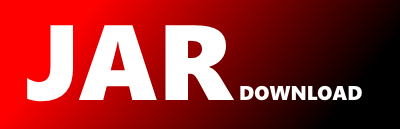
cz.proto.FieldStatsValue Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickzetta-java Show documentation
Show all versions of clickzetta-java Show documentation
The java SDK for clickzetta's Lakehouse
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: statistics.proto
package cz.proto;
/**
* Protobuf type {@code cz.proto.FieldStatsValue}
*/
public final class FieldStatsValue extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.FieldStatsValue)
FieldStatsValueOrBuilder {
private static final long serialVersionUID = 0L;
// Use FieldStatsValue.newBuilder() to construct.
private FieldStatsValue(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private FieldStatsValue() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new FieldStatsValue();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private FieldStatsValue(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
value_ = input.readInt64();
valueCase_ = 1;
break;
}
case 16: {
value_ = input.readInt64();
valueCase_ = 2;
break;
}
case 24: {
value_ = input.readInt64();
valueCase_ = 3;
break;
}
case 34: {
cz.proto.Expression.Constant.Builder subBuilder = null;
if (valueCase_ == 4) {
subBuilder = ((cz.proto.Expression.Constant) value_).toBuilder();
}
value_ =
input.readMessage(cz.proto.Expression.Constant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((cz.proto.Expression.Constant) value_);
value_ = subBuilder.buildPartial();
}
valueCase_ = 4;
break;
}
case 42: {
cz.proto.Expression.Constant.Builder subBuilder = null;
if (valueCase_ == 5) {
subBuilder = ((cz.proto.Expression.Constant) value_).toBuilder();
}
value_ =
input.readMessage(cz.proto.Expression.Constant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((cz.proto.Expression.Constant) value_);
value_ = subBuilder.buildPartial();
}
valueCase_ = 5;
break;
}
case 49: {
value_ = input.readDouble();
valueCase_ = 6;
break;
}
case 56: {
value_ = input.readInt64();
valueCase_ = 7;
break;
}
case 64: {
value_ = input.readInt64();
valueCase_ = 8;
break;
}
case 72: {
value_ = input.readInt64();
valueCase_ = 9;
break;
}
case 82: {
cz.proto.TopK.Builder subBuilder = null;
if (valueCase_ == 10) {
subBuilder = ((cz.proto.TopK) value_).toBuilder();
}
value_ =
input.readMessage(cz.proto.TopK.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((cz.proto.TopK) value_);
value_ = subBuilder.buildPartial();
}
valueCase_ = 10;
break;
}
case 90: {
cz.proto.Histogram.Builder subBuilder = null;
if (valueCase_ == 11) {
subBuilder = ((cz.proto.Histogram) value_).toBuilder();
}
value_ =
input.readMessage(cz.proto.Histogram.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((cz.proto.Histogram) value_);
value_ = subBuilder.buildPartial();
}
valueCase_ = 11;
break;
}
case 96: {
value_ = input.readInt64();
valueCase_ = 12;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.Statistics.internal_static_cz_proto_FieldStatsValue_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.Statistics.internal_static_cz_proto_FieldStatsValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.FieldStatsValue.class, cz.proto.FieldStatsValue.Builder.class);
}
private int valueCase_ = 0;
private java.lang.Object value_;
public enum ValueCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
NAN_COUNT(1),
VALUE_COUNT(2),
NULL_COUNT(3),
LOWER_BOUNDS(4),
UPPER_BOUNDS(5),
AVG_SIZE(6),
MAX_SIZE(7),
COMPRESSED_SIZE(8),
DISTINCT_NUMBER(9),
TOP_K(10),
HISTOGRAM(11),
RAW_SIZE_IN_BYTES(12),
VALUE_NOT_SET(0);
private final int value;
private ValueCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ValueCase valueOf(int value) {
return forNumber(value);
}
public static ValueCase forNumber(int value) {
switch (value) {
case 1: return NAN_COUNT;
case 2: return VALUE_COUNT;
case 3: return NULL_COUNT;
case 4: return LOWER_BOUNDS;
case 5: return UPPER_BOUNDS;
case 6: return AVG_SIZE;
case 7: return MAX_SIZE;
case 8: return COMPRESSED_SIZE;
case 9: return DISTINCT_NUMBER;
case 10: return TOP_K;
case 11: return HISTOGRAM;
case 12: return RAW_SIZE_IN_BYTES;
case 0: return VALUE_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public ValueCase
getValueCase() {
return ValueCase.forNumber(
valueCase_);
}
public static final int NAN_COUNT_FIELD_NUMBER = 1;
/**
* int64 nan_count = 1;
* @return Whether the nanCount field is set.
*/
@java.lang.Override
public boolean hasNanCount() {
return valueCase_ == 1;
}
/**
* int64 nan_count = 1;
* @return The nanCount.
*/
@java.lang.Override
public long getNanCount() {
if (valueCase_ == 1) {
return (java.lang.Long) value_;
}
return 0L;
}
public static final int VALUE_COUNT_FIELD_NUMBER = 2;
/**
*
* For storage, value_count not includes null data. And for complex type,
* only non-null leaf will be counted.
*
*
* int64 value_count = 2;
* @return Whether the valueCount field is set.
*/
@java.lang.Override
public boolean hasValueCount() {
return valueCase_ == 2;
}
/**
*
* For storage, value_count not includes null data. And for complex type,
* only non-null leaf will be counted.
*
*
* int64 value_count = 2;
* @return The valueCount.
*/
@java.lang.Override
public long getValueCount() {
if (valueCase_ == 2) {
return (java.lang.Long) value_;
}
return 0L;
}
public static final int NULL_COUNT_FIELD_NUMBER = 3;
/**
* int64 null_count = 3;
* @return Whether the nullCount field is set.
*/
@java.lang.Override
public boolean hasNullCount() {
return valueCase_ == 3;
}
/**
* int64 null_count = 3;
* @return The nullCount.
*/
@java.lang.Override
public long getNullCount() {
if (valueCase_ == 3) {
return (java.lang.Long) value_;
}
return 0L;
}
public static final int LOWER_BOUNDS_FIELD_NUMBER = 4;
/**
* .cz.proto.Constant lower_bounds = 4;
* @return Whether the lowerBounds field is set.
*/
@java.lang.Override
public boolean hasLowerBounds() {
return valueCase_ == 4;
}
/**
* .cz.proto.Constant lower_bounds = 4;
* @return The lowerBounds.
*/
@java.lang.Override
public cz.proto.Expression.Constant getLowerBounds() {
if (valueCase_ == 4) {
return (cz.proto.Expression.Constant) value_;
}
return cz.proto.Expression.Constant.getDefaultInstance();
}
/**
* .cz.proto.Constant lower_bounds = 4;
*/
@java.lang.Override
public cz.proto.Expression.ConstantOrBuilder getLowerBoundsOrBuilder() {
if (valueCase_ == 4) {
return (cz.proto.Expression.Constant) value_;
}
return cz.proto.Expression.Constant.getDefaultInstance();
}
public static final int UPPER_BOUNDS_FIELD_NUMBER = 5;
/**
* .cz.proto.Constant upper_bounds = 5;
* @return Whether the upperBounds field is set.
*/
@java.lang.Override
public boolean hasUpperBounds() {
return valueCase_ == 5;
}
/**
* .cz.proto.Constant upper_bounds = 5;
* @return The upperBounds.
*/
@java.lang.Override
public cz.proto.Expression.Constant getUpperBounds() {
if (valueCase_ == 5) {
return (cz.proto.Expression.Constant) value_;
}
return cz.proto.Expression.Constant.getDefaultInstance();
}
/**
* .cz.proto.Constant upper_bounds = 5;
*/
@java.lang.Override
public cz.proto.Expression.ConstantOrBuilder getUpperBoundsOrBuilder() {
if (valueCase_ == 5) {
return (cz.proto.Expression.Constant) value_;
}
return cz.proto.Expression.Constant.getDefaultInstance();
}
public static final int AVG_SIZE_FIELD_NUMBER = 6;
/**
* double avg_size = 6;
* @return Whether the avgSize field is set.
*/
@java.lang.Override
public boolean hasAvgSize() {
return valueCase_ == 6;
}
/**
* double avg_size = 6;
* @return The avgSize.
*/
@java.lang.Override
public double getAvgSize() {
if (valueCase_ == 6) {
return (java.lang.Double) value_;
}
return 0D;
}
public static final int MAX_SIZE_FIELD_NUMBER = 7;
/**
* int64 max_size = 7;
* @return Whether the maxSize field is set.
*/
@java.lang.Override
public boolean hasMaxSize() {
return valueCase_ == 7;
}
/**
* int64 max_size = 7;
* @return The maxSize.
*/
@java.lang.Override
public long getMaxSize() {
if (valueCase_ == 7) {
return (java.lang.Long) value_;
}
return 0L;
}
public static final int COMPRESSED_SIZE_FIELD_NUMBER = 8;
/**
* int64 compressed_size = 8;
* @return Whether the compressedSize field is set.
*/
@java.lang.Override
public boolean hasCompressedSize() {
return valueCase_ == 8;
}
/**
* int64 compressed_size = 8;
* @return The compressedSize.
*/
@java.lang.Override
public long getCompressedSize() {
if (valueCase_ == 8) {
return (java.lang.Long) value_;
}
return 0L;
}
public static final int DISTINCT_NUMBER_FIELD_NUMBER = 9;
/**
* int64 distinct_number = 9;
* @return Whether the distinctNumber field is set.
*/
@java.lang.Override
public boolean hasDistinctNumber() {
return valueCase_ == 9;
}
/**
* int64 distinct_number = 9;
* @return The distinctNumber.
*/
@java.lang.Override
public long getDistinctNumber() {
if (valueCase_ == 9) {
return (java.lang.Long) value_;
}
return 0L;
}
public static final int TOP_K_FIELD_NUMBER = 10;
/**
* .cz.proto.TopK top_k = 10;
* @return Whether the topK field is set.
*/
@java.lang.Override
public boolean hasTopK() {
return valueCase_ == 10;
}
/**
* .cz.proto.TopK top_k = 10;
* @return The topK.
*/
@java.lang.Override
public cz.proto.TopK getTopK() {
if (valueCase_ == 10) {
return (cz.proto.TopK) value_;
}
return cz.proto.TopK.getDefaultInstance();
}
/**
* .cz.proto.TopK top_k = 10;
*/
@java.lang.Override
public cz.proto.TopKOrBuilder getTopKOrBuilder() {
if (valueCase_ == 10) {
return (cz.proto.TopK) value_;
}
return cz.proto.TopK.getDefaultInstance();
}
public static final int HISTOGRAM_FIELD_NUMBER = 11;
/**
* .cz.proto.Histogram histogram = 11;
* @return Whether the histogram field is set.
*/
@java.lang.Override
public boolean hasHistogram() {
return valueCase_ == 11;
}
/**
* .cz.proto.Histogram histogram = 11;
* @return The histogram.
*/
@java.lang.Override
public cz.proto.Histogram getHistogram() {
if (valueCase_ == 11) {
return (cz.proto.Histogram) value_;
}
return cz.proto.Histogram.getDefaultInstance();
}
/**
* .cz.proto.Histogram histogram = 11;
*/
@java.lang.Override
public cz.proto.HistogramOrBuilder getHistogramOrBuilder() {
if (valueCase_ == 11) {
return (cz.proto.Histogram) value_;
}
return cz.proto.Histogram.getDefaultInstance();
}
public static final int RAW_SIZE_IN_BYTES_FIELD_NUMBER = 12;
/**
*
* For storage, Size in bytes of columnar data before any encoding or compression.
* * For fix-sized type, it's always `value_count * parquet::GetTypeByteSize(T)`,
* value_count includes null data.
* * For variable-length type, it's `value_count * parquet::GetTypeByteSize(T)
* + variable-length`.
* For complex type, leaf will be counted, and non-leaf types would be the
* sum of all leaf-type raw size.
*
*
* int64 raw_size_in_bytes = 12;
* @return Whether the rawSizeInBytes field is set.
*/
@java.lang.Override
public boolean hasRawSizeInBytes() {
return valueCase_ == 12;
}
/**
*
* For storage, Size in bytes of columnar data before any encoding or compression.
* * For fix-sized type, it's always `value_count * parquet::GetTypeByteSize(T)`,
* value_count includes null data.
* * For variable-length type, it's `value_count * parquet::GetTypeByteSize(T)
* + variable-length`.
* For complex type, leaf will be counted, and non-leaf types would be the
* sum of all leaf-type raw size.
*
*
* int64 raw_size_in_bytes = 12;
* @return The rawSizeInBytes.
*/
@java.lang.Override
public long getRawSizeInBytes() {
if (valueCase_ == 12) {
return (java.lang.Long) value_;
}
return 0L;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (valueCase_ == 1) {
output.writeInt64(
1, (long)((java.lang.Long) value_));
}
if (valueCase_ == 2) {
output.writeInt64(
2, (long)((java.lang.Long) value_));
}
if (valueCase_ == 3) {
output.writeInt64(
3, (long)((java.lang.Long) value_));
}
if (valueCase_ == 4) {
output.writeMessage(4, (cz.proto.Expression.Constant) value_);
}
if (valueCase_ == 5) {
output.writeMessage(5, (cz.proto.Expression.Constant) value_);
}
if (valueCase_ == 6) {
output.writeDouble(
6, (double)((java.lang.Double) value_));
}
if (valueCase_ == 7) {
output.writeInt64(
7, (long)((java.lang.Long) value_));
}
if (valueCase_ == 8) {
output.writeInt64(
8, (long)((java.lang.Long) value_));
}
if (valueCase_ == 9) {
output.writeInt64(
9, (long)((java.lang.Long) value_));
}
if (valueCase_ == 10) {
output.writeMessage(10, (cz.proto.TopK) value_);
}
if (valueCase_ == 11) {
output.writeMessage(11, (cz.proto.Histogram) value_);
}
if (valueCase_ == 12) {
output.writeInt64(
12, (long)((java.lang.Long) value_));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (valueCase_ == 1) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(
1, (long)((java.lang.Long) value_));
}
if (valueCase_ == 2) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(
2, (long)((java.lang.Long) value_));
}
if (valueCase_ == 3) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(
3, (long)((java.lang.Long) value_));
}
if (valueCase_ == 4) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, (cz.proto.Expression.Constant) value_);
}
if (valueCase_ == 5) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, (cz.proto.Expression.Constant) value_);
}
if (valueCase_ == 6) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(
6, (double)((java.lang.Double) value_));
}
if (valueCase_ == 7) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(
7, (long)((java.lang.Long) value_));
}
if (valueCase_ == 8) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(
8, (long)((java.lang.Long) value_));
}
if (valueCase_ == 9) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(
9, (long)((java.lang.Long) value_));
}
if (valueCase_ == 10) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, (cz.proto.TopK) value_);
}
if (valueCase_ == 11) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, (cz.proto.Histogram) value_);
}
if (valueCase_ == 12) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(
12, (long)((java.lang.Long) value_));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.FieldStatsValue)) {
return super.equals(obj);
}
cz.proto.FieldStatsValue other = (cz.proto.FieldStatsValue) obj;
if (!getValueCase().equals(other.getValueCase())) return false;
switch (valueCase_) {
case 1:
if (getNanCount()
!= other.getNanCount()) return false;
break;
case 2:
if (getValueCount()
!= other.getValueCount()) return false;
break;
case 3:
if (getNullCount()
!= other.getNullCount()) return false;
break;
case 4:
if (!getLowerBounds()
.equals(other.getLowerBounds())) return false;
break;
case 5:
if (!getUpperBounds()
.equals(other.getUpperBounds())) return false;
break;
case 6:
if (java.lang.Double.doubleToLongBits(getAvgSize())
!= java.lang.Double.doubleToLongBits(
other.getAvgSize())) return false;
break;
case 7:
if (getMaxSize()
!= other.getMaxSize()) return false;
break;
case 8:
if (getCompressedSize()
!= other.getCompressedSize()) return false;
break;
case 9:
if (getDistinctNumber()
!= other.getDistinctNumber()) return false;
break;
case 10:
if (!getTopK()
.equals(other.getTopK())) return false;
break;
case 11:
if (!getHistogram()
.equals(other.getHistogram())) return false;
break;
case 12:
if (getRawSizeInBytes()
!= other.getRawSizeInBytes()) return false;
break;
case 0:
default:
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
switch (valueCase_) {
case 1:
hash = (37 * hash) + NAN_COUNT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getNanCount());
break;
case 2:
hash = (37 * hash) + VALUE_COUNT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getValueCount());
break;
case 3:
hash = (37 * hash) + NULL_COUNT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getNullCount());
break;
case 4:
hash = (37 * hash) + LOWER_BOUNDS_FIELD_NUMBER;
hash = (53 * hash) + getLowerBounds().hashCode();
break;
case 5:
hash = (37 * hash) + UPPER_BOUNDS_FIELD_NUMBER;
hash = (53 * hash) + getUpperBounds().hashCode();
break;
case 6:
hash = (37 * hash) + AVG_SIZE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getAvgSize()));
break;
case 7:
hash = (37 * hash) + MAX_SIZE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getMaxSize());
break;
case 8:
hash = (37 * hash) + COMPRESSED_SIZE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getCompressedSize());
break;
case 9:
hash = (37 * hash) + DISTINCT_NUMBER_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getDistinctNumber());
break;
case 10:
hash = (37 * hash) + TOP_K_FIELD_NUMBER;
hash = (53 * hash) + getTopK().hashCode();
break;
case 11:
hash = (37 * hash) + HISTOGRAM_FIELD_NUMBER;
hash = (53 * hash) + getHistogram().hashCode();
break;
case 12:
hash = (37 * hash) + RAW_SIZE_IN_BYTES_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getRawSizeInBytes());
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.FieldStatsValue parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.FieldStatsValue parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.FieldStatsValue parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.FieldStatsValue parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.FieldStatsValue parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.FieldStatsValue parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.FieldStatsValue parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.FieldStatsValue parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.FieldStatsValue parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.FieldStatsValue parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.FieldStatsValue parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.FieldStatsValue parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.FieldStatsValue prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.FieldStatsValue}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.FieldStatsValue)
cz.proto.FieldStatsValueOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.Statistics.internal_static_cz_proto_FieldStatsValue_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.Statistics.internal_static_cz_proto_FieldStatsValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.FieldStatsValue.class, cz.proto.FieldStatsValue.Builder.class);
}
// Construct using cz.proto.FieldStatsValue.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
valueCase_ = 0;
value_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.Statistics.internal_static_cz_proto_FieldStatsValue_descriptor;
}
@java.lang.Override
public cz.proto.FieldStatsValue getDefaultInstanceForType() {
return cz.proto.FieldStatsValue.getDefaultInstance();
}
@java.lang.Override
public cz.proto.FieldStatsValue build() {
cz.proto.FieldStatsValue result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.FieldStatsValue buildPartial() {
cz.proto.FieldStatsValue result = new cz.proto.FieldStatsValue(this);
if (valueCase_ == 1) {
result.value_ = value_;
}
if (valueCase_ == 2) {
result.value_ = value_;
}
if (valueCase_ == 3) {
result.value_ = value_;
}
if (valueCase_ == 4) {
if (lowerBoundsBuilder_ == null) {
result.value_ = value_;
} else {
result.value_ = lowerBoundsBuilder_.build();
}
}
if (valueCase_ == 5) {
if (upperBoundsBuilder_ == null) {
result.value_ = value_;
} else {
result.value_ = upperBoundsBuilder_.build();
}
}
if (valueCase_ == 6) {
result.value_ = value_;
}
if (valueCase_ == 7) {
result.value_ = value_;
}
if (valueCase_ == 8) {
result.value_ = value_;
}
if (valueCase_ == 9) {
result.value_ = value_;
}
if (valueCase_ == 10) {
if (topKBuilder_ == null) {
result.value_ = value_;
} else {
result.value_ = topKBuilder_.build();
}
}
if (valueCase_ == 11) {
if (histogramBuilder_ == null) {
result.value_ = value_;
} else {
result.value_ = histogramBuilder_.build();
}
}
if (valueCase_ == 12) {
result.value_ = value_;
}
result.valueCase_ = valueCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.FieldStatsValue) {
return mergeFrom((cz.proto.FieldStatsValue)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.FieldStatsValue other) {
if (other == cz.proto.FieldStatsValue.getDefaultInstance()) return this;
switch (other.getValueCase()) {
case NAN_COUNT: {
setNanCount(other.getNanCount());
break;
}
case VALUE_COUNT: {
setValueCount(other.getValueCount());
break;
}
case NULL_COUNT: {
setNullCount(other.getNullCount());
break;
}
case LOWER_BOUNDS: {
mergeLowerBounds(other.getLowerBounds());
break;
}
case UPPER_BOUNDS: {
mergeUpperBounds(other.getUpperBounds());
break;
}
case AVG_SIZE: {
setAvgSize(other.getAvgSize());
break;
}
case MAX_SIZE: {
setMaxSize(other.getMaxSize());
break;
}
case COMPRESSED_SIZE: {
setCompressedSize(other.getCompressedSize());
break;
}
case DISTINCT_NUMBER: {
setDistinctNumber(other.getDistinctNumber());
break;
}
case TOP_K: {
mergeTopK(other.getTopK());
break;
}
case HISTOGRAM: {
mergeHistogram(other.getHistogram());
break;
}
case RAW_SIZE_IN_BYTES: {
setRawSizeInBytes(other.getRawSizeInBytes());
break;
}
case VALUE_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.FieldStatsValue parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.FieldStatsValue) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int valueCase_ = 0;
private java.lang.Object value_;
public ValueCase
getValueCase() {
return ValueCase.forNumber(
valueCase_);
}
public Builder clearValue() {
valueCase_ = 0;
value_ = null;
onChanged();
return this;
}
/**
* int64 nan_count = 1;
* @return Whether the nanCount field is set.
*/
public boolean hasNanCount() {
return valueCase_ == 1;
}
/**
* int64 nan_count = 1;
* @return The nanCount.
*/
public long getNanCount() {
if (valueCase_ == 1) {
return (java.lang.Long) value_;
}
return 0L;
}
/**
* int64 nan_count = 1;
* @param value The nanCount to set.
* @return This builder for chaining.
*/
public Builder setNanCount(long value) {
valueCase_ = 1;
value_ = value;
onChanged();
return this;
}
/**
* int64 nan_count = 1;
* @return This builder for chaining.
*/
public Builder clearNanCount() {
if (valueCase_ == 1) {
valueCase_ = 0;
value_ = null;
onChanged();
}
return this;
}
/**
*
* For storage, value_count not includes null data. And for complex type,
* only non-null leaf will be counted.
*
*
* int64 value_count = 2;
* @return Whether the valueCount field is set.
*/
public boolean hasValueCount() {
return valueCase_ == 2;
}
/**
*
* For storage, value_count not includes null data. And for complex type,
* only non-null leaf will be counted.
*
*
* int64 value_count = 2;
* @return The valueCount.
*/
public long getValueCount() {
if (valueCase_ == 2) {
return (java.lang.Long) value_;
}
return 0L;
}
/**
*
* For storage, value_count not includes null data. And for complex type,
* only non-null leaf will be counted.
*
*
* int64 value_count = 2;
* @param value The valueCount to set.
* @return This builder for chaining.
*/
public Builder setValueCount(long value) {
valueCase_ = 2;
value_ = value;
onChanged();
return this;
}
/**
*
* For storage, value_count not includes null data. And for complex type,
* only non-null leaf will be counted.
*
*
* int64 value_count = 2;
* @return This builder for chaining.
*/
public Builder clearValueCount() {
if (valueCase_ == 2) {
valueCase_ = 0;
value_ = null;
onChanged();
}
return this;
}
/**
* int64 null_count = 3;
* @return Whether the nullCount field is set.
*/
public boolean hasNullCount() {
return valueCase_ == 3;
}
/**
* int64 null_count = 3;
* @return The nullCount.
*/
public long getNullCount() {
if (valueCase_ == 3) {
return (java.lang.Long) value_;
}
return 0L;
}
/**
* int64 null_count = 3;
* @param value The nullCount to set.
* @return This builder for chaining.
*/
public Builder setNullCount(long value) {
valueCase_ = 3;
value_ = value;
onChanged();
return this;
}
/**
* int64 null_count = 3;
* @return This builder for chaining.
*/
public Builder clearNullCount() {
if (valueCase_ == 3) {
valueCase_ = 0;
value_ = null;
onChanged();
}
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.Constant, cz.proto.Expression.Constant.Builder, cz.proto.Expression.ConstantOrBuilder> lowerBoundsBuilder_;
/**
* .cz.proto.Constant lower_bounds = 4;
* @return Whether the lowerBounds field is set.
*/
@java.lang.Override
public boolean hasLowerBounds() {
return valueCase_ == 4;
}
/**
* .cz.proto.Constant lower_bounds = 4;
* @return The lowerBounds.
*/
@java.lang.Override
public cz.proto.Expression.Constant getLowerBounds() {
if (lowerBoundsBuilder_ == null) {
if (valueCase_ == 4) {
return (cz.proto.Expression.Constant) value_;
}
return cz.proto.Expression.Constant.getDefaultInstance();
} else {
if (valueCase_ == 4) {
return lowerBoundsBuilder_.getMessage();
}
return cz.proto.Expression.Constant.getDefaultInstance();
}
}
/**
* .cz.proto.Constant lower_bounds = 4;
*/
public Builder setLowerBounds(cz.proto.Expression.Constant value) {
if (lowerBoundsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
value_ = value;
onChanged();
} else {
lowerBoundsBuilder_.setMessage(value);
}
valueCase_ = 4;
return this;
}
/**
* .cz.proto.Constant lower_bounds = 4;
*/
public Builder setLowerBounds(
cz.proto.Expression.Constant.Builder builderForValue) {
if (lowerBoundsBuilder_ == null) {
value_ = builderForValue.build();
onChanged();
} else {
lowerBoundsBuilder_.setMessage(builderForValue.build());
}
valueCase_ = 4;
return this;
}
/**
* .cz.proto.Constant lower_bounds = 4;
*/
public Builder mergeLowerBounds(cz.proto.Expression.Constant value) {
if (lowerBoundsBuilder_ == null) {
if (valueCase_ == 4 &&
value_ != cz.proto.Expression.Constant.getDefaultInstance()) {
value_ = cz.proto.Expression.Constant.newBuilder((cz.proto.Expression.Constant) value_)
.mergeFrom(value).buildPartial();
} else {
value_ = value;
}
onChanged();
} else {
if (valueCase_ == 4) {
lowerBoundsBuilder_.mergeFrom(value);
}
lowerBoundsBuilder_.setMessage(value);
}
valueCase_ = 4;
return this;
}
/**
* .cz.proto.Constant lower_bounds = 4;
*/
public Builder clearLowerBounds() {
if (lowerBoundsBuilder_ == null) {
if (valueCase_ == 4) {
valueCase_ = 0;
value_ = null;
onChanged();
}
} else {
if (valueCase_ == 4) {
valueCase_ = 0;
value_ = null;
}
lowerBoundsBuilder_.clear();
}
return this;
}
/**
* .cz.proto.Constant lower_bounds = 4;
*/
public cz.proto.Expression.Constant.Builder getLowerBoundsBuilder() {
return getLowerBoundsFieldBuilder().getBuilder();
}
/**
* .cz.proto.Constant lower_bounds = 4;
*/
@java.lang.Override
public cz.proto.Expression.ConstantOrBuilder getLowerBoundsOrBuilder() {
if ((valueCase_ == 4) && (lowerBoundsBuilder_ != null)) {
return lowerBoundsBuilder_.getMessageOrBuilder();
} else {
if (valueCase_ == 4) {
return (cz.proto.Expression.Constant) value_;
}
return cz.proto.Expression.Constant.getDefaultInstance();
}
}
/**
* .cz.proto.Constant lower_bounds = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.Constant, cz.proto.Expression.Constant.Builder, cz.proto.Expression.ConstantOrBuilder>
getLowerBoundsFieldBuilder() {
if (lowerBoundsBuilder_ == null) {
if (!(valueCase_ == 4)) {
value_ = cz.proto.Expression.Constant.getDefaultInstance();
}
lowerBoundsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.Constant, cz.proto.Expression.Constant.Builder, cz.proto.Expression.ConstantOrBuilder>(
(cz.proto.Expression.Constant) value_,
getParentForChildren(),
isClean());
value_ = null;
}
valueCase_ = 4;
onChanged();;
return lowerBoundsBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.Constant, cz.proto.Expression.Constant.Builder, cz.proto.Expression.ConstantOrBuilder> upperBoundsBuilder_;
/**
* .cz.proto.Constant upper_bounds = 5;
* @return Whether the upperBounds field is set.
*/
@java.lang.Override
public boolean hasUpperBounds() {
return valueCase_ == 5;
}
/**
* .cz.proto.Constant upper_bounds = 5;
* @return The upperBounds.
*/
@java.lang.Override
public cz.proto.Expression.Constant getUpperBounds() {
if (upperBoundsBuilder_ == null) {
if (valueCase_ == 5) {
return (cz.proto.Expression.Constant) value_;
}
return cz.proto.Expression.Constant.getDefaultInstance();
} else {
if (valueCase_ == 5) {
return upperBoundsBuilder_.getMessage();
}
return cz.proto.Expression.Constant.getDefaultInstance();
}
}
/**
* .cz.proto.Constant upper_bounds = 5;
*/
public Builder setUpperBounds(cz.proto.Expression.Constant value) {
if (upperBoundsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
value_ = value;
onChanged();
} else {
upperBoundsBuilder_.setMessage(value);
}
valueCase_ = 5;
return this;
}
/**
* .cz.proto.Constant upper_bounds = 5;
*/
public Builder setUpperBounds(
cz.proto.Expression.Constant.Builder builderForValue) {
if (upperBoundsBuilder_ == null) {
value_ = builderForValue.build();
onChanged();
} else {
upperBoundsBuilder_.setMessage(builderForValue.build());
}
valueCase_ = 5;
return this;
}
/**
* .cz.proto.Constant upper_bounds = 5;
*/
public Builder mergeUpperBounds(cz.proto.Expression.Constant value) {
if (upperBoundsBuilder_ == null) {
if (valueCase_ == 5 &&
value_ != cz.proto.Expression.Constant.getDefaultInstance()) {
value_ = cz.proto.Expression.Constant.newBuilder((cz.proto.Expression.Constant) value_)
.mergeFrom(value).buildPartial();
} else {
value_ = value;
}
onChanged();
} else {
if (valueCase_ == 5) {
upperBoundsBuilder_.mergeFrom(value);
}
upperBoundsBuilder_.setMessage(value);
}
valueCase_ = 5;
return this;
}
/**
* .cz.proto.Constant upper_bounds = 5;
*/
public Builder clearUpperBounds() {
if (upperBoundsBuilder_ == null) {
if (valueCase_ == 5) {
valueCase_ = 0;
value_ = null;
onChanged();
}
} else {
if (valueCase_ == 5) {
valueCase_ = 0;
value_ = null;
}
upperBoundsBuilder_.clear();
}
return this;
}
/**
* .cz.proto.Constant upper_bounds = 5;
*/
public cz.proto.Expression.Constant.Builder getUpperBoundsBuilder() {
return getUpperBoundsFieldBuilder().getBuilder();
}
/**
* .cz.proto.Constant upper_bounds = 5;
*/
@java.lang.Override
public cz.proto.Expression.ConstantOrBuilder getUpperBoundsOrBuilder() {
if ((valueCase_ == 5) && (upperBoundsBuilder_ != null)) {
return upperBoundsBuilder_.getMessageOrBuilder();
} else {
if (valueCase_ == 5) {
return (cz.proto.Expression.Constant) value_;
}
return cz.proto.Expression.Constant.getDefaultInstance();
}
}
/**
* .cz.proto.Constant upper_bounds = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.Constant, cz.proto.Expression.Constant.Builder, cz.proto.Expression.ConstantOrBuilder>
getUpperBoundsFieldBuilder() {
if (upperBoundsBuilder_ == null) {
if (!(valueCase_ == 5)) {
value_ = cz.proto.Expression.Constant.getDefaultInstance();
}
upperBoundsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Expression.Constant, cz.proto.Expression.Constant.Builder, cz.proto.Expression.ConstantOrBuilder>(
(cz.proto.Expression.Constant) value_,
getParentForChildren(),
isClean());
value_ = null;
}
valueCase_ = 5;
onChanged();;
return upperBoundsBuilder_;
}
/**
* double avg_size = 6;
* @return Whether the avgSize field is set.
*/
public boolean hasAvgSize() {
return valueCase_ == 6;
}
/**
* double avg_size = 6;
* @return The avgSize.
*/
public double getAvgSize() {
if (valueCase_ == 6) {
return (java.lang.Double) value_;
}
return 0D;
}
/**
* double avg_size = 6;
* @param value The avgSize to set.
* @return This builder for chaining.
*/
public Builder setAvgSize(double value) {
valueCase_ = 6;
value_ = value;
onChanged();
return this;
}
/**
* double avg_size = 6;
* @return This builder for chaining.
*/
public Builder clearAvgSize() {
if (valueCase_ == 6) {
valueCase_ = 0;
value_ = null;
onChanged();
}
return this;
}
/**
* int64 max_size = 7;
* @return Whether the maxSize field is set.
*/
public boolean hasMaxSize() {
return valueCase_ == 7;
}
/**
* int64 max_size = 7;
* @return The maxSize.
*/
public long getMaxSize() {
if (valueCase_ == 7) {
return (java.lang.Long) value_;
}
return 0L;
}
/**
* int64 max_size = 7;
* @param value The maxSize to set.
* @return This builder for chaining.
*/
public Builder setMaxSize(long value) {
valueCase_ = 7;
value_ = value;
onChanged();
return this;
}
/**
* int64 max_size = 7;
* @return This builder for chaining.
*/
public Builder clearMaxSize() {
if (valueCase_ == 7) {
valueCase_ = 0;
value_ = null;
onChanged();
}
return this;
}
/**
* int64 compressed_size = 8;
* @return Whether the compressedSize field is set.
*/
public boolean hasCompressedSize() {
return valueCase_ == 8;
}
/**
* int64 compressed_size = 8;
* @return The compressedSize.
*/
public long getCompressedSize() {
if (valueCase_ == 8) {
return (java.lang.Long) value_;
}
return 0L;
}
/**
* int64 compressed_size = 8;
* @param value The compressedSize to set.
* @return This builder for chaining.
*/
public Builder setCompressedSize(long value) {
valueCase_ = 8;
value_ = value;
onChanged();
return this;
}
/**
* int64 compressed_size = 8;
* @return This builder for chaining.
*/
public Builder clearCompressedSize() {
if (valueCase_ == 8) {
valueCase_ = 0;
value_ = null;
onChanged();
}
return this;
}
/**
* int64 distinct_number = 9;
* @return Whether the distinctNumber field is set.
*/
public boolean hasDistinctNumber() {
return valueCase_ == 9;
}
/**
* int64 distinct_number = 9;
* @return The distinctNumber.
*/
public long getDistinctNumber() {
if (valueCase_ == 9) {
return (java.lang.Long) value_;
}
return 0L;
}
/**
* int64 distinct_number = 9;
* @param value The distinctNumber to set.
* @return This builder for chaining.
*/
public Builder setDistinctNumber(long value) {
valueCase_ = 9;
value_ = value;
onChanged();
return this;
}
/**
* int64 distinct_number = 9;
* @return This builder for chaining.
*/
public Builder clearDistinctNumber() {
if (valueCase_ == 9) {
valueCase_ = 0;
value_ = null;
onChanged();
}
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.TopK, cz.proto.TopK.Builder, cz.proto.TopKOrBuilder> topKBuilder_;
/**
* .cz.proto.TopK top_k = 10;
* @return Whether the topK field is set.
*/
@java.lang.Override
public boolean hasTopK() {
return valueCase_ == 10;
}
/**
* .cz.proto.TopK top_k = 10;
* @return The topK.
*/
@java.lang.Override
public cz.proto.TopK getTopK() {
if (topKBuilder_ == null) {
if (valueCase_ == 10) {
return (cz.proto.TopK) value_;
}
return cz.proto.TopK.getDefaultInstance();
} else {
if (valueCase_ == 10) {
return topKBuilder_.getMessage();
}
return cz.proto.TopK.getDefaultInstance();
}
}
/**
* .cz.proto.TopK top_k = 10;
*/
public Builder setTopK(cz.proto.TopK value) {
if (topKBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
value_ = value;
onChanged();
} else {
topKBuilder_.setMessage(value);
}
valueCase_ = 10;
return this;
}
/**
* .cz.proto.TopK top_k = 10;
*/
public Builder setTopK(
cz.proto.TopK.Builder builderForValue) {
if (topKBuilder_ == null) {
value_ = builderForValue.build();
onChanged();
} else {
topKBuilder_.setMessage(builderForValue.build());
}
valueCase_ = 10;
return this;
}
/**
* .cz.proto.TopK top_k = 10;
*/
public Builder mergeTopK(cz.proto.TopK value) {
if (topKBuilder_ == null) {
if (valueCase_ == 10 &&
value_ != cz.proto.TopK.getDefaultInstance()) {
value_ = cz.proto.TopK.newBuilder((cz.proto.TopK) value_)
.mergeFrom(value).buildPartial();
} else {
value_ = value;
}
onChanged();
} else {
if (valueCase_ == 10) {
topKBuilder_.mergeFrom(value);
}
topKBuilder_.setMessage(value);
}
valueCase_ = 10;
return this;
}
/**
* .cz.proto.TopK top_k = 10;
*/
public Builder clearTopK() {
if (topKBuilder_ == null) {
if (valueCase_ == 10) {
valueCase_ = 0;
value_ = null;
onChanged();
}
} else {
if (valueCase_ == 10) {
valueCase_ = 0;
value_ = null;
}
topKBuilder_.clear();
}
return this;
}
/**
* .cz.proto.TopK top_k = 10;
*/
public cz.proto.TopK.Builder getTopKBuilder() {
return getTopKFieldBuilder().getBuilder();
}
/**
* .cz.proto.TopK top_k = 10;
*/
@java.lang.Override
public cz.proto.TopKOrBuilder getTopKOrBuilder() {
if ((valueCase_ == 10) && (topKBuilder_ != null)) {
return topKBuilder_.getMessageOrBuilder();
} else {
if (valueCase_ == 10) {
return (cz.proto.TopK) value_;
}
return cz.proto.TopK.getDefaultInstance();
}
}
/**
* .cz.proto.TopK top_k = 10;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.TopK, cz.proto.TopK.Builder, cz.proto.TopKOrBuilder>
getTopKFieldBuilder() {
if (topKBuilder_ == null) {
if (!(valueCase_ == 10)) {
value_ = cz.proto.TopK.getDefaultInstance();
}
topKBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.TopK, cz.proto.TopK.Builder, cz.proto.TopKOrBuilder>(
(cz.proto.TopK) value_,
getParentForChildren(),
isClean());
value_ = null;
}
valueCase_ = 10;
onChanged();;
return topKBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Histogram, cz.proto.Histogram.Builder, cz.proto.HistogramOrBuilder> histogramBuilder_;
/**
* .cz.proto.Histogram histogram = 11;
* @return Whether the histogram field is set.
*/
@java.lang.Override
public boolean hasHistogram() {
return valueCase_ == 11;
}
/**
* .cz.proto.Histogram histogram = 11;
* @return The histogram.
*/
@java.lang.Override
public cz.proto.Histogram getHistogram() {
if (histogramBuilder_ == null) {
if (valueCase_ == 11) {
return (cz.proto.Histogram) value_;
}
return cz.proto.Histogram.getDefaultInstance();
} else {
if (valueCase_ == 11) {
return histogramBuilder_.getMessage();
}
return cz.proto.Histogram.getDefaultInstance();
}
}
/**
* .cz.proto.Histogram histogram = 11;
*/
public Builder setHistogram(cz.proto.Histogram value) {
if (histogramBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
value_ = value;
onChanged();
} else {
histogramBuilder_.setMessage(value);
}
valueCase_ = 11;
return this;
}
/**
* .cz.proto.Histogram histogram = 11;
*/
public Builder setHistogram(
cz.proto.Histogram.Builder builderForValue) {
if (histogramBuilder_ == null) {
value_ = builderForValue.build();
onChanged();
} else {
histogramBuilder_.setMessage(builderForValue.build());
}
valueCase_ = 11;
return this;
}
/**
* .cz.proto.Histogram histogram = 11;
*/
public Builder mergeHistogram(cz.proto.Histogram value) {
if (histogramBuilder_ == null) {
if (valueCase_ == 11 &&
value_ != cz.proto.Histogram.getDefaultInstance()) {
value_ = cz.proto.Histogram.newBuilder((cz.proto.Histogram) value_)
.mergeFrom(value).buildPartial();
} else {
value_ = value;
}
onChanged();
} else {
if (valueCase_ == 11) {
histogramBuilder_.mergeFrom(value);
}
histogramBuilder_.setMessage(value);
}
valueCase_ = 11;
return this;
}
/**
* .cz.proto.Histogram histogram = 11;
*/
public Builder clearHistogram() {
if (histogramBuilder_ == null) {
if (valueCase_ == 11) {
valueCase_ = 0;
value_ = null;
onChanged();
}
} else {
if (valueCase_ == 11) {
valueCase_ = 0;
value_ = null;
}
histogramBuilder_.clear();
}
return this;
}
/**
* .cz.proto.Histogram histogram = 11;
*/
public cz.proto.Histogram.Builder getHistogramBuilder() {
return getHistogramFieldBuilder().getBuilder();
}
/**
* .cz.proto.Histogram histogram = 11;
*/
@java.lang.Override
public cz.proto.HistogramOrBuilder getHistogramOrBuilder() {
if ((valueCase_ == 11) && (histogramBuilder_ != null)) {
return histogramBuilder_.getMessageOrBuilder();
} else {
if (valueCase_ == 11) {
return (cz.proto.Histogram) value_;
}
return cz.proto.Histogram.getDefaultInstance();
}
}
/**
* .cz.proto.Histogram histogram = 11;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Histogram, cz.proto.Histogram.Builder, cz.proto.HistogramOrBuilder>
getHistogramFieldBuilder() {
if (histogramBuilder_ == null) {
if (!(valueCase_ == 11)) {
value_ = cz.proto.Histogram.getDefaultInstance();
}
histogramBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Histogram, cz.proto.Histogram.Builder, cz.proto.HistogramOrBuilder>(
(cz.proto.Histogram) value_,
getParentForChildren(),
isClean());
value_ = null;
}
valueCase_ = 11;
onChanged();;
return histogramBuilder_;
}
/**
*
* For storage, Size in bytes of columnar data before any encoding or compression.
* * For fix-sized type, it's always `value_count * parquet::GetTypeByteSize(T)`,
* value_count includes null data.
* * For variable-length type, it's `value_count * parquet::GetTypeByteSize(T)
* + variable-length`.
* For complex type, leaf will be counted, and non-leaf types would be the
* sum of all leaf-type raw size.
*
*
* int64 raw_size_in_bytes = 12;
* @return Whether the rawSizeInBytes field is set.
*/
public boolean hasRawSizeInBytes() {
return valueCase_ == 12;
}
/**
*
* For storage, Size in bytes of columnar data before any encoding or compression.
* * For fix-sized type, it's always `value_count * parquet::GetTypeByteSize(T)`,
* value_count includes null data.
* * For variable-length type, it's `value_count * parquet::GetTypeByteSize(T)
* + variable-length`.
* For complex type, leaf will be counted, and non-leaf types would be the
* sum of all leaf-type raw size.
*
*
* int64 raw_size_in_bytes = 12;
* @return The rawSizeInBytes.
*/
public long getRawSizeInBytes() {
if (valueCase_ == 12) {
return (java.lang.Long) value_;
}
return 0L;
}
/**
*
* For storage, Size in bytes of columnar data before any encoding or compression.
* * For fix-sized type, it's always `value_count * parquet::GetTypeByteSize(T)`,
* value_count includes null data.
* * For variable-length type, it's `value_count * parquet::GetTypeByteSize(T)
* + variable-length`.
* For complex type, leaf will be counted, and non-leaf types would be the
* sum of all leaf-type raw size.
*
*
* int64 raw_size_in_bytes = 12;
* @param value The rawSizeInBytes to set.
* @return This builder for chaining.
*/
public Builder setRawSizeInBytes(long value) {
valueCase_ = 12;
value_ = value;
onChanged();
return this;
}
/**
*
* For storage, Size in bytes of columnar data before any encoding or compression.
* * For fix-sized type, it's always `value_count * parquet::GetTypeByteSize(T)`,
* value_count includes null data.
* * For variable-length type, it's `value_count * parquet::GetTypeByteSize(T)
* + variable-length`.
* For complex type, leaf will be counted, and non-leaf types would be the
* sum of all leaf-type raw size.
*
*
* int64 raw_size_in_bytes = 12;
* @return This builder for chaining.
*/
public Builder clearRawSizeInBytes() {
if (valueCase_ == 12) {
valueCase_ = 0;
value_ = null;
onChanged();
}
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.FieldStatsValue)
}
// @@protoc_insertion_point(class_scope:cz.proto.FieldStatsValue)
private static final cz.proto.FieldStatsValue DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.FieldStatsValue();
}
public static cz.proto.FieldStatsValue getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public FieldStatsValue parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new FieldStatsValue(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.FieldStatsValue getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy