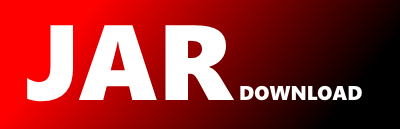
cz.proto.OperatorOrBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickzetta-java Show documentation
Show all versions of clickzetta-java Show documentation
The java SDK for clickzetta's Lakehouse
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: operator.proto
package cz.proto;
public interface OperatorOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.Operator)
com.google.protobuf.MessageOrBuilder {
/**
* string id = 1;
* @return The id.
*/
java.lang.String getId();
/**
* string id = 1;
* @return The bytes for id.
*/
com.google.protobuf.ByteString
getIdBytes();
/**
* repeated string inputIds = 2;
* @return A list containing the inputIds.
*/
java.util.List
getInputIdsList();
/**
* repeated string inputIds = 2;
* @return The count of inputIds.
*/
int getInputIdsCount();
/**
* repeated string inputIds = 2;
* @param index The index of the element to return.
* @return The inputIds at the given index.
*/
java.lang.String getInputIds(int index);
/**
* repeated string inputIds = 2;
* @param index The index of the value to return.
* @return The bytes of the inputIds at the given index.
*/
com.google.protobuf.ByteString
getInputIdsBytes(int index);
/**
* .cz.proto.DataType schema = 3;
* @return Whether the schema field is set.
*/
boolean hasSchema();
/**
* .cz.proto.DataType schema = 3;
* @return The schema.
*/
cz.proto.DataType getSchema();
/**
* .cz.proto.DataType schema = 3;
*/
cz.proto.DataTypeOrBuilder getSchemaOrBuilder();
/**
* repeated .cz.proto.ColumnMapping columnMappings = 4;
*/
java.util.List
getColumnMappingsList();
/**
* repeated .cz.proto.ColumnMapping columnMappings = 4;
*/
cz.proto.ColumnMapping getColumnMappings(int index);
/**
* repeated .cz.proto.ColumnMapping columnMappings = 4;
*/
int getColumnMappingsCount();
/**
* repeated .cz.proto.ColumnMapping columnMappings = 4;
*/
java.util.List extends cz.proto.ColumnMappingOrBuilder>
getColumnMappingsOrBuilderList();
/**
* repeated .cz.proto.ColumnMapping columnMappings = 4;
*/
cz.proto.ColumnMappingOrBuilder getColumnMappingsOrBuilder(
int index);
/**
* uint64 signature = 5;
* @return The signature.
*/
long getSignature();
/**
* uint32 traits = 6;
* @return The traits.
*/
int getTraits();
/**
* .cz.proto.TableScan table_scan = 10;
* @return Whether the tableScan field is set.
*/
boolean hasTableScan();
/**
* .cz.proto.TableScan table_scan = 10;
* @return The tableScan.
*/
cz.proto.TableScan getTableScan();
/**
* .cz.proto.TableScan table_scan = 10;
*/
cz.proto.TableScanOrBuilder getTableScanOrBuilder();
/**
* .cz.proto.TableSink table_sink = 11;
* @return Whether the tableSink field is set.
*/
boolean hasTableSink();
/**
* .cz.proto.TableSink table_sink = 11;
* @return The tableSink.
*/
cz.proto.TableSink getTableSink();
/**
* .cz.proto.TableSink table_sink = 11;
*/
cz.proto.TableSinkOrBuilder getTableSinkOrBuilder();
/**
* .cz.proto.Calc calc = 12;
* @return Whether the calc field is set.
*/
boolean hasCalc();
/**
* .cz.proto.Calc calc = 12;
* @return The calc.
*/
cz.proto.Calc getCalc();
/**
* .cz.proto.Calc calc = 12;
*/
cz.proto.CalcOrBuilder getCalcOrBuilder();
/**
* .cz.proto.MergeSort merge_sort = 13;
* @return Whether the mergeSort field is set.
*/
boolean hasMergeSort();
/**
* .cz.proto.MergeSort merge_sort = 13;
* @return The mergeSort.
*/
cz.proto.MergeSort getMergeSort();
/**
* .cz.proto.MergeSort merge_sort = 13;
*/
cz.proto.MergeSortOrBuilder getMergeSortOrBuilder();
/**
* .cz.proto.ShuffleWrite shuffle_write = 14;
* @return Whether the shuffleWrite field is set.
*/
boolean hasShuffleWrite();
/**
* .cz.proto.ShuffleWrite shuffle_write = 14;
* @return The shuffleWrite.
*/
cz.proto.ShuffleWrite getShuffleWrite();
/**
* .cz.proto.ShuffleWrite shuffle_write = 14;
*/
cz.proto.ShuffleWriteOrBuilder getShuffleWriteOrBuilder();
/**
* .cz.proto.ShuffleRead shuffle_read = 15;
* @return Whether the shuffleRead field is set.
*/
boolean hasShuffleRead();
/**
* .cz.proto.ShuffleRead shuffle_read = 15;
* @return The shuffleRead.
*/
cz.proto.ShuffleRead getShuffleRead();
/**
* .cz.proto.ShuffleRead shuffle_read = 15;
*/
cz.proto.ShuffleReadOrBuilder getShuffleReadOrBuilder();
/**
* .cz.proto.Values values = 16;
* @return Whether the values field is set.
*/
boolean hasValues();
/**
* .cz.proto.Values values = 16;
* @return The values.
*/
cz.proto.Values getValues();
/**
* .cz.proto.Values values = 16;
*/
cz.proto.ValuesOrBuilder getValuesOrBuilder();
/**
* .cz.proto.HashAggregate hash_agg = 17;
* @return Whether the hashAgg field is set.
*/
boolean hasHashAgg();
/**
* .cz.proto.HashAggregate hash_agg = 17;
* @return The hashAgg.
*/
cz.proto.HashAggregate getHashAgg();
/**
* .cz.proto.HashAggregate hash_agg = 17;
*/
cz.proto.HashAggregateOrBuilder getHashAggOrBuilder();
/**
* .cz.proto.SortedAggregate sorted_agg = 18;
* @return Whether the sortedAgg field is set.
*/
boolean hasSortedAgg();
/**
* .cz.proto.SortedAggregate sorted_agg = 18;
* @return The sortedAgg.
*/
cz.proto.SortedAggregate getSortedAgg();
/**
* .cz.proto.SortedAggregate sorted_agg = 18;
*/
cz.proto.SortedAggregateOrBuilder getSortedAggOrBuilder();
/**
* .cz.proto.SortMergeJoin merge_join = 19;
* @return Whether the mergeJoin field is set.
*/
boolean hasMergeJoin();
/**
* .cz.proto.SortMergeJoin merge_join = 19;
* @return The mergeJoin.
*/
cz.proto.SortMergeJoin getMergeJoin();
/**
* .cz.proto.SortMergeJoin merge_join = 19;
*/
cz.proto.SortMergeJoinOrBuilder getMergeJoinOrBuilder();
/**
* .cz.proto.HashJoin hash_join = 20;
* @return Whether the hashJoin field is set.
*/
boolean hasHashJoin();
/**
* .cz.proto.HashJoin hash_join = 20;
* @return The hashJoin.
*/
cz.proto.HashJoin getHashJoin();
/**
* .cz.proto.HashJoin hash_join = 20;
*/
cz.proto.HashJoinOrBuilder getHashJoinOrBuilder();
/**
* .cz.proto.LocalSort local_sort = 21;
* @return Whether the localSort field is set.
*/
boolean hasLocalSort();
/**
* .cz.proto.LocalSort local_sort = 21;
* @return The localSort.
*/
cz.proto.LocalSort getLocalSort();
/**
* .cz.proto.LocalSort local_sort = 21;
*/
cz.proto.LocalSortOrBuilder getLocalSortOrBuilder();
/**
* .cz.proto.UnionAll union_all = 22;
* @return Whether the unionAll field is set.
*/
boolean hasUnionAll();
/**
* .cz.proto.UnionAll union_all = 22;
* @return The unionAll.
*/
cz.proto.UnionAll getUnionAll();
/**
* .cz.proto.UnionAll union_all = 22;
*/
cz.proto.UnionAllOrBuilder getUnionAllOrBuilder();
/**
* .cz.proto.Buffer buffer = 23;
* @return Whether the buffer field is set.
*/
boolean hasBuffer();
/**
* .cz.proto.Buffer buffer = 23;
* @return The buffer.
*/
cz.proto.Buffer getBuffer();
/**
* .cz.proto.Buffer buffer = 23;
*/
cz.proto.BufferOrBuilder getBufferOrBuilder();
/**
* .cz.proto.Window window = 24;
* @return Whether the window field is set.
*/
boolean hasWindow();
/**
* .cz.proto.Window window = 24;
* @return The window.
*/
cz.proto.Window getWindow();
/**
* .cz.proto.Window window = 24;
*/
cz.proto.WindowOrBuilder getWindowOrBuilder();
/**
* .cz.proto.Expand expand = 25;
* @return Whether the expand field is set.
*/
boolean hasExpand();
/**
* .cz.proto.Expand expand = 25;
* @return The expand.
*/
cz.proto.Expand getExpand();
/**
* .cz.proto.Expand expand = 25;
*/
cz.proto.ExpandOrBuilder getExpandOrBuilder();
/**
* .cz.proto.LateralView lateral_view = 26;
* @return Whether the lateralView field is set.
*/
boolean hasLateralView();
/**
* .cz.proto.LateralView lateral_view = 26;
* @return The lateralView.
*/
cz.proto.LateralView getLateralView();
/**
* .cz.proto.LateralView lateral_view = 26;
*/
cz.proto.LateralViewOrBuilder getLateralViewOrBuilder();
/**
* .cz.proto.Grouping grouping = 27;
* @return Whether the grouping field is set.
*/
boolean hasGrouping();
/**
* .cz.proto.Grouping grouping = 27;
* @return The grouping.
*/
cz.proto.Grouping getGrouping();
/**
* .cz.proto.Grouping grouping = 27;
*/
cz.proto.GroupingOrBuilder getGroupingOrBuilder();
/**
* .cz.proto.LogicalJoin join = 50;
* @return Whether the join field is set.
*/
boolean hasJoin();
/**
* .cz.proto.LogicalJoin join = 50;
* @return The join.
*/
cz.proto.LogicalJoin getJoin();
/**
* .cz.proto.LogicalJoin join = 50;
*/
cz.proto.LogicalJoinOrBuilder getJoinOrBuilder();
/**
* .cz.proto.LogicalAggregate aggregate = 51;
* @return Whether the aggregate field is set.
*/
boolean hasAggregate();
/**
* .cz.proto.LogicalAggregate aggregate = 51;
* @return The aggregate.
*/
cz.proto.LogicalAggregate getAggregate();
/**
* .cz.proto.LogicalAggregate aggregate = 51;
*/
cz.proto.LogicalAggregateOrBuilder getAggregateOrBuilder();
/**
* .cz.proto.LogicalCalc logical_calc = 52;
* @return Whether the logicalCalc field is set.
*/
boolean hasLogicalCalc();
/**
* .cz.proto.LogicalCalc logical_calc = 52;
* @return The logicalCalc.
*/
cz.proto.LogicalCalc getLogicalCalc();
/**
* .cz.proto.LogicalCalc logical_calc = 52;
*/
cz.proto.LogicalCalcOrBuilder getLogicalCalcOrBuilder();
/**
* .cz.proto.LogicalSort logical_sort = 53;
* @return Whether the logicalSort field is set.
*/
boolean hasLogicalSort();
/**
* .cz.proto.LogicalSort logical_sort = 53;
* @return The logicalSort.
*/
cz.proto.LogicalSort getLogicalSort();
/**
* .cz.proto.LogicalSort logical_sort = 53;
*/
cz.proto.LogicalSortOrBuilder getLogicalSortOrBuilder();
/**
* .cz.proto.SetOperator set_operator = 54;
* @return Whether the setOperator field is set.
*/
boolean hasSetOperator();
/**
* .cz.proto.SetOperator set_operator = 54;
* @return The setOperator.
*/
cz.proto.SetOperator getSetOperator();
/**
* .cz.proto.SetOperator set_operator = 54;
*/
cz.proto.SetOperatorOrBuilder getSetOperatorOrBuilder();
/**
* .cz.proto.AggregatePhase agg_phase = 55;
* @return Whether the aggPhase field is set.
*/
boolean hasAggPhase();
/**
* .cz.proto.AggregatePhase agg_phase = 55;
* @return The aggPhase.
*/
cz.proto.AggregatePhase getAggPhase();
/**
* .cz.proto.AggregatePhase agg_phase = 55;
*/
cz.proto.AggregatePhaseOrBuilder getAggPhaseOrBuilder();
/**
* .cz.proto.Spool spool = 56;
* @return Whether the spool field is set.
*/
boolean hasSpool();
/**
* .cz.proto.Spool spool = 56;
* @return The spool.
*/
cz.proto.Spool getSpool();
/**
* .cz.proto.Spool spool = 56;
*/
cz.proto.SpoolOrBuilder getSpoolOrBuilder();
/**
* .cz.proto.PartialWindowFilter partial_window_filter = 57;
* @return Whether the partialWindowFilter field is set.
*/
boolean hasPartialWindowFilter();
/**
* .cz.proto.PartialWindowFilter partial_window_filter = 57;
* @return The partialWindowFilter.
*/
cz.proto.PartialWindowFilter getPartialWindowFilter();
/**
* .cz.proto.PartialWindowFilter partial_window_filter = 57;
*/
cz.proto.PartialWindowFilterOrBuilder getPartialWindowFilterOrBuilder();
/**
* .cz.proto.TreeJoin tree_join = 58;
* @return Whether the treeJoin field is set.
*/
boolean hasTreeJoin();
/**
* .cz.proto.TreeJoin tree_join = 58;
* @return The treeJoin.
*/
cz.proto.TreeJoin getTreeJoin();
/**
* .cz.proto.TreeJoin tree_join = 58;
*/
cz.proto.TreeJoinOrBuilder getTreeJoinOrBuilder();
/**
* .cz.proto.TreeJoinLeaf tree_join_leaf = 59;
* @return Whether the treeJoinLeaf field is set.
*/
boolean hasTreeJoinLeaf();
/**
* .cz.proto.TreeJoinLeaf tree_join_leaf = 59;
* @return The treeJoinLeaf.
*/
cz.proto.TreeJoinLeaf getTreeJoinLeaf();
/**
* .cz.proto.TreeJoinLeaf tree_join_leaf = 59;
*/
cz.proto.TreeJoinLeafOrBuilder getTreeJoinLeafOrBuilder();
/**
* .cz.proto.LocalExchange local_exchange = 60;
* @return Whether the localExchange field is set.
*/
boolean hasLocalExchange();
/**
* .cz.proto.LocalExchange local_exchange = 60;
* @return The localExchange.
*/
cz.proto.LocalExchange getLocalExchange();
/**
* .cz.proto.LocalExchange local_exchange = 60;
*/
cz.proto.LocalExchangeOrBuilder getLocalExchangeOrBuilder();
/**
* .cz.proto.ParseTreeInfo pt = 100;
* @return Whether the pt field is set.
*/
boolean hasPt();
/**
* .cz.proto.ParseTreeInfo pt = 100;
* @return The pt.
*/
cz.proto.Expression.ParseTreeInfo getPt();
/**
* .cz.proto.ParseTreeInfo pt = 100;
*/
cz.proto.Expression.ParseTreeInfoOrBuilder getPtOrBuilder();
public cz.proto.Operator.OpCase getOpCase();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy