = s;
break;
}
case 72: {
value_ = input.readBool();
valueCase_ = 9;
break;
}
case 82: {
java.lang.String s = input.readStringRequireUtf8();
valueCase_ = 10;
value_ = s;
break;
}
case 90: {
java.lang.String s = input.readStringRequireUtf8();
valueCase_ = 11;
value_ = s;
break;
}
case 98: {
java.lang.String s = input.readStringRequireUtf8();
valueCase_ = 12;
value_ = s;
break;
}
case 106: {
value_ = input.readBytes();
valueCase_ = 13;
break;
}
case 112: {
value_ = input.readInt32();
valueCase_ = 14;
break;
}
case 120: {
value_ = input.readInt64();
valueCase_ = 15;
break;
}
case 128: {
value_ = input.readInt64();
valueCase_ = 16;
break;
}
case 138: {
cz.proto.Expression.IntervalDayTime.Builder subBuilder = null;
if (valueCase_ == 17) {
subBuilder = ((cz.proto.Expression.IntervalDayTime) value_).toBuilder();
}
value_ =
input.readMessage(cz.proto.Expression.IntervalDayTime.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((cz.proto.Expression.IntervalDayTime) value_);
value_ = subBuilder.buildPartial();
}
valueCase_ = 17;
break;
}
case 802: {
cz.proto.Expression.ArrayValue.Builder subBuilder = null;
if (valueCase_ == 100) {
subBuilder = ((cz.proto.Expression.ArrayValue) value_).toBuilder();
}
value_ =
input.readMessage(cz.proto.Expression.ArrayValue.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((cz.proto.Expression.ArrayValue) value_);
value_ = subBuilder.buildPartial();
}
valueCase_ = 100;
break;
}
case 810: {
cz.proto.Expression.MapValue.Builder subBuilder = null;
if (valueCase_ == 101) {
subBuilder = ((cz.proto.Expression.MapValue) value_).toBuilder();
}
value_ =
input.readMessage(cz.proto.Expression.MapValue.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((cz.proto.Expression.MapValue) value_);
value_ = subBuilder.buildPartial();
}
valueCase_ = 101;
break;
}
case 818: {
cz.proto.Expression.StructValue.Builder subBuilder = null;
if (valueCase_ == 102) {
subBuilder = ((cz.proto.Expression.StructValue) value_).toBuilder();
}
value_ =
input.readMessage(cz.proto.Expression.StructValue.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((cz.proto.Expression.StructValue) value_);
value_ = subBuilder.buildPartial();
}
valueCase_ = 102;
break;
}
case 826: {
cz.proto.Expression.BitmapValue.Builder subBuilder = null;
if (valueCase_ == 103) {
subBuilder = ((cz.proto.Expression.BitmapValue) value_).toBuilder();
}
value_ =
input.readMessage(cz.proto.Expression.BitmapValue.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((cz.proto.Expression.BitmapValue) value_);
value_ = subBuilder.buildPartial();
}
valueCase_ = 103;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.Expression.internal_static_cz_proto_Constant_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.Expression.internal_static_cz_proto_Constant_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.Expression.Constant.class, cz.proto.Expression.Constant.Builder.class);
}
private int valueCase_ = 0;
private java.lang.Object value_;
public enum ValueCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
NULL(1),
TINYINT(2),
SMALLINT(3),
INT(4),
BIGINT(5),
FLOAT(6),
DOUBLE(7),
DECIMAL(8),
BOOLEAN(9),
CHAR(10),
VARCHAR(11),
STRING(12),
BINARY(13),
DATE(14),
TIMESTAMP(15),
INTERVALYEARMONTH(16),
INTERVALDAYTIME(17),
ARRAY(100),
MAP(101),
STRUCT(102),
BITMAP(103),
VALUE_NOT_SET(0);
private final int value;
private ValueCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ValueCase valueOf(int value) {
return forNumber(value);
}
public static ValueCase forNumber(int value) {
switch (value) {
case 1: return NULL;
case 2: return TINYINT;
case 3: return SMALLINT;
case 4: return INT;
case 5: return BIGINT;
case 6: return FLOAT;
case 7: return DOUBLE;
case 8: return DECIMAL;
case 9: return BOOLEAN;
case 10: return CHAR;
case 11: return VARCHAR;
case 12: return STRING;
case 13: return BINARY;
case 14: return DATE;
case 15: return TIMESTAMP;
case 16: return INTERVALYEARMONTH;
case 17: return INTERVALDAYTIME;
case 100: return ARRAY;
case 101: return MAP;
case 102: return STRUCT;
case 103: return BITMAP;
case 0: return VALUE_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public ValueCase
getValueCase() {
return ValueCase.forNumber(
valueCase_);
}
public static final int NULL_FIELD_NUMBER = 1;
/**
* bool null = 1;
* @return Whether the null field is set.
*/
@java.lang.Override
public boolean hasNull() {
return valueCase_ == 1;
}
/**
* bool null = 1;
* @return The null.
*/
@java.lang.Override
public boolean getNull() {
if (valueCase_ == 1) {
return (java.lang.Boolean) value_;
}
return false;
}
public static final int TINYINT_FIELD_NUMBER = 2;
/**
* int32 tinyint = 2;
* @return Whether the tinyint field is set.
*/
@java.lang.Override
public boolean hasTinyint() {
return valueCase_ == 2;
}
/**
* int32 tinyint = 2;
* @return The tinyint.
*/
@java.lang.Override
public int getTinyint() {
if (valueCase_ == 2) {
return (java.lang.Integer) value_;
}
return 0;
}
public static final int SMALLINT_FIELD_NUMBER = 3;
/**
* int32 smallInt = 3;
* @return Whether the smallInt field is set.
*/
@java.lang.Override
public boolean hasSmallInt() {
return valueCase_ == 3;
}
/**
* int32 smallInt = 3;
* @return The smallInt.
*/
@java.lang.Override
public int getSmallInt() {
if (valueCase_ == 3) {
return (java.lang.Integer) value_;
}
return 0;
}
public static final int INT_FIELD_NUMBER = 4;
/**
* int32 int = 4;
* @return Whether the int field is set.
*/
@java.lang.Override
public boolean hasInt() {
return valueCase_ == 4;
}
/**
* int32 int = 4;
* @return The int.
*/
@java.lang.Override
public int getInt() {
if (valueCase_ == 4) {
return (java.lang.Integer) value_;
}
return 0;
}
public static final int BIGINT_FIELD_NUMBER = 5;
/**
* int64 bigint = 5;
* @return Whether the bigint field is set.
*/
@java.lang.Override
public boolean hasBigint() {
return valueCase_ == 5;
}
/**
* int64 bigint = 5;
* @return The bigint.
*/
@java.lang.Override
public long getBigint() {
if (valueCase_ == 5) {
return (java.lang.Long) value_;
}
return 0L;
}
public static final int FLOAT_FIELD_NUMBER = 6;
/**
* float float = 6;
* @return Whether the float field is set.
*/
@java.lang.Override
public boolean hasFloat() {
return valueCase_ == 6;
}
/**
* float float = 6;
* @return The float.
*/
@java.lang.Override
public float getFloat() {
if (valueCase_ == 6) {
return (java.lang.Float) value_;
}
return 0F;
}
public static final int DOUBLE_FIELD_NUMBER = 7;
/**
* double double = 7;
* @return Whether the double field is set.
*/
@java.lang.Override
public boolean hasDouble() {
return valueCase_ == 7;
}
/**
* double double = 7;
* @return The double.
*/
@java.lang.Override
public double getDouble() {
if (valueCase_ == 7) {
return (java.lang.Double) value_;
}
return 0D;
}
public static final int DECIMAL_FIELD_NUMBER = 8;
/**
* string decimal = 8;
* @return Whether the decimal field is set.
*/
public boolean hasDecimal() {
return valueCase_ == 8;
}
/**
* string decimal = 8;
* @return The decimal.
*/
public java.lang.String getDecimal() {
java.lang.Object ref = "";
if (valueCase_ == 8) {
ref = value_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (valueCase_ == 8) {
value_ = s;
}
return s;
}
}
/**
* string decimal = 8;
* @return The bytes for decimal.
*/
public com.google.protobuf.ByteString
getDecimalBytes() {
java.lang.Object ref = "";
if (valueCase_ == 8) {
ref = value_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (valueCase_ == 8) {
value_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int BOOLEAN_FIELD_NUMBER = 9;
/**
* bool boolean = 9;
* @return Whether the boolean field is set.
*/
@java.lang.Override
public boolean hasBoolean() {
return valueCase_ == 9;
}
/**
* bool boolean = 9;
* @return The boolean.
*/
@java.lang.Override
public boolean getBoolean() {
if (valueCase_ == 9) {
return (java.lang.Boolean) value_;
}
return false;
}
public static final int CHAR_FIELD_NUMBER = 10;
/**
* string char = 10;
* @return Whether the char field is set.
*/
public boolean hasChar() {
return valueCase_ == 10;
}
/**
* string char = 10;
* @return The char.
*/
public java.lang.String getChar() {
java.lang.Object ref = "";
if (valueCase_ == 10) {
ref = value_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (valueCase_ == 10) {
value_ = s;
}
return s;
}
}
/**
* string char = 10;
* @return The bytes for char.
*/
public com.google.protobuf.ByteString
getCharBytes() {
java.lang.Object ref = "";
if (valueCase_ == 10) {
ref = value_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (valueCase_ == 10) {
value_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VARCHAR_FIELD_NUMBER = 11;
/**
* string varchar = 11;
* @return Whether the varchar field is set.
*/
public boolean hasVarchar() {
return valueCase_ == 11;
}
/**
* string varchar = 11;
* @return The varchar.
*/
public java.lang.String getVarchar() {
java.lang.Object ref = "";
if (valueCase_ == 11) {
ref = value_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (valueCase_ == 11) {
value_ = s;
}
return s;
}
}
/**
* string varchar = 11;
* @return The bytes for varchar.
*/
public com.google.protobuf.ByteString
getVarcharBytes() {
java.lang.Object ref = "";
if (valueCase_ == 11) {
ref = value_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (valueCase_ == 11) {
value_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STRING_FIELD_NUMBER = 12;
/**
* string string = 12;
* @return Whether the string field is set.
*/
public boolean hasString() {
return valueCase_ == 12;
}
/**
* string string = 12;
* @return The string.
*/
public java.lang.String getString() {
java.lang.Object ref = "";
if (valueCase_ == 12) {
ref = value_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (valueCase_ == 12) {
value_ = s;
}
return s;
}
}
/**
* string string = 12;
* @return The bytes for string.
*/
public com.google.protobuf.ByteString
getStringBytes() {
java.lang.Object ref = "";
if (valueCase_ == 12) {
ref = value_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (valueCase_ == 12) {
value_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int BINARY_FIELD_NUMBER = 13;
/**
* bytes binary = 13;
* @return Whether the binary field is set.
*/
@java.lang.Override
public boolean hasBinary() {
return valueCase_ == 13;
}
/**
* bytes binary = 13;
* @return The binary.
*/
@java.lang.Override
public com.google.protobuf.ByteString getBinary() {
if (valueCase_ == 13) {
return (com.google.protobuf.ByteString) value_;
}
return com.google.protobuf.ByteString.EMPTY;
}
public static final int DATE_FIELD_NUMBER = 14;
/**
* int32 date = 14;
* @return Whether the date field is set.
*/
@java.lang.Override
public boolean hasDate() {
return valueCase_ == 14;
}
/**
* int32 date = 14;
* @return The date.
*/
@java.lang.Override
public int getDate() {
if (valueCase_ == 14) {
return (java.lang.Integer) value_;
}
return 0;
}
public static final int TIMESTAMP_FIELD_NUMBER = 15;
/**
* int64 timestamp = 15;
* @return Whether the timestamp field is set.
*/
@java.lang.Override
public boolean hasTimestamp() {
return valueCase_ == 15;
}
/**
* int64 timestamp = 15;
* @return The timestamp.
*/
@java.lang.Override
public long getTimestamp() {
if (valueCase_ == 15) {
return (java.lang.Long) value_;
}
return 0L;
}
public static final int INTERVALYEARMONTH_FIELD_NUMBER = 16;
/**
* int64 IntervalYearMonth = 16;
* @return Whether the intervalYearMonth field is set.
*/
@java.lang.Override
public boolean hasIntervalYearMonth() {
return valueCase_ == 16;
}
/**
* int64 IntervalYearMonth = 16;
* @return The intervalYearMonth.
*/
@java.lang.Override
public long getIntervalYearMonth() {
if (valueCase_ == 16) {
return (java.lang.Long) value_;
}
return 0L;
}
public static final int INTERVALDAYTIME_FIELD_NUMBER = 17;
/**
* .cz.proto.IntervalDayTime IntervalDayTime = 17;
* @return Whether the intervalDayTime field is set.
*/
@java.lang.Override
public boolean hasIntervalDayTime() {
return valueCase_ == 17;
}
/**
* .cz.proto.IntervalDayTime IntervalDayTime = 17;
* @return The intervalDayTime.
*/
@java.lang.Override
public cz.proto.Expression.IntervalDayTime getIntervalDayTime() {
if (valueCase_ == 17) {
return (cz.proto.Expression.IntervalDayTime) value_;
}
return cz.proto.Expression.IntervalDayTime.getDefaultInstance();
}
/**
* .cz.proto.IntervalDayTime IntervalDayTime = 17;
*/
@java.lang.Override
public cz.proto.Expression.IntervalDayTimeOrBuilder getIntervalDayTimeOrBuilder() {
if (valueCase_ == 17) {
return (cz.proto.Expression.IntervalDayTime) value_;
}
return cz.proto.Expression.IntervalDayTime.getDefaultInstance();
}
public static final int ARRAY_FIELD_NUMBER = 100;
/**
* .cz.proto.ArrayValue array = 100;
* @return Whether the array field is set.
*/
@java.lang.Override
public boolean hasArray() {
return valueCase_ == 100;
}
/**
* .cz.proto.ArrayValue array = 100;
* @return The array.
*/
@java.lang.Override
public cz.proto.Expression.ArrayValue getArray() {
if (valueCase_ == 100) {
return (cz.proto.Expression.ArrayValue) value_;
}
return cz.proto.Expression.ArrayValue.getDefaultInstance();
}
/**
* .cz.proto.ArrayValue array = 100;
*/
@java.lang.Override
public cz.proto.Expression.ArrayValueOrBuilder getArrayOrBuilder() {
if (valueCase_ == 100) {
return (cz.proto.Expression.ArrayValue) value_;
}
return cz.proto.Expression.ArrayValue.getDefaultInstance();
}
public static final int MAP_FIELD_NUMBER = 101;
/**
* .cz.proto.MapValue map = 101;
* @return Whether the map field is set.
*/
@java.lang.Override
public boolean hasMap() {
return valueCase_ == 101;
}
/**
* .cz.proto.MapValue map = 101;
* @return The map.
*/
@java.lang.Override
public cz.proto.Expression.MapValue getMap() {
if (valueCase_ == 101) {
return (cz.proto.Expression.MapValue) value_;
}
return cz.proto.Expression.MapValue.getDefaultInstance();
}
/**
* .cz.proto.MapValue map = 101;
*/
@java.lang.Override
public cz.proto.Expression.MapValueOrBuilder getMapOrBuilder() {
if (valueCase_ == 101) {
return (cz.proto.Expression.MapValue) value_;
}
return cz.proto.Expression.MapValue.getDefaultInstance();
}
public static final int STRUCT_FIELD_NUMBER = 102;
/**
* .cz.proto.StructValue struct = 102;
* @return Whether the struct field is set.
*/
@java.lang.Override
public boolean hasStruct() {
return valueCase_ == 102;
}
/**
* .cz.proto.StructValue struct = 102;
* @return The struct.
*/
@java.lang.Override
public cz.proto.Expression.StructValue getStruct() {
if (valueCase_ == 102) {
return (cz.proto.Expression.StructValue) value_;
}
return cz.proto.Expression.StructValue.getDefaultInstance();
}
/**
* .cz.proto.StructValue struct = 102;
*/
@java.lang.Override
public cz.proto.Expression.StructValueOrBuilder getStructOrBuilder() {
if (valueCase_ == 102) {
return (cz.proto.Expression.StructValue) value_;
}
return cz.proto.Expression.StructValue.getDefaultInstance();
}
public static final int BITMAP_FIELD_NUMBER = 103;
/**
* .cz.proto.BitmapValue bitmap = 103;
* @return Whether the bitmap field is set.
*/
@java.lang.Override
public boolean hasBitmap() {
return valueCase_ == 103;
}
/**
* .cz.proto.BitmapValue bitmap = 103;
* @return The bitmap.
*/
@java.lang.Override
public cz.proto.Expression.BitmapValue getBitmap() {
if (valueCase_ == 103) {
return (cz.proto.Expression.BitmapValue) value_;
}
return cz.proto.Expression.BitmapValue.getDefaultInstance();
}
/**
* .cz.proto.BitmapValue bitmap = 103;
*/
@java.lang.Override
public cz.proto.Expression.BitmapValueOrBuilder getBitmapOrBuilder() {
if (valueCase_ == 103) {
return (cz.proto.Expression.BitmapValue) value_;
}
return cz.proto.Expression.BitmapValue.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (valueCase_ == 1) {
output.writeBool(
1, (boolean)((java.lang.Boolean) value_));
}
if (valueCase_ == 2) {
output.writeInt32(
2, (int)((java.lang.Integer) value_));
}
if (valueCase_ == 3) {
output.writeInt32(
3, (int)((java.lang.Integer) value_));
}
if (valueCase_ == 4) {
output.writeInt32(
4, (int)((java.lang.Integer) value_));
}
if (valueCase_ == 5) {
output.writeInt64(
5, (long)((java.lang.Long) value_));
}
if (valueCase_ == 6) {
output.writeFloat(
6, (float)((java.lang.Float) value_));
}
if (valueCase_ == 7) {
output.writeDouble(
7, (double)((java.lang.Double) value_));
}
if (valueCase_ == 8) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 8, value_);
}
if (valueCase_ == 9) {
output.writeBool(
9, (boolean)((java.lang.Boolean) value_));
}
if (valueCase_ == 10) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 10, value_);
}
if (valueCase_ == 11) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 11, value_);
}
if (valueCase_ == 12) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 12, value_);
}
if (valueCase_ == 13) {
output.writeBytes(
13, (com.google.protobuf.ByteString) value_);
}
if (valueCase_ == 14) {
output.writeInt32(
14, (int)((java.lang.Integer) value_));
}
if (valueCase_ == 15) {
output.writeInt64(
15, (long)((java.lang.Long) value_));
}
if (valueCase_ == 16) {
output.writeInt64(
16, (long)((java.lang.Long) value_));
}
if (valueCase_ == 17) {
output.writeMessage(17, (cz.proto.Expression.IntervalDayTime) value_);
}
if (valueCase_ == 100) {
output.writeMessage(100, (cz.proto.Expression.ArrayValue) value_);
}
if (valueCase_ == 101) {
output.writeMessage(101, (cz.proto.Expression.MapValue) value_);
}
if (valueCase_ == 102) {
output.writeMessage(102, (cz.proto.Expression.StructValue) value_);
}
if (valueCase_ == 103) {
output.writeMessage(103, (cz.proto.Expression.BitmapValue) value_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (valueCase_ == 1) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(
1, (boolean)((java.lang.Boolean) value_));
}
if (valueCase_ == 2) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(
2, (int)((java.lang.Integer) value_));
}
if (valueCase_ == 3) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(
3, (int)((java.lang.Integer) value_));
}
if (valueCase_ == 4) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(
4, (int)((java.lang.Integer) value_));
}
if (valueCase_ == 5) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(
5, (long)((java.lang.Long) value_));
}
if (valueCase_ == 6) {
size += com.google.protobuf.CodedOutputStream
.computeFloatSize(
6, (float)((java.lang.Float) value_));
}
if (valueCase_ == 7) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(
7, (double)((java.lang.Double) value_));
}
if (valueCase_ == 8) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(8, value_);
}
if (valueCase_ == 9) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(
9, (boolean)((java.lang.Boolean) value_));
}
if (valueCase_ == 10) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(10, value_);
}
if (valueCase_ == 11) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(11, value_);
}
if (valueCase_ == 12) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(12, value_);
}
if (valueCase_ == 13) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(
13, (com.google.protobuf.ByteString) value_);
}
if (valueCase_ == 14) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(
14, (int)((java.lang.Integer) value_));
}
if (valueCase_ == 15) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(
15, (long)((java.lang.Long) value_));
}
if (valueCase_ == 16) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(
16, (long)((java.lang.Long) value_));
}
if (valueCase_ == 17) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(17, (cz.proto.Expression.IntervalDayTime) value_);
}
if (valueCase_ == 100) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(100, (cz.proto.Expression.ArrayValue) value_);
}
if (valueCase_ == 101) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(101, (cz.proto.Expression.MapValue) value_);
}
if (valueCase_ == 102) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(102, (cz.proto.Expression.StructValue) value_);
}
if (valueCase_ == 103) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(103, (cz.proto.Expression.BitmapValue) value_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.Expression.Constant)) {
return super.equals(obj);
}
cz.proto.Expression.Constant other = (cz.proto.Expression.Constant) obj;
if (!getValueCase().equals(other.getValueCase())) return false;
switch (valueCase_) {
case 1:
if (getNull()
!= other.getNull()) return false;
break;
case 2:
if (getTinyint()
!= other.getTinyint()) return false;
break;
case 3:
if (getSmallInt()
!= other.getSmallInt()) return false;
break;
case 4:
if (getInt()
!= other.getInt()) return false;
break;
case 5:
if (getBigint()
!= other.getBigint()) return false;
break;
case 6:
if (java.lang.Float.floatToIntBits(getFloat())
!= java.lang.Float.floatToIntBits(
other.getFloat())) return false;
break;
case 7:
if (java.lang.Double.doubleToLongBits(getDouble())
!= java.lang.Double.doubleToLongBits(
other.getDouble())) return false;
break;
case 8:
if (!getDecimal()
.equals(other.getDecimal())) return false;
break;
case 9:
if (getBoolean()
!= other.getBoolean()) return false;
break;
case 10:
if (!getChar()
.equals(other.getChar())) return false;
break;
case 11:
if (!getVarchar()
.equals(other.getVarchar())) return false;
break;
case 12:
if (!getString()
.equals(other.getString())) return false;
break;
case 13:
if (!getBinary()
.equals(other.getBinary())) return false;
break;
case 14:
if (getDate()
!= other.getDate()) return false;
break;
case 15:
if (getTimestamp()
!= other.getTimestamp()) return false;
break;
case 16:
if (getIntervalYearMonth()
!= other.getIntervalYearMonth()) return false;
break;
case 17:
if (!getIntervalDayTime()
.equals(other.getIntervalDayTime())) return false;
break;
case 100:
if (!getArray()
.equals(other.getArray())) return false;
break;
case 101:
if (!getMap()
.equals(other.getMap())) return false;
break;
case 102:
if (!getStruct()
.equals(other.getStruct())) return false;
break;
case 103:
if (!getBitmap()
.equals(other.getBitmap())) return false;
break;
case 0:
default:
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
switch (valueCase_) {
case 1:
hash = (37 * hash) + NULL_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getNull());
break;
case 2:
hash = (37 * hash) + TINYINT_FIELD_NUMBER;
hash = (53 * hash) + getTinyint();
break;
case 3:
hash = (37 * hash) + SMALLINT_FIELD_NUMBER;
hash = (53 * hash) + getSmallInt();
break;
case 4:
hash = (37 * hash) + INT_FIELD_NUMBER;
hash = (53 * hash) + getInt();
break;
case 5:
hash = (37 * hash) + BIGINT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getBigint());
break;
case 6:
hash = (37 * hash) + FLOAT_FIELD_NUMBER;
hash = (53 * hash) + java.lang.Float.floatToIntBits(
getFloat());
break;
case 7:
hash = (37 * hash) + DOUBLE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getDouble()));
break;
case 8:
hash = (37 * hash) + DECIMAL_FIELD_NUMBER;
hash = (53 * hash) + getDecimal().hashCode();
break;
case 9:
hash = (37 * hash) + BOOLEAN_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getBoolean());
break;
case 10:
hash = (37 * hash) + CHAR_FIELD_NUMBER;
hash = (53 * hash) + getChar().hashCode();
break;
case 11:
hash = (37 * hash) + VARCHAR_FIELD_NUMBER;
hash = (53 * hash) + getVarchar().hashCode();
break;
case 12:
hash = (37 * hash) + STRING_FIELD_NUMBER;
hash = (53 * hash) + getString().hashCode();
break;
case 13:
hash = (37 * hash) + BINARY_FIELD_NUMBER;
hash = (53 * hash) + getBinary().hashCode();
break;
case 14:
hash = (37 * hash) + DATE_FIELD_NUMBER;
hash = (53 * hash) + getDate();
break;
case 15:
hash = (37 * hash) + TIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTimestamp());
break;
case 16:
hash = (37 * hash) + INTERVALYEARMONTH_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getIntervalYearMonth());
break;
case 17:
hash = (37 * hash) + INTERVALDAYTIME_FIELD_NUMBER;
hash = (53 * hash) + getIntervalDayTime().hashCode();
break;
case 100:
hash = (37 * hash) + ARRAY_FIELD_NUMBER;
hash = (53 * hash) + getArray().hashCode();
break;
case 101:
hash = (37 * hash) + MAP_FIELD_NUMBER;
hash = (53 * hash) + getMap().hashCode();
break;
case 102:
hash = (37 * hash) + STRUCT_FIELD_NUMBER;
hash = (53 * hash) + getStruct().hashCode();
break;
case 103:
hash = (37 * hash) + BITMAP_FIELD_NUMBER;
hash = (53 * hash) + getBitmap().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.Expression.Constant parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.Constant parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.Constant parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.Constant parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.Constant parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.Constant parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.Constant parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.Expression.Constant parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.Expression.Constant parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.Expression.Constant parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.Expression.Constant parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.Expression.Constant parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.Expression.Constant prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.Constant}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.Constant)
cz.proto.Expression.ConstantOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.Expression.internal_static_cz_proto_Constant_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.Expression.internal_static_cz_proto_Constant_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.Expression.Constant.class, cz.proto.Expression.Constant.Builder.class);
}
// Construct using cz.proto.Expression.Constant.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
valueCase_ = 0;
value_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.Expression.internal_static_cz_proto_Constant_descriptor;
}
@java.lang.Override
public cz.proto.Expression.Constant getDefaultInstanceForType() {
return cz.proto.Expression.Constant.getDefaultInstance();
}
@java.lang.Override
public cz.proto.Expression.Constant build() {
cz.proto.Expression.Constant result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.Expression.Constant buildPartial() {
cz.proto.Expression.Constant result = new cz.proto.Expression.Constant(this);
if (valueCase_ == 1) {
result.value_ = value_;
}
if (valueCase_ == 2) {
result.value_ = value_;
}
if (valueCase_ == 3) {
result.value_ = value_;
}
if (valueCase_ == 4) {
result.value_ = value_;
}
if (valueCase_ == 5) {
result.value_ = value_;
}
if (valueCase_ == 6) {
result.value_ = value_;
}
if (valueCase_ == 7) {
result.value_ = value_;
}
if (valueCase_ == 8) {
result.value_ = value_;
}
if (valueCase_ == 9) {
result.value_ = value_;
}
if (valueCase_ == 10) {
result.value_ = value_;
}
if (valueCase_ == 11) {
result.value_ = value_;
}
if (valueCase_ == 12) {
result.value_ = value_;
}
if (valueCase_ == 13) {
result.value_ = value_;
}
if (valueCase_ == 14) {
result.value_ = value_;
}
if (valueCase_ == 15) {
result.value_ = value_;
}
if (valueCase_ == 16) {
result.value_ = value_;
}
if (valueCase_ == 17) {
if (intervalDayTimeBuilder_ == null) {
result.value_ = value_;
} else {
result.value_ = intervalDayTimeBuilder_.build();
}
}
if (valueCase_ == 100) {
if (arrayBuilder_ == null) {
result.value_ = value_;
} else {
result.value_ = arrayBuilder_.build();
}
}
if (valueCase_ == 101) {
if (mapBuilder_ == null) {
result.value_ = value_;
} else {
result.value_ = mapBuilder_.build();
}
}
if (valueCase_ == 102) {
if (structBuilder_ == null) {
result.value_ = value_;
} else {
result.value_ = structBuilder_.build();
}
}
if (valueCase_ == 103) {
if (bitmapBuilder_ == null) {
result.value_ = value_;
} else {
result.value_ = bitmapBuilder_.build();
}
}
result.valueCase_ = valueCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.Expression.Constant) {
return mergeFrom((cz.proto.Expression.Constant)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.Expression.Constant other) {
if (other == cz.proto.Expression.Constant.getDefaultInstance()) return this;
switch (other.getValueCase()) {
case NULL: {
setNull(other.getNull());
break;
}
case TINYINT: {
setTinyint(other.getTinyint());
break;
}
case SMALLINT: {
setSmallInt(other.getSmallInt());
break;
}
case INT: {
setInt(other.getInt());
break;
}
case BIGINT: {
setBigint(other.getBigint());
break;
}
case FLOAT: {
setFloat(other.getFloat());
break;
}
case DOUBLE: {
setDouble(other.getDouble());
break;
}
case DECIMAL: {
valueCase_ = 8;
value_ = other.value_;
onChanged();
break;
}
case BOOLEAN: {
setBoolean(other.getBoolean());
break;
}
case CHAR: {
valueCase_ = 10;
value_ = other.value_;
onChanged();
break;
}
case VARCHAR: {
valueCase_ = 11;
value_ = other.value_;
onChanged();
break;
}
case STRING: {
valueCase_ = 12;
value_ = other.value_;
onChanged();
break;
}
case BINARY: {
setBinary(other.getBinary());
break;
}
case DATE: {
setDate(other.getDate());
break;
}
case TIMESTAMP: {
setTimestamp(other.getTimestamp());
break;
}
case INTERVALYEARMONTH: {
setIntervalYearMonth(other.getIntervalYearMonth());
break;
}
case INTERVALDAYTIME: {
mergeIntervalDayTime(other.getIntervalDayTime());
break;
}
case ARRAY: {
mergeArray(other.getArray());
break;
}
case MAP: {
mergeMap(other.getMap());
break;
}
case STRUCT: {
mergeStruct(other.getStruct());
break;
}
case BITMAP: {
mergeBitmap(other.getBitmap());
break;
}
case VALUE_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.Expression.Constant parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.Expression.Constant) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int valueCase_ = 0;
private java.lang.Object value_;
public ValueCase
getValueCase() {
return ValueCase.forNumber(
valueCase_);
}
public Builder clearValue() {
valueCase_ = 0;
value_ = null;
onChanged();
return this;
}
/**
* bool null = 1;
* @return Whether the null field is set.
*/
public boolean hasNull() {
return valueCase_ == 1;
}
/**
* bool null = 1;
* @return The null.
*/
public boolean getNull() {
if (valueCase_ == 1) {
return (java.lang.Boolean) value_;
}
return false;
}
/**
* bool null = 1;
* @param value The null to set.
* @return This builder for chaining.
*/
public Builder setNull(boolean value) {
valueCase_ = 1;
value_ = value;
onChanged();
return this;
}
/**
* bool null = 1;
* @return This builder for chaining.
*/
public Builder clearNull() {
if (valueCase_ == 1) {
valueCase_ = 0;
value_ = null;
onChanged();
}
return this;
}
/**
* int32 tinyint = 2;
* @return Whether the tinyint field is set.
*/
public boolean hasTinyint() {
return valueCase_ == 2;
}
/**
* int32 tinyint = 2;
* @return The tinyint.
*/
public int getTinyint() {
if (valueCase_ == 2) {
return (java.lang.Integer) value_;
}
return 0;
}
/**
* int32 tinyint = 2;
* @param value The tinyint to set.
* @return This builder for chaining.
*/
public Builder setTinyint(int value) {
valueCase_ = 2;
value_ = value;
onChanged();
return this;
}
/**
* int32 tinyint = 2;
* @return This builder for chaining.
*/
public Builder clearTinyint() {
if (valueCase_ == 2) {
valueCase_ = 0;
value_ = null;
onChanged();
}
return this;
}
/**
* int32 smallInt = 3;
* @return Whether the smallInt field is set.
*/
public boolean hasSmallInt() {
return valueCase_ == 3;
}
/**
* int32 smallInt = 3;
* @return The smallInt.
*/
public int getSmallInt() {
if (valueCase_ == 3) {
return (java.lang.Integer) value_;
}
return 0;
}
/**
* int32 smallInt = 3;
* @param value The smallInt to set.
* @return This builder for chaining.
*/
public Builder setSmallInt(int value) {
valueCase_ = 3;
value_ = value;
onChanged();
return this;
}
/**
* int32 smallInt = 3;
* @return This builder for chaining.
*/
public Builder clearSmallInt() {
if (valueCase_ == 3) {
valueCase_ = 0;
value_ = null;
onChanged();
}
return this;
}
/**
* int32 int = 4;
* @return Whether the int field is set.
*/
public boolean hasInt() {
return valueCase_ == 4;
}
/**
* int32 int = 4;
* @return The int.
*/
public int getInt() {
if (valueCase_ == 4) {
return (java.lang.Integer) value_;
}
return 0;
}
/**
* int32 int = 4;
* @param value The int to set.
* @return This builder for chaining.
*/
public Builder setInt(int value) {
valueCase_ = 4;
value_ = value;
onChanged();
return this;
}
/**
* int32 int = 4;
* @return This builder for chaining.
*/
public Builder clearInt() {
if (valueCase_ == 4) {
valueCase_ = 0;
value_ = null;
onChanged();
}
return this;
}
/**
* int64 bigint = 5;
* @return Whether the bigint field is set.
*/
public boolean hasBigint() {
return valueCase_ == 5;
}
/**
* int64 bigint = 5;
* @return The bigint.
*/
public long getBigint() {
if (valueCase_ == 5) {
return (java.lang.Long) value_;
}
return 0L;
}
/**
* int64 bigint = 5;
* @param value The bigint to set.
* @return This builder for chaining.
*/
public Builder setBigint(long value) {
valueCase_ = 5;
value_ = value;
onChanged();
return this;
}
/**
* int64 bigint = 5;
* @return This builder for chaining.
*/
public Builder clearBigint() {
if (valueCase_ == 5) {
valueCase_ = 0;
value_ = null;
onChanged();
}
return this;
}
/**
* float float = 6;
* @return Whether the float field is set.
*/
public boolean hasFloat() {
return valueCase_ == 6;
}
/**
* float float = 6;
* @return The float.
*/
public float getFloat() {
if (valueCase_ == 6) {
return (java.lang.Float) value_;
}
return 0F;
}
/**
* float float = 6;
* @param value The float to set.
* @return This builder for chaining.
*/
public Builder setFloat(float value) {
valueCase_ = 6;
value_ = value;
onChanged();
return this;
}
/**
* float float = 6;
* @return This builder for chaining.
*/
public Builder clearFloat() {
if (valueCase_ == 6) {
valueCase_ = 0;
value_ = null;
onChanged();
}
return this;
}
/**
* double double = 7;
* @return Whether the double field is set.
*/
public boolean hasDouble() {
return valueCase_ == 7;
}
/**
* double double = 7;
* @return The double.
*/
public double getDouble() {
if (valueCase_ == 7) {
return (java.lang.Double) value_;
}
return 0D;
}
/**
* double double = 7;
* @param value The double to set.
* @return This builder for chaining.
*/
public Builder setDouble(double value) {
valueCase_ = 7;
value_ = value;
onChanged();
return this;
}
/**
* double double = 7;
* @return This builder for chaining.
*/
public Builder clearDouble() {
if (valueCase_ == 7) {
valueCase_ = 0;
value_ = null;
onChanged();
}
return this;
}
/**
* string decimal = 8;
* @return Whether the decimal field is set.
*/
@java.lang.Override
public boolean hasDecimal() {
return valueCase_ == 8;
}
/**
* string decimal = 8;
* @return The decimal.
*/
@java.lang.Override
public java.lang.String getDecimal() {
java.lang.Object ref = "";
if (valueCase_ == 8) {
ref = value_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (valueCase_ == 8) {
value_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string decimal = 8;
* @return The bytes for decimal.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getDecimalBytes() {
java.lang.Object ref = "";
if (valueCase_ == 8) {
ref = value_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (valueCase_ == 8) {
value_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string decimal = 8;
* @param value The decimal to set.
* @return This builder for chaining.
*/
public Builder setDecimal(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
valueCase_ = 8;
value_ = value;
onChanged();
return this;
}
/**
* string decimal = 8;
* @return This builder for chaining.
*/
public Builder clearDecimal() {
if (valueCase_ == 8) {
valueCase_ = 0;
value_ = null;
onChanged();
}
return this;
}
/**
* string decimal = 8;
* @param value The bytes for decimal to set.
* @return This builder for chaining.
*/
public Builder setDecimalBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
valueCase_ = 8;
value_ = value;
onChanged();
return this;
}
/**
* bool boolean = 9;
* @return Whether the boolean field is set.
*/
public boolean hasBoolean() {
return valueCase_ == 9;
}
/**
* bool boolean = 9;
* @return The boolean.
*/
public boolean getBoolean() {
if (valueCase_ == 9) {
return (java.lang.Boolean) value_;
}
return false;
}
/**
* bool boolean = 9;
* @param value The boolean to set.
* @return This builder for chaining.
*/
public Builder setBoolean(boolean value) {
valueCase_ = 9;
value_ = value;
onChanged();
return this;
}
/**
* bool boolean = 9;
* @return This builder for chaining.
*/
public Builder clearBoolean() {
if (valueCase_ == 9) {
valueCase_ = 0;
value_ = null;
onChanged();
}
return this;
}
/**
* string char = 10;
* @return Whether the char field is set.
*/
@java.lang.Override
public boolean hasChar() {
return valueCase_ == 10;
}
/**
* string char = 10;
* @return The char.
*/
@java.lang.Override
public java.lang.String getChar() {
java.lang.Object ref = "";
if (valueCase_ == 10) {
ref = value_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (valueCase_ == 10) {
value_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string char = 10;
* @return The bytes for char.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCharBytes() {
java.lang.Object ref = "";
if (valueCase_ == 10) {
ref = value_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (valueCase_ == 10) {
value_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string char = 10;
* @param value The char to set.
* @return This builder for chaining.
*/
public Builder setChar(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
valueCase_ = 10;
value_ = value;
onChanged();
return this;
}
/**
* string char = 10;
* @return This builder for chaining.
*/
public Builder clearChar() {
if (valueCase_ == 10) {
valueCase_ = 0;
value_ = null;
onChanged();
}
return this;
}
/**
* string char = 10;
* @param value The bytes for char to set.
* @return This builder for chaining.
*/
public Builder setCharBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
valueCase_ = 10;
value_ = value;
onChanged();
return this;
}
/**
* string varchar = 11;
* @return Whether the varchar field is set.
*/
@java.lang.Override
public boolean hasVarchar() {
return valueCase_ == 11;
}
/**
* string varchar = 11;
* @return The varchar.
*/
@java.lang.Override
public java.lang.String getVarchar() {
java.lang.Object ref = "";
if (valueCase_ == 11) {
ref = value_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (valueCase_ == 11) {
value_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string varchar = 11;
* @return The bytes for varchar.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getVarcharBytes() {
java.lang.Object ref = "";
if (valueCase_ == 11) {
ref = value_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (valueCase_ == 11) {
value_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string varchar = 11;
* @param value The varchar to set.
* @return This builder for chaining.
*/
public Builder setVarchar(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
valueCase_ = 11;
value_ = value;
onChanged();
return this;
}
/**
* string varchar = 11;
* @return This builder for chaining.
*/
public Builder clearVarchar() {
if (valueCase_ == 11) {
valueCase_ = 0;
value_ = null;
onChanged();
}
return this;
}
/**
* string varchar = 11;
* @param value The bytes for varchar to set.
* @return This builder for chaining.
*/
public Builder setVarcharBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
valueCase_ = 11;
value_ = value;
onChanged();
return this;
}
/**
* string string = 12;
* @return Whether the string field is set.
*/
@java.lang.Override
public boolean hasString() {
return valueCase_ == 12;
}
/**
* string string = 12;
* @return The string.
*/
@java.lang.Override
public java.lang.String getString() {
java.lang.Object ref = "";
if (valueCase_ == 12) {
ref = value_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (valueCase_ == 12) {
value_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string string = 12;
* @return The bytes for string.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getStringBytes() {
java.lang.Object ref = "";
if (valueCase_ == 12) {
ref = value_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (valueCase_ == 12) {
value_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string string = 12;
* @param value The string to set.
* @return This builder for chaining.
*/
public Builder setString(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
valueCase_ = 12;
value_ = value;
onChanged();
return this;
}
/**
* string string = 12;
* @return This builder for chaining.
*/
public Builder clearString() {
if (valueCase_ == 12) {
valueCase_ = 0;
value_ = null;
onChanged();
}
return this;
}
/**
* string string = 12;
* @param value The bytes for string to set.
* @return This builder for chaining.
*/
public Builder setStringBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
valueCase_ = 12;
value_ = value;
onChanged();
return this;
}
/**
* bytes binary = 13;
* @return Whether the binary field is set.
*/
public boolean hasBinary() {
return valueCase_ == 13;
}
/**
* bytes binary = 13;
* @return The binary.
*/
public com.google.protobuf.ByteString getBinary() {
if (valueCase_ == 13) {
return (com.google.protobuf.ByteString) value_;
}
return com.google.protobuf.ByteString.EMPTY;
}
/**
* bytes binary = 13;
* @param value The binary to set.
* @return This builder for chaining.
*/
public Builder setBinary(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
valueCase_ = 13;
value_ = value;
onChanged();
return this;
}
/**
* bytes binary = 13;
* @return This builder for chaining.
*/
public Builder clearBinary() {
if (valueCase_ == 13) {
valueCase_ = 0;
value_ = null;
onChanged();
}
return this;
}
/**
* int32 date = 14;
* @return Whether the date field is set.
*/
public boolean hasDate() {
return valueCase_ == 14;
}
/**
* int32 date = 14;
* @return The date.
*/
public int getDate() {
if (valueCase_ == 14) {
return (java.lang.Integer) value_;
}
return 0;
}
/**
* int32 date = 14;
* @param value The date to set.
* @return This builder for chaining.
*/
public Builder setDate(int value) {
valueCase_ = 14;
value_ = value;
onChanged();
return this;
}
/**
* int32 date = 14;
* @return This builder for chaining.
*/
public Builder clearDate() {
if (valueCase_ == 14) {
valueCase_ = 0;
value_ = null;
onChanged();
}
return this;
}
/**
* int64 timestamp = 15;
* @return Whether the timestamp field is set.
*/
public boolean hasTimestamp() {
return valueCase_ == 15;
}
/**
* int64 timestamp = 15;
* @return The timestamp.
*/
public long getTimestamp() {
if (valueCase_ == 15) {
return (java.lang.Long) value_;
}
return 0L;
}
/**
* int64 timestamp = 15;
* @param value The timestamp to set.
* @return This builder for chaining.
*/
public Builder setTimestamp(long value) {
valueCase_ = 15;
value_ = value;
onChanged();
return this;
}
/**
* int64 timestamp = 15;
* @return This builder for chaining.
*/
public Builder clearTimestamp() {
if (valueCase_ == 15) {
valueCase_ = 0;
value_ = null;
onChanged();
}
return this;
}
/**
* int64 IntervalYearMonth = 16;
* @return Whether the intervalYearMonth field is set.
*/
public boolean hasIntervalYearMonth() {
return valueCase_ == 16;
}
/**
* int64 IntervalYearMonth = 16;
* @return The intervalYearMonth.
*/
public long getIntervalYearMonth() {
if (valueCase_ == 16) {
return (java.lang.Long) value_;
}
return 0L;
}
/**
* int64 IntervalYearMonth = 16;
* @param value The intervalYearMonth to set.
* @return This builder for chaining.
*/
public Builder setIntervalYearMonth(long value) {
valueCase_ = 16;
value_ = value;
onChanged();
return this;
}
/**
* int64 IntervalYearMonth = 16;
* @return This builder for chaining.
*/
public Builder clearIntervalYearMonth() {
if (valueCase_ == 16) {
valueCase_ = 0;
value_ = null;
onChanged();
}
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.IntervalDayTime, cz.proto.Expression.IntervalDayTime.Builder, cz.proto.Expression.IntervalDayTimeOrBuilder> intervalDayTimeBuilder_;
/**
* .cz.proto.IntervalDayTime IntervalDayTime = 17;
* @return Whether the intervalDayTime field is set.
*/
@java.lang.Override
public boolean hasIntervalDayTime() {
return valueCase_ == 17;
}
/**
* .cz.proto.IntervalDayTime IntervalDayTime = 17;
* @return The intervalDayTime.
*/
@java.lang.Override
public cz.proto.Expression.IntervalDayTime getIntervalDayTime() {
if (intervalDayTimeBuilder_ == null) {
if (valueCase_ == 17) {
return (cz.proto.Expression.IntervalDayTime) value_;
}
return cz.proto.Expression.IntervalDayTime.getDefaultInstance();
} else {
if (valueCase_ == 17) {
return intervalDayTimeBuilder_.getMessage();
}
return cz.proto.Expression.IntervalDayTime.getDefaultInstance();
}
}
/**
* .cz.proto.IntervalDayTime IntervalDayTime = 17;
*/
public Builder setIntervalDayTime(cz.proto.Expression.IntervalDayTime value) {
if (intervalDayTimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
value_ = value;
onChanged();
} else {
intervalDayTimeBuilder_.setMessage(value);
}
valueCase_ = 17;
return this;
}
/**
* .cz.proto.IntervalDayTime IntervalDayTime = 17;
*/
public Builder setIntervalDayTime(
cz.proto.Expression.IntervalDayTime.Builder builderForValue) {
if (intervalDayTimeBuilder_ == null) {
value_ = builderForValue.build();
onChanged();
} else {
intervalDayTimeBuilder_.setMessage(builderForValue.build());
}
valueCase_ = 17;
return this;
}
/**
* .cz.proto.IntervalDayTime IntervalDayTime = 17;
*/
public Builder mergeIntervalDayTime(cz.proto.Expression.IntervalDayTime value) {
if (intervalDayTimeBuilder_ == null) {
if (valueCase_ == 17 &&
value_ != cz.proto.Expression.IntervalDayTime.getDefaultInstance()) {
value_ = cz.proto.Expression.IntervalDayTime.newBuilder((cz.proto.Expression.IntervalDayTime) value_)
.mergeFrom(value).buildPartial();
} else {
value_ = value;
}
onChanged();
} else {
if (valueCase_ == 17) {
intervalDayTimeBuilder_.mergeFrom(value);
}
intervalDayTimeBuilder_.setMessage(value);
}
valueCase_ = 17;
return this;
}
/**
* .cz.proto.IntervalDayTime IntervalDayTime = 17;
*/
public Builder clearIntervalDayTime() {
if (intervalDayTimeBuilder_ == null) {
if (valueCase_ == 17) {
valueCase_ = 0;
value_ = null;
onChanged();
}
} else {
if (valueCase_ == 17) {
valueCase_ = 0;
value_ = null;
}
intervalDayTimeBuilder_.clear();
}
return this;
}
/**
* .cz.proto.IntervalDayTime IntervalDayTime = 17;
*/
public cz.proto.Expression.IntervalDayTime.Builder getIntervalDayTimeBuilder() {
return getIntervalDayTimeFieldBuilder().getBuilder();
}
/**
* .cz.proto.IntervalDayTime IntervalDayTime = 17;
*/
@java.lang.Override
public cz.proto.Expression.IntervalDayTimeOrBuilder getIntervalDayTimeOrBuilder() {
if ((valueCase_ == 17) && (intervalDayTimeBuilder_ != null)) {
return intervalDayTimeBuilder_.getMessageOrBuilder();
} else {
if (valueCase_ == 17) {
return (cz.proto.Expression.IntervalDayTime) value_;
}
return cz.proto.Expression.IntervalDayTime.getDefaultInstance();
}
}
/**
* .cz.proto.IntervalDayTime IntervalDayTime = 17;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.IntervalDayTime, cz.proto.Expression.IntervalDayTime.Builder, cz.proto.Expression.IntervalDayTimeOrBuilder>
getIntervalDayTimeFieldBuilder() {
if (intervalDayTimeBuilder_ == null) {
if (!(valueCase_ == 17)) {
value_ = cz.proto.Expression.IntervalDayTime.getDefaultInstance();
}
intervalDayTimeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.IntervalDayTime, cz.proto.Expression.IntervalDayTime.Builder, cz.proto.Expression.IntervalDayTimeOrBuilder>(
(cz.proto.Expression.IntervalDayTime) value_,
getParentForChildren(),
isClean());
value_ = null;
}
valueCase_ = 17;
onChanged();;
return intervalDayTimeBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.ArrayValue, cz.proto.Expression.ArrayValue.Builder, cz.proto.Expression.ArrayValueOrBuilder> arrayBuilder_;
/**
* .cz.proto.ArrayValue array = 100;
* @return Whether the array field is set.
*/
@java.lang.Override
public boolean hasArray() {
return valueCase_ == 100;
}
/**
* .cz.proto.ArrayValue array = 100;
* @return The array.
*/
@java.lang.Override
public cz.proto.Expression.ArrayValue getArray() {
if (arrayBuilder_ == null) {
if (valueCase_ == 100) {
return (cz.proto.Expression.ArrayValue) value_;
}
return cz.proto.Expression.ArrayValue.getDefaultInstance();
} else {
if (valueCase_ == 100) {
return arrayBuilder_.getMessage();
}
return cz.proto.Expression.ArrayValue.getDefaultInstance();
}
}
/**
* .cz.proto.ArrayValue array = 100;
*/
public Builder setArray(cz.proto.Expression.ArrayValue value) {
if (arrayBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
value_ = value;
onChanged();
} else {
arrayBuilder_.setMessage(value);
}
valueCase_ = 100;
return this;
}
/**
* .cz.proto.ArrayValue array = 100;
*/
public Builder setArray(
cz.proto.Expression.ArrayValue.Builder builderForValue) {
if (arrayBuilder_ == null) {
value_ = builderForValue.build();
onChanged();
} else {
arrayBuilder_.setMessage(builderForValue.build());
}
valueCase_ = 100;
return this;
}
/**
* .cz.proto.ArrayValue array = 100;
*/
public Builder mergeArray(cz.proto.Expression.ArrayValue value) {
if (arrayBuilder_ == null) {
if (valueCase_ == 100 &&
value_ != cz.proto.Expression.ArrayValue.getDefaultInstance()) {
value_ = cz.proto.Expression.ArrayValue.newBuilder((cz.proto.Expression.ArrayValue) value_)
.mergeFrom(value).buildPartial();
} else {
value_ = value;
}
onChanged();
} else {
if (valueCase_ == 100) {
arrayBuilder_.mergeFrom(value);
}
arrayBuilder_.setMessage(value);
}
valueCase_ = 100;
return this;
}
/**
* .cz.proto.ArrayValue array = 100;
*/
public Builder clearArray() {
if (arrayBuilder_ == null) {
if (valueCase_ == 100) {
valueCase_ = 0;
value_ = null;
onChanged();
}
} else {
if (valueCase_ == 100) {
valueCase_ = 0;
value_ = null;
}
arrayBuilder_.clear();
}
return this;
}
/**
* .cz.proto.ArrayValue array = 100;
*/
public cz.proto.Expression.ArrayValue.Builder getArrayBuilder() {
return getArrayFieldBuilder().getBuilder();
}
/**
* .cz.proto.ArrayValue array = 100;
*/
@java.lang.Override
public cz.proto.Expression.ArrayValueOrBuilder getArrayOrBuilder() {
if ((valueCase_ == 100) && (arrayBuilder_ != null)) {
return arrayBuilder_.getMessageOrBuilder();
} else {
if (valueCase_ == 100) {
return (cz.proto.Expression.ArrayValue) value_;
}
return cz.proto.Expression.ArrayValue.getDefaultInstance();
}
}
/**
* .cz.proto.ArrayValue array = 100;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.ArrayValue, cz.proto.Expression.ArrayValue.Builder, cz.proto.Expression.ArrayValueOrBuilder>
getArrayFieldBuilder() {
if (arrayBuilder_ == null) {
if (!(valueCase_ == 100)) {
value_ = cz.proto.Expression.ArrayValue.getDefaultInstance();
}
arrayBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.ArrayValue, cz.proto.Expression.ArrayValue.Builder, cz.proto.Expression.ArrayValueOrBuilder>(
(cz.proto.Expression.ArrayValue) value_,
getParentForChildren(),
isClean());
value_ = null;
}
valueCase_ = 100;
onChanged();;
return arrayBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.MapValue, cz.proto.Expression.MapValue.Builder, cz.proto.Expression.MapValueOrBuilder> mapBuilder_;
/**
* .cz.proto.MapValue map = 101;
* @return Whether the map field is set.
*/
@java.lang.Override
public boolean hasMap() {
return valueCase_ == 101;
}
/**
* .cz.proto.MapValue map = 101;
* @return The map.
*/
@java.lang.Override
public cz.proto.Expression.MapValue getMap() {
if (mapBuilder_ == null) {
if (valueCase_ == 101) {
return (cz.proto.Expression.MapValue) value_;
}
return cz.proto.Expression.MapValue.getDefaultInstance();
} else {
if (valueCase_ == 101) {
return mapBuilder_.getMessage();
}
return cz.proto.Expression.MapValue.getDefaultInstance();
}
}
/**
* .cz.proto.MapValue map = 101;
*/
public Builder setMap(cz.proto.Expression.MapValue value) {
if (mapBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
value_ = value;
onChanged();
} else {
mapBuilder_.setMessage(value);
}
valueCase_ = 101;
return this;
}
/**
* .cz.proto.MapValue map = 101;
*/
public Builder setMap(
cz.proto.Expression.MapValue.Builder builderForValue) {
if (mapBuilder_ == null) {
value_ = builderForValue.build();
onChanged();
} else {
mapBuilder_.setMessage(builderForValue.build());
}
valueCase_ = 101;
return this;
}
/**
* .cz.proto.MapValue map = 101;
*/
public Builder mergeMap(cz.proto.Expression.MapValue value) {
if (mapBuilder_ == null) {
if (valueCase_ == 101 &&
value_ != cz.proto.Expression.MapValue.getDefaultInstance()) {
value_ = cz.proto.Expression.MapValue.newBuilder((cz.proto.Expression.MapValue) value_)
.mergeFrom(value).buildPartial();
} else {
value_ = value;
}
onChanged();
} else {
if (valueCase_ == 101) {
mapBuilder_.mergeFrom(value);
}
mapBuilder_.setMessage(value);
}
valueCase_ = 101;
return this;
}
/**
* .cz.proto.MapValue map = 101;
*/
public Builder clearMap() {
if (mapBuilder_ == null) {
if (valueCase_ == 101) {
valueCase_ = 0;
value_ = null;
onChanged();
}
} else {
if (valueCase_ == 101) {
valueCase_ = 0;
value_ = null;
}
mapBuilder_.clear();
}
return this;
}
/**
* .cz.proto.MapValue map = 101;
*/
public cz.proto.Expression.MapValue.Builder getMapBuilder() {
return getMapFieldBuilder().getBuilder();
}
/**
* .cz.proto.MapValue map = 101;
*/
@java.lang.Override
public cz.proto.Expression.MapValueOrBuilder getMapOrBuilder() {
if ((valueCase_ == 101) && (mapBuilder_ != null)) {
return mapBuilder_.getMessageOrBuilder();
} else {
if (valueCase_ == 101) {
return (cz.proto.Expression.MapValue) value_;
}
return cz.proto.Expression.MapValue.getDefaultInstance();
}
}
/**
* .cz.proto.MapValue map = 101;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.MapValue, cz.proto.Expression.MapValue.Builder, cz.proto.Expression.MapValueOrBuilder>
getMapFieldBuilder() {
if (mapBuilder_ == null) {
if (!(valueCase_ == 101)) {
value_ = cz.proto.Expression.MapValue.getDefaultInstance();
}
mapBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.MapValue, cz.proto.Expression.MapValue.Builder, cz.proto.Expression.MapValueOrBuilder>(
(cz.proto.Expression.MapValue) value_,
getParentForChildren(),
isClean());
value_ = null;
}
valueCase_ = 101;
onChanged();;
return mapBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.StructValue, cz.proto.Expression.StructValue.Builder, cz.proto.Expression.StructValueOrBuilder> structBuilder_;
/**
* .cz.proto.StructValue struct = 102;
* @return Whether the struct field is set.
*/
@java.lang.Override
public boolean hasStruct() {
return valueCase_ == 102;
}
/**
* .cz.proto.StructValue struct = 102;
* @return The struct.
*/
@java.lang.Override
public cz.proto.Expression.StructValue getStruct() {
if (structBuilder_ == null) {
if (valueCase_ == 102) {
return (cz.proto.Expression.StructValue) value_;
}
return cz.proto.Expression.StructValue.getDefaultInstance();
} else {
if (valueCase_ == 102) {
return structBuilder_.getMessage();
}
return cz.proto.Expression.StructValue.getDefaultInstance();
}
}
/**
* .cz.proto.StructValue struct = 102;
*/
public Builder setStruct(cz.proto.Expression.StructValue value) {
if (structBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
value_ = value;
onChanged();
} else {
structBuilder_.setMessage(value);
}
valueCase_ = 102;
return this;
}
/**
* .cz.proto.StructValue struct = 102;
*/
public Builder setStruct(
cz.proto.Expression.StructValue.Builder builderForValue) {
if (structBuilder_ == null) {
value_ = builderForValue.build();
onChanged();
} else {
structBuilder_.setMessage(builderForValue.build());
}
valueCase_ = 102;
return this;
}
/**
* .cz.proto.StructValue struct = 102;
*/
public Builder mergeStruct(cz.proto.Expression.StructValue value) {
if (structBuilder_ == null) {
if (valueCase_ == 102 &&
value_ != cz.proto.Expression.StructValue.getDefaultInstance()) {
value_ = cz.proto.Expression.StructValue.newBuilder((cz.proto.Expression.StructValue) value_)
.mergeFrom(value).buildPartial();
} else {
value_ = value;
}
onChanged();
} else {
if (valueCase_ == 102) {
structBuilder_.mergeFrom(value);
}
structBuilder_.setMessage(value);
}
valueCase_ = 102;
return this;
}
/**
* .cz.proto.StructValue struct = 102;
*/
public Builder clearStruct() {
if (structBuilder_ == null) {
if (valueCase_ == 102) {
valueCase_ = 0;
value_ = null;
onChanged();
}
} else {
if (valueCase_ == 102) {
valueCase_ = 0;
value_ = null;
}
structBuilder_.clear();
}
return this;
}
/**
* .cz.proto.StructValue struct = 102;
*/
public cz.proto.Expression.StructValue.Builder getStructBuilder() {
return getStructFieldBuilder().getBuilder();
}
/**
* .cz.proto.StructValue struct = 102;
*/
@java.lang.Override
public cz.proto.Expression.StructValueOrBuilder getStructOrBuilder() {
if ((valueCase_ == 102) && (structBuilder_ != null)) {
return structBuilder_.getMessageOrBuilder();
} else {
if (valueCase_ == 102) {
return (cz.proto.Expression.StructValue) value_;
}
return cz.proto.Expression.StructValue.getDefaultInstance();
}
}
/**
* .cz.proto.StructValue struct = 102;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.StructValue, cz.proto.Expression.StructValue.Builder, cz.proto.Expression.StructValueOrBuilder>
getStructFieldBuilder() {
if (structBuilder_ == null) {
if (!(valueCase_ == 102)) {
value_ = cz.proto.Expression.StructValue.getDefaultInstance();
}
structBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.StructValue, cz.proto.Expression.StructValue.Builder, cz.proto.Expression.StructValueOrBuilder>(
(cz.proto.Expression.StructValue) value_,
getParentForChildren(),
isClean());
value_ = null;
}
valueCase_ = 102;
onChanged();;
return structBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.BitmapValue, cz.proto.Expression.BitmapValue.Builder, cz.proto.Expression.BitmapValueOrBuilder> bitmapBuilder_;
/**
* .cz.proto.BitmapValue bitmap = 103;
* @return Whether the bitmap field is set.
*/
@java.lang.Override
public boolean hasBitmap() {
return valueCase_ == 103;
}
/**
* .cz.proto.BitmapValue bitmap = 103;
* @return The bitmap.
*/
@java.lang.Override
public cz.proto.Expression.BitmapValue getBitmap() {
if (bitmapBuilder_ == null) {
if (valueCase_ == 103) {
return (cz.proto.Expression.BitmapValue) value_;
}
return cz.proto.Expression.BitmapValue.getDefaultInstance();
} else {
if (valueCase_ == 103) {
return bitmapBuilder_.getMessage();
}
return cz.proto.Expression.BitmapValue.getDefaultInstance();
}
}
/**
* .cz.proto.BitmapValue bitmap = 103;
*/
public Builder setBitmap(cz.proto.Expression.BitmapValue value) {
if (bitmapBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
value_ = value;
onChanged();
} else {
bitmapBuilder_.setMessage(value);
}
valueCase_ = 103;
return this;
}
/**
* .cz.proto.BitmapValue bitmap = 103;
*/
public Builder setBitmap(
cz.proto.Expression.BitmapValue.Builder builderForValue) {
if (bitmapBuilder_ == null) {
value_ = builderForValue.build();
onChanged();
} else {
bitmapBuilder_.setMessage(builderForValue.build());
}
valueCase_ = 103;
return this;
}
/**
* .cz.proto.BitmapValue bitmap = 103;
*/
public Builder mergeBitmap(cz.proto.Expression.BitmapValue value) {
if (bitmapBuilder_ == null) {
if (valueCase_ == 103 &&
value_ != cz.proto.Expression.BitmapValue.getDefaultInstance()) {
value_ = cz.proto.Expression.BitmapValue.newBuilder((cz.proto.Expression.BitmapValue) value_)
.mergeFrom(value).buildPartial();
} else {
value_ = value;
}
onChanged();
} else {
if (valueCase_ == 103) {
bitmapBuilder_.mergeFrom(value);
}
bitmapBuilder_.setMessage(value);
}
valueCase_ = 103;
return this;
}
/**
* .cz.proto.BitmapValue bitmap = 103;
*/
public Builder clearBitmap() {
if (bitmapBuilder_ == null) {
if (valueCase_ == 103) {
valueCase_ = 0;
value_ = null;
onChanged();
}
} else {
if (valueCase_ == 103) {
valueCase_ = 0;
value_ = null;
}
bitmapBuilder_.clear();
}
return this;
}
/**
* .cz.proto.BitmapValue bitmap = 103;
*/
public cz.proto.Expression.BitmapValue.Builder getBitmapBuilder() {
return getBitmapFieldBuilder().getBuilder();
}
/**
* .cz.proto.BitmapValue bitmap = 103;
*/
@java.lang.Override
public cz.proto.Expression.BitmapValueOrBuilder getBitmapOrBuilder() {
if ((valueCase_ == 103) && (bitmapBuilder_ != null)) {
return bitmapBuilder_.getMessageOrBuilder();
} else {
if (valueCase_ == 103) {
return (cz.proto.Expression.BitmapValue) value_;
}
return cz.proto.Expression.BitmapValue.getDefaultInstance();
}
}
/**
* .cz.proto.BitmapValue bitmap = 103;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.BitmapValue, cz.proto.Expression.BitmapValue.Builder, cz.proto.Expression.BitmapValueOrBuilder>
getBitmapFieldBuilder() {
if (bitmapBuilder_ == null) {
if (!(valueCase_ == 103)) {
value_ = cz.proto.Expression.BitmapValue.getDefaultInstance();
}
bitmapBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.BitmapValue, cz.proto.Expression.BitmapValue.Builder, cz.proto.Expression.BitmapValueOrBuilder>(
(cz.proto.Expression.BitmapValue) value_,
getParentForChildren(),
isClean());
value_ = null;
}
valueCase_ = 103;
onChanged();;
return bitmapBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.Constant)
}
// @@protoc_insertion_point(class_scope:cz.proto.Constant)
private static final cz.proto.Expression.Constant DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.Expression.Constant();
}
public static cz.proto.Expression.Constant getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Constant parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Constant(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.Expression.Constant getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface SubFieldPruningOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.SubFieldPruning)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.Constant id = 1;
* @return Whether the id field is set.
*/
boolean hasId();
/**
* .cz.proto.Constant id = 1;
* @return The id.
*/
cz.proto.Expression.Constant getId();
/**
* .cz.proto.Constant id = 1;
*/
cz.proto.Expression.ConstantOrBuilder getIdOrBuilder();
/**
* .cz.proto.SubFieldsPruning subfields = 2;
* @return Whether the subfields field is set.
*/
boolean hasSubfields();
/**
* .cz.proto.SubFieldsPruning subfields = 2;
* @return The subfields.
*/
cz.proto.Expression.SubFieldsPruning getSubfields();
/**
* .cz.proto.SubFieldsPruning subfields = 2;
*/
cz.proto.Expression.SubFieldsPruningOrBuilder getSubfieldsOrBuilder();
}
/**
* Protobuf type {@code cz.proto.SubFieldPruning}
*/
public static final class SubFieldPruning extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.SubFieldPruning)
SubFieldPruningOrBuilder {
private static final long serialVersionUID = 0L;
// Use SubFieldPruning.newBuilder() to construct.
private SubFieldPruning(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SubFieldPruning() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new SubFieldPruning();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SubFieldPruning(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.Expression.Constant.Builder subBuilder = null;
if (id_ != null) {
subBuilder = id_.toBuilder();
}
id_ = input.readMessage(cz.proto.Expression.Constant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(id_);
id_ = subBuilder.buildPartial();
}
break;
}
case 18: {
cz.proto.Expression.SubFieldsPruning.Builder subBuilder = null;
if (subfields_ != null) {
subBuilder = subfields_.toBuilder();
}
subfields_ = input.readMessage(cz.proto.Expression.SubFieldsPruning.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(subfields_);
subfields_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.Expression.internal_static_cz_proto_SubFieldPruning_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.Expression.internal_static_cz_proto_SubFieldPruning_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.Expression.SubFieldPruning.class, cz.proto.Expression.SubFieldPruning.Builder.class);
}
public static final int ID_FIELD_NUMBER = 1;
private cz.proto.Expression.Constant id_;
/**
* .cz.proto.Constant id = 1;
* @return Whether the id field is set.
*/
@java.lang.Override
public boolean hasId() {
return id_ != null;
}
/**
* .cz.proto.Constant id = 1;
* @return The id.
*/
@java.lang.Override
public cz.proto.Expression.Constant getId() {
return id_ == null ? cz.proto.Expression.Constant.getDefaultInstance() : id_;
}
/**
* .cz.proto.Constant id = 1;
*/
@java.lang.Override
public cz.proto.Expression.ConstantOrBuilder getIdOrBuilder() {
return getId();
}
public static final int SUBFIELDS_FIELD_NUMBER = 2;
private cz.proto.Expression.SubFieldsPruning subfields_;
/**
* .cz.proto.SubFieldsPruning subfields = 2;
* @return Whether the subfields field is set.
*/
@java.lang.Override
public boolean hasSubfields() {
return subfields_ != null;
}
/**
* .cz.proto.SubFieldsPruning subfields = 2;
* @return The subfields.
*/
@java.lang.Override
public cz.proto.Expression.SubFieldsPruning getSubfields() {
return subfields_ == null ? cz.proto.Expression.SubFieldsPruning.getDefaultInstance() : subfields_;
}
/**
* .cz.proto.SubFieldsPruning subfields = 2;
*/
@java.lang.Override
public cz.proto.Expression.SubFieldsPruningOrBuilder getSubfieldsOrBuilder() {
return getSubfields();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (id_ != null) {
output.writeMessage(1, getId());
}
if (subfields_ != null) {
output.writeMessage(2, getSubfields());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (id_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getId());
}
if (subfields_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getSubfields());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.Expression.SubFieldPruning)) {
return super.equals(obj);
}
cz.proto.Expression.SubFieldPruning other = (cz.proto.Expression.SubFieldPruning) obj;
if (hasId() != other.hasId()) return false;
if (hasId()) {
if (!getId()
.equals(other.getId())) return false;
}
if (hasSubfields() != other.hasSubfields()) return false;
if (hasSubfields()) {
if (!getSubfields()
.equals(other.getSubfields())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasId()) {
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId().hashCode();
}
if (hasSubfields()) {
hash = (37 * hash) + SUBFIELDS_FIELD_NUMBER;
hash = (53 * hash) + getSubfields().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.Expression.SubFieldPruning parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.SubFieldPruning parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.SubFieldPruning parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.SubFieldPruning parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.SubFieldPruning parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.SubFieldPruning parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.SubFieldPruning parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.Expression.SubFieldPruning parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.Expression.SubFieldPruning parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.Expression.SubFieldPruning parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.Expression.SubFieldPruning parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.Expression.SubFieldPruning parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.Expression.SubFieldPruning prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.SubFieldPruning}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.SubFieldPruning)
cz.proto.Expression.SubFieldPruningOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.Expression.internal_static_cz_proto_SubFieldPruning_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.Expression.internal_static_cz_proto_SubFieldPruning_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.Expression.SubFieldPruning.class, cz.proto.Expression.SubFieldPruning.Builder.class);
}
// Construct using cz.proto.Expression.SubFieldPruning.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (idBuilder_ == null) {
id_ = null;
} else {
id_ = null;
idBuilder_ = null;
}
if (subfieldsBuilder_ == null) {
subfields_ = null;
} else {
subfields_ = null;
subfieldsBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.Expression.internal_static_cz_proto_SubFieldPruning_descriptor;
}
@java.lang.Override
public cz.proto.Expression.SubFieldPruning getDefaultInstanceForType() {
return cz.proto.Expression.SubFieldPruning.getDefaultInstance();
}
@java.lang.Override
public cz.proto.Expression.SubFieldPruning build() {
cz.proto.Expression.SubFieldPruning result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.Expression.SubFieldPruning buildPartial() {
cz.proto.Expression.SubFieldPruning result = new cz.proto.Expression.SubFieldPruning(this);
if (idBuilder_ == null) {
result.id_ = id_;
} else {
result.id_ = idBuilder_.build();
}
if (subfieldsBuilder_ == null) {
result.subfields_ = subfields_;
} else {
result.subfields_ = subfieldsBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.Expression.SubFieldPruning) {
return mergeFrom((cz.proto.Expression.SubFieldPruning)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.Expression.SubFieldPruning other) {
if (other == cz.proto.Expression.SubFieldPruning.getDefaultInstance()) return this;
if (other.hasId()) {
mergeId(other.getId());
}
if (other.hasSubfields()) {
mergeSubfields(other.getSubfields());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.Expression.SubFieldPruning parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.Expression.SubFieldPruning) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cz.proto.Expression.Constant id_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.Constant, cz.proto.Expression.Constant.Builder, cz.proto.Expression.ConstantOrBuilder> idBuilder_;
/**
* .cz.proto.Constant id = 1;
* @return Whether the id field is set.
*/
public boolean hasId() {
return idBuilder_ != null || id_ != null;
}
/**
* .cz.proto.Constant id = 1;
* @return The id.
*/
public cz.proto.Expression.Constant getId() {
if (idBuilder_ == null) {
return id_ == null ? cz.proto.Expression.Constant.getDefaultInstance() : id_;
} else {
return idBuilder_.getMessage();
}
}
/**
* .cz.proto.Constant id = 1;
*/
public Builder setId(cz.proto.Expression.Constant value) {
if (idBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
id_ = value;
onChanged();
} else {
idBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.Constant id = 1;
*/
public Builder setId(
cz.proto.Expression.Constant.Builder builderForValue) {
if (idBuilder_ == null) {
id_ = builderForValue.build();
onChanged();
} else {
idBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.Constant id = 1;
*/
public Builder mergeId(cz.proto.Expression.Constant value) {
if (idBuilder_ == null) {
if (id_ != null) {
id_ =
cz.proto.Expression.Constant.newBuilder(id_).mergeFrom(value).buildPartial();
} else {
id_ = value;
}
onChanged();
} else {
idBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.Constant id = 1;
*/
public Builder clearId() {
if (idBuilder_ == null) {
id_ = null;
onChanged();
} else {
id_ = null;
idBuilder_ = null;
}
return this;
}
/**
* .cz.proto.Constant id = 1;
*/
public cz.proto.Expression.Constant.Builder getIdBuilder() {
onChanged();
return getIdFieldBuilder().getBuilder();
}
/**
* .cz.proto.Constant id = 1;
*/
public cz.proto.Expression.ConstantOrBuilder getIdOrBuilder() {
if (idBuilder_ != null) {
return idBuilder_.getMessageOrBuilder();
} else {
return id_ == null ?
cz.proto.Expression.Constant.getDefaultInstance() : id_;
}
}
/**
* .cz.proto.Constant id = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.Constant, cz.proto.Expression.Constant.Builder, cz.proto.Expression.ConstantOrBuilder>
getIdFieldBuilder() {
if (idBuilder_ == null) {
idBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.Constant, cz.proto.Expression.Constant.Builder, cz.proto.Expression.ConstantOrBuilder>(
getId(),
getParentForChildren(),
isClean());
id_ = null;
}
return idBuilder_;
}
private cz.proto.Expression.SubFieldsPruning subfields_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.SubFieldsPruning, cz.proto.Expression.SubFieldsPruning.Builder, cz.proto.Expression.SubFieldsPruningOrBuilder> subfieldsBuilder_;
/**
* .cz.proto.SubFieldsPruning subfields = 2;
* @return Whether the subfields field is set.
*/
public boolean hasSubfields() {
return subfieldsBuilder_ != null || subfields_ != null;
}
/**
* .cz.proto.SubFieldsPruning subfields = 2;
* @return The subfields.
*/
public cz.proto.Expression.SubFieldsPruning getSubfields() {
if (subfieldsBuilder_ == null) {
return subfields_ == null ? cz.proto.Expression.SubFieldsPruning.getDefaultInstance() : subfields_;
} else {
return subfieldsBuilder_.getMessage();
}
}
/**
* .cz.proto.SubFieldsPruning subfields = 2;
*/
public Builder setSubfields(cz.proto.Expression.SubFieldsPruning value) {
if (subfieldsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
subfields_ = value;
onChanged();
} else {
subfieldsBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.SubFieldsPruning subfields = 2;
*/
public Builder setSubfields(
cz.proto.Expression.SubFieldsPruning.Builder builderForValue) {
if (subfieldsBuilder_ == null) {
subfields_ = builderForValue.build();
onChanged();
} else {
subfieldsBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.SubFieldsPruning subfields = 2;
*/
public Builder mergeSubfields(cz.proto.Expression.SubFieldsPruning value) {
if (subfieldsBuilder_ == null) {
if (subfields_ != null) {
subfields_ =
cz.proto.Expression.SubFieldsPruning.newBuilder(subfields_).mergeFrom(value).buildPartial();
} else {
subfields_ = value;
}
onChanged();
} else {
subfieldsBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.SubFieldsPruning subfields = 2;
*/
public Builder clearSubfields() {
if (subfieldsBuilder_ == null) {
subfields_ = null;
onChanged();
} else {
subfields_ = null;
subfieldsBuilder_ = null;
}
return this;
}
/**
* .cz.proto.SubFieldsPruning subfields = 2;
*/
public cz.proto.Expression.SubFieldsPruning.Builder getSubfieldsBuilder() {
onChanged();
return getSubfieldsFieldBuilder().getBuilder();
}
/**
* .cz.proto.SubFieldsPruning subfields = 2;
*/
public cz.proto.Expression.SubFieldsPruningOrBuilder getSubfieldsOrBuilder() {
if (subfieldsBuilder_ != null) {
return subfieldsBuilder_.getMessageOrBuilder();
} else {
return subfields_ == null ?
cz.proto.Expression.SubFieldsPruning.getDefaultInstance() : subfields_;
}
}
/**
* .cz.proto.SubFieldsPruning subfields = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.SubFieldsPruning, cz.proto.Expression.SubFieldsPruning.Builder, cz.proto.Expression.SubFieldsPruningOrBuilder>
getSubfieldsFieldBuilder() {
if (subfieldsBuilder_ == null) {
subfieldsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.SubFieldsPruning, cz.proto.Expression.SubFieldsPruning.Builder, cz.proto.Expression.SubFieldsPruningOrBuilder>(
getSubfields(),
getParentForChildren(),
isClean());
subfields_ = null;
}
return subfieldsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.SubFieldPruning)
}
// @@protoc_insertion_point(class_scope:cz.proto.SubFieldPruning)
private static final cz.proto.Expression.SubFieldPruning DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.Expression.SubFieldPruning();
}
public static cz.proto.Expression.SubFieldPruning getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public SubFieldPruning parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SubFieldPruning(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.Expression.SubFieldPruning getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface SubFieldsPruningOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.SubFieldsPruning)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .cz.proto.SubFieldPruning fields = 1;
*/
java.util.List
getFieldsList();
/**
* repeated .cz.proto.SubFieldPruning fields = 1;
*/
cz.proto.Expression.SubFieldPruning getFields(int index);
/**
* repeated .cz.proto.SubFieldPruning fields = 1;
*/
int getFieldsCount();
/**
* repeated .cz.proto.SubFieldPruning fields = 1;
*/
java.util.List extends cz.proto.Expression.SubFieldPruningOrBuilder>
getFieldsOrBuilderList();
/**
* repeated .cz.proto.SubFieldPruning fields = 1;
*/
cz.proto.Expression.SubFieldPruningOrBuilder getFieldsOrBuilder(
int index);
/**
* bool reserve_size = 2;
* @return The reserveSize.
*/
boolean getReserveSize();
}
/**
* Protobuf type {@code cz.proto.SubFieldsPruning}
*/
public static final class SubFieldsPruning extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.SubFieldsPruning)
SubFieldsPruningOrBuilder {
private static final long serialVersionUID = 0L;
// Use SubFieldsPruning.newBuilder() to construct.
private SubFieldsPruning(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SubFieldsPruning() {
fields_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new SubFieldsPruning();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SubFieldsPruning(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
fields_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
fields_.add(
input.readMessage(cz.proto.Expression.SubFieldPruning.parser(), extensionRegistry));
break;
}
case 16: {
reserveSize_ = input.readBool();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
fields_ = java.util.Collections.unmodifiableList(fields_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.Expression.internal_static_cz_proto_SubFieldsPruning_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.Expression.internal_static_cz_proto_SubFieldsPruning_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.Expression.SubFieldsPruning.class, cz.proto.Expression.SubFieldsPruning.Builder.class);
}
public static final int FIELDS_FIELD_NUMBER = 1;
private java.util.List fields_;
/**
* repeated .cz.proto.SubFieldPruning fields = 1;
*/
@java.lang.Override
public java.util.List getFieldsList() {
return fields_;
}
/**
* repeated .cz.proto.SubFieldPruning fields = 1;
*/
@java.lang.Override
public java.util.List extends cz.proto.Expression.SubFieldPruningOrBuilder>
getFieldsOrBuilderList() {
return fields_;
}
/**
* repeated .cz.proto.SubFieldPruning fields = 1;
*/
@java.lang.Override
public int getFieldsCount() {
return fields_.size();
}
/**
* repeated .cz.proto.SubFieldPruning fields = 1;
*/
@java.lang.Override
public cz.proto.Expression.SubFieldPruning getFields(int index) {
return fields_.get(index);
}
/**
* repeated .cz.proto.SubFieldPruning fields = 1;
*/
@java.lang.Override
public cz.proto.Expression.SubFieldPruningOrBuilder getFieldsOrBuilder(
int index) {
return fields_.get(index);
}
public static final int RESERVE_SIZE_FIELD_NUMBER = 2;
private boolean reserveSize_;
/**
* bool reserve_size = 2;
* @return The reserveSize.
*/
@java.lang.Override
public boolean getReserveSize() {
return reserveSize_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < fields_.size(); i++) {
output.writeMessage(1, fields_.get(i));
}
if (reserveSize_ != false) {
output.writeBool(2, reserveSize_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < fields_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, fields_.get(i));
}
if (reserveSize_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(2, reserveSize_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.Expression.SubFieldsPruning)) {
return super.equals(obj);
}
cz.proto.Expression.SubFieldsPruning other = (cz.proto.Expression.SubFieldsPruning) obj;
if (!getFieldsList()
.equals(other.getFieldsList())) return false;
if (getReserveSize()
!= other.getReserveSize()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getFieldsCount() > 0) {
hash = (37 * hash) + FIELDS_FIELD_NUMBER;
hash = (53 * hash) + getFieldsList().hashCode();
}
hash = (37 * hash) + RESERVE_SIZE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getReserveSize());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.Expression.SubFieldsPruning parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.SubFieldsPruning parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.SubFieldsPruning parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.SubFieldsPruning parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.SubFieldsPruning parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.SubFieldsPruning parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.SubFieldsPruning parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.Expression.SubFieldsPruning parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.Expression.SubFieldsPruning parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.Expression.SubFieldsPruning parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.Expression.SubFieldsPruning parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.Expression.SubFieldsPruning parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.Expression.SubFieldsPruning prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.SubFieldsPruning}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.SubFieldsPruning)
cz.proto.Expression.SubFieldsPruningOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.Expression.internal_static_cz_proto_SubFieldsPruning_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.Expression.internal_static_cz_proto_SubFieldsPruning_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.Expression.SubFieldsPruning.class, cz.proto.Expression.SubFieldsPruning.Builder.class);
}
// Construct using cz.proto.Expression.SubFieldsPruning.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getFieldsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (fieldsBuilder_ == null) {
fields_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
fieldsBuilder_.clear();
}
reserveSize_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.Expression.internal_static_cz_proto_SubFieldsPruning_descriptor;
}
@java.lang.Override
public cz.proto.Expression.SubFieldsPruning getDefaultInstanceForType() {
return cz.proto.Expression.SubFieldsPruning.getDefaultInstance();
}
@java.lang.Override
public cz.proto.Expression.SubFieldsPruning build() {
cz.proto.Expression.SubFieldsPruning result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.Expression.SubFieldsPruning buildPartial() {
cz.proto.Expression.SubFieldsPruning result = new cz.proto.Expression.SubFieldsPruning(this);
int from_bitField0_ = bitField0_;
if (fieldsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
fields_ = java.util.Collections.unmodifiableList(fields_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.fields_ = fields_;
} else {
result.fields_ = fieldsBuilder_.build();
}
result.reserveSize_ = reserveSize_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.Expression.SubFieldsPruning) {
return mergeFrom((cz.proto.Expression.SubFieldsPruning)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.Expression.SubFieldsPruning other) {
if (other == cz.proto.Expression.SubFieldsPruning.getDefaultInstance()) return this;
if (fieldsBuilder_ == null) {
if (!other.fields_.isEmpty()) {
if (fields_.isEmpty()) {
fields_ = other.fields_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureFieldsIsMutable();
fields_.addAll(other.fields_);
}
onChanged();
}
} else {
if (!other.fields_.isEmpty()) {
if (fieldsBuilder_.isEmpty()) {
fieldsBuilder_.dispose();
fieldsBuilder_ = null;
fields_ = other.fields_;
bitField0_ = (bitField0_ & ~0x00000001);
fieldsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getFieldsFieldBuilder() : null;
} else {
fieldsBuilder_.addAllMessages(other.fields_);
}
}
}
if (other.getReserveSize() != false) {
setReserveSize(other.getReserveSize());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.Expression.SubFieldsPruning parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.Expression.SubFieldsPruning) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List fields_ =
java.util.Collections.emptyList();
private void ensureFieldsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
fields_ = new java.util.ArrayList(fields_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.Expression.SubFieldPruning, cz.proto.Expression.SubFieldPruning.Builder, cz.proto.Expression.SubFieldPruningOrBuilder> fieldsBuilder_;
/**
* repeated .cz.proto.SubFieldPruning fields = 1;
*/
public java.util.List getFieldsList() {
if (fieldsBuilder_ == null) {
return java.util.Collections.unmodifiableList(fields_);
} else {
return fieldsBuilder_.getMessageList();
}
}
/**
* repeated .cz.proto.SubFieldPruning fields = 1;
*/
public int getFieldsCount() {
if (fieldsBuilder_ == null) {
return fields_.size();
} else {
return fieldsBuilder_.getCount();
}
}
/**
* repeated .cz.proto.SubFieldPruning fields = 1;
*/
public cz.proto.Expression.SubFieldPruning getFields(int index) {
if (fieldsBuilder_ == null) {
return fields_.get(index);
} else {
return fieldsBuilder_.getMessage(index);
}
}
/**
* repeated .cz.proto.SubFieldPruning fields = 1;
*/
public Builder setFields(
int index, cz.proto.Expression.SubFieldPruning value) {
if (fieldsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFieldsIsMutable();
fields_.set(index, value);
onChanged();
} else {
fieldsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .cz.proto.SubFieldPruning fields = 1;
*/
public Builder setFields(
int index, cz.proto.Expression.SubFieldPruning.Builder builderForValue) {
if (fieldsBuilder_ == null) {
ensureFieldsIsMutable();
fields_.set(index, builderForValue.build());
onChanged();
} else {
fieldsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.SubFieldPruning fields = 1;
*/
public Builder addFields(cz.proto.Expression.SubFieldPruning value) {
if (fieldsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFieldsIsMutable();
fields_.add(value);
onChanged();
} else {
fieldsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .cz.proto.SubFieldPruning fields = 1;
*/
public Builder addFields(
int index, cz.proto.Expression.SubFieldPruning value) {
if (fieldsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFieldsIsMutable();
fields_.add(index, value);
onChanged();
} else {
fieldsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .cz.proto.SubFieldPruning fields = 1;
*/
public Builder addFields(
cz.proto.Expression.SubFieldPruning.Builder builderForValue) {
if (fieldsBuilder_ == null) {
ensureFieldsIsMutable();
fields_.add(builderForValue.build());
onChanged();
} else {
fieldsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.SubFieldPruning fields = 1;
*/
public Builder addFields(
int index, cz.proto.Expression.SubFieldPruning.Builder builderForValue) {
if (fieldsBuilder_ == null) {
ensureFieldsIsMutable();
fields_.add(index, builderForValue.build());
onChanged();
} else {
fieldsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.SubFieldPruning fields = 1;
*/
public Builder addAllFields(
java.lang.Iterable extends cz.proto.Expression.SubFieldPruning> values) {
if (fieldsBuilder_ == null) {
ensureFieldsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, fields_);
onChanged();
} else {
fieldsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .cz.proto.SubFieldPruning fields = 1;
*/
public Builder clearFields() {
if (fieldsBuilder_ == null) {
fields_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
fieldsBuilder_.clear();
}
return this;
}
/**
* repeated .cz.proto.SubFieldPruning fields = 1;
*/
public Builder removeFields(int index) {
if (fieldsBuilder_ == null) {
ensureFieldsIsMutable();
fields_.remove(index);
onChanged();
} else {
fieldsBuilder_.remove(index);
}
return this;
}
/**
* repeated .cz.proto.SubFieldPruning fields = 1;
*/
public cz.proto.Expression.SubFieldPruning.Builder getFieldsBuilder(
int index) {
return getFieldsFieldBuilder().getBuilder(index);
}
/**
* repeated .cz.proto.SubFieldPruning fields = 1;
*/
public cz.proto.Expression.SubFieldPruningOrBuilder getFieldsOrBuilder(
int index) {
if (fieldsBuilder_ == null) {
return fields_.get(index); } else {
return fieldsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .cz.proto.SubFieldPruning fields = 1;
*/
public java.util.List extends cz.proto.Expression.SubFieldPruningOrBuilder>
getFieldsOrBuilderList() {
if (fieldsBuilder_ != null) {
return fieldsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(fields_);
}
}
/**
* repeated .cz.proto.SubFieldPruning fields = 1;
*/
public cz.proto.Expression.SubFieldPruning.Builder addFieldsBuilder() {
return getFieldsFieldBuilder().addBuilder(
cz.proto.Expression.SubFieldPruning.getDefaultInstance());
}
/**
* repeated .cz.proto.SubFieldPruning fields = 1;
*/
public cz.proto.Expression.SubFieldPruning.Builder addFieldsBuilder(
int index) {
return getFieldsFieldBuilder().addBuilder(
index, cz.proto.Expression.SubFieldPruning.getDefaultInstance());
}
/**
* repeated .cz.proto.SubFieldPruning fields = 1;
*/
public java.util.List
getFieldsBuilderList() {
return getFieldsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.Expression.SubFieldPruning, cz.proto.Expression.SubFieldPruning.Builder, cz.proto.Expression.SubFieldPruningOrBuilder>
getFieldsFieldBuilder() {
if (fieldsBuilder_ == null) {
fieldsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.Expression.SubFieldPruning, cz.proto.Expression.SubFieldPruning.Builder, cz.proto.Expression.SubFieldPruningOrBuilder>(
fields_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
fields_ = null;
}
return fieldsBuilder_;
}
private boolean reserveSize_ ;
/**
* bool reserve_size = 2;
* @return The reserveSize.
*/
@java.lang.Override
public boolean getReserveSize() {
return reserveSize_;
}
/**
* bool reserve_size = 2;
* @param value The reserveSize to set.
* @return This builder for chaining.
*/
public Builder setReserveSize(boolean value) {
reserveSize_ = value;
onChanged();
return this;
}
/**
* bool reserve_size = 2;
* @return This builder for chaining.
*/
public Builder clearReserveSize() {
reserveSize_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.SubFieldsPruning)
}
// @@protoc_insertion_point(class_scope:cz.proto.SubFieldsPruning)
private static final cz.proto.Expression.SubFieldsPruning DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.Expression.SubFieldsPruning();
}
public static cz.proto.Expression.SubFieldsPruning getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public SubFieldsPruning parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SubFieldsPruning(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.Expression.SubFieldsPruning getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ReferenceOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.Reference)
com.google.protobuf.MessageOrBuilder {
/**
* uint64 id = 1;
* @return The id.
*/
long getId();
/**
* bool local = 2;
* @return The local.
*/
boolean getLocal();
/**
* string from = 3;
* @return The from.
*/
java.lang.String getFrom();
/**
* string from = 3;
* @return The bytes for from.
*/
com.google.protobuf.ByteString
getFromBytes();
/**
* string name = 4;
* @return The name.
*/
java.lang.String getName();
/**
* string name = 4;
* @return The bytes for name.
*/
com.google.protobuf.ByteString
getNameBytes();
/**
* .cz.proto.ReferenceType ref_type = 5;
* @return The enum numeric value on the wire for refType.
*/
int getRefTypeValue();
/**
* .cz.proto.ReferenceType ref_type = 5;
* @return The refType.
*/
cz.proto.Expression.ReferenceType getRefType();
}
/**
* Protobuf type {@code cz.proto.Reference}
*/
public static final class Reference extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.Reference)
ReferenceOrBuilder {
private static final long serialVersionUID = 0L;
// Use Reference.newBuilder() to construct.
private Reference(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Reference() {
from_ = "";
name_ = "";
refType_ = 0;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Reference();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Reference(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
id_ = input.readUInt64();
break;
}
case 16: {
local_ = input.readBool();
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
from_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
name_ = s;
break;
}
case 40: {
int rawValue = input.readEnum();
refType_ = rawValue;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.Expression.internal_static_cz_proto_Reference_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.Expression.internal_static_cz_proto_Reference_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.Expression.Reference.class, cz.proto.Expression.Reference.Builder.class);
}
public static final int ID_FIELD_NUMBER = 1;
private long id_;
/**
* uint64 id = 1;
* @return The id.
*/
@java.lang.Override
public long getId() {
return id_;
}
public static final int LOCAL_FIELD_NUMBER = 2;
private boolean local_;
/**
* bool local = 2;
* @return The local.
*/
@java.lang.Override
public boolean getLocal() {
return local_;
}
public static final int FROM_FIELD_NUMBER = 3;
private volatile java.lang.Object from_;
/**
* string from = 3;
* @return The from.
*/
@java.lang.Override
public java.lang.String getFrom() {
java.lang.Object ref = from_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
from_ = s;
return s;
}
}
/**
* string from = 3;
* @return The bytes for from.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getFromBytes() {
java.lang.Object ref = from_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
from_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NAME_FIELD_NUMBER = 4;
private volatile java.lang.Object name_;
/**
* string name = 4;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
* string name = 4;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int REF_TYPE_FIELD_NUMBER = 5;
private int refType_;
/**
* .cz.proto.ReferenceType ref_type = 5;
* @return The enum numeric value on the wire for refType.
*/
@java.lang.Override public int getRefTypeValue() {
return refType_;
}
/**
* .cz.proto.ReferenceType ref_type = 5;
* @return The refType.
*/
@java.lang.Override public cz.proto.Expression.ReferenceType getRefType() {
@SuppressWarnings("deprecation")
cz.proto.Expression.ReferenceType result = cz.proto.Expression.ReferenceType.valueOf(refType_);
return result == null ? cz.proto.Expression.ReferenceType.UNRECOGNIZED : result;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (id_ != 0L) {
output.writeUInt64(1, id_);
}
if (local_ != false) {
output.writeBool(2, local_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(from_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, from_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(name_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, name_);
}
if (refType_ != cz.proto.Expression.ReferenceType.LOGICAL_FIELD.getNumber()) {
output.writeEnum(5, refType_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (id_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(1, id_);
}
if (local_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(2, local_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(from_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, from_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(name_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, name_);
}
if (refType_ != cz.proto.Expression.ReferenceType.LOGICAL_FIELD.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(5, refType_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.Expression.Reference)) {
return super.equals(obj);
}
cz.proto.Expression.Reference other = (cz.proto.Expression.Reference) obj;
if (getId()
!= other.getId()) return false;
if (getLocal()
!= other.getLocal()) return false;
if (!getFrom()
.equals(other.getFrom())) return false;
if (!getName()
.equals(other.getName())) return false;
if (refType_ != other.refType_) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getId());
hash = (37 * hash) + LOCAL_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getLocal());
hash = (37 * hash) + FROM_FIELD_NUMBER;
hash = (53 * hash) + getFrom().hashCode();
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
hash = (37 * hash) + REF_TYPE_FIELD_NUMBER;
hash = (53 * hash) + refType_;
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.Expression.Reference parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.Reference parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.Reference parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.Reference parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.Reference parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.Reference parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.Reference parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.Expression.Reference parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.Expression.Reference parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.Expression.Reference parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.Expression.Reference parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.Expression.Reference parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.Expression.Reference prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.Reference}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.Reference)
cz.proto.Expression.ReferenceOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.Expression.internal_static_cz_proto_Reference_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.Expression.internal_static_cz_proto_Reference_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.Expression.Reference.class, cz.proto.Expression.Reference.Builder.class);
}
// Construct using cz.proto.Expression.Reference.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
id_ = 0L;
local_ = false;
from_ = "";
name_ = "";
refType_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.Expression.internal_static_cz_proto_Reference_descriptor;
}
@java.lang.Override
public cz.proto.Expression.Reference getDefaultInstanceForType() {
return cz.proto.Expression.Reference.getDefaultInstance();
}
@java.lang.Override
public cz.proto.Expression.Reference build() {
cz.proto.Expression.Reference result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.Expression.Reference buildPartial() {
cz.proto.Expression.Reference result = new cz.proto.Expression.Reference(this);
result.id_ = id_;
result.local_ = local_;
result.from_ = from_;
result.name_ = name_;
result.refType_ = refType_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.Expression.Reference) {
return mergeFrom((cz.proto.Expression.Reference)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.Expression.Reference other) {
if (other == cz.proto.Expression.Reference.getDefaultInstance()) return this;
if (other.getId() != 0L) {
setId(other.getId());
}
if (other.getLocal() != false) {
setLocal(other.getLocal());
}
if (!other.getFrom().isEmpty()) {
from_ = other.from_;
onChanged();
}
if (!other.getName().isEmpty()) {
name_ = other.name_;
onChanged();
}
if (other.refType_ != 0) {
setRefTypeValue(other.getRefTypeValue());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.Expression.Reference parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.Expression.Reference) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long id_ ;
/**
* uint64 id = 1;
* @return The id.
*/
@java.lang.Override
public long getId() {
return id_;
}
/**
* uint64 id = 1;
* @param value The id to set.
* @return This builder for chaining.
*/
public Builder setId(long value) {
id_ = value;
onChanged();
return this;
}
/**
* uint64 id = 1;
* @return This builder for chaining.
*/
public Builder clearId() {
id_ = 0L;
onChanged();
return this;
}
private boolean local_ ;
/**
* bool local = 2;
* @return The local.
*/
@java.lang.Override
public boolean getLocal() {
return local_;
}
/**
* bool local = 2;
* @param value The local to set.
* @return This builder for chaining.
*/
public Builder setLocal(boolean value) {
local_ = value;
onChanged();
return this;
}
/**
* bool local = 2;
* @return This builder for chaining.
*/
public Builder clearLocal() {
local_ = false;
onChanged();
return this;
}
private java.lang.Object from_ = "";
/**
* string from = 3;
* @return The from.
*/
public java.lang.String getFrom() {
java.lang.Object ref = from_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
from_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string from = 3;
* @return The bytes for from.
*/
public com.google.protobuf.ByteString
getFromBytes() {
java.lang.Object ref = from_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
from_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string from = 3;
* @param value The from to set.
* @return This builder for chaining.
*/
public Builder setFrom(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
from_ = value;
onChanged();
return this;
}
/**
* string from = 3;
* @return This builder for chaining.
*/
public Builder clearFrom() {
from_ = getDefaultInstance().getFrom();
onChanged();
return this;
}
/**
* string from = 3;
* @param value The bytes for from to set.
* @return This builder for chaining.
*/
public Builder setFromBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
from_ = value;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
* string name = 4;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string name = 4;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string name = 4;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
onChanged();
return this;
}
/**
* string name = 4;
* @return This builder for chaining.
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* string name = 4;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
onChanged();
return this;
}
private int refType_ = 0;
/**
* .cz.proto.ReferenceType ref_type = 5;
* @return The enum numeric value on the wire for refType.
*/
@java.lang.Override public int getRefTypeValue() {
return refType_;
}
/**
* .cz.proto.ReferenceType ref_type = 5;
* @param value The enum numeric value on the wire for refType to set.
* @return This builder for chaining.
*/
public Builder setRefTypeValue(int value) {
refType_ = value;
onChanged();
return this;
}
/**
* .cz.proto.ReferenceType ref_type = 5;
* @return The refType.
*/
@java.lang.Override
public cz.proto.Expression.ReferenceType getRefType() {
@SuppressWarnings("deprecation")
cz.proto.Expression.ReferenceType result = cz.proto.Expression.ReferenceType.valueOf(refType_);
return result == null ? cz.proto.Expression.ReferenceType.UNRECOGNIZED : result;
}
/**
* .cz.proto.ReferenceType ref_type = 5;
* @param value The refType to set.
* @return This builder for chaining.
*/
public Builder setRefType(cz.proto.Expression.ReferenceType value) {
if (value == null) {
throw new NullPointerException();
}
refType_ = value.getNumber();
onChanged();
return this;
}
/**
* .cz.proto.ReferenceType ref_type = 5;
* @return This builder for chaining.
*/
public Builder clearRefType() {
refType_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.Reference)
}
// @@protoc_insertion_point(class_scope:cz.proto.Reference)
private static final cz.proto.Expression.Reference DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.Expression.Reference();
}
public static cz.proto.Expression.Reference getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Reference parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Reference(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.Expression.Reference getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface UdfOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.Udf)
com.google.protobuf.MessageOrBuilder {
/**
* int64 instanceId = 1;
* @return The instanceId.
*/
long getInstanceId();
/**
* repeated string path = 2;
* @return A list containing the path.
*/
java.util.List
getPathList();
/**
* repeated string path = 2;
* @return The count of path.
*/
int getPathCount();
/**
* repeated string path = 2;
* @param index The index of the element to return.
* @return The path at the given index.
*/
java.lang.String getPath(int index);
/**
* repeated string path = 2;
* @param index The index of the value to return.
* @return The bytes of the path at the given index.
*/
com.google.protobuf.ByteString
getPathBytes(int index);
/**
* int64 creator = 3;
* @return The creator.
*/
long getCreator();
}
/**
* Protobuf type {@code cz.proto.Udf}
*/
public static final class Udf extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.Udf)
UdfOrBuilder {
private static final long serialVersionUID = 0L;
// Use Udf.newBuilder() to construct.
private Udf(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Udf() {
path_ = com.google.protobuf.LazyStringArrayList.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Udf();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Udf(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
instanceId_ = input.readInt64();
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
path_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000001;
}
path_.add(s);
break;
}
case 24: {
creator_ = input.readInt64();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
path_ = path_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.Expression.internal_static_cz_proto_Udf_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.Expression.internal_static_cz_proto_Udf_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.Expression.Udf.class, cz.proto.Expression.Udf.Builder.class);
}
public static final int INSTANCEID_FIELD_NUMBER = 1;
private long instanceId_;
/**
* int64 instanceId = 1;
* @return The instanceId.
*/
@java.lang.Override
public long getInstanceId() {
return instanceId_;
}
public static final int PATH_FIELD_NUMBER = 2;
private com.google.protobuf.LazyStringList path_;
/**
* repeated string path = 2;
* @return A list containing the path.
*/
public com.google.protobuf.ProtocolStringList
getPathList() {
return path_;
}
/**
* repeated string path = 2;
* @return The count of path.
*/
public int getPathCount() {
return path_.size();
}
/**
* repeated string path = 2;
* @param index The index of the element to return.
* @return The path at the given index.
*/
public java.lang.String getPath(int index) {
return path_.get(index);
}
/**
* repeated string path = 2;
* @param index The index of the value to return.
* @return The bytes of the path at the given index.
*/
public com.google.protobuf.ByteString
getPathBytes(int index) {
return path_.getByteString(index);
}
public static final int CREATOR_FIELD_NUMBER = 3;
private long creator_;
/**
* int64 creator = 3;
* @return The creator.
*/
@java.lang.Override
public long getCreator() {
return creator_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (instanceId_ != 0L) {
output.writeInt64(1, instanceId_);
}
for (int i = 0; i < path_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, path_.getRaw(i));
}
if (creator_ != 0L) {
output.writeInt64(3, creator_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (instanceId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, instanceId_);
}
{
int dataSize = 0;
for (int i = 0; i < path_.size(); i++) {
dataSize += computeStringSizeNoTag(path_.getRaw(i));
}
size += dataSize;
size += 1 * getPathList().size();
}
if (creator_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, creator_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.Expression.Udf)) {
return super.equals(obj);
}
cz.proto.Expression.Udf other = (cz.proto.Expression.Udf) obj;
if (getInstanceId()
!= other.getInstanceId()) return false;
if (!getPathList()
.equals(other.getPathList())) return false;
if (getCreator()
!= other.getCreator()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + INSTANCEID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getInstanceId());
if (getPathCount() > 0) {
hash = (37 * hash) + PATH_FIELD_NUMBER;
hash = (53 * hash) + getPathList().hashCode();
}
hash = (37 * hash) + CREATOR_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getCreator());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.Expression.Udf parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.Udf parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.Udf parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.Udf parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.Udf parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.Udf parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.Udf parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.Expression.Udf parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.Expression.Udf parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.Expression.Udf parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.Expression.Udf parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.Expression.Udf parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.Expression.Udf prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.Udf}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.Udf)
cz.proto.Expression.UdfOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.Expression.internal_static_cz_proto_Udf_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.Expression.internal_static_cz_proto_Udf_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.Expression.Udf.class, cz.proto.Expression.Udf.Builder.class);
}
// Construct using cz.proto.Expression.Udf.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
instanceId_ = 0L;
path_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
creator_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.Expression.internal_static_cz_proto_Udf_descriptor;
}
@java.lang.Override
public cz.proto.Expression.Udf getDefaultInstanceForType() {
return cz.proto.Expression.Udf.getDefaultInstance();
}
@java.lang.Override
public cz.proto.Expression.Udf build() {
cz.proto.Expression.Udf result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.Expression.Udf buildPartial() {
cz.proto.Expression.Udf result = new cz.proto.Expression.Udf(this);
int from_bitField0_ = bitField0_;
result.instanceId_ = instanceId_;
if (((bitField0_ & 0x00000001) != 0)) {
path_ = path_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.path_ = path_;
result.creator_ = creator_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.Expression.Udf) {
return mergeFrom((cz.proto.Expression.Udf)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.Expression.Udf other) {
if (other == cz.proto.Expression.Udf.getDefaultInstance()) return this;
if (other.getInstanceId() != 0L) {
setInstanceId(other.getInstanceId());
}
if (!other.path_.isEmpty()) {
if (path_.isEmpty()) {
path_ = other.path_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensurePathIsMutable();
path_.addAll(other.path_);
}
onChanged();
}
if (other.getCreator() != 0L) {
setCreator(other.getCreator());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.Expression.Udf parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.Expression.Udf) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private long instanceId_ ;
/**
* int64 instanceId = 1;
* @return The instanceId.
*/
@java.lang.Override
public long getInstanceId() {
return instanceId_;
}
/**
* int64 instanceId = 1;
* @param value The instanceId to set.
* @return This builder for chaining.
*/
public Builder setInstanceId(long value) {
instanceId_ = value;
onChanged();
return this;
}
/**
* int64 instanceId = 1;
* @return This builder for chaining.
*/
public Builder clearInstanceId() {
instanceId_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList path_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensurePathIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
path_ = new com.google.protobuf.LazyStringArrayList(path_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated string path = 2;
* @return A list containing the path.
*/
public com.google.protobuf.ProtocolStringList
getPathList() {
return path_.getUnmodifiableView();
}
/**
* repeated string path = 2;
* @return The count of path.
*/
public int getPathCount() {
return path_.size();
}
/**
* repeated string path = 2;
* @param index The index of the element to return.
* @return The path at the given index.
*/
public java.lang.String getPath(int index) {
return path_.get(index);
}
/**
* repeated string path = 2;
* @param index The index of the value to return.
* @return The bytes of the path at the given index.
*/
public com.google.protobuf.ByteString
getPathBytes(int index) {
return path_.getByteString(index);
}
/**
* repeated string path = 2;
* @param index The index to set the value at.
* @param value The path to set.
* @return This builder for chaining.
*/
public Builder setPath(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensurePathIsMutable();
path_.set(index, value);
onChanged();
return this;
}
/**
* repeated string path = 2;
* @param value The path to add.
* @return This builder for chaining.
*/
public Builder addPath(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensurePathIsMutable();
path_.add(value);
onChanged();
return this;
}
/**
* repeated string path = 2;
* @param values The path to add.
* @return This builder for chaining.
*/
public Builder addAllPath(
java.lang.Iterable values) {
ensurePathIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, path_);
onChanged();
return this;
}
/**
* repeated string path = 2;
* @return This builder for chaining.
*/
public Builder clearPath() {
path_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* repeated string path = 2;
* @param value The bytes of the path to add.
* @return This builder for chaining.
*/
public Builder addPathBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensurePathIsMutable();
path_.add(value);
onChanged();
return this;
}
private long creator_ ;
/**
* int64 creator = 3;
* @return The creator.
*/
@java.lang.Override
public long getCreator() {
return creator_;
}
/**
* int64 creator = 3;
* @param value The creator to set.
* @return This builder for chaining.
*/
public Builder setCreator(long value) {
creator_ = value;
onChanged();
return this;
}
/**
* int64 creator = 3;
* @return This builder for chaining.
*/
public Builder clearCreator() {
creator_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.Udf)
}
// @@protoc_insertion_point(class_scope:cz.proto.Udf)
private static final cz.proto.Expression.Udf DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.Expression.Udf();
}
public static cz.proto.Expression.Udf getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Udf parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Udf(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.Expression.Udf getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ScalarFunctionOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ScalarFunction)
com.google.protobuf.MessageOrBuilder {
/**
* string from = 1;
* @return The from.
*/
java.lang.String getFrom();
/**
* string from = 1;
* @return The bytes for from.
*/
com.google.protobuf.ByteString
getFromBytes();
/**
* string name = 2;
* @return The name.
*/
java.lang.String getName();
/**
* string name = 2;
* @return The bytes for name.
*/
com.google.protobuf.ByteString
getNameBytes();
/**
* bool builtIn = 3;
* @return The builtIn.
*/
boolean getBuiltIn();
/**
* repeated .cz.proto.ScalarExpression arguments = 4;
*/
java.util.List
getArgumentsList();
/**
* repeated .cz.proto.ScalarExpression arguments = 4;
*/
cz.proto.Expression.ScalarExpression getArguments(int index);
/**
* repeated .cz.proto.ScalarExpression arguments = 4;
*/
int getArgumentsCount();
/**
* repeated .cz.proto.ScalarExpression arguments = 4;
*/
java.util.List extends cz.proto.Expression.ScalarExpressionOrBuilder>
getArgumentsOrBuilderList();
/**
* repeated .cz.proto.ScalarExpression arguments = 4;
*/
cz.proto.Expression.ScalarExpressionOrBuilder getArgumentsOrBuilder(
int index);
/**
* .cz.proto.Properties properties = 5;
* @return Whether the properties field is set.
*/
boolean hasProperties();
/**
* .cz.proto.Properties properties = 5;
* @return The properties.
*/
cz.proto.PropertyOuterClass.Properties getProperties();
/**
* .cz.proto.Properties properties = 5;
*/
cz.proto.PropertyOuterClass.PropertiesOrBuilder getPropertiesOrBuilder();
/**
* string execDesc = 6;
* @return The execDesc.
*/
java.lang.String getExecDesc();
/**
* string execDesc = 6;
* @return The bytes for execDesc.
*/
com.google.protobuf.ByteString
getExecDescBytes();
/**
* .cz.proto.Properties functionProperties = 7;
* @return Whether the functionProperties field is set.
*/
boolean hasFunctionProperties();
/**
* .cz.proto.Properties functionProperties = 7;
* @return The functionProperties.
*/
cz.proto.PropertyOuterClass.Properties getFunctionProperties();
/**
* .cz.proto.Properties functionProperties = 7;
*/
cz.proto.PropertyOuterClass.PropertiesOrBuilder getFunctionPropertiesOrBuilder();
/**
* .cz.proto.Udf udf = 10;
* @return Whether the udf field is set.
*/
boolean hasUdf();
/**
* .cz.proto.Udf udf = 10;
* @return The udf.
*/
cz.proto.Expression.Udf getUdf();
/**
* .cz.proto.Udf udf = 10;
*/
cz.proto.Expression.UdfOrBuilder getUdfOrBuilder();
public cz.proto.Expression.ScalarFunction.DerivedCase getDerivedCase();
}
/**
* Protobuf type {@code cz.proto.ScalarFunction}
*/
public static final class ScalarFunction extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ScalarFunction)
ScalarFunctionOrBuilder {
private static final long serialVersionUID = 0L;
// Use ScalarFunction.newBuilder() to construct.
private ScalarFunction(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ScalarFunction() {
from_ = "";
name_ = "";
arguments_ = java.util.Collections.emptyList();
execDesc_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ScalarFunction();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ScalarFunction(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
from_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
name_ = s;
break;
}
case 24: {
builtIn_ = input.readBool();
break;
}
case 34: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
arguments_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
arguments_.add(
input.readMessage(cz.proto.Expression.ScalarExpression.parser(), extensionRegistry));
break;
}
case 42: {
cz.proto.PropertyOuterClass.Properties.Builder subBuilder = null;
if (properties_ != null) {
subBuilder = properties_.toBuilder();
}
properties_ = input.readMessage(cz.proto.PropertyOuterClass.Properties.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(properties_);
properties_ = subBuilder.buildPartial();
}
break;
}
case 50: {
java.lang.String s = input.readStringRequireUtf8();
execDesc_ = s;
break;
}
case 58: {
cz.proto.PropertyOuterClass.Properties.Builder subBuilder = null;
if (functionProperties_ != null) {
subBuilder = functionProperties_.toBuilder();
}
functionProperties_ = input.readMessage(cz.proto.PropertyOuterClass.Properties.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(functionProperties_);
functionProperties_ = subBuilder.buildPartial();
}
break;
}
case 82: {
cz.proto.Expression.Udf.Builder subBuilder = null;
if (derivedCase_ == 10) {
subBuilder = ((cz.proto.Expression.Udf) derived_).toBuilder();
}
derived_ =
input.readMessage(cz.proto.Expression.Udf.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((cz.proto.Expression.Udf) derived_);
derived_ = subBuilder.buildPartial();
}
derivedCase_ = 10;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
arguments_ = java.util.Collections.unmodifiableList(arguments_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.Expression.internal_static_cz_proto_ScalarFunction_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.Expression.internal_static_cz_proto_ScalarFunction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.Expression.ScalarFunction.class, cz.proto.Expression.ScalarFunction.Builder.class);
}
private int derivedCase_ = 0;
private java.lang.Object derived_;
public enum DerivedCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
UDF(10),
DERIVED_NOT_SET(0);
private final int value;
private DerivedCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static DerivedCase valueOf(int value) {
return forNumber(value);
}
public static DerivedCase forNumber(int value) {
switch (value) {
case 10: return UDF;
case 0: return DERIVED_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public DerivedCase
getDerivedCase() {
return DerivedCase.forNumber(
derivedCase_);
}
public static final int FROM_FIELD_NUMBER = 1;
private volatile java.lang.Object from_;
/**
* string from = 1;
* @return The from.
*/
@java.lang.Override
public java.lang.String getFrom() {
java.lang.Object ref = from_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
from_ = s;
return s;
}
}
/**
* string from = 1;
* @return The bytes for from.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getFromBytes() {
java.lang.Object ref = from_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
from_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NAME_FIELD_NUMBER = 2;
private volatile java.lang.Object name_;
/**
* string name = 2;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
* string name = 2;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int BUILTIN_FIELD_NUMBER = 3;
private boolean builtIn_;
/**
* bool builtIn = 3;
* @return The builtIn.
*/
@java.lang.Override
public boolean getBuiltIn() {
return builtIn_;
}
public static final int ARGUMENTS_FIELD_NUMBER = 4;
private java.util.List arguments_;
/**
* repeated .cz.proto.ScalarExpression arguments = 4;
*/
@java.lang.Override
public java.util.List getArgumentsList() {
return arguments_;
}
/**
* repeated .cz.proto.ScalarExpression arguments = 4;
*/
@java.lang.Override
public java.util.List extends cz.proto.Expression.ScalarExpressionOrBuilder>
getArgumentsOrBuilderList() {
return arguments_;
}
/**
* repeated .cz.proto.ScalarExpression arguments = 4;
*/
@java.lang.Override
public int getArgumentsCount() {
return arguments_.size();
}
/**
* repeated .cz.proto.ScalarExpression arguments = 4;
*/
@java.lang.Override
public cz.proto.Expression.ScalarExpression getArguments(int index) {
return arguments_.get(index);
}
/**
* repeated .cz.proto.ScalarExpression arguments = 4;
*/
@java.lang.Override
public cz.proto.Expression.ScalarExpressionOrBuilder getArgumentsOrBuilder(
int index) {
return arguments_.get(index);
}
public static final int PROPERTIES_FIELD_NUMBER = 5;
private cz.proto.PropertyOuterClass.Properties properties_;
/**
* .cz.proto.Properties properties = 5;
* @return Whether the properties field is set.
*/
@java.lang.Override
public boolean hasProperties() {
return properties_ != null;
}
/**
* .cz.proto.Properties properties = 5;
* @return The properties.
*/
@java.lang.Override
public cz.proto.PropertyOuterClass.Properties getProperties() {
return properties_ == null ? cz.proto.PropertyOuterClass.Properties.getDefaultInstance() : properties_;
}
/**
* .cz.proto.Properties properties = 5;
*/
@java.lang.Override
public cz.proto.PropertyOuterClass.PropertiesOrBuilder getPropertiesOrBuilder() {
return getProperties();
}
public static final int EXECDESC_FIELD_NUMBER = 6;
private volatile java.lang.Object execDesc_;
/**
* string execDesc = 6;
* @return The execDesc.
*/
@java.lang.Override
public java.lang.String getExecDesc() {
java.lang.Object ref = execDesc_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
execDesc_ = s;
return s;
}
}
/**
* string execDesc = 6;
* @return The bytes for execDesc.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getExecDescBytes() {
java.lang.Object ref = execDesc_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
execDesc_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int FUNCTIONPROPERTIES_FIELD_NUMBER = 7;
private cz.proto.PropertyOuterClass.Properties functionProperties_;
/**
* .cz.proto.Properties functionProperties = 7;
* @return Whether the functionProperties field is set.
*/
@java.lang.Override
public boolean hasFunctionProperties() {
return functionProperties_ != null;
}
/**
* .cz.proto.Properties functionProperties = 7;
* @return The functionProperties.
*/
@java.lang.Override
public cz.proto.PropertyOuterClass.Properties getFunctionProperties() {
return functionProperties_ == null ? cz.proto.PropertyOuterClass.Properties.getDefaultInstance() : functionProperties_;
}
/**
* .cz.proto.Properties functionProperties = 7;
*/
@java.lang.Override
public cz.proto.PropertyOuterClass.PropertiesOrBuilder getFunctionPropertiesOrBuilder() {
return getFunctionProperties();
}
public static final int UDF_FIELD_NUMBER = 10;
/**
* .cz.proto.Udf udf = 10;
* @return Whether the udf field is set.
*/
@java.lang.Override
public boolean hasUdf() {
return derivedCase_ == 10;
}
/**
* .cz.proto.Udf udf = 10;
* @return The udf.
*/
@java.lang.Override
public cz.proto.Expression.Udf getUdf() {
if (derivedCase_ == 10) {
return (cz.proto.Expression.Udf) derived_;
}
return cz.proto.Expression.Udf.getDefaultInstance();
}
/**
* .cz.proto.Udf udf = 10;
*/
@java.lang.Override
public cz.proto.Expression.UdfOrBuilder getUdfOrBuilder() {
if (derivedCase_ == 10) {
return (cz.proto.Expression.Udf) derived_;
}
return cz.proto.Expression.Udf.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(from_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, from_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(name_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, name_);
}
if (builtIn_ != false) {
output.writeBool(3, builtIn_);
}
for (int i = 0; i < arguments_.size(); i++) {
output.writeMessage(4, arguments_.get(i));
}
if (properties_ != null) {
output.writeMessage(5, getProperties());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(execDesc_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, execDesc_);
}
if (functionProperties_ != null) {
output.writeMessage(7, getFunctionProperties());
}
if (derivedCase_ == 10) {
output.writeMessage(10, (cz.proto.Expression.Udf) derived_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(from_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, from_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(name_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, name_);
}
if (builtIn_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(3, builtIn_);
}
for (int i = 0; i < arguments_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, arguments_.get(i));
}
if (properties_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getProperties());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(execDesc_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(6, execDesc_);
}
if (functionProperties_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, getFunctionProperties());
}
if (derivedCase_ == 10) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, (cz.proto.Expression.Udf) derived_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.Expression.ScalarFunction)) {
return super.equals(obj);
}
cz.proto.Expression.ScalarFunction other = (cz.proto.Expression.ScalarFunction) obj;
if (!getFrom()
.equals(other.getFrom())) return false;
if (!getName()
.equals(other.getName())) return false;
if (getBuiltIn()
!= other.getBuiltIn()) return false;
if (!getArgumentsList()
.equals(other.getArgumentsList())) return false;
if (hasProperties() != other.hasProperties()) return false;
if (hasProperties()) {
if (!getProperties()
.equals(other.getProperties())) return false;
}
if (!getExecDesc()
.equals(other.getExecDesc())) return false;
if (hasFunctionProperties() != other.hasFunctionProperties()) return false;
if (hasFunctionProperties()) {
if (!getFunctionProperties()
.equals(other.getFunctionProperties())) return false;
}
if (!getDerivedCase().equals(other.getDerivedCase())) return false;
switch (derivedCase_) {
case 10:
if (!getUdf()
.equals(other.getUdf())) return false;
break;
case 0:
default:
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + FROM_FIELD_NUMBER;
hash = (53 * hash) + getFrom().hashCode();
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
hash = (37 * hash) + BUILTIN_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getBuiltIn());
if (getArgumentsCount() > 0) {
hash = (37 * hash) + ARGUMENTS_FIELD_NUMBER;
hash = (53 * hash) + getArgumentsList().hashCode();
}
if (hasProperties()) {
hash = (37 * hash) + PROPERTIES_FIELD_NUMBER;
hash = (53 * hash) + getProperties().hashCode();
}
hash = (37 * hash) + EXECDESC_FIELD_NUMBER;
hash = (53 * hash) + getExecDesc().hashCode();
if (hasFunctionProperties()) {
hash = (37 * hash) + FUNCTIONPROPERTIES_FIELD_NUMBER;
hash = (53 * hash) + getFunctionProperties().hashCode();
}
switch (derivedCase_) {
case 10:
hash = (37 * hash) + UDF_FIELD_NUMBER;
hash = (53 * hash) + getUdf().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.Expression.ScalarFunction parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.ScalarFunction parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.ScalarFunction parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.ScalarFunction parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.ScalarFunction parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.ScalarFunction parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.ScalarFunction parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.Expression.ScalarFunction parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.Expression.ScalarFunction parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.Expression.ScalarFunction parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.Expression.ScalarFunction parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.Expression.ScalarFunction parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.Expression.ScalarFunction prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ScalarFunction}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ScalarFunction)
cz.proto.Expression.ScalarFunctionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.Expression.internal_static_cz_proto_ScalarFunction_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.Expression.internal_static_cz_proto_ScalarFunction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.Expression.ScalarFunction.class, cz.proto.Expression.ScalarFunction.Builder.class);
}
// Construct using cz.proto.Expression.ScalarFunction.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getArgumentsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
from_ = "";
name_ = "";
builtIn_ = false;
if (argumentsBuilder_ == null) {
arguments_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
argumentsBuilder_.clear();
}
if (propertiesBuilder_ == null) {
properties_ = null;
} else {
properties_ = null;
propertiesBuilder_ = null;
}
execDesc_ = "";
if (functionPropertiesBuilder_ == null) {
functionProperties_ = null;
} else {
functionProperties_ = null;
functionPropertiesBuilder_ = null;
}
derivedCase_ = 0;
derived_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.Expression.internal_static_cz_proto_ScalarFunction_descriptor;
}
@java.lang.Override
public cz.proto.Expression.ScalarFunction getDefaultInstanceForType() {
return cz.proto.Expression.ScalarFunction.getDefaultInstance();
}
@java.lang.Override
public cz.proto.Expression.ScalarFunction build() {
cz.proto.Expression.ScalarFunction result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.Expression.ScalarFunction buildPartial() {
cz.proto.Expression.ScalarFunction result = new cz.proto.Expression.ScalarFunction(this);
int from_bitField0_ = bitField0_;
result.from_ = from_;
result.name_ = name_;
result.builtIn_ = builtIn_;
if (argumentsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
arguments_ = java.util.Collections.unmodifiableList(arguments_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.arguments_ = arguments_;
} else {
result.arguments_ = argumentsBuilder_.build();
}
if (propertiesBuilder_ == null) {
result.properties_ = properties_;
} else {
result.properties_ = propertiesBuilder_.build();
}
result.execDesc_ = execDesc_;
if (functionPropertiesBuilder_ == null) {
result.functionProperties_ = functionProperties_;
} else {
result.functionProperties_ = functionPropertiesBuilder_.build();
}
if (derivedCase_ == 10) {
if (udfBuilder_ == null) {
result.derived_ = derived_;
} else {
result.derived_ = udfBuilder_.build();
}
}
result.derivedCase_ = derivedCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.Expression.ScalarFunction) {
return mergeFrom((cz.proto.Expression.ScalarFunction)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.Expression.ScalarFunction other) {
if (other == cz.proto.Expression.ScalarFunction.getDefaultInstance()) return this;
if (!other.getFrom().isEmpty()) {
from_ = other.from_;
onChanged();
}
if (!other.getName().isEmpty()) {
name_ = other.name_;
onChanged();
}
if (other.getBuiltIn() != false) {
setBuiltIn(other.getBuiltIn());
}
if (argumentsBuilder_ == null) {
if (!other.arguments_.isEmpty()) {
if (arguments_.isEmpty()) {
arguments_ = other.arguments_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureArgumentsIsMutable();
arguments_.addAll(other.arguments_);
}
onChanged();
}
} else {
if (!other.arguments_.isEmpty()) {
if (argumentsBuilder_.isEmpty()) {
argumentsBuilder_.dispose();
argumentsBuilder_ = null;
arguments_ = other.arguments_;
bitField0_ = (bitField0_ & ~0x00000001);
argumentsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getArgumentsFieldBuilder() : null;
} else {
argumentsBuilder_.addAllMessages(other.arguments_);
}
}
}
if (other.hasProperties()) {
mergeProperties(other.getProperties());
}
if (!other.getExecDesc().isEmpty()) {
execDesc_ = other.execDesc_;
onChanged();
}
if (other.hasFunctionProperties()) {
mergeFunctionProperties(other.getFunctionProperties());
}
switch (other.getDerivedCase()) {
case UDF: {
mergeUdf(other.getUdf());
break;
}
case DERIVED_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.Expression.ScalarFunction parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.Expression.ScalarFunction) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int derivedCase_ = 0;
private java.lang.Object derived_;
public DerivedCase
getDerivedCase() {
return DerivedCase.forNumber(
derivedCase_);
}
public Builder clearDerived() {
derivedCase_ = 0;
derived_ = null;
onChanged();
return this;
}
private int bitField0_;
private java.lang.Object from_ = "";
/**
* string from = 1;
* @return The from.
*/
public java.lang.String getFrom() {
java.lang.Object ref = from_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
from_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string from = 1;
* @return The bytes for from.
*/
public com.google.protobuf.ByteString
getFromBytes() {
java.lang.Object ref = from_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
from_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string from = 1;
* @param value The from to set.
* @return This builder for chaining.
*/
public Builder setFrom(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
from_ = value;
onChanged();
return this;
}
/**
* string from = 1;
* @return This builder for chaining.
*/
public Builder clearFrom() {
from_ = getDefaultInstance().getFrom();
onChanged();
return this;
}
/**
* string from = 1;
* @param value The bytes for from to set.
* @return This builder for chaining.
*/
public Builder setFromBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
from_ = value;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
* string name = 2;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string name = 2;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string name = 2;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
onChanged();
return this;
}
/**
* string name = 2;
* @return This builder for chaining.
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* string name = 2;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
onChanged();
return this;
}
private boolean builtIn_ ;
/**
* bool builtIn = 3;
* @return The builtIn.
*/
@java.lang.Override
public boolean getBuiltIn() {
return builtIn_;
}
/**
* bool builtIn = 3;
* @param value The builtIn to set.
* @return This builder for chaining.
*/
public Builder setBuiltIn(boolean value) {
builtIn_ = value;
onChanged();
return this;
}
/**
* bool builtIn = 3;
* @return This builder for chaining.
*/
public Builder clearBuiltIn() {
builtIn_ = false;
onChanged();
return this;
}
private java.util.List arguments_ =
java.util.Collections.emptyList();
private void ensureArgumentsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
arguments_ = new java.util.ArrayList(arguments_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.Expression.ScalarExpression, cz.proto.Expression.ScalarExpression.Builder, cz.proto.Expression.ScalarExpressionOrBuilder> argumentsBuilder_;
/**
* repeated .cz.proto.ScalarExpression arguments = 4;
*/
public java.util.List getArgumentsList() {
if (argumentsBuilder_ == null) {
return java.util.Collections.unmodifiableList(arguments_);
} else {
return argumentsBuilder_.getMessageList();
}
}
/**
* repeated .cz.proto.ScalarExpression arguments = 4;
*/
public int getArgumentsCount() {
if (argumentsBuilder_ == null) {
return arguments_.size();
} else {
return argumentsBuilder_.getCount();
}
}
/**
* repeated .cz.proto.ScalarExpression arguments = 4;
*/
public cz.proto.Expression.ScalarExpression getArguments(int index) {
if (argumentsBuilder_ == null) {
return arguments_.get(index);
} else {
return argumentsBuilder_.getMessage(index);
}
}
/**
* repeated .cz.proto.ScalarExpression arguments = 4;
*/
public Builder setArguments(
int index, cz.proto.Expression.ScalarExpression value) {
if (argumentsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureArgumentsIsMutable();
arguments_.set(index, value);
onChanged();
} else {
argumentsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .cz.proto.ScalarExpression arguments = 4;
*/
public Builder setArguments(
int index, cz.proto.Expression.ScalarExpression.Builder builderForValue) {
if (argumentsBuilder_ == null) {
ensureArgumentsIsMutable();
arguments_.set(index, builderForValue.build());
onChanged();
} else {
argumentsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.ScalarExpression arguments = 4;
*/
public Builder addArguments(cz.proto.Expression.ScalarExpression value) {
if (argumentsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureArgumentsIsMutable();
arguments_.add(value);
onChanged();
} else {
argumentsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .cz.proto.ScalarExpression arguments = 4;
*/
public Builder addArguments(
int index, cz.proto.Expression.ScalarExpression value) {
if (argumentsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureArgumentsIsMutable();
arguments_.add(index, value);
onChanged();
} else {
argumentsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .cz.proto.ScalarExpression arguments = 4;
*/
public Builder addArguments(
cz.proto.Expression.ScalarExpression.Builder builderForValue) {
if (argumentsBuilder_ == null) {
ensureArgumentsIsMutable();
arguments_.add(builderForValue.build());
onChanged();
} else {
argumentsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.ScalarExpression arguments = 4;
*/
public Builder addArguments(
int index, cz.proto.Expression.ScalarExpression.Builder builderForValue) {
if (argumentsBuilder_ == null) {
ensureArgumentsIsMutable();
arguments_.add(index, builderForValue.build());
onChanged();
} else {
argumentsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.ScalarExpression arguments = 4;
*/
public Builder addAllArguments(
java.lang.Iterable extends cz.proto.Expression.ScalarExpression> values) {
if (argumentsBuilder_ == null) {
ensureArgumentsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, arguments_);
onChanged();
} else {
argumentsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .cz.proto.ScalarExpression arguments = 4;
*/
public Builder clearArguments() {
if (argumentsBuilder_ == null) {
arguments_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
argumentsBuilder_.clear();
}
return this;
}
/**
* repeated .cz.proto.ScalarExpression arguments = 4;
*/
public Builder removeArguments(int index) {
if (argumentsBuilder_ == null) {
ensureArgumentsIsMutable();
arguments_.remove(index);
onChanged();
} else {
argumentsBuilder_.remove(index);
}
return this;
}
/**
* repeated .cz.proto.ScalarExpression arguments = 4;
*/
public cz.proto.Expression.ScalarExpression.Builder getArgumentsBuilder(
int index) {
return getArgumentsFieldBuilder().getBuilder(index);
}
/**
* repeated .cz.proto.ScalarExpression arguments = 4;
*/
public cz.proto.Expression.ScalarExpressionOrBuilder getArgumentsOrBuilder(
int index) {
if (argumentsBuilder_ == null) {
return arguments_.get(index); } else {
return argumentsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .cz.proto.ScalarExpression arguments = 4;
*/
public java.util.List extends cz.proto.Expression.ScalarExpressionOrBuilder>
getArgumentsOrBuilderList() {
if (argumentsBuilder_ != null) {
return argumentsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(arguments_);
}
}
/**
* repeated .cz.proto.ScalarExpression arguments = 4;
*/
public cz.proto.Expression.ScalarExpression.Builder addArgumentsBuilder() {
return getArgumentsFieldBuilder().addBuilder(
cz.proto.Expression.ScalarExpression.getDefaultInstance());
}
/**
* repeated .cz.proto.ScalarExpression arguments = 4;
*/
public cz.proto.Expression.ScalarExpression.Builder addArgumentsBuilder(
int index) {
return getArgumentsFieldBuilder().addBuilder(
index, cz.proto.Expression.ScalarExpression.getDefaultInstance());
}
/**
* repeated .cz.proto.ScalarExpression arguments = 4;
*/
public java.util.List
getArgumentsBuilderList() {
return getArgumentsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.Expression.ScalarExpression, cz.proto.Expression.ScalarExpression.Builder, cz.proto.Expression.ScalarExpressionOrBuilder>
getArgumentsFieldBuilder() {
if (argumentsBuilder_ == null) {
argumentsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.Expression.ScalarExpression, cz.proto.Expression.ScalarExpression.Builder, cz.proto.Expression.ScalarExpressionOrBuilder>(
arguments_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
arguments_ = null;
}
return argumentsBuilder_;
}
private cz.proto.PropertyOuterClass.Properties properties_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.PropertyOuterClass.Properties, cz.proto.PropertyOuterClass.Properties.Builder, cz.proto.PropertyOuterClass.PropertiesOrBuilder> propertiesBuilder_;
/**
* .cz.proto.Properties properties = 5;
* @return Whether the properties field is set.
*/
public boolean hasProperties() {
return propertiesBuilder_ != null || properties_ != null;
}
/**
* .cz.proto.Properties properties = 5;
* @return The properties.
*/
public cz.proto.PropertyOuterClass.Properties getProperties() {
if (propertiesBuilder_ == null) {
return properties_ == null ? cz.proto.PropertyOuterClass.Properties.getDefaultInstance() : properties_;
} else {
return propertiesBuilder_.getMessage();
}
}
/**
* .cz.proto.Properties properties = 5;
*/
public Builder setProperties(cz.proto.PropertyOuterClass.Properties value) {
if (propertiesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
properties_ = value;
onChanged();
} else {
propertiesBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.Properties properties = 5;
*/
public Builder setProperties(
cz.proto.PropertyOuterClass.Properties.Builder builderForValue) {
if (propertiesBuilder_ == null) {
properties_ = builderForValue.build();
onChanged();
} else {
propertiesBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.Properties properties = 5;
*/
public Builder mergeProperties(cz.proto.PropertyOuterClass.Properties value) {
if (propertiesBuilder_ == null) {
if (properties_ != null) {
properties_ =
cz.proto.PropertyOuterClass.Properties.newBuilder(properties_).mergeFrom(value).buildPartial();
} else {
properties_ = value;
}
onChanged();
} else {
propertiesBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.Properties properties = 5;
*/
public Builder clearProperties() {
if (propertiesBuilder_ == null) {
properties_ = null;
onChanged();
} else {
properties_ = null;
propertiesBuilder_ = null;
}
return this;
}
/**
* .cz.proto.Properties properties = 5;
*/
public cz.proto.PropertyOuterClass.Properties.Builder getPropertiesBuilder() {
onChanged();
return getPropertiesFieldBuilder().getBuilder();
}
/**
* .cz.proto.Properties properties = 5;
*/
public cz.proto.PropertyOuterClass.PropertiesOrBuilder getPropertiesOrBuilder() {
if (propertiesBuilder_ != null) {
return propertiesBuilder_.getMessageOrBuilder();
} else {
return properties_ == null ?
cz.proto.PropertyOuterClass.Properties.getDefaultInstance() : properties_;
}
}
/**
* .cz.proto.Properties properties = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.PropertyOuterClass.Properties, cz.proto.PropertyOuterClass.Properties.Builder, cz.proto.PropertyOuterClass.PropertiesOrBuilder>
getPropertiesFieldBuilder() {
if (propertiesBuilder_ == null) {
propertiesBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.PropertyOuterClass.Properties, cz.proto.PropertyOuterClass.Properties.Builder, cz.proto.PropertyOuterClass.PropertiesOrBuilder>(
getProperties(),
getParentForChildren(),
isClean());
properties_ = null;
}
return propertiesBuilder_;
}
private java.lang.Object execDesc_ = "";
/**
* string execDesc = 6;
* @return The execDesc.
*/
public java.lang.String getExecDesc() {
java.lang.Object ref = execDesc_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
execDesc_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string execDesc = 6;
* @return The bytes for execDesc.
*/
public com.google.protobuf.ByteString
getExecDescBytes() {
java.lang.Object ref = execDesc_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
execDesc_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string execDesc = 6;
* @param value The execDesc to set.
* @return This builder for chaining.
*/
public Builder setExecDesc(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
execDesc_ = value;
onChanged();
return this;
}
/**
* string execDesc = 6;
* @return This builder for chaining.
*/
public Builder clearExecDesc() {
execDesc_ = getDefaultInstance().getExecDesc();
onChanged();
return this;
}
/**
* string execDesc = 6;
* @param value The bytes for execDesc to set.
* @return This builder for chaining.
*/
public Builder setExecDescBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
execDesc_ = value;
onChanged();
return this;
}
private cz.proto.PropertyOuterClass.Properties functionProperties_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.PropertyOuterClass.Properties, cz.proto.PropertyOuterClass.Properties.Builder, cz.proto.PropertyOuterClass.PropertiesOrBuilder> functionPropertiesBuilder_;
/**
* .cz.proto.Properties functionProperties = 7;
* @return Whether the functionProperties field is set.
*/
public boolean hasFunctionProperties() {
return functionPropertiesBuilder_ != null || functionProperties_ != null;
}
/**
* .cz.proto.Properties functionProperties = 7;
* @return The functionProperties.
*/
public cz.proto.PropertyOuterClass.Properties getFunctionProperties() {
if (functionPropertiesBuilder_ == null) {
return functionProperties_ == null ? cz.proto.PropertyOuterClass.Properties.getDefaultInstance() : functionProperties_;
} else {
return functionPropertiesBuilder_.getMessage();
}
}
/**
* .cz.proto.Properties functionProperties = 7;
*/
public Builder setFunctionProperties(cz.proto.PropertyOuterClass.Properties value) {
if (functionPropertiesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
functionProperties_ = value;
onChanged();
} else {
functionPropertiesBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.Properties functionProperties = 7;
*/
public Builder setFunctionProperties(
cz.proto.PropertyOuterClass.Properties.Builder builderForValue) {
if (functionPropertiesBuilder_ == null) {
functionProperties_ = builderForValue.build();
onChanged();
} else {
functionPropertiesBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.Properties functionProperties = 7;
*/
public Builder mergeFunctionProperties(cz.proto.PropertyOuterClass.Properties value) {
if (functionPropertiesBuilder_ == null) {
if (functionProperties_ != null) {
functionProperties_ =
cz.proto.PropertyOuterClass.Properties.newBuilder(functionProperties_).mergeFrom(value).buildPartial();
} else {
functionProperties_ = value;
}
onChanged();
} else {
functionPropertiesBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.Properties functionProperties = 7;
*/
public Builder clearFunctionProperties() {
if (functionPropertiesBuilder_ == null) {
functionProperties_ = null;
onChanged();
} else {
functionProperties_ = null;
functionPropertiesBuilder_ = null;
}
return this;
}
/**
* .cz.proto.Properties functionProperties = 7;
*/
public cz.proto.PropertyOuterClass.Properties.Builder getFunctionPropertiesBuilder() {
onChanged();
return getFunctionPropertiesFieldBuilder().getBuilder();
}
/**
* .cz.proto.Properties functionProperties = 7;
*/
public cz.proto.PropertyOuterClass.PropertiesOrBuilder getFunctionPropertiesOrBuilder() {
if (functionPropertiesBuilder_ != null) {
return functionPropertiesBuilder_.getMessageOrBuilder();
} else {
return functionProperties_ == null ?
cz.proto.PropertyOuterClass.Properties.getDefaultInstance() : functionProperties_;
}
}
/**
* .cz.proto.Properties functionProperties = 7;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.PropertyOuterClass.Properties, cz.proto.PropertyOuterClass.Properties.Builder, cz.proto.PropertyOuterClass.PropertiesOrBuilder>
getFunctionPropertiesFieldBuilder() {
if (functionPropertiesBuilder_ == null) {
functionPropertiesBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.PropertyOuterClass.Properties, cz.proto.PropertyOuterClass.Properties.Builder, cz.proto.PropertyOuterClass.PropertiesOrBuilder>(
getFunctionProperties(),
getParentForChildren(),
isClean());
functionProperties_ = null;
}
return functionPropertiesBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.Udf, cz.proto.Expression.Udf.Builder, cz.proto.Expression.UdfOrBuilder> udfBuilder_;
/**
* .cz.proto.Udf udf = 10;
* @return Whether the udf field is set.
*/
@java.lang.Override
public boolean hasUdf() {
return derivedCase_ == 10;
}
/**
* .cz.proto.Udf udf = 10;
* @return The udf.
*/
@java.lang.Override
public cz.proto.Expression.Udf getUdf() {
if (udfBuilder_ == null) {
if (derivedCase_ == 10) {
return (cz.proto.Expression.Udf) derived_;
}
return cz.proto.Expression.Udf.getDefaultInstance();
} else {
if (derivedCase_ == 10) {
return udfBuilder_.getMessage();
}
return cz.proto.Expression.Udf.getDefaultInstance();
}
}
/**
* .cz.proto.Udf udf = 10;
*/
public Builder setUdf(cz.proto.Expression.Udf value) {
if (udfBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
derived_ = value;
onChanged();
} else {
udfBuilder_.setMessage(value);
}
derivedCase_ = 10;
return this;
}
/**
* .cz.proto.Udf udf = 10;
*/
public Builder setUdf(
cz.proto.Expression.Udf.Builder builderForValue) {
if (udfBuilder_ == null) {
derived_ = builderForValue.build();
onChanged();
} else {
udfBuilder_.setMessage(builderForValue.build());
}
derivedCase_ = 10;
return this;
}
/**
* .cz.proto.Udf udf = 10;
*/
public Builder mergeUdf(cz.proto.Expression.Udf value) {
if (udfBuilder_ == null) {
if (derivedCase_ == 10 &&
derived_ != cz.proto.Expression.Udf.getDefaultInstance()) {
derived_ = cz.proto.Expression.Udf.newBuilder((cz.proto.Expression.Udf) derived_)
.mergeFrom(value).buildPartial();
} else {
derived_ = value;
}
onChanged();
} else {
if (derivedCase_ == 10) {
udfBuilder_.mergeFrom(value);
}
udfBuilder_.setMessage(value);
}
derivedCase_ = 10;
return this;
}
/**
* .cz.proto.Udf udf = 10;
*/
public Builder clearUdf() {
if (udfBuilder_ == null) {
if (derivedCase_ == 10) {
derivedCase_ = 0;
derived_ = null;
onChanged();
}
} else {
if (derivedCase_ == 10) {
derivedCase_ = 0;
derived_ = null;
}
udfBuilder_.clear();
}
return this;
}
/**
* .cz.proto.Udf udf = 10;
*/
public cz.proto.Expression.Udf.Builder getUdfBuilder() {
return getUdfFieldBuilder().getBuilder();
}
/**
* .cz.proto.Udf udf = 10;
*/
@java.lang.Override
public cz.proto.Expression.UdfOrBuilder getUdfOrBuilder() {
if ((derivedCase_ == 10) && (udfBuilder_ != null)) {
return udfBuilder_.getMessageOrBuilder();
} else {
if (derivedCase_ == 10) {
return (cz.proto.Expression.Udf) derived_;
}
return cz.proto.Expression.Udf.getDefaultInstance();
}
}
/**
* .cz.proto.Udf udf = 10;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.Udf, cz.proto.Expression.Udf.Builder, cz.proto.Expression.UdfOrBuilder>
getUdfFieldBuilder() {
if (udfBuilder_ == null) {
if (!(derivedCase_ == 10)) {
derived_ = cz.proto.Expression.Udf.getDefaultInstance();
}
udfBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.Udf, cz.proto.Expression.Udf.Builder, cz.proto.Expression.UdfOrBuilder>(
(cz.proto.Expression.Udf) derived_,
getParentForChildren(),
isClean());
derived_ = null;
}
derivedCase_ = 10;
onChanged();;
return udfBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ScalarFunction)
}
// @@protoc_insertion_point(class_scope:cz.proto.ScalarFunction)
private static final cz.proto.Expression.ScalarFunction DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.Expression.ScalarFunction();
}
public static cz.proto.Expression.ScalarFunction getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ScalarFunction parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ScalarFunction(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.Expression.ScalarFunction getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface VariableDefOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.VariableDef)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.DataType type = 1;
* @return Whether the type field is set.
*/
boolean hasType();
/**
* .cz.proto.DataType type = 1;
* @return The type.
*/
cz.proto.DataType getType();
/**
* .cz.proto.DataType type = 1;
*/
cz.proto.DataTypeOrBuilder getTypeOrBuilder();
/**
* uint64 id = 2;
* @return The id.
*/
long getId();
}
/**
* Protobuf type {@code cz.proto.VariableDef}
*/
public static final class VariableDef extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.VariableDef)
VariableDefOrBuilder {
private static final long serialVersionUID = 0L;
// Use VariableDef.newBuilder() to construct.
private VariableDef(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private VariableDef() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new VariableDef();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private VariableDef(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.DataType.Builder subBuilder = null;
if (type_ != null) {
subBuilder = type_.toBuilder();
}
type_ = input.readMessage(cz.proto.DataType.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(type_);
type_ = subBuilder.buildPartial();
}
break;
}
case 16: {
id_ = input.readUInt64();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.Expression.internal_static_cz_proto_VariableDef_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.Expression.internal_static_cz_proto_VariableDef_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.Expression.VariableDef.class, cz.proto.Expression.VariableDef.Builder.class);
}
public static final int TYPE_FIELD_NUMBER = 1;
private cz.proto.DataType type_;
/**
* .cz.proto.DataType type = 1;
* @return Whether the type field is set.
*/
@java.lang.Override
public boolean hasType() {
return type_ != null;
}
/**
* .cz.proto.DataType type = 1;
* @return The type.
*/
@java.lang.Override
public cz.proto.DataType getType() {
return type_ == null ? cz.proto.DataType.getDefaultInstance() : type_;
}
/**
* .cz.proto.DataType type = 1;
*/
@java.lang.Override
public cz.proto.DataTypeOrBuilder getTypeOrBuilder() {
return getType();
}
public static final int ID_FIELD_NUMBER = 2;
private long id_;
/**
* uint64 id = 2;
* @return The id.
*/
@java.lang.Override
public long getId() {
return id_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (type_ != null) {
output.writeMessage(1, getType());
}
if (id_ != 0L) {
output.writeUInt64(2, id_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (type_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getType());
}
if (id_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(2, id_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.Expression.VariableDef)) {
return super.equals(obj);
}
cz.proto.Expression.VariableDef other = (cz.proto.Expression.VariableDef) obj;
if (hasType() != other.hasType()) return false;
if (hasType()) {
if (!getType()
.equals(other.getType())) return false;
}
if (getId()
!= other.getId()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasType()) {
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + getType().hashCode();
}
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getId());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.Expression.VariableDef parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.VariableDef parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.VariableDef parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.VariableDef parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.VariableDef parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.VariableDef parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.VariableDef parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.Expression.VariableDef parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.Expression.VariableDef parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.Expression.VariableDef parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.Expression.VariableDef parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.Expression.VariableDef parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.Expression.VariableDef prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.VariableDef}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.VariableDef)
cz.proto.Expression.VariableDefOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.Expression.internal_static_cz_proto_VariableDef_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.Expression.internal_static_cz_proto_VariableDef_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.Expression.VariableDef.class, cz.proto.Expression.VariableDef.Builder.class);
}
// Construct using cz.proto.Expression.VariableDef.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (typeBuilder_ == null) {
type_ = null;
} else {
type_ = null;
typeBuilder_ = null;
}
id_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.Expression.internal_static_cz_proto_VariableDef_descriptor;
}
@java.lang.Override
public cz.proto.Expression.VariableDef getDefaultInstanceForType() {
return cz.proto.Expression.VariableDef.getDefaultInstance();
}
@java.lang.Override
public cz.proto.Expression.VariableDef build() {
cz.proto.Expression.VariableDef result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.Expression.VariableDef buildPartial() {
cz.proto.Expression.VariableDef result = new cz.proto.Expression.VariableDef(this);
if (typeBuilder_ == null) {
result.type_ = type_;
} else {
result.type_ = typeBuilder_.build();
}
result.id_ = id_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.Expression.VariableDef) {
return mergeFrom((cz.proto.Expression.VariableDef)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.Expression.VariableDef other) {
if (other == cz.proto.Expression.VariableDef.getDefaultInstance()) return this;
if (other.hasType()) {
mergeType(other.getType());
}
if (other.getId() != 0L) {
setId(other.getId());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.Expression.VariableDef parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.Expression.VariableDef) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cz.proto.DataType type_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.DataType, cz.proto.DataType.Builder, cz.proto.DataTypeOrBuilder> typeBuilder_;
/**
* .cz.proto.DataType type = 1;
* @return Whether the type field is set.
*/
public boolean hasType() {
return typeBuilder_ != null || type_ != null;
}
/**
* .cz.proto.DataType type = 1;
* @return The type.
*/
public cz.proto.DataType getType() {
if (typeBuilder_ == null) {
return type_ == null ? cz.proto.DataType.getDefaultInstance() : type_;
} else {
return typeBuilder_.getMessage();
}
}
/**
* .cz.proto.DataType type = 1;
*/
public Builder setType(cz.proto.DataType value) {
if (typeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
type_ = value;
onChanged();
} else {
typeBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.DataType type = 1;
*/
public Builder setType(
cz.proto.DataType.Builder builderForValue) {
if (typeBuilder_ == null) {
type_ = builderForValue.build();
onChanged();
} else {
typeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.DataType type = 1;
*/
public Builder mergeType(cz.proto.DataType value) {
if (typeBuilder_ == null) {
if (type_ != null) {
type_ =
cz.proto.DataType.newBuilder(type_).mergeFrom(value).buildPartial();
} else {
type_ = value;
}
onChanged();
} else {
typeBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.DataType type = 1;
*/
public Builder clearType() {
if (typeBuilder_ == null) {
type_ = null;
onChanged();
} else {
type_ = null;
typeBuilder_ = null;
}
return this;
}
/**
* .cz.proto.DataType type = 1;
*/
public cz.proto.DataType.Builder getTypeBuilder() {
onChanged();
return getTypeFieldBuilder().getBuilder();
}
/**
* .cz.proto.DataType type = 1;
*/
public cz.proto.DataTypeOrBuilder getTypeOrBuilder() {
if (typeBuilder_ != null) {
return typeBuilder_.getMessageOrBuilder();
} else {
return type_ == null ?
cz.proto.DataType.getDefaultInstance() : type_;
}
}
/**
* .cz.proto.DataType type = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.DataType, cz.proto.DataType.Builder, cz.proto.DataTypeOrBuilder>
getTypeFieldBuilder() {
if (typeBuilder_ == null) {
typeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.DataType, cz.proto.DataType.Builder, cz.proto.DataTypeOrBuilder>(
getType(),
getParentForChildren(),
isClean());
type_ = null;
}
return typeBuilder_;
}
private long id_ ;
/**
* uint64 id = 2;
* @return The id.
*/
@java.lang.Override
public long getId() {
return id_;
}
/**
* uint64 id = 2;
* @param value The id to set.
* @return This builder for chaining.
*/
public Builder setId(long value) {
id_ = value;
onChanged();
return this;
}
/**
* uint64 id = 2;
* @return This builder for chaining.
*/
public Builder clearId() {
id_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.VariableDef)
}
// @@protoc_insertion_point(class_scope:cz.proto.VariableDef)
private static final cz.proto.Expression.VariableDef DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.Expression.VariableDef();
}
public static cz.proto.Expression.VariableDef getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public VariableDef parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new VariableDef(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.Expression.VariableDef getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface LambdaFunctionOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.LambdaFunction)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .cz.proto.VariableDef params = 1;
*/
java.util.List
getParamsList();
/**
* repeated .cz.proto.VariableDef params = 1;
*/
cz.proto.Expression.VariableDef getParams(int index);
/**
* repeated .cz.proto.VariableDef params = 1;
*/
int getParamsCount();
/**
* repeated .cz.proto.VariableDef params = 1;
*/
java.util.List extends cz.proto.Expression.VariableDefOrBuilder>
getParamsOrBuilderList();
/**
* repeated .cz.proto.VariableDef params = 1;
*/
cz.proto.Expression.VariableDefOrBuilder getParamsOrBuilder(
int index);
/**
* .cz.proto.ScalarExpression impl = 2;
* @return Whether the impl field is set.
*/
boolean hasImpl();
/**
* .cz.proto.ScalarExpression impl = 2;
* @return The impl.
*/
cz.proto.Expression.ScalarExpression getImpl();
/**
* .cz.proto.ScalarExpression impl = 2;
*/
cz.proto.Expression.ScalarExpressionOrBuilder getImplOrBuilder();
}
/**
* Protobuf type {@code cz.proto.LambdaFunction}
*/
public static final class LambdaFunction extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.LambdaFunction)
LambdaFunctionOrBuilder {
private static final long serialVersionUID = 0L;
// Use LambdaFunction.newBuilder() to construct.
private LambdaFunction(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private LambdaFunction() {
params_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new LambdaFunction();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private LambdaFunction(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
params_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
params_.add(
input.readMessage(cz.proto.Expression.VariableDef.parser(), extensionRegistry));
break;
}
case 18: {
cz.proto.Expression.ScalarExpression.Builder subBuilder = null;
if (impl_ != null) {
subBuilder = impl_.toBuilder();
}
impl_ = input.readMessage(cz.proto.Expression.ScalarExpression.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(impl_);
impl_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
params_ = java.util.Collections.unmodifiableList(params_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.Expression.internal_static_cz_proto_LambdaFunction_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.Expression.internal_static_cz_proto_LambdaFunction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.Expression.LambdaFunction.class, cz.proto.Expression.LambdaFunction.Builder.class);
}
public static final int PARAMS_FIELD_NUMBER = 1;
private java.util.List params_;
/**
* repeated .cz.proto.VariableDef params = 1;
*/
@java.lang.Override
public java.util.List getParamsList() {
return params_;
}
/**
* repeated .cz.proto.VariableDef params = 1;
*/
@java.lang.Override
public java.util.List extends cz.proto.Expression.VariableDefOrBuilder>
getParamsOrBuilderList() {
return params_;
}
/**
* repeated .cz.proto.VariableDef params = 1;
*/
@java.lang.Override
public int getParamsCount() {
return params_.size();
}
/**
* repeated .cz.proto.VariableDef params = 1;
*/
@java.lang.Override
public cz.proto.Expression.VariableDef getParams(int index) {
return params_.get(index);
}
/**
* repeated .cz.proto.VariableDef params = 1;
*/
@java.lang.Override
public cz.proto.Expression.VariableDefOrBuilder getParamsOrBuilder(
int index) {
return params_.get(index);
}
public static final int IMPL_FIELD_NUMBER = 2;
private cz.proto.Expression.ScalarExpression impl_;
/**
* .cz.proto.ScalarExpression impl = 2;
* @return Whether the impl field is set.
*/
@java.lang.Override
public boolean hasImpl() {
return impl_ != null;
}
/**
* .cz.proto.ScalarExpression impl = 2;
* @return The impl.
*/
@java.lang.Override
public cz.proto.Expression.ScalarExpression getImpl() {
return impl_ == null ? cz.proto.Expression.ScalarExpression.getDefaultInstance() : impl_;
}
/**
* .cz.proto.ScalarExpression impl = 2;
*/
@java.lang.Override
public cz.proto.Expression.ScalarExpressionOrBuilder getImplOrBuilder() {
return getImpl();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < params_.size(); i++) {
output.writeMessage(1, params_.get(i));
}
if (impl_ != null) {
output.writeMessage(2, getImpl());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < params_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, params_.get(i));
}
if (impl_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getImpl());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.Expression.LambdaFunction)) {
return super.equals(obj);
}
cz.proto.Expression.LambdaFunction other = (cz.proto.Expression.LambdaFunction) obj;
if (!getParamsList()
.equals(other.getParamsList())) return false;
if (hasImpl() != other.hasImpl()) return false;
if (hasImpl()) {
if (!getImpl()
.equals(other.getImpl())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getParamsCount() > 0) {
hash = (37 * hash) + PARAMS_FIELD_NUMBER;
hash = (53 * hash) + getParamsList().hashCode();
}
if (hasImpl()) {
hash = (37 * hash) + IMPL_FIELD_NUMBER;
hash = (53 * hash) + getImpl().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.Expression.LambdaFunction parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.LambdaFunction parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.LambdaFunction parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.LambdaFunction parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.LambdaFunction parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.LambdaFunction parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.LambdaFunction parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.Expression.LambdaFunction parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.Expression.LambdaFunction parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.Expression.LambdaFunction parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.Expression.LambdaFunction parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.Expression.LambdaFunction parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.Expression.LambdaFunction prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.LambdaFunction}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.LambdaFunction)
cz.proto.Expression.LambdaFunctionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.Expression.internal_static_cz_proto_LambdaFunction_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.Expression.internal_static_cz_proto_LambdaFunction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.Expression.LambdaFunction.class, cz.proto.Expression.LambdaFunction.Builder.class);
}
// Construct using cz.proto.Expression.LambdaFunction.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getParamsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (paramsBuilder_ == null) {
params_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
paramsBuilder_.clear();
}
if (implBuilder_ == null) {
impl_ = null;
} else {
impl_ = null;
implBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.Expression.internal_static_cz_proto_LambdaFunction_descriptor;
}
@java.lang.Override
public cz.proto.Expression.LambdaFunction getDefaultInstanceForType() {
return cz.proto.Expression.LambdaFunction.getDefaultInstance();
}
@java.lang.Override
public cz.proto.Expression.LambdaFunction build() {
cz.proto.Expression.LambdaFunction result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.Expression.LambdaFunction buildPartial() {
cz.proto.Expression.LambdaFunction result = new cz.proto.Expression.LambdaFunction(this);
int from_bitField0_ = bitField0_;
if (paramsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
params_ = java.util.Collections.unmodifiableList(params_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.params_ = params_;
} else {
result.params_ = paramsBuilder_.build();
}
if (implBuilder_ == null) {
result.impl_ = impl_;
} else {
result.impl_ = implBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.Expression.LambdaFunction) {
return mergeFrom((cz.proto.Expression.LambdaFunction)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.Expression.LambdaFunction other) {
if (other == cz.proto.Expression.LambdaFunction.getDefaultInstance()) return this;
if (paramsBuilder_ == null) {
if (!other.params_.isEmpty()) {
if (params_.isEmpty()) {
params_ = other.params_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureParamsIsMutable();
params_.addAll(other.params_);
}
onChanged();
}
} else {
if (!other.params_.isEmpty()) {
if (paramsBuilder_.isEmpty()) {
paramsBuilder_.dispose();
paramsBuilder_ = null;
params_ = other.params_;
bitField0_ = (bitField0_ & ~0x00000001);
paramsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getParamsFieldBuilder() : null;
} else {
paramsBuilder_.addAllMessages(other.params_);
}
}
}
if (other.hasImpl()) {
mergeImpl(other.getImpl());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.Expression.LambdaFunction parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.Expression.LambdaFunction) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List params_ =
java.util.Collections.emptyList();
private void ensureParamsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
params_ = new java.util.ArrayList(params_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.Expression.VariableDef, cz.proto.Expression.VariableDef.Builder, cz.proto.Expression.VariableDefOrBuilder> paramsBuilder_;
/**
* repeated .cz.proto.VariableDef params = 1;
*/
public java.util.List getParamsList() {
if (paramsBuilder_ == null) {
return java.util.Collections.unmodifiableList(params_);
} else {
return paramsBuilder_.getMessageList();
}
}
/**
* repeated .cz.proto.VariableDef params = 1;
*/
public int getParamsCount() {
if (paramsBuilder_ == null) {
return params_.size();
} else {
return paramsBuilder_.getCount();
}
}
/**
* repeated .cz.proto.VariableDef params = 1;
*/
public cz.proto.Expression.VariableDef getParams(int index) {
if (paramsBuilder_ == null) {
return params_.get(index);
} else {
return paramsBuilder_.getMessage(index);
}
}
/**
* repeated .cz.proto.VariableDef params = 1;
*/
public Builder setParams(
int index, cz.proto.Expression.VariableDef value) {
if (paramsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureParamsIsMutable();
params_.set(index, value);
onChanged();
} else {
paramsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .cz.proto.VariableDef params = 1;
*/
public Builder setParams(
int index, cz.proto.Expression.VariableDef.Builder builderForValue) {
if (paramsBuilder_ == null) {
ensureParamsIsMutable();
params_.set(index, builderForValue.build());
onChanged();
} else {
paramsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.VariableDef params = 1;
*/
public Builder addParams(cz.proto.Expression.VariableDef value) {
if (paramsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureParamsIsMutable();
params_.add(value);
onChanged();
} else {
paramsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .cz.proto.VariableDef params = 1;
*/
public Builder addParams(
int index, cz.proto.Expression.VariableDef value) {
if (paramsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureParamsIsMutable();
params_.add(index, value);
onChanged();
} else {
paramsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .cz.proto.VariableDef params = 1;
*/
public Builder addParams(
cz.proto.Expression.VariableDef.Builder builderForValue) {
if (paramsBuilder_ == null) {
ensureParamsIsMutable();
params_.add(builderForValue.build());
onChanged();
} else {
paramsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.VariableDef params = 1;
*/
public Builder addParams(
int index, cz.proto.Expression.VariableDef.Builder builderForValue) {
if (paramsBuilder_ == null) {
ensureParamsIsMutable();
params_.add(index, builderForValue.build());
onChanged();
} else {
paramsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.VariableDef params = 1;
*/
public Builder addAllParams(
java.lang.Iterable extends cz.proto.Expression.VariableDef> values) {
if (paramsBuilder_ == null) {
ensureParamsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, params_);
onChanged();
} else {
paramsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .cz.proto.VariableDef params = 1;
*/
public Builder clearParams() {
if (paramsBuilder_ == null) {
params_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
paramsBuilder_.clear();
}
return this;
}
/**
* repeated .cz.proto.VariableDef params = 1;
*/
public Builder removeParams(int index) {
if (paramsBuilder_ == null) {
ensureParamsIsMutable();
params_.remove(index);
onChanged();
} else {
paramsBuilder_.remove(index);
}
return this;
}
/**
* repeated .cz.proto.VariableDef params = 1;
*/
public cz.proto.Expression.VariableDef.Builder getParamsBuilder(
int index) {
return getParamsFieldBuilder().getBuilder(index);
}
/**
* repeated .cz.proto.VariableDef params = 1;
*/
public cz.proto.Expression.VariableDefOrBuilder getParamsOrBuilder(
int index) {
if (paramsBuilder_ == null) {
return params_.get(index); } else {
return paramsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .cz.proto.VariableDef params = 1;
*/
public java.util.List extends cz.proto.Expression.VariableDefOrBuilder>
getParamsOrBuilderList() {
if (paramsBuilder_ != null) {
return paramsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(params_);
}
}
/**
* repeated .cz.proto.VariableDef params = 1;
*/
public cz.proto.Expression.VariableDef.Builder addParamsBuilder() {
return getParamsFieldBuilder().addBuilder(
cz.proto.Expression.VariableDef.getDefaultInstance());
}
/**
* repeated .cz.proto.VariableDef params = 1;
*/
public cz.proto.Expression.VariableDef.Builder addParamsBuilder(
int index) {
return getParamsFieldBuilder().addBuilder(
index, cz.proto.Expression.VariableDef.getDefaultInstance());
}
/**
* repeated .cz.proto.VariableDef params = 1;
*/
public java.util.List
getParamsBuilderList() {
return getParamsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.Expression.VariableDef, cz.proto.Expression.VariableDef.Builder, cz.proto.Expression.VariableDefOrBuilder>
getParamsFieldBuilder() {
if (paramsBuilder_ == null) {
paramsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.Expression.VariableDef, cz.proto.Expression.VariableDef.Builder, cz.proto.Expression.VariableDefOrBuilder>(
params_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
params_ = null;
}
return paramsBuilder_;
}
private cz.proto.Expression.ScalarExpression impl_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.ScalarExpression, cz.proto.Expression.ScalarExpression.Builder, cz.proto.Expression.ScalarExpressionOrBuilder> implBuilder_;
/**
* .cz.proto.ScalarExpression impl = 2;
* @return Whether the impl field is set.
*/
public boolean hasImpl() {
return implBuilder_ != null || impl_ != null;
}
/**
* .cz.proto.ScalarExpression impl = 2;
* @return The impl.
*/
public cz.proto.Expression.ScalarExpression getImpl() {
if (implBuilder_ == null) {
return impl_ == null ? cz.proto.Expression.ScalarExpression.getDefaultInstance() : impl_;
} else {
return implBuilder_.getMessage();
}
}
/**
* .cz.proto.ScalarExpression impl = 2;
*/
public Builder setImpl(cz.proto.Expression.ScalarExpression value) {
if (implBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
impl_ = value;
onChanged();
} else {
implBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ScalarExpression impl = 2;
*/
public Builder setImpl(
cz.proto.Expression.ScalarExpression.Builder builderForValue) {
if (implBuilder_ == null) {
impl_ = builderForValue.build();
onChanged();
} else {
implBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ScalarExpression impl = 2;
*/
public Builder mergeImpl(cz.proto.Expression.ScalarExpression value) {
if (implBuilder_ == null) {
if (impl_ != null) {
impl_ =
cz.proto.Expression.ScalarExpression.newBuilder(impl_).mergeFrom(value).buildPartial();
} else {
impl_ = value;
}
onChanged();
} else {
implBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ScalarExpression impl = 2;
*/
public Builder clearImpl() {
if (implBuilder_ == null) {
impl_ = null;
onChanged();
} else {
impl_ = null;
implBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ScalarExpression impl = 2;
*/
public cz.proto.Expression.ScalarExpression.Builder getImplBuilder() {
onChanged();
return getImplFieldBuilder().getBuilder();
}
/**
* .cz.proto.ScalarExpression impl = 2;
*/
public cz.proto.Expression.ScalarExpressionOrBuilder getImplOrBuilder() {
if (implBuilder_ != null) {
return implBuilder_.getMessageOrBuilder();
} else {
return impl_ == null ?
cz.proto.Expression.ScalarExpression.getDefaultInstance() : impl_;
}
}
/**
* .cz.proto.ScalarExpression impl = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.ScalarExpression, cz.proto.Expression.ScalarExpression.Builder, cz.proto.Expression.ScalarExpressionOrBuilder>
getImplFieldBuilder() {
if (implBuilder_ == null) {
implBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.ScalarExpression, cz.proto.Expression.ScalarExpression.Builder, cz.proto.Expression.ScalarExpressionOrBuilder>(
getImpl(),
getParentForChildren(),
isClean());
impl_ = null;
}
return implBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.LambdaFunction)
}
// @@protoc_insertion_point(class_scope:cz.proto.LambdaFunction)
private static final cz.proto.Expression.LambdaFunction DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.Expression.LambdaFunction();
}
public static cz.proto.Expression.LambdaFunction getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public LambdaFunction parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new LambdaFunction(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.Expression.LambdaFunction getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ScalarExpressionOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ScalarExpression)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.DataType type = 1;
* @return Whether the type field is set.
*/
boolean hasType();
/**
* .cz.proto.DataType type = 1;
* @return The type.
*/
cz.proto.DataType getType();
/**
* .cz.proto.DataType type = 1;
*/
cz.proto.DataTypeOrBuilder getTypeOrBuilder();
/**
* .cz.proto.Constant constant = 2;
* @return Whether the constant field is set.
*/
boolean hasConstant();
/**
* .cz.proto.Constant constant = 2;
* @return The constant.
*/
cz.proto.Expression.Constant getConstant();
/**
* .cz.proto.Constant constant = 2;
*/
cz.proto.Expression.ConstantOrBuilder getConstantOrBuilder();
/**
* .cz.proto.Reference reference = 3;
* @return Whether the reference field is set.
*/
boolean hasReference();
/**
* .cz.proto.Reference reference = 3;
* @return The reference.
*/
cz.proto.Expression.Reference getReference();
/**
* .cz.proto.Reference reference = 3;
*/
cz.proto.Expression.ReferenceOrBuilder getReferenceOrBuilder();
/**
* .cz.proto.ScalarFunction function = 5;
* @return Whether the function field is set.
*/
boolean hasFunction();
/**
* .cz.proto.ScalarFunction function = 5;
* @return The function.
*/
cz.proto.Expression.ScalarFunction getFunction();
/**
* .cz.proto.ScalarFunction function = 5;
*/
cz.proto.Expression.ScalarFunctionOrBuilder getFunctionOrBuilder();
/**
* .cz.proto.LambdaFunction lambda = 6;
* @return Whether the lambda field is set.
*/
boolean hasLambda();
/**
* .cz.proto.LambdaFunction lambda = 6;
* @return The lambda.
*/
cz.proto.Expression.LambdaFunction getLambda();
/**
* .cz.proto.LambdaFunction lambda = 6;
*/
cz.proto.Expression.LambdaFunctionOrBuilder getLambdaOrBuilder();
/**
* .cz.proto.ParseTreeInfo pt = 10;
* @return Whether the pt field is set.
*/
boolean hasPt();
/**
* .cz.proto.ParseTreeInfo pt = 10;
* @return The pt.
*/
cz.proto.Expression.ParseTreeInfo getPt();
/**
* .cz.proto.ParseTreeInfo pt = 10;
*/
cz.proto.Expression.ParseTreeInfoOrBuilder getPtOrBuilder();
public cz.proto.Expression.ScalarExpression.ValueCase getValueCase();
}
/**
* Protobuf type {@code cz.proto.ScalarExpression}
*/
public static final class ScalarExpression extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ScalarExpression)
ScalarExpressionOrBuilder {
private static final long serialVersionUID = 0L;
// Use ScalarExpression.newBuilder() to construct.
private ScalarExpression(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ScalarExpression() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ScalarExpression();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ScalarExpression(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.DataType.Builder subBuilder = null;
if (type_ != null) {
subBuilder = type_.toBuilder();
}
type_ = input.readMessage(cz.proto.DataType.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(type_);
type_ = subBuilder.buildPartial();
}
break;
}
case 18: {
cz.proto.Expression.Constant.Builder subBuilder = null;
if (valueCase_ == 2) {
subBuilder = ((cz.proto.Expression.Constant) value_).toBuilder();
}
value_ =
input.readMessage(cz.proto.Expression.Constant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((cz.proto.Expression.Constant) value_);
value_ = subBuilder.buildPartial();
}
valueCase_ = 2;
break;
}
case 26: {
cz.proto.Expression.Reference.Builder subBuilder = null;
if (valueCase_ == 3) {
subBuilder = ((cz.proto.Expression.Reference) value_).toBuilder();
}
value_ =
input.readMessage(cz.proto.Expression.Reference.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((cz.proto.Expression.Reference) value_);
value_ = subBuilder.buildPartial();
}
valueCase_ = 3;
break;
}
case 42: {
cz.proto.Expression.ScalarFunction.Builder subBuilder = null;
if (valueCase_ == 5) {
subBuilder = ((cz.proto.Expression.ScalarFunction) value_).toBuilder();
}
value_ =
input.readMessage(cz.proto.Expression.ScalarFunction.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((cz.proto.Expression.ScalarFunction) value_);
value_ = subBuilder.buildPartial();
}
valueCase_ = 5;
break;
}
case 50: {
cz.proto.Expression.LambdaFunction.Builder subBuilder = null;
if (valueCase_ == 6) {
subBuilder = ((cz.proto.Expression.LambdaFunction) value_).toBuilder();
}
value_ =
input.readMessage(cz.proto.Expression.LambdaFunction.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((cz.proto.Expression.LambdaFunction) value_);
value_ = subBuilder.buildPartial();
}
valueCase_ = 6;
break;
}
case 82: {
cz.proto.Expression.ParseTreeInfo.Builder subBuilder = null;
if (pt_ != null) {
subBuilder = pt_.toBuilder();
}
pt_ = input.readMessage(cz.proto.Expression.ParseTreeInfo.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(pt_);
pt_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.Expression.internal_static_cz_proto_ScalarExpression_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.Expression.internal_static_cz_proto_ScalarExpression_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.Expression.ScalarExpression.class, cz.proto.Expression.ScalarExpression.Builder.class);
}
private int valueCase_ = 0;
private java.lang.Object value_;
public enum ValueCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
CONSTANT(2),
REFERENCE(3),
FUNCTION(5),
LAMBDA(6),
VALUE_NOT_SET(0);
private final int value;
private ValueCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ValueCase valueOf(int value) {
return forNumber(value);
}
public static ValueCase forNumber(int value) {
switch (value) {
case 2: return CONSTANT;
case 3: return REFERENCE;
case 5: return FUNCTION;
case 6: return LAMBDA;
case 0: return VALUE_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public ValueCase
getValueCase() {
return ValueCase.forNumber(
valueCase_);
}
public static final int TYPE_FIELD_NUMBER = 1;
private cz.proto.DataType type_;
/**
* .cz.proto.DataType type = 1;
* @return Whether the type field is set.
*/
@java.lang.Override
public boolean hasType() {
return type_ != null;
}
/**
* .cz.proto.DataType type = 1;
* @return The type.
*/
@java.lang.Override
public cz.proto.DataType getType() {
return type_ == null ? cz.proto.DataType.getDefaultInstance() : type_;
}
/**
* .cz.proto.DataType type = 1;
*/
@java.lang.Override
public cz.proto.DataTypeOrBuilder getTypeOrBuilder() {
return getType();
}
public static final int CONSTANT_FIELD_NUMBER = 2;
/**
* .cz.proto.Constant constant = 2;
* @return Whether the constant field is set.
*/
@java.lang.Override
public boolean hasConstant() {
return valueCase_ == 2;
}
/**
* .cz.proto.Constant constant = 2;
* @return The constant.
*/
@java.lang.Override
public cz.proto.Expression.Constant getConstant() {
if (valueCase_ == 2) {
return (cz.proto.Expression.Constant) value_;
}
return cz.proto.Expression.Constant.getDefaultInstance();
}
/**
* .cz.proto.Constant constant = 2;
*/
@java.lang.Override
public cz.proto.Expression.ConstantOrBuilder getConstantOrBuilder() {
if (valueCase_ == 2) {
return (cz.proto.Expression.Constant) value_;
}
return cz.proto.Expression.Constant.getDefaultInstance();
}
public static final int REFERENCE_FIELD_NUMBER = 3;
/**
* .cz.proto.Reference reference = 3;
* @return Whether the reference field is set.
*/
@java.lang.Override
public boolean hasReference() {
return valueCase_ == 3;
}
/**
* .cz.proto.Reference reference = 3;
* @return The reference.
*/
@java.lang.Override
public cz.proto.Expression.Reference getReference() {
if (valueCase_ == 3) {
return (cz.proto.Expression.Reference) value_;
}
return cz.proto.Expression.Reference.getDefaultInstance();
}
/**
* .cz.proto.Reference reference = 3;
*/
@java.lang.Override
public cz.proto.Expression.ReferenceOrBuilder getReferenceOrBuilder() {
if (valueCase_ == 3) {
return (cz.proto.Expression.Reference) value_;
}
return cz.proto.Expression.Reference.getDefaultInstance();
}
public static final int FUNCTION_FIELD_NUMBER = 5;
/**
* .cz.proto.ScalarFunction function = 5;
* @return Whether the function field is set.
*/
@java.lang.Override
public boolean hasFunction() {
return valueCase_ == 5;
}
/**
* .cz.proto.ScalarFunction function = 5;
* @return The function.
*/
@java.lang.Override
public cz.proto.Expression.ScalarFunction getFunction() {
if (valueCase_ == 5) {
return (cz.proto.Expression.ScalarFunction) value_;
}
return cz.proto.Expression.ScalarFunction.getDefaultInstance();
}
/**
* .cz.proto.ScalarFunction function = 5;
*/
@java.lang.Override
public cz.proto.Expression.ScalarFunctionOrBuilder getFunctionOrBuilder() {
if (valueCase_ == 5) {
return (cz.proto.Expression.ScalarFunction) value_;
}
return cz.proto.Expression.ScalarFunction.getDefaultInstance();
}
public static final int LAMBDA_FIELD_NUMBER = 6;
/**
* .cz.proto.LambdaFunction lambda = 6;
* @return Whether the lambda field is set.
*/
@java.lang.Override
public boolean hasLambda() {
return valueCase_ == 6;
}
/**
* .cz.proto.LambdaFunction lambda = 6;
* @return The lambda.
*/
@java.lang.Override
public cz.proto.Expression.LambdaFunction getLambda() {
if (valueCase_ == 6) {
return (cz.proto.Expression.LambdaFunction) value_;
}
return cz.proto.Expression.LambdaFunction.getDefaultInstance();
}
/**
* .cz.proto.LambdaFunction lambda = 6;
*/
@java.lang.Override
public cz.proto.Expression.LambdaFunctionOrBuilder getLambdaOrBuilder() {
if (valueCase_ == 6) {
return (cz.proto.Expression.LambdaFunction) value_;
}
return cz.proto.Expression.LambdaFunction.getDefaultInstance();
}
public static final int PT_FIELD_NUMBER = 10;
private cz.proto.Expression.ParseTreeInfo pt_;
/**
* .cz.proto.ParseTreeInfo pt = 10;
* @return Whether the pt field is set.
*/
@java.lang.Override
public boolean hasPt() {
return pt_ != null;
}
/**
* .cz.proto.ParseTreeInfo pt = 10;
* @return The pt.
*/
@java.lang.Override
public cz.proto.Expression.ParseTreeInfo getPt() {
return pt_ == null ? cz.proto.Expression.ParseTreeInfo.getDefaultInstance() : pt_;
}
/**
* .cz.proto.ParseTreeInfo pt = 10;
*/
@java.lang.Override
public cz.proto.Expression.ParseTreeInfoOrBuilder getPtOrBuilder() {
return getPt();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (type_ != null) {
output.writeMessage(1, getType());
}
if (valueCase_ == 2) {
output.writeMessage(2, (cz.proto.Expression.Constant) value_);
}
if (valueCase_ == 3) {
output.writeMessage(3, (cz.proto.Expression.Reference) value_);
}
if (valueCase_ == 5) {
output.writeMessage(5, (cz.proto.Expression.ScalarFunction) value_);
}
if (valueCase_ == 6) {
output.writeMessage(6, (cz.proto.Expression.LambdaFunction) value_);
}
if (pt_ != null) {
output.writeMessage(10, getPt());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (type_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getType());
}
if (valueCase_ == 2) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, (cz.proto.Expression.Constant) value_);
}
if (valueCase_ == 3) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, (cz.proto.Expression.Reference) value_);
}
if (valueCase_ == 5) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, (cz.proto.Expression.ScalarFunction) value_);
}
if (valueCase_ == 6) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, (cz.proto.Expression.LambdaFunction) value_);
}
if (pt_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, getPt());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.Expression.ScalarExpression)) {
return super.equals(obj);
}
cz.proto.Expression.ScalarExpression other = (cz.proto.Expression.ScalarExpression) obj;
if (hasType() != other.hasType()) return false;
if (hasType()) {
if (!getType()
.equals(other.getType())) return false;
}
if (hasPt() != other.hasPt()) return false;
if (hasPt()) {
if (!getPt()
.equals(other.getPt())) return false;
}
if (!getValueCase().equals(other.getValueCase())) return false;
switch (valueCase_) {
case 2:
if (!getConstant()
.equals(other.getConstant())) return false;
break;
case 3:
if (!getReference()
.equals(other.getReference())) return false;
break;
case 5:
if (!getFunction()
.equals(other.getFunction())) return false;
break;
case 6:
if (!getLambda()
.equals(other.getLambda())) return false;
break;
case 0:
default:
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasType()) {
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + getType().hashCode();
}
if (hasPt()) {
hash = (37 * hash) + PT_FIELD_NUMBER;
hash = (53 * hash) + getPt().hashCode();
}
switch (valueCase_) {
case 2:
hash = (37 * hash) + CONSTANT_FIELD_NUMBER;
hash = (53 * hash) + getConstant().hashCode();
break;
case 3:
hash = (37 * hash) + REFERENCE_FIELD_NUMBER;
hash = (53 * hash) + getReference().hashCode();
break;
case 5:
hash = (37 * hash) + FUNCTION_FIELD_NUMBER;
hash = (53 * hash) + getFunction().hashCode();
break;
case 6:
hash = (37 * hash) + LAMBDA_FIELD_NUMBER;
hash = (53 * hash) + getLambda().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.Expression.ScalarExpression parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.ScalarExpression parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.ScalarExpression parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.ScalarExpression parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.ScalarExpression parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.Expression.ScalarExpression parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.Expression.ScalarExpression parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.Expression.ScalarExpression parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.Expression.ScalarExpression parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.Expression.ScalarExpression parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.Expression.ScalarExpression parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.Expression.ScalarExpression parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.Expression.ScalarExpression prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ScalarExpression}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ScalarExpression)
cz.proto.Expression.ScalarExpressionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.Expression.internal_static_cz_proto_ScalarExpression_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.Expression.internal_static_cz_proto_ScalarExpression_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.Expression.ScalarExpression.class, cz.proto.Expression.ScalarExpression.Builder.class);
}
// Construct using cz.proto.Expression.ScalarExpression.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (typeBuilder_ == null) {
type_ = null;
} else {
type_ = null;
typeBuilder_ = null;
}
if (ptBuilder_ == null) {
pt_ = null;
} else {
pt_ = null;
ptBuilder_ = null;
}
valueCase_ = 0;
value_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.Expression.internal_static_cz_proto_ScalarExpression_descriptor;
}
@java.lang.Override
public cz.proto.Expression.ScalarExpression getDefaultInstanceForType() {
return cz.proto.Expression.ScalarExpression.getDefaultInstance();
}
@java.lang.Override
public cz.proto.Expression.ScalarExpression build() {
cz.proto.Expression.ScalarExpression result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.Expression.ScalarExpression buildPartial() {
cz.proto.Expression.ScalarExpression result = new cz.proto.Expression.ScalarExpression(this);
if (typeBuilder_ == null) {
result.type_ = type_;
} else {
result.type_ = typeBuilder_.build();
}
if (valueCase_ == 2) {
if (constantBuilder_ == null) {
result.value_ = value_;
} else {
result.value_ = constantBuilder_.build();
}
}
if (valueCase_ == 3) {
if (referenceBuilder_ == null) {
result.value_ = value_;
} else {
result.value_ = referenceBuilder_.build();
}
}
if (valueCase_ == 5) {
if (functionBuilder_ == null) {
result.value_ = value_;
} else {
result.value_ = functionBuilder_.build();
}
}
if (valueCase_ == 6) {
if (lambdaBuilder_ == null) {
result.value_ = value_;
} else {
result.value_ = lambdaBuilder_.build();
}
}
if (ptBuilder_ == null) {
result.pt_ = pt_;
} else {
result.pt_ = ptBuilder_.build();
}
result.valueCase_ = valueCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.Expression.ScalarExpression) {
return mergeFrom((cz.proto.Expression.ScalarExpression)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.Expression.ScalarExpression other) {
if (other == cz.proto.Expression.ScalarExpression.getDefaultInstance()) return this;
if (other.hasType()) {
mergeType(other.getType());
}
if (other.hasPt()) {
mergePt(other.getPt());
}
switch (other.getValueCase()) {
case CONSTANT: {
mergeConstant(other.getConstant());
break;
}
case REFERENCE: {
mergeReference(other.getReference());
break;
}
case FUNCTION: {
mergeFunction(other.getFunction());
break;
}
case LAMBDA: {
mergeLambda(other.getLambda());
break;
}
case VALUE_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.Expression.ScalarExpression parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.Expression.ScalarExpression) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int valueCase_ = 0;
private java.lang.Object value_;
public ValueCase
getValueCase() {
return ValueCase.forNumber(
valueCase_);
}
public Builder clearValue() {
valueCase_ = 0;
value_ = null;
onChanged();
return this;
}
private cz.proto.DataType type_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.DataType, cz.proto.DataType.Builder, cz.proto.DataTypeOrBuilder> typeBuilder_;
/**
* .cz.proto.DataType type = 1;
* @return Whether the type field is set.
*/
public boolean hasType() {
return typeBuilder_ != null || type_ != null;
}
/**
* .cz.proto.DataType type = 1;
* @return The type.
*/
public cz.proto.DataType getType() {
if (typeBuilder_ == null) {
return type_ == null ? cz.proto.DataType.getDefaultInstance() : type_;
} else {
return typeBuilder_.getMessage();
}
}
/**
* .cz.proto.DataType type = 1;
*/
public Builder setType(cz.proto.DataType value) {
if (typeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
type_ = value;
onChanged();
} else {
typeBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.DataType type = 1;
*/
public Builder setType(
cz.proto.DataType.Builder builderForValue) {
if (typeBuilder_ == null) {
type_ = builderForValue.build();
onChanged();
} else {
typeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.DataType type = 1;
*/
public Builder mergeType(cz.proto.DataType value) {
if (typeBuilder_ == null) {
if (type_ != null) {
type_ =
cz.proto.DataType.newBuilder(type_).mergeFrom(value).buildPartial();
} else {
type_ = value;
}
onChanged();
} else {
typeBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.DataType type = 1;
*/
public Builder clearType() {
if (typeBuilder_ == null) {
type_ = null;
onChanged();
} else {
type_ = null;
typeBuilder_ = null;
}
return this;
}
/**
* .cz.proto.DataType type = 1;
*/
public cz.proto.DataType.Builder getTypeBuilder() {
onChanged();
return getTypeFieldBuilder().getBuilder();
}
/**
* .cz.proto.DataType type = 1;
*/
public cz.proto.DataTypeOrBuilder getTypeOrBuilder() {
if (typeBuilder_ != null) {
return typeBuilder_.getMessageOrBuilder();
} else {
return type_ == null ?
cz.proto.DataType.getDefaultInstance() : type_;
}
}
/**
* .cz.proto.DataType type = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.DataType, cz.proto.DataType.Builder, cz.proto.DataTypeOrBuilder>
getTypeFieldBuilder() {
if (typeBuilder_ == null) {
typeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.DataType, cz.proto.DataType.Builder, cz.proto.DataTypeOrBuilder>(
getType(),
getParentForChildren(),
isClean());
type_ = null;
}
return typeBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.Constant, cz.proto.Expression.Constant.Builder, cz.proto.Expression.ConstantOrBuilder> constantBuilder_;
/**
* .cz.proto.Constant constant = 2;
* @return Whether the constant field is set.
*/
@java.lang.Override
public boolean hasConstant() {
return valueCase_ == 2;
}
/**
* .cz.proto.Constant constant = 2;
* @return The constant.
*/
@java.lang.Override
public cz.proto.Expression.Constant getConstant() {
if (constantBuilder_ == null) {
if (valueCase_ == 2) {
return (cz.proto.Expression.Constant) value_;
}
return cz.proto.Expression.Constant.getDefaultInstance();
} else {
if (valueCase_ == 2) {
return constantBuilder_.getMessage();
}
return cz.proto.Expression.Constant.getDefaultInstance();
}
}
/**
* .cz.proto.Constant constant = 2;
*/
public Builder setConstant(cz.proto.Expression.Constant value) {
if (constantBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
value_ = value;
onChanged();
} else {
constantBuilder_.setMessage(value);
}
valueCase_ = 2;
return this;
}
/**
* .cz.proto.Constant constant = 2;
*/
public Builder setConstant(
cz.proto.Expression.Constant.Builder builderForValue) {
if (constantBuilder_ == null) {
value_ = builderForValue.build();
onChanged();
} else {
constantBuilder_.setMessage(builderForValue.build());
}
valueCase_ = 2;
return this;
}
/**
* .cz.proto.Constant constant = 2;
*/
public Builder mergeConstant(cz.proto.Expression.Constant value) {
if (constantBuilder_ == null) {
if (valueCase_ == 2 &&
value_ != cz.proto.Expression.Constant.getDefaultInstance()) {
value_ = cz.proto.Expression.Constant.newBuilder((cz.proto.Expression.Constant) value_)
.mergeFrom(value).buildPartial();
} else {
value_ = value;
}
onChanged();
} else {
if (valueCase_ == 2) {
constantBuilder_.mergeFrom(value);
}
constantBuilder_.setMessage(value);
}
valueCase_ = 2;
return this;
}
/**
* .cz.proto.Constant constant = 2;
*/
public Builder clearConstant() {
if (constantBuilder_ == null) {
if (valueCase_ == 2) {
valueCase_ = 0;
value_ = null;
onChanged();
}
} else {
if (valueCase_ == 2) {
valueCase_ = 0;
value_ = null;
}
constantBuilder_.clear();
}
return this;
}
/**
* .cz.proto.Constant constant = 2;
*/
public cz.proto.Expression.Constant.Builder getConstantBuilder() {
return getConstantFieldBuilder().getBuilder();
}
/**
* .cz.proto.Constant constant = 2;
*/
@java.lang.Override
public cz.proto.Expression.ConstantOrBuilder getConstantOrBuilder() {
if ((valueCase_ == 2) && (constantBuilder_ != null)) {
return constantBuilder_.getMessageOrBuilder();
} else {
if (valueCase_ == 2) {
return (cz.proto.Expression.Constant) value_;
}
return cz.proto.Expression.Constant.getDefaultInstance();
}
}
/**
* .cz.proto.Constant constant = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.Constant, cz.proto.Expression.Constant.Builder, cz.proto.Expression.ConstantOrBuilder>
getConstantFieldBuilder() {
if (constantBuilder_ == null) {
if (!(valueCase_ == 2)) {
value_ = cz.proto.Expression.Constant.getDefaultInstance();
}
constantBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.Constant, cz.proto.Expression.Constant.Builder, cz.proto.Expression.ConstantOrBuilder>(
(cz.proto.Expression.Constant) value_,
getParentForChildren(),
isClean());
value_ = null;
}
valueCase_ = 2;
onChanged();;
return constantBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.Reference, cz.proto.Expression.Reference.Builder, cz.proto.Expression.ReferenceOrBuilder> referenceBuilder_;
/**
* .cz.proto.Reference reference = 3;
* @return Whether the reference field is set.
*/
@java.lang.Override
public boolean hasReference() {
return valueCase_ == 3;
}
/**
* .cz.proto.Reference reference = 3;
* @return The reference.
*/
@java.lang.Override
public cz.proto.Expression.Reference getReference() {
if (referenceBuilder_ == null) {
if (valueCase_ == 3) {
return (cz.proto.Expression.Reference) value_;
}
return cz.proto.Expression.Reference.getDefaultInstance();
} else {
if (valueCase_ == 3) {
return referenceBuilder_.getMessage();
}
return cz.proto.Expression.Reference.getDefaultInstance();
}
}
/**
* .cz.proto.Reference reference = 3;
*/
public Builder setReference(cz.proto.Expression.Reference value) {
if (referenceBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
value_ = value;
onChanged();
} else {
referenceBuilder_.setMessage(value);
}
valueCase_ = 3;
return this;
}
/**
* .cz.proto.Reference reference = 3;
*/
public Builder setReference(
cz.proto.Expression.Reference.Builder builderForValue) {
if (referenceBuilder_ == null) {
value_ = builderForValue.build();
onChanged();
} else {
referenceBuilder_.setMessage(builderForValue.build());
}
valueCase_ = 3;
return this;
}
/**
* .cz.proto.Reference reference = 3;
*/
public Builder mergeReference(cz.proto.Expression.Reference value) {
if (referenceBuilder_ == null) {
if (valueCase_ == 3 &&
value_ != cz.proto.Expression.Reference.getDefaultInstance()) {
value_ = cz.proto.Expression.Reference.newBuilder((cz.proto.Expression.Reference) value_)
.mergeFrom(value).buildPartial();
} else {
value_ = value;
}
onChanged();
} else {
if (valueCase_ == 3) {
referenceBuilder_.mergeFrom(value);
}
referenceBuilder_.setMessage(value);
}
valueCase_ = 3;
return this;
}
/**
* .cz.proto.Reference reference = 3;
*/
public Builder clearReference() {
if (referenceBuilder_ == null) {
if (valueCase_ == 3) {
valueCase_ = 0;
value_ = null;
onChanged();
}
} else {
if (valueCase_ == 3) {
valueCase_ = 0;
value_ = null;
}
referenceBuilder_.clear();
}
return this;
}
/**
* .cz.proto.Reference reference = 3;
*/
public cz.proto.Expression.Reference.Builder getReferenceBuilder() {
return getReferenceFieldBuilder().getBuilder();
}
/**
* .cz.proto.Reference reference = 3;
*/
@java.lang.Override
public cz.proto.Expression.ReferenceOrBuilder getReferenceOrBuilder() {
if ((valueCase_ == 3) && (referenceBuilder_ != null)) {
return referenceBuilder_.getMessageOrBuilder();
} else {
if (valueCase_ == 3) {
return (cz.proto.Expression.Reference) value_;
}
return cz.proto.Expression.Reference.getDefaultInstance();
}
}
/**
* .cz.proto.Reference reference = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.Reference, cz.proto.Expression.Reference.Builder, cz.proto.Expression.ReferenceOrBuilder>
getReferenceFieldBuilder() {
if (referenceBuilder_ == null) {
if (!(valueCase_ == 3)) {
value_ = cz.proto.Expression.Reference.getDefaultInstance();
}
referenceBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.Reference, cz.proto.Expression.Reference.Builder, cz.proto.Expression.ReferenceOrBuilder>(
(cz.proto.Expression.Reference) value_,
getParentForChildren(),
isClean());
value_ = null;
}
valueCase_ = 3;
onChanged();;
return referenceBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.ScalarFunction, cz.proto.Expression.ScalarFunction.Builder, cz.proto.Expression.ScalarFunctionOrBuilder> functionBuilder_;
/**
* .cz.proto.ScalarFunction function = 5;
* @return Whether the function field is set.
*/
@java.lang.Override
public boolean hasFunction() {
return valueCase_ == 5;
}
/**
* .cz.proto.ScalarFunction function = 5;
* @return The function.
*/
@java.lang.Override
public cz.proto.Expression.ScalarFunction getFunction() {
if (functionBuilder_ == null) {
if (valueCase_ == 5) {
return (cz.proto.Expression.ScalarFunction) value_;
}
return cz.proto.Expression.ScalarFunction.getDefaultInstance();
} else {
if (valueCase_ == 5) {
return functionBuilder_.getMessage();
}
return cz.proto.Expression.ScalarFunction.getDefaultInstance();
}
}
/**
* .cz.proto.ScalarFunction function = 5;
*/
public Builder setFunction(cz.proto.Expression.ScalarFunction value) {
if (functionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
value_ = value;
onChanged();
} else {
functionBuilder_.setMessage(value);
}
valueCase_ = 5;
return this;
}
/**
* .cz.proto.ScalarFunction function = 5;
*/
public Builder setFunction(
cz.proto.Expression.ScalarFunction.Builder builderForValue) {
if (functionBuilder_ == null) {
value_ = builderForValue.build();
onChanged();
} else {
functionBuilder_.setMessage(builderForValue.build());
}
valueCase_ = 5;
return this;
}
/**
* .cz.proto.ScalarFunction function = 5;
*/
public Builder mergeFunction(cz.proto.Expression.ScalarFunction value) {
if (functionBuilder_ == null) {
if (valueCase_ == 5 &&
value_ != cz.proto.Expression.ScalarFunction.getDefaultInstance()) {
value_ = cz.proto.Expression.ScalarFunction.newBuilder((cz.proto.Expression.ScalarFunction) value_)
.mergeFrom(value).buildPartial();
} else {
value_ = value;
}
onChanged();
} else {
if (valueCase_ == 5) {
functionBuilder_.mergeFrom(value);
}
functionBuilder_.setMessage(value);
}
valueCase_ = 5;
return this;
}
/**
* .cz.proto.ScalarFunction function = 5;
*/
public Builder clearFunction() {
if (functionBuilder_ == null) {
if (valueCase_ == 5) {
valueCase_ = 0;
value_ = null;
onChanged();
}
} else {
if (valueCase_ == 5) {
valueCase_ = 0;
value_ = null;
}
functionBuilder_.clear();
}
return this;
}
/**
* .cz.proto.ScalarFunction function = 5;
*/
public cz.proto.Expression.ScalarFunction.Builder getFunctionBuilder() {
return getFunctionFieldBuilder().getBuilder();
}
/**
* .cz.proto.ScalarFunction function = 5;
*/
@java.lang.Override
public cz.proto.Expression.ScalarFunctionOrBuilder getFunctionOrBuilder() {
if ((valueCase_ == 5) && (functionBuilder_ != null)) {
return functionBuilder_.getMessageOrBuilder();
} else {
if (valueCase_ == 5) {
return (cz.proto.Expression.ScalarFunction) value_;
}
return cz.proto.Expression.ScalarFunction.getDefaultInstance();
}
}
/**
* .cz.proto.ScalarFunction function = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.ScalarFunction, cz.proto.Expression.ScalarFunction.Builder, cz.proto.Expression.ScalarFunctionOrBuilder>
getFunctionFieldBuilder() {
if (functionBuilder_ == null) {
if (!(valueCase_ == 5)) {
value_ = cz.proto.Expression.ScalarFunction.getDefaultInstance();
}
functionBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.ScalarFunction, cz.proto.Expression.ScalarFunction.Builder, cz.proto.Expression.ScalarFunctionOrBuilder>(
(cz.proto.Expression.ScalarFunction) value_,
getParentForChildren(),
isClean());
value_ = null;
}
valueCase_ = 5;
onChanged();;
return functionBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.LambdaFunction, cz.proto.Expression.LambdaFunction.Builder, cz.proto.Expression.LambdaFunctionOrBuilder> lambdaBuilder_;
/**
* .cz.proto.LambdaFunction lambda = 6;
* @return Whether the lambda field is set.
*/
@java.lang.Override
public boolean hasLambda() {
return valueCase_ == 6;
}
/**
* .cz.proto.LambdaFunction lambda = 6;
* @return The lambda.
*/
@java.lang.Override
public cz.proto.Expression.LambdaFunction getLambda() {
if (lambdaBuilder_ == null) {
if (valueCase_ == 6) {
return (cz.proto.Expression.LambdaFunction) value_;
}
return cz.proto.Expression.LambdaFunction.getDefaultInstance();
} else {
if (valueCase_ == 6) {
return lambdaBuilder_.getMessage();
}
return cz.proto.Expression.LambdaFunction.getDefaultInstance();
}
}
/**
* .cz.proto.LambdaFunction lambda = 6;
*/
public Builder setLambda(cz.proto.Expression.LambdaFunction value) {
if (lambdaBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
value_ = value;
onChanged();
} else {
lambdaBuilder_.setMessage(value);
}
valueCase_ = 6;
return this;
}
/**
* .cz.proto.LambdaFunction lambda = 6;
*/
public Builder setLambda(
cz.proto.Expression.LambdaFunction.Builder builderForValue) {
if (lambdaBuilder_ == null) {
value_ = builderForValue.build();
onChanged();
} else {
lambdaBuilder_.setMessage(builderForValue.build());
}
valueCase_ = 6;
return this;
}
/**
* .cz.proto.LambdaFunction lambda = 6;
*/
public Builder mergeLambda(cz.proto.Expression.LambdaFunction value) {
if (lambdaBuilder_ == null) {
if (valueCase_ == 6 &&
value_ != cz.proto.Expression.LambdaFunction.getDefaultInstance()) {
value_ = cz.proto.Expression.LambdaFunction.newBuilder((cz.proto.Expression.LambdaFunction) value_)
.mergeFrom(value).buildPartial();
} else {
value_ = value;
}
onChanged();
} else {
if (valueCase_ == 6) {
lambdaBuilder_.mergeFrom(value);
}
lambdaBuilder_.setMessage(value);
}
valueCase_ = 6;
return this;
}
/**
* .cz.proto.LambdaFunction lambda = 6;
*/
public Builder clearLambda() {
if (lambdaBuilder_ == null) {
if (valueCase_ == 6) {
valueCase_ = 0;
value_ = null;
onChanged();
}
} else {
if (valueCase_ == 6) {
valueCase_ = 0;
value_ = null;
}
lambdaBuilder_.clear();
}
return this;
}
/**
* .cz.proto.LambdaFunction lambda = 6;
*/
public cz.proto.Expression.LambdaFunction.Builder getLambdaBuilder() {
return getLambdaFieldBuilder().getBuilder();
}
/**
* .cz.proto.LambdaFunction lambda = 6;
*/
@java.lang.Override
public cz.proto.Expression.LambdaFunctionOrBuilder getLambdaOrBuilder() {
if ((valueCase_ == 6) && (lambdaBuilder_ != null)) {
return lambdaBuilder_.getMessageOrBuilder();
} else {
if (valueCase_ == 6) {
return (cz.proto.Expression.LambdaFunction) value_;
}
return cz.proto.Expression.LambdaFunction.getDefaultInstance();
}
}
/**
* .cz.proto.LambdaFunction lambda = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.LambdaFunction, cz.proto.Expression.LambdaFunction.Builder, cz.proto.Expression.LambdaFunctionOrBuilder>
getLambdaFieldBuilder() {
if (lambdaBuilder_ == null) {
if (!(valueCase_ == 6)) {
value_ = cz.proto.Expression.LambdaFunction.getDefaultInstance();
}
lambdaBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.LambdaFunction, cz.proto.Expression.LambdaFunction.Builder, cz.proto.Expression.LambdaFunctionOrBuilder>(
(cz.proto.Expression.LambdaFunction) value_,
getParentForChildren(),
isClean());
value_ = null;
}
valueCase_ = 6;
onChanged();;
return lambdaBuilder_;
}
private cz.proto.Expression.ParseTreeInfo pt_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.ParseTreeInfo, cz.proto.Expression.ParseTreeInfo.Builder, cz.proto.Expression.ParseTreeInfoOrBuilder> ptBuilder_;
/**
* .cz.proto.ParseTreeInfo pt = 10;
* @return Whether the pt field is set.
*/
public boolean hasPt() {
return ptBuilder_ != null || pt_ != null;
}
/**
* .cz.proto.ParseTreeInfo pt = 10;
* @return The pt.
*/
public cz.proto.Expression.ParseTreeInfo getPt() {
if (ptBuilder_ == null) {
return pt_ == null ? cz.proto.Expression.ParseTreeInfo.getDefaultInstance() : pt_;
} else {
return ptBuilder_.getMessage();
}
}
/**
* .cz.proto.ParseTreeInfo pt = 10;
*/
public Builder setPt(cz.proto.Expression.ParseTreeInfo value) {
if (ptBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
pt_ = value;
onChanged();
} else {
ptBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ParseTreeInfo pt = 10;
*/
public Builder setPt(
cz.proto.Expression.ParseTreeInfo.Builder builderForValue) {
if (ptBuilder_ == null) {
pt_ = builderForValue.build();
onChanged();
} else {
ptBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ParseTreeInfo pt = 10;
*/
public Builder mergePt(cz.proto.Expression.ParseTreeInfo value) {
if (ptBuilder_ == null) {
if (pt_ != null) {
pt_ =
cz.proto.Expression.ParseTreeInfo.newBuilder(pt_).mergeFrom(value).buildPartial();
} else {
pt_ = value;
}
onChanged();
} else {
ptBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ParseTreeInfo pt = 10;
*/
public Builder clearPt() {
if (ptBuilder_ == null) {
pt_ = null;
onChanged();
} else {
pt_ = null;
ptBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ParseTreeInfo pt = 10;
*/
public cz.proto.Expression.ParseTreeInfo.Builder getPtBuilder() {
onChanged();
return getPtFieldBuilder().getBuilder();
}
/**
* .cz.proto.ParseTreeInfo pt = 10;
*/
public cz.proto.Expression.ParseTreeInfoOrBuilder getPtOrBuilder() {
if (ptBuilder_ != null) {
return ptBuilder_.getMessageOrBuilder();
} else {
return pt_ == null ?
cz.proto.Expression.ParseTreeInfo.getDefaultInstance() : pt_;
}
}
/**
* .cz.proto.ParseTreeInfo pt = 10;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.ParseTreeInfo, cz.proto.Expression.ParseTreeInfo.Builder, cz.proto.Expression.ParseTreeInfoOrBuilder>
getPtFieldBuilder() {
if (ptBuilder_ == null) {
ptBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.ParseTreeInfo, cz.proto.Expression.ParseTreeInfo.Builder, cz.proto.Expression.ParseTreeInfoOrBuilder>(
getPt(),
getParentForChildren(),
isClean());
pt_ = null;
}
return ptBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ScalarExpression)
}
// @@protoc_insertion_point(class_scope:cz.proto.ScalarExpression)
private static final cz.proto.Expression.ScalarExpression DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.Expression.ScalarExpression();
}
public static cz.proto.Expression.ScalarExpression getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ScalarExpression parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ScalarExpression(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.Expression.ScalarExpression getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cz_proto_ParseTreeInfo_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cz_proto_ParseTreeInfo_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cz_proto_ParseTreeInfo_LocationInfo_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cz_proto_ParseTreeInfo_LocationInfo_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cz_proto_IntervalDayTime_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cz_proto_IntervalDayTime_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cz_proto_ArrayValue_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cz_proto_ArrayValue_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cz_proto_MapValue_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cz_proto_MapValue_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cz_proto_StructValue_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cz_proto_StructValue_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cz_proto_BitmapValue_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cz_proto_BitmapValue_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cz_proto_Constant_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cz_proto_Constant_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cz_proto_SubFieldPruning_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cz_proto_SubFieldPruning_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cz_proto_SubFieldsPruning_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cz_proto_SubFieldsPruning_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cz_proto_Reference_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cz_proto_Reference_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cz_proto_Udf_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cz_proto_Udf_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cz_proto_ScalarFunction_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cz_proto_ScalarFunction_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cz_proto_VariableDef_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cz_proto_VariableDef_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cz_proto_LambdaFunction_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cz_proto_LambdaFunction_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cz_proto_ScalarExpression_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cz_proto_ScalarExpression_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\020expression.proto\022\010cz.proto\032\017data_type." +
"proto\032\016property.proto\"\257\001\n\rParseTreeInfo\022" +
"3\n\005start\030\001 \001(\0132$.cz.proto.ParseTreeInfo." +
"LocationInfo\0221\n\003end\030\002 \001(\0132$.cz.proto.Par" +
"seTreeInfo.LocationInfo\0326\n\014LocationInfo\022" +
"\014\n\004line\030\001 \001(\r\022\013\n\003col\030\002 \001(\r\022\013\n\003pos\030\003 \001(\r\"" +
"1\n\017IntervalDayTime\022\017\n\007seconds\030\001 \001(\003\022\r\n\005n" +
"anos\030\002 \001(\005\"2\n\nArrayValue\022$\n\010elements\030\001 \003" +
"(\0132\022.cz.proto.Constant\"P\n\010MapValue\022 \n\004ke" +
"ys\030\001 \003(\0132\022.cz.proto.Constant\022\"\n\006values\030\002" +
" \003(\0132\022.cz.proto.Constant\"1\n\013StructValue\022" +
"\"\n\006fields\030\001 \003(\0132\022.cz.proto.Constant\"\034\n\013B" +
"itmapValue\022\r\n\005value\030\001 \001(\014\"\217\004\n\010Constant\022\016" +
"\n\004null\030\001 \001(\010H\000\022\021\n\007tinyint\030\002 \001(\005H\000\022\022\n\010sma" +
"llInt\030\003 \001(\005H\000\022\r\n\003int\030\004 \001(\005H\000\022\020\n\006bigint\030\005" +
" \001(\003H\000\022\017\n\005float\030\006 \001(\002H\000\022\020\n\006double\030\007 \001(\001H" +
"\000\022\021\n\007decimal\030\010 \001(\tH\000\022\021\n\007boolean\030\t \001(\010H\000\022" +
"\016\n\004char\030\n \001(\tH\000\022\021\n\007varchar\030\013 \001(\tH\000\022\020\n\006st" +
"ring\030\014 \001(\tH\000\022\020\n\006binary\030\r \001(\014H\000\022\016\n\004date\030\016" +
" \001(\005H\000\022\023\n\ttimestamp\030\017 \001(\003H\000\022\033\n\021IntervalY" +
"earMonth\030\020 \001(\003H\000\0224\n\017IntervalDayTime\030\021 \001(" +
"\0132\031.cz.proto.IntervalDayTimeH\000\022%\n\005array\030" +
"d \001(\0132\024.cz.proto.ArrayValueH\000\022!\n\003map\030e \001" +
"(\0132\022.cz.proto.MapValueH\000\022\'\n\006struct\030f \001(\013" +
"2\025.cz.proto.StructValueH\000\022\'\n\006bitmap\030g \001(" +
"\0132\025.cz.proto.BitmapValueH\000B\007\n\005value\"`\n\017S" +
"ubFieldPruning\022\036\n\002id\030\001 \001(\0132\022.cz.proto.Co" +
"nstant\022-\n\tsubfields\030\002 \001(\0132\032.cz.proto.Sub" +
"FieldsPruning\"S\n\020SubFieldsPruning\022)\n\006fie" +
"lds\030\001 \003(\0132\031.cz.proto.SubFieldPruning\022\024\n\014" +
"reserve_size\030\002 \001(\010\"m\n\tReference\022\n\n\002id\030\001 " +
"\001(\004\022\r\n\005local\030\002 \001(\010\022\014\n\004from\030\003 \001(\t\022\014\n\004name" +
"\030\004 \001(\t\022)\n\010ref_type\030\005 \001(\0162\027.cz.proto.Refe" +
"renceType\"8\n\003Udf\022\022\n\ninstanceId\030\001 \001(\003\022\014\n\004" +
"path\030\002 \003(\t\022\017\n\007creator\030\003 \001(\003\"\203\002\n\016ScalarFu" +
"nction\022\014\n\004from\030\001 \001(\t\022\014\n\004name\030\002 \001(\t\022\017\n\007bu" +
"iltIn\030\003 \001(\010\022-\n\targuments\030\004 \003(\0132\032.cz.prot" +
"o.ScalarExpression\022(\n\nproperties\030\005 \001(\0132\024" +
".cz.proto.Properties\022\020\n\010execDesc\030\006 \001(\t\0220" +
"\n\022functionProperties\030\007 \001(\0132\024.cz.proto.Pr" +
"operties\022\034\n\003udf\030\n \001(\0132\r.cz.proto.UdfH\000B\t" +
"\n\007derived\";\n\013VariableDef\022 \n\004type\030\001 \001(\0132\022" +
".cz.proto.DataType\022\n\n\002id\030\002 \001(\004\"a\n\016Lambda" +
"Function\022%\n\006params\030\001 \003(\0132\025.cz.proto.Vari" +
"ableDef\022(\n\004impl\030\002 \001(\0132\032.cz.proto.ScalarE" +
"xpression\"\216\002\n\020ScalarExpression\022 \n\004type\030\001" +
" \001(\0132\022.cz.proto.DataType\022&\n\010constant\030\002 \001" +
"(\0132\022.cz.proto.ConstantH\000\022(\n\treference\030\003 " +
"\001(\0132\023.cz.proto.ReferenceH\000\022,\n\010function\030\005" +
" \001(\0132\030.cz.proto.ScalarFunctionH\000\022*\n\006lamb" +
"da\030\006 \001(\0132\030.cz.proto.LambdaFunctionH\000\022#\n\002" +
"pt\030\n \001(\0132\027.cz.proto.ParseTreeInfoB\007\n\005val" +
"ue*W\n\rReferenceType\022\021\n\rLOGICAL_FIELD\020\000\022\r" +
"\n\tREF_LOCAL\020\001\022\022\n\016PHYSICAL_FIELD\020\002\022\020\n\014REF" +
"_VARIABLE\020\003b\006proto3"
};
descriptor = com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
cz.proto.DataTypeOuterClass.getDescriptor(),
cz.proto.PropertyOuterClass.getDescriptor(),
});
internal_static_cz_proto_ParseTreeInfo_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_cz_proto_ParseTreeInfo_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cz_proto_ParseTreeInfo_descriptor,
new java.lang.String[] { "Start", "End", });
internal_static_cz_proto_ParseTreeInfo_LocationInfo_descriptor =
internal_static_cz_proto_ParseTreeInfo_descriptor.getNestedTypes().get(0);
internal_static_cz_proto_ParseTreeInfo_LocationInfo_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cz_proto_ParseTreeInfo_LocationInfo_descriptor,
new java.lang.String[] { "Line", "Col", "Pos", });
internal_static_cz_proto_IntervalDayTime_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_cz_proto_IntervalDayTime_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cz_proto_IntervalDayTime_descriptor,
new java.lang.String[] { "Seconds", "Nanos", });
internal_static_cz_proto_ArrayValue_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_cz_proto_ArrayValue_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cz_proto_ArrayValue_descriptor,
new java.lang.String[] { "Elements", });
internal_static_cz_proto_MapValue_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_cz_proto_MapValue_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cz_proto_MapValue_descriptor,
new java.lang.String[] { "Keys", "Values", });
internal_static_cz_proto_StructValue_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_cz_proto_StructValue_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cz_proto_StructValue_descriptor,
new java.lang.String[] { "Fields", });
internal_static_cz_proto_BitmapValue_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_cz_proto_BitmapValue_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cz_proto_BitmapValue_descriptor,
new java.lang.String[] { "Value", });
internal_static_cz_proto_Constant_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_cz_proto_Constant_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cz_proto_Constant_descriptor,
new java.lang.String[] { "Null", "Tinyint", "SmallInt", "Int", "Bigint", "Float", "Double", "Decimal", "Boolean", "Char", "Varchar", "String", "Binary", "Date", "Timestamp", "IntervalYearMonth", "IntervalDayTime", "Array", "Map", "Struct", "Bitmap", "Value", });
internal_static_cz_proto_SubFieldPruning_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_cz_proto_SubFieldPruning_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cz_proto_SubFieldPruning_descriptor,
new java.lang.String[] { "Id", "Subfields", });
internal_static_cz_proto_SubFieldsPruning_descriptor =
getDescriptor().getMessageTypes().get(8);
internal_static_cz_proto_SubFieldsPruning_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cz_proto_SubFieldsPruning_descriptor,
new java.lang.String[] { "Fields", "ReserveSize", });
internal_static_cz_proto_Reference_descriptor =
getDescriptor().getMessageTypes().get(9);
internal_static_cz_proto_Reference_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cz_proto_Reference_descriptor,
new java.lang.String[] { "Id", "Local", "From", "Name", "RefType", });
internal_static_cz_proto_Udf_descriptor =
getDescriptor().getMessageTypes().get(10);
internal_static_cz_proto_Udf_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cz_proto_Udf_descriptor,
new java.lang.String[] { "InstanceId", "Path", "Creator", });
internal_static_cz_proto_ScalarFunction_descriptor =
getDescriptor().getMessageTypes().get(11);
internal_static_cz_proto_ScalarFunction_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cz_proto_ScalarFunction_descriptor,
new java.lang.String[] { "From", "Name", "BuiltIn", "Arguments", "Properties", "ExecDesc", "FunctionProperties", "Udf", "Derived", });
internal_static_cz_proto_VariableDef_descriptor =
getDescriptor().getMessageTypes().get(12);
internal_static_cz_proto_VariableDef_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cz_proto_VariableDef_descriptor,
new java.lang.String[] { "Type", "Id", });
internal_static_cz_proto_LambdaFunction_descriptor =
getDescriptor().getMessageTypes().get(13);
internal_static_cz_proto_LambdaFunction_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cz_proto_LambdaFunction_descriptor,
new java.lang.String[] { "Params", "Impl", });
internal_static_cz_proto_ScalarExpression_descriptor =
getDescriptor().getMessageTypes().get(14);
internal_static_cz_proto_ScalarExpression_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cz_proto_ScalarExpression_descriptor,
new java.lang.String[] { "Type", "Constant", "Reference", "Function", "Lambda", "Pt", "Value", });
cz.proto.DataTypeOuterClass.getDescriptor();
cz.proto.PropertyOuterClass.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy